mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-02 02:48:17 +08:00
Clean up border code
Removed caching that really didn't do anything anymore.
This commit is contained in:
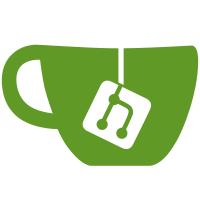
committed by
Patrik Svensson
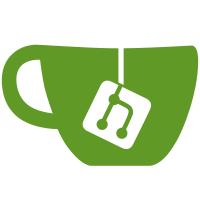
parent
a2f8652575
commit
b1db8a9403
@ -1,7 +1,3 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Globalization;
|
||||
using System.Linq;
|
||||
using Spectre.Console.Rendering;
|
||||
|
||||
namespace Spectre.Console
|
||||
@ -11,70 +7,16 @@ namespace Spectre.Console
|
||||
/// </summary>
|
||||
public abstract partial class BoxBorder
|
||||
{
|
||||
private readonly Dictionary<BoxBorderPart, string> _lookup;
|
||||
|
||||
/// <summary>
|
||||
/// Gets the safe border for this border or <c>null</c> if none exist.
|
||||
/// </summary>
|
||||
public virtual BoxBorder? SafeBorder { get; }
|
||||
|
||||
/// <summary>
|
||||
/// Initializes a new instance of the <see cref="BoxBorder"/> class.
|
||||
/// </summary>
|
||||
protected BoxBorder()
|
||||
{
|
||||
_lookup = Initialize();
|
||||
}
|
||||
|
||||
private Dictionary<BoxBorderPart, string> Initialize()
|
||||
{
|
||||
var lookup = new Dictionary<BoxBorderPart, string>();
|
||||
foreach (BoxBorderPart? part in Enum.GetValues(typeof(BoxBorderPart)))
|
||||
{
|
||||
if (part == null)
|
||||
{
|
||||
continue;
|
||||
}
|
||||
|
||||
var text = GetBorderPart(part.Value);
|
||||
if (text.Length > 1)
|
||||
{
|
||||
throw new InvalidOperationException("A box part cannot contain more than one character.");
|
||||
}
|
||||
|
||||
lookup.Add(part.Value, GetBorderPart(part.Value));
|
||||
}
|
||||
|
||||
return lookup;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the string representation of a specific border part.
|
||||
/// </summary>
|
||||
/// <param name="part">The part to get a string representation for.</param>
|
||||
/// <param name="count">The number of repetitions.</param>
|
||||
/// <returns>A string representation of the specified border part.</returns>
|
||||
public string GetPart(BoxBorderPart part, int count)
|
||||
{
|
||||
// TODO: This need some optimization...
|
||||
return string.Join(string.Empty, Enumerable.Repeat(GetBorderPart(part)[0], count));
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the string representation of a specific border part.
|
||||
/// </summary>
|
||||
/// <param name="part">The part to get a string representation for.</param>
|
||||
/// <returns>A string representation of the specified border part.</returns>
|
||||
public string GetPart(BoxBorderPart part)
|
||||
{
|
||||
return _lookup[part].ToString(CultureInfo.InvariantCulture);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the character representing the specified border part.
|
||||
/// Gets the string representation of the specified border part.
|
||||
/// </summary>
|
||||
/// <param name="part">The part to get the character representation for.</param>
|
||||
/// <returns>A character representation of the specified border part.</returns>
|
||||
protected abstract string GetBorderPart(BoxBorderPart part);
|
||||
public abstract string GetPart(BoxBorderPart part);
|
||||
}
|
||||
}
|
||||
|
@ -81,7 +81,7 @@ namespace Spectre.Console.Internal
|
||||
return result.ToArray();
|
||||
}
|
||||
|
||||
public static string Multiply(this string text, int count)
|
||||
public static string Repeat(this string text, int count)
|
||||
{
|
||||
if (text is null)
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class AsciiBoxBorder : BoxBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(BoxBorderPart part)
|
||||
public override string GetPart(BoxBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class DoubleBoxBorder : BoxBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(BoxBorderPart part)
|
||||
public override string GetPart(BoxBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override BoxBorder? SafeBorder => BoxBorder.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(BoxBorderPart part)
|
||||
public override string GetPart(BoxBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -6,7 +6,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class NoBoxBorder : BoxBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(BoxBorderPart part)
|
||||
public override string GetPart(BoxBorderPart part)
|
||||
{
|
||||
return " ";
|
||||
}
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override BoxBorder? SafeBorder => BoxBorder.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(BoxBorderPart part)
|
||||
public override string GetPart(BoxBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class SquareBoxBorder : BoxBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(BoxBorderPart part)
|
||||
