mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-08-01 09:45:58 +08:00
Get color names from lookup table
Also adds tests for Color struct and fixes a bug that had to do with equality.
This commit is contained in:
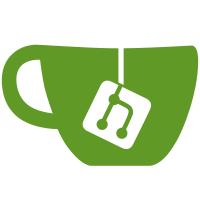
committed by
Patrik Svensson
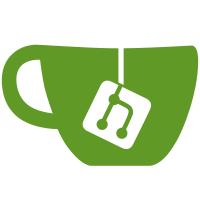
parent
f19202b427
commit
5267ebda49
240
src/Spectre.Console.Tests/Unit/ColorTests.cs
Normal file
240
src/Spectre.Console.Tests/Unit/ColorTests.cs
Normal file
@ -0,0 +1,240 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Linq;
|
||||
using Shouldly;
|
||||
using Xunit;
|
||||
|
||||
namespace Spectre.Console.Tests.Unit
|
||||
{
|
||||
public sealed class ColorTests
|
||||
{
|
||||
public sealed class TheEqualsMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Consider_Color_And_Non_Color_Equal()
|
||||
{
|
||||
// Given
|
||||
var color1 = new Color(128, 0, 128);
|
||||
|
||||
// When
|
||||
var result = color1.Equals("Foo");
|
||||
|
||||
// Then
|
||||
result.ShouldBeFalse();
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Consider_Same_Colors_Equal_By_Component()
|
||||
{
|
||||
// Given
|
||||
var color1 = new Color(128, 0, 128);
|
||||
var color2 = new Color(128, 0, 128);
|
||||
|
||||
// When
|
||||
var result = color1.Equals(color2);
|
||||
|
||||
// Then
|
||||
result.ShouldBeTrue();
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Consider_Same_Known_Colors_Equal()
|
||||
{
|
||||
// Given
|
||||
var color1 = Color.Cyan1;
|
||||
var color2 = Color.Cyan1;
|
||||
|
||||
// When
|
||||
var result = color1.Equals(color2);
|
||||
|
||||
// Then
|
||||
result.ShouldBeTrue();
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Consider_Known_Color_And_Color_With_Same_Components_Equal()
|
||||
{
|
||||
// Given
|
||||
var color1 = Color.Cyan1;
|
||||
var color2 = new Color(0, 255, 255);
|
||||
|
||||
// When
|
||||
var result = color1.Equals(color2);
|
||||
|
||||
// Then
|
||||
result.ShouldBeTrue();
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Not_Consider_Different_Colors_Equal()
|
||||
{
|
||||
// Given
|
||||
var color1 = new Color(128, 0, 128);
|
||||
var color2 = new Color(128, 128, 128);
|
||||
|
||||
// When
|
||||
var result = color1.Equals(color2);
|
||||
|
||||
// Then
|
||||
result.ShouldBeFalse();
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class TheGetHashCodeMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Same_HashCode_For_Same_Colors()
|
||||
{
|
||||
// Given
|
||||
var color1 = new Color(128, 0, 128);
|
||||
var color2 = new Color(128, 0, 128);
|
||||
|
||||
// When
|
||||
var hash1 = color1.GetHashCode();
|
||||
var hash2 = color2.GetHashCode();
|
||||
|
||||
// Then
|
||||
hash1.ShouldBe(hash2);
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Return_Different_HashCode_For_Different_Colors()
|
||||
{
|
||||
// Given
|
||||
var color1 = new Color(128, 0, 128);
|
||||
var color2 = new Color(128, 128, 128);
|
||||
|
||||
// When
|
||||
var hash1 = color1.GetHashCode();
|
||||
var hash2 = color2.GetHashCode();
|
||||
|
||||
// Then
|
||||
hash1.ShouldNotBe(hash2);
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class ImplicitConversions
|
||||
{
|
||||
public sealed class Int32ToColor
|
||||
{
|
||||
public static IEnumerable<object[]> Data =>
|
||||
Enumerable.Range(0, 255)
|
||||
.Select(number => new object[] { number });
|
||||
|
||||
[Theory]
|
||||
[MemberData(nameof(Data))]
|
||||
public void Should_Return_Expected_Color(int number)
|
||||
{
|
||||
// Given, When
|
||||
var result = (Color)number;
|
||||
|
||||
// Then
|
||||
result.ShouldBe(Color.FromInt32(number));
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Throw_If_Integer_Is_Lower_Than_Zero()
