mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-14 16:02:50 +08:00
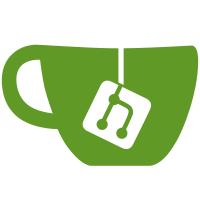
* Adding social card infrastructure * Upgrades doc project to .NET 6 * Adds Playwright * Changes the console to a web project for Playwright * Adds social card template * Added blog content * Parallelized social image processing * Updating CI to use .NET 6 for docs build
2.2 KiB
2.2 KiB
Title: Text prompt Order: 0 RedirectFrom: prompt Description: "Spectre.Console has multiple input functions for helping receive strongly typed input from a user." Highlights: - Confirmation. - Strongly typed input. - Input restricted to specific items. - Secrets such as passwords or keys.
Sometimes you want to get some input from the user, and for this
you can use the Prompt<TResult>
.
The use of prompts insides status or progress displays is not supported.
Confirmation
if (!AnsiConsole.Confirm("Run example?"))
{
return;
}
Run example? [y/n] (y): _
Simple
// Ask for the user's name
string name = AnsiConsole.Ask<string>("What's your [green]name[/]?");
// Ask for the user's age
int age = AnsiConsole.Ask<int>("What's your [green]age[/]?");
What's your name? Patrik
What's your age? 37
Choices
var fruit = AnsiConsole.Prompt(
new TextPrompt<string>("What's your [green]favorite fruit[/]?")
.InvalidChoiceMessage("[red]That's not a valid fruit[/]")
.DefaultValue("Orange")
.AddChoice("Apple")
.AddChoice("Banana")
.AddChoice("Orange"));
What's your favorite fruit? [Apple/Banana/Orange] (Orange): _
Validation
var age = AnsiConsole.Prompt(
new TextPrompt<int>("What's the secret number?")
.Validate(age =>
{
return age switch
{
< 99 => ValidationResult.Error("[red]Too low[/]"),
> 99 => ValidationResult.Error("[red]Too high[/]"),
_ => ValidationResult.Success(),
};
}));
What's the secret number? 32
Too low
What's the secret number? 102
Too high
What's the secret number? _
Secrets
var password = AnsiConsole.Prompt(
new TextPrompt<string>("Enter [green]password[/]")
.PromptStyle("red")
.Secret());
Enter password: ************_
Optional
var color = AnsiConsole.Prompt(
new TextPrompt<string>("[grey][[Optional]][/] [green]Favorite color[/]?")
.AllowEmpty());
[Optional] Favorite color? _