public override string GetPart(BoxBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class Ascii2TableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class AsciiDoubleHeadTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class AsciiTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class DoubleEdgeTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class DoubleTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override TableBorder? SafeBorder => TableBorder.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override TableBorder? SafeBorder => TableBorder.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override TableBorder? SafeBorder => TableBorder.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class HorizontalTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class MarkdownTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
@ -57,39 +57,39 @@ namespace Spectre.Console.Rendering
|
||||
if (padding.Left > 0)
|
||||
{
|
||||
// Left padding
|
||||
builder.Append(" ".Multiply(padding.Left));
|
||||
builder.Append(" ".Repeat(padding.Left));
|
||||
}
|
||||
|
||||
var justification = columns[columnIndex].Alignment;
|
||||
if (justification == null)
|
||||
{
|
||||
// No alignment
|
||||
builder.Append(center.Multiply(columnWidth));
|
||||
builder.Append(center.Repeat(columnWidth));
|
||||
}
|
||||
else if (justification.Value == Justify.Left)
|
||||
{
|
||||
// Left
|
||||
builder.Append(':');
|
||||
builder.Append(center.Multiply(columnWidth - 1));
|
||||
builder.Append(center.Repeat(columnWidth - 1));
|
||||
}
|
||||
else if (justification.Value == Justify.Center)
|
||||
{
|
||||
// Centered
|
||||
builder.Append(':');
|
||||
builder.Append(center.Multiply(columnWidth - 2));
|
||||
builder.Append(center.Repeat(columnWidth - 2));
|
||||
builder.Append(':');
|
||||
}
|
||||
else if (justification.Value == Justify.Right)
|
||||
{
|
||||
// Right
|
||||
builder.Append(center.Multiply(columnWidth - 1));
|
||||
builder.Append(center.Repeat(columnWidth - 1));
|
||||
builder.Append(':');
|
||||
}
|
||||
|
||||
// Right padding
|
||||
if (padding.Right > 0)
|
||||
{
|
||||
builder.Append(" ".Multiply(padding.Right));
|
||||
builder.Append(" ".Repeat(padding.Right));
|
||||
}
|
||||
|
||||
if (!lastColumn)
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class MinimalDoubleHeadTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override TableBorder? SafeBorder => TableBorder.Minimal;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class MinimalTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -9,7 +9,7 @@ namespace Spectre.Console.Rendering
|
||||
public override bool Visible => false;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return " ";
|
||||
}
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override TableBorder? SafeBorder => TableBorder.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -11,7 +11,7 @@ namespace Spectre.Console.Rendering
|
||||
public override TableBorder? SafeBorder => TableBorder.Simple;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class SimpleTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -8,7 +8,7 @@ namespace Spectre.Console.Rendering
|
||||
public sealed class SquareTableBorder : TableBorder
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBorderPart(TableBorderPart part)
|
||||
public override string GetPart(TableBorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -13,8 +13,6 @@ namespace Spectre.Console
|
||||
/// </summary>
|
||||
public abstract partial class TableBorder
|
||||
{
|
||||
private readonly Dictionary<TableBorderPart, string> _lookup;
|
||||
|
||||
/// <summary>
|
||||
/// Gets a value indicating whether or not the border is visible.
|
||||
/// </summary>
|
||||
@ -26,46 +24,11 @@ namespace Spectre.Console
|
||||
public virtual TableBorder? SafeBorder { get; }
|
||||
|
||||
/// <summary>
|
||||
/// Initializes a new instance of the <see cref="TableBorder"/> class.
|
||||
/// Gets the string representation of a specified table border part.
|
||||
/// </summary>
|
||||
protected TableBorder()
|
||||
{
|
||||
_lookup = Initialize();
|
||||
}
|
||||
|
||||
private Dictionary<TableBorderPart, string> Initialize()
|
||||
{
|
||||
var lookup = new Dictionary<TableBorderPart, string>();
|
||||
foreach (TableBorderPart? part in Enum.GetValues(typeof(TableBorderPart)))
|
||||
{
|
||||
if (part == null)
|
||||
{
|
||||
continue;
|
||||
}
|
||||
|
||||
var text = GetBorderPart(part.Value);
|
||||
if (text.Length > 1)
|
||||
{
|
||||
throw new InvalidOperationException("A box part cannot contain more than one character.");
|
||||
}
|
||||
|
||||
lookup.Add(part.Value, GetBorderPart(part.Value));
|
||||
}
|
||||
|
||||
return lookup;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the string representation of a specific border part.
