mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-31 09:15:58 +08:00
Throw if markup contains unescaped close tag
This commit is contained in:
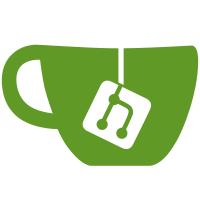
committed by
Patrik Svensson
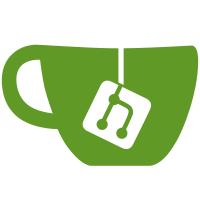
parent
31f117aed0
commit
decb887b0a
40
src/Spectre.Console.Tests/Unit/MarkupTests.cs
Normal file
40
src/Spectre.Console.Tests/Unit/MarkupTests.cs
Normal file
@ -0,0 +1,40 @@
|
||||
using System;
|
||||
using Shouldly;
|
||||
using Xunit;
|
||||
|
||||
namespace Spectre.Console.Tests.Unit
|
||||
{
|
||||
public sealed class MarkupTests
|
||||
{
|
||||
[Theory]
|
||||
[InlineData("Hello [[ World ]")]
|
||||
[InlineData("Hello [[ World ] !")]
|
||||
public void Should_Throw_If_Closing_Tag_Is_Not_Properly_Escaped(string input)
|
||||
{
|
||||
// Given
|
||||
var fixture = new PlainConsole();
|
||||
|
||||
// When
|
||||
var result = Record.Exception(() => new Markup(input));
|
||||
|
||||
// Then
|
||||
result.ShouldNotBeNull();
|
||||
result.ShouldBeOfType<InvalidOperationException>();
|
||||
result.Message.ShouldBe("Encountered unescaped ']' token at position 16");
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Escape_Markup_Blocks_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var fixture = new PlainConsole();
|
||||
var markup = new Markup("Hello [[ World ]] !");
|
||||
|
||||
// When
|
||||
fixture.Render(markup);
|
||||
|
||||
// Then
|
||||
fixture.Output.ShouldBe("Hello [ World ] !");
|
||||
}
|
||||
}
|
||||
}
|
@ -91,6 +91,8 @@ namespace Spectre.Console.Internal
|
||||
{
|
||||
var position = _reader.Position;
|
||||
var builder = new StringBuilder();
|
||||
|
||||
var encounteredClosing = false;
|
||||
while (!_reader.Eof)
|
||||
{
|
||||
current = _reader.Peek();
|
||||
@ -98,10 +100,34 @@ namespace Spectre.Console.Internal
|
||||
{
|
||||
break;
|
||||
}
|
||||
else if (current == ']')
|
||||
{
|
||||
if (encounteredClosing)
|
||||
{
|
||||
_reader.Read();
|
||||
encounteredClosing = false;
|
||||
continue;
|
||||
}
|
||||
|
||||
encounteredClosing = true;
|
||||
}
|
||||
else
|
||||
{
|
||||
if (encounteredClosing)
|
||||
{
|
||||
throw new InvalidOperationException(
|
||||
$"Encountered unescaped ']' token at position {_reader.Position}");
|
||||
}
|
||||
}
|
||||
|
||||
builder.Append(_reader.Read());
|
||||
}
|
||||
|
||||
if (encounteredClosing)
|
||||
{
|
||||
throw new InvalidOperationException($"Encountered unescaped ']' token at position {_reader.Position}");
|
||||
}
|
||||
|
||||
Current = new MarkupToken(MarkupTokenKind.Text, builder.ToString(), position);
|
||||
return true;
|
||||
}
|
||||
|
Reference in New Issue
Block a user