mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-06 04:28:15 +08:00
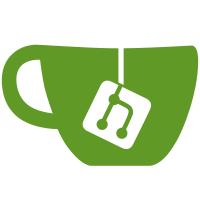
committed by
Phil Scott
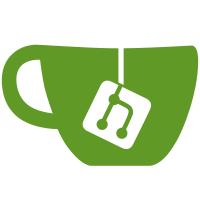
parent
57a8e6ccc1
commit
9502aaf2b9
@ -25,6 +25,22 @@ namespace Spectre.Console.Tests.Unit
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class TheRemoveMethod
|
||||
{
|
||||
[Theory]
|
||||
[InlineData("Hello World", "Hello World")]
|
||||
[InlineData("Hello [blue]World", "Hello World")]
|
||||
[InlineData("Hello [blue]World[/]", "Hello World")]
|
||||
public void Should_Remove_Markup_From_Text(string input, string expected)
|
||||
{
|
||||
// Given, When
|
||||
var result = Markup.Remove(input);
|
||||
|
||||
// Then
|
||||
result.ShouldBe(expected);
|
||||
}
|
||||
}
|
||||
|
||||
[Theory]
|
||||
[InlineData("Hello [[ World ]")]
|
||||
[InlineData("Hello [[ World ] !")]
|
||||
|
@ -34,6 +34,32 @@ namespace Spectre.Console
|
||||
.ReplaceExact("]", "]]");
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Removes markup from the specified string.
|
||||
/// </summary>
|
||||
/// <param name="text">The text to remove markup from.</param>
|
||||
/// <returns>A string that does not have any markup.</returns>
|
||||
public static string RemoveMarkup(this string? text)
|
||||
{
|
||||
if (string.IsNullOrWhiteSpace(text))
|
||||
{
|
||||
return string.Empty;
|
||||
}
|
||||
|
||||
var result = new StringBuilder();
|
||||
|
||||
var tokenizer = new MarkupTokenizer(text);
|
||||
while (tokenizer.MoveNext() && tokenizer.Current != null)
|
||||
{
|
||||
if (tokenizer.Current.Kind == MarkupTokenKind.Text)
|
||||
{
|
||||
result.Append(tokenizer.Current.Value);
|
||||
}
|
||||
}
|
||||
|
||||
return result.ToString();
|
||||
}
|
||||
|
||||
internal static int CellLength(this string text, RenderContext context)
|
||||
{
|
||||
if (context is null)
|
||||
|
@ -63,5 +63,20 @@ namespace Spectre.Console
|
||||
|
||||
return text.EscapeMarkup();
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Removes markup from the specified string.
|
||||
/// </summary>
|
||||
/// <param name="text">The text to remove markup from.</param>
|
||||
/// <returns>A string that does not have any markup.</returns>
|
||||
public static string Remove(string text)
|
||||
{
|
||||
if (text is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(text));
|
||||
}
|
||||
|
||||
return text.RemoveMarkup();
|
||||
}
|
||||
}
|
||||
}
|
||||
|
@ -81,10 +81,11 @@ namespace Spectre.Console
|
||||
var checkbox = selected ? SelectedCheckbox : Checkbox;
|
||||
|
||||
var style = current ? _highlightStyle : Style.Plain;
|
||||
var item = current
|
||||
? new Text(choice.Item.RemoveMarkup(), style)
|
||||
: (IRenderable)new Markup(choice.Item, style);
|
||||
|
||||
grid.AddRow(
|
||||
new Markup($"{prompt}{checkbox}", style),
|
||||
new Markup(choice.Item.EscapeMarkup(), style));
|
||||
grid.AddRow(new Markup(prompt + checkbox, style), item);
|
||||
}
|
||||
|
||||
list.Add(grid);
|
||||
|
@ -56,9 +56,11 @@ namespace Spectre.Console
|
||||
var prompt = choice.Index == pointerIndex ? Prompt : string.Empty;
|
||||
var style = current ? _highlightStyle : Style.Plain;
|
||||
|
||||
grid.AddRow(
|
||||
new Markup(prompt, style),
|
||||
new Markup(choice.Item.EscapeMarkup(), style));
|
||||
var item = current
|
||||
? new Text(choice.Item.RemoveMarkup(), style)
|
||||
: (IRenderable)new Markup(choice.Item, style);
|
||||
|
||||
grid.AddRow(new Markup(prompt, style), item);
|
||||
}
|
||||
|
||||
list.Add(grid);
|
||||
|
Reference in New Issue
Block a user