|
||||
/// </summary>
|
||||
/// <param name="part">The part to get a string representation for.</param>
|
||||
/// <param name="count">The number of repetitions.</param>
|
||||
/// <returns>A string representation of the specified border part.</returns>
|
||||
public string GetPart(TableBorderPart part, int count)
|
||||
{
|
||||
// TODO: This need some optimization...
|
||||
return string.Join(string.Empty, Enumerable.Repeat(GetBorderPart(part)[0], count));
|
||||
}
|
||||
/// <param name="part">The part to get the character representation for.</param>
|
||||
/// <returns>A character representation of the specified border part.</returns>
|
||||
public abstract string GetPart(TableBorderPart part);
|
||||
|
||||
/// <summary>
|
||||
/// Gets a whole column row for the specific column row part.
|
||||
@ -95,7 +58,7 @@ namespace Spectre.Console
|
||||
{
|
||||
var padding = columns[columnIndex].Padding;
|
||||
var centerWidth = padding.Left + columnWidth + padding.Right;
|
||||
builder.Append(center.Multiply(centerWidth));
|
||||
builder.Append(center.Repeat(centerWidth));
|
||||
|
||||
if (!lastColumn)
|
||||
{
|
||||
@ -107,30 +70,12 @@ namespace Spectre.Console
|
||||
return builder.ToString();
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the string representation of a specific border part.
|
||||
/// </summary>
|
||||
/// <param name="part">The part to get a string representation for.</param>
|
||||
/// <returns>A string representation of the specified border part.</returns>
|
||||
public string GetPart(TableBorderPart part)
|
||||
{
|
||||
return _lookup[part].ToString(CultureInfo.InvariantCulture);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the character representing the specified border part.
|
||||
/// </summary>
|
||||
/// <param name="part">The part to get the character representation for.</param>
|
||||
/// <returns>A character representation of the specified border part.</returns>
|
||||
protected abstract string GetBorderPart(TableBorderPart part);
|
||||
|
||||
/// <summary>
|
||||
/// Gets the table parts used to render a specific table row.
|
||||
/// </summary>
|
||||
/// <param name="part">The table part.</param>
|
||||
/// <returns>The table parts used to render the specific table row.</returns>
|
||||
protected (string Left, string Center, string Separator, string Right)
|
||||
GetTableParts(TablePart part)
|
||||
protected (string Left, string Center, string Separator, string Right) GetTableParts(TablePart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
|
@ -1,6 +1,7 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Linq;
|
||||
using Spectre.Console.Internal;
|
||||
using Spectre.Console.Rendering;
|
||||
|
||||
namespace Spectre.Console
|
||||
@ -123,7 +124,7 @@ namespace Spectre.Console
|
||||
private static void AddBottomBorder(List<Segment> result, BoxBorder border, Style borderStyle, int panelWidth)
|
||||
{
|
||||
result.Add(new Segment(border.GetPart(BoxBorderPart.BottomLeft), borderStyle));
|
||||
result.Add(new Segment(border.GetPart(BoxBorderPart.Bottom, panelWidth - EdgeWidth), borderStyle));
|
||||
result.Add(new Segment(border.GetPart(BoxBorderPart.Bottom).Repeat(panelWidth - EdgeWidth), borderStyle));
|
||||
result.Add(new Segment(border.GetPart(BoxBorderPart.BottomRight), borderStyle));
|
||||
result.Add(Segment.LineBreak);
|
||||
}
|
||||
@ -160,13 +161,13 @@ namespace Spectre.Console
|
||||
}
|
||||
}
|
||||
|
||||
segments.Add(new Segment(border.GetPart(BoxBorderPart.Top, leftSpacing + 1), borderStyle));
|
||||
segments.Add(new Segment(border.GetPart(BoxBorderPart.Top).Repeat(leftSpacing + 1), borderStyle));
|
||||
segments.Add(header);
|
||||
segments.Add(new Segment(border.GetPart(BoxBorderPart.Top, rightSpacing + 1), borderStyle));
|
||||
segments.Add(new Segment(border.GetPart(BoxBorderPart.Top).Repeat(rightSpacing + 1), borderStyle));
|
||||
}
|
||||
else
|
||||
{
|
||||
segments.Add(new Segment(border.GetPart(BoxBorderPart.Top, panelWidth - EdgeWidth), borderStyle));
|
||||
segments.Add(new Segment(border.GetPart(BoxBorderPart.Top).Repeat(panelWidth - EdgeWidth), borderStyle));
|
||||
}
|
||||
|
||||
segments.Add(new Segment(border.GetPart(BoxBorderPart.TopRight), borderStyle));
|
||||
|
Reference in New Issue
Block a user