mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-16 00:42:51 +08:00
2.0 KiB
2.0 KiB
Title: Progress Order: 5
Spectre.Console can display information about long running tasks in the console.

If the current terminal isn't considered "interactive", such as when running in a continuous integration system, or the terminal can't display ANSI control sequence, any progress will be displayed in a simpler way.
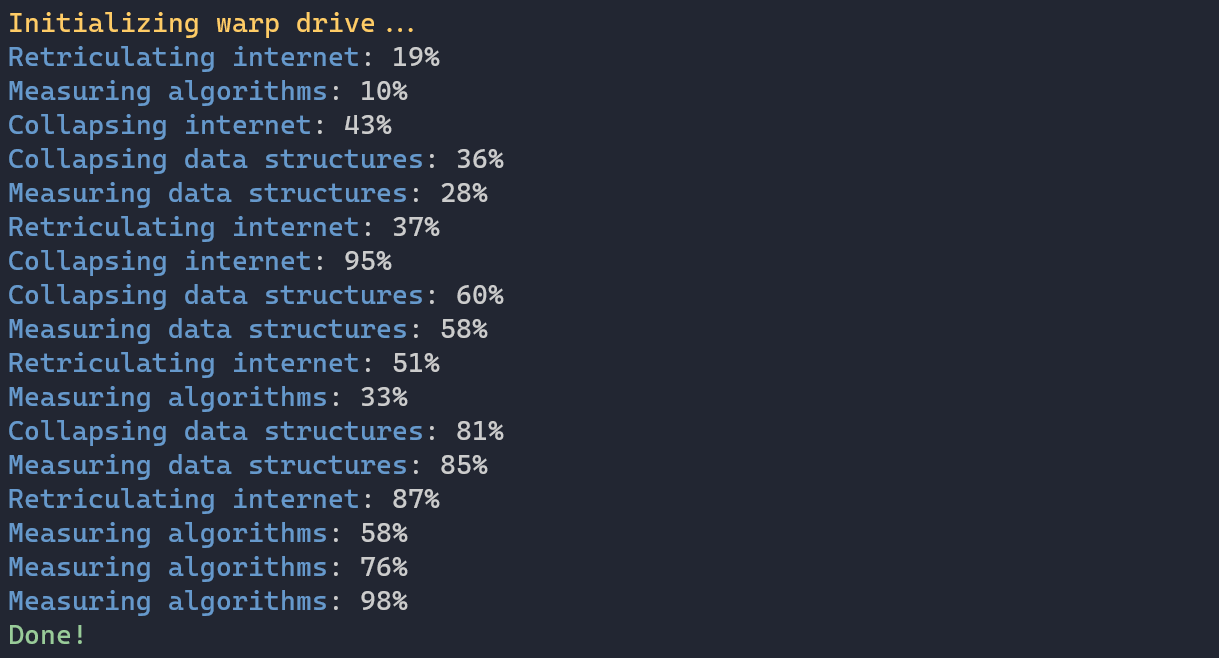
Usage
// Synchronous
AnsiConsole.Progress()
.Start(ctx =>
{
// Define tasks
var task1 = ctx.AddTask("[green]Reticulating splines[/]");
var task2 = ctx.AddTask("[green]Folding space[/]");
while(!ctx.IsFinished)
{
task1.Increment(1.5);
task2.Increment(0.5);
}
});
Asynchronous progress
If you prefer to use async/await, you can use StartAsync
instead of Start
.
// Asynchronous
await AnsiConsole.Progress()
.StartAsync(async ctx =>
{
// Define tasks
var task1 = ctx.AddTask("[green]Reticulating splines[/]");
var task2 = ctx.AddTask("[green]Folding space[/]");
while (!ctx.IsFinished)
{
// Simulate some work
await Task.Delay(250);
// Increment
task1.Increment(1.5);
task2.Increment(0.5);
}
});
Configure
// Asynchronous
AnsiConsole.Progress()
.AutoRefresh(false) // Turn off auto refresh
.AutoClear(false) // Do not remove the task list when done
.HideCompleted(false) // Hide tasks as they are completed
.Columns(new ProgressColumn[]
{
new TaskDescriptionColumn(), // Task description
new ProgressBarColumn(), // Progress bar
new PercentageColumn(), // Percentage
new RemainingTimeColumn(), // Remaining time
new SpinnerColumn(), // Spinner
})
.Start(ctx =>
{
// Omitted
});