mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-08-01 09:45:58 +08:00
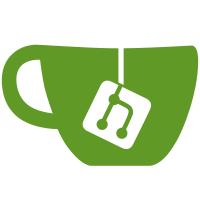
committed by
Patrik Svensson
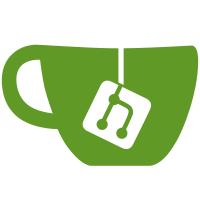
parent
68e92f3365
commit
ae92c606bb
@ -30,6 +30,25 @@ var phrase = "Mmmm :birthday_cake:";
|
||||
var rendered = Emoji.Replace(phrase);
|
||||
```
|
||||
|
||||
# Remapping or adding an emoji
|
||||
|
||||
Sometimes you want to remap an existing emoji, or
|
||||
add a completely new one. For this you can use the
|
||||
`Emoji.Remap` method. This approach works both with
|
||||
markup strings and `Emoji.Replace`.
|
||||
|
||||
```csharp
|
||||
// Remap the emoji
|
||||
Emoji.Remap("globe_showing_europe_africa", "😄");
|
||||
|
||||
// Render markup
|
||||
AnsiConsole.MarkupLine("Hello :globe_showing_europe_africa:!");
|
||||
|
||||
// Replace emojis in string
|
||||
var phrase = "Hello :globe_showing_europe_africa:!";
|
||||
var rendered = Emoji.Replace(phrase);
|
||||
```
|
||||
|
||||
# Emojis
|
||||
|
||||
_The images in the table below might not render correctly in your
|
||||
|
@ -6,11 +6,19 @@ namespace EmojiExample
|
||||
{
|
||||
public static void Main(string[] args)
|
||||
{
|
||||
// Markup
|
||||
// Show a known emoji
|
||||
RenderEmoji();
|
||||
|
||||
// Show a remapped emoji
|
||||
Emoji.Remap("globe_showing_europe_africa", Emoji.Known.GrinningFaceWithSmilingEyes);
|
||||
RenderEmoji();
|
||||
}
|
||||
|
||||
private static void RenderEmoji()
|
||||
{
|
||||
AnsiConsole.Render(
|
||||
new Panel("[yellow]Hello :globe_showing_europe_africa:![/]")
|
||||
.RoundedBorder()
|
||||
.SetHeader("Markup"));
|
||||
.RoundedBorder());
|
||||
}
|
||||
}
|
||||
}
|
||||
|
@ -86,4 +86,7 @@ dotnet_diagnostic.RCS1057.severity = none
|
||||
dotnet_diagnostic.RCS1227.severity = none
|
||||
|
||||
# IDE0004: Remove Unnecessary Cast
|
||||
dotnet_diagnostic.IDE0004.severity = warning
|
||||
dotnet_diagnostic.IDE0004.severity = warning
|
||||
|
||||
# CA1810: Initialize reference type static fields inline
|
||||
dotnet_diagnostic.CA1810.severity = none
|
@ -1,3 +1,5 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Text.RegularExpressions;
|
||||
|
||||
namespace Spectre.Console
|
||||
@ -8,6 +10,35 @@ namespace Spectre.Console
|
||||
public static partial class Emoji
|
||||
{
|
||||
private static readonly Regex _emojiCode = new Regex(@"(:(\S*?):)", RegexOptions.Compiled);
|
||||
private static readonly Dictionary<string, string> _remappings;
|
||||
|
||||
static Emoji()
|
||||
{
|
||||
_remappings = new Dictionary<string, string>(StringComparer.OrdinalIgnoreCase);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Remaps a specific emoji tag with a new emoji.
|
||||
/// </summary>
|
||||
/// <param name="tag">The emoji tag.</param>
|
||||
/// <param name="emoji">The emoji.</param>
|
||||
public static void Remap(string tag, string emoji)
|
||||
{
|
||||
if (tag is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(tag));
|
||||
}
|
||||
|
||||
if (emoji is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(emoji));
|
||||
}
|
||||
|
||||
tag = tag.TrimStart(':').TrimEnd(':');
|
||||
emoji = emoji.TrimStart(':').TrimEnd(':');
|
||||
|
||||
_remappings[tag] = emoji;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Replaces emoji markup with corresponding unicode characters.
|
||||
@ -19,6 +50,12 @@ namespace Spectre.Console
|
||||
static string ReplaceEmoji(Match match)
|
||||
{
|
||||
var key = match.Groups[2].Value;
|
||||
|
||||
if (_remappings.Count > 0 && _remappings.TryGetValue(key, out var remappedEmoji))
|
||||
{
|
||||
return remappedEmoji;
|
||||
}
|
||||
|
||||
if (_emojis.TryGetValue(key, out var emoji))
|
||||
{
|
||||
return emoji;
|
||||
|
Reference in New Issue
Block a user