|
||||
{
|
||||
// Given, When
|
||||
var result = Record.Exception(() => (Color)(-1));
|
||||
|
||||
// Then
|
||||
result.ShouldBeOfType<InvalidOperationException>();
|
||||
result.Message.ShouldBe("Color number must be between 0 and 255");
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Throw_If_Integer_Is_Higher_Than_255()
|
||||
{
|
||||
// Given, When
|
||||
var result = Record.Exception(() => (Color)256);
|
||||
|
||||
// Then
|
||||
result.ShouldBeOfType<InvalidOperationException>();
|
||||
result.Message.ShouldBe("Color number must be between 0 and 255");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class ConsoleColorToColor
|
||||
{
|
||||
[Theory]
|
||||
[InlineData(ConsoleColor.Black, 0)]
|
||||
[InlineData(ConsoleColor.DarkRed, 1)]
|
||||
[InlineData(ConsoleColor.DarkGreen, 2)]
|
||||
[InlineData(ConsoleColor.DarkYellow, 3)]
|
||||
[InlineData(ConsoleColor.DarkBlue, 4)]
|
||||
[InlineData(ConsoleColor.DarkMagenta, 5)]
|
||||
[InlineData(ConsoleColor.DarkCyan, 6)]
|
||||
[InlineData(ConsoleColor.Gray, 7)]
|
||||
[InlineData(ConsoleColor.DarkGray, 8)]
|
||||
[InlineData(ConsoleColor.Red, 9)]
|
||||
[InlineData(ConsoleColor.Green, 10)]
|
||||
[InlineData(ConsoleColor.Yellow, 11)]
|
||||
[InlineData(ConsoleColor.Blue, 12)]
|
||||
[InlineData(ConsoleColor.Magenta, 13)]
|
||||
[InlineData(ConsoleColor.Cyan, 14)]
|
||||
[InlineData(ConsoleColor.White, 15)]
|
||||
public void Should_Return_Expected_Color(ConsoleColor color, int expected)
|
||||
{
|
||||
// Given, When
|
||||
var result = (Color)color;
|
||||
|
||||
// Then
|
||||
result.ShouldBe(Color.FromInt32(expected));
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class ColorToConsoleColor
|
||||
{
|
||||
[Theory]
|
||||
[InlineData(0, ConsoleColor.Black)]
|
||||
[InlineData(1, ConsoleColor.DarkRed)]
|
||||
[InlineData(2, ConsoleColor.DarkGreen)]
|
||||
[InlineData(3, ConsoleColor.DarkYellow)]
|
||||
[InlineData(4, ConsoleColor.DarkBlue)]
|
||||
[InlineData(5, ConsoleColor.DarkMagenta)]
|
||||
[InlineData(6, ConsoleColor.DarkCyan)]
|
||||
[InlineData(7, ConsoleColor.Gray)]
|
||||
[InlineData(8, ConsoleColor.DarkGray)]
|
||||
[InlineData(9, ConsoleColor.Red)]
|
||||
[InlineData(10, ConsoleColor.Green)]
|
||||
[InlineData(11, ConsoleColor.Yellow)]
|
||||
[InlineData(12, ConsoleColor.Blue)]
|
||||
[InlineData(13, ConsoleColor.Magenta)]
|
||||
[InlineData(14, ConsoleColor.Cyan)]
|
||||
[InlineData(15, ConsoleColor.White)]
|
||||
public void Should_Return_Expected_ConsoleColor_For_Known_Color(int color, ConsoleColor expected)
|
||||
{
|
||||
// Given, When
|
||||
var result = (ConsoleColor)Color.FromInt32(color);
|
||||
|
||||
// Then
|
||||
result.ShouldBe(expected);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class TheToStringMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Color_Name_For_Known_Colors()
|
||||
{
|
||||
// Given, When
|
||||
var name = Color.Fuchsia.ToString();
|
||||
|
||||
// Then
|
||||
name.ShouldBe("fuchsia");
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Return_Hex_String_For_Unknown_Colors()
|
||||
{
|
||||
// Given, When
|
||||
var name = new Color(128, 0, 128).ToString();
|
||||
|
||||
// Then
|
||||
name.ShouldBe("#800080 (RGB=128,0,128)");
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
File diff suppressed because it is too large
Load Diff
@ -1,7 +1,6 @@
|
||||
using System;
|
||||
using System.Diagnostics;
|
||||
using System.Globalization;
|
||||
using System.Linq;
|
||||
using Spectre.Console.Internal;
|
||||
|
||||
namespace Spectre.Console
|
||||
@ -18,7 +17,7 @@ namespace Spectre.Console
|
||||
|
||||
static Color()
|
||||
{
|
||||
Default = new Color(0, "default", 0, 0, 0, true);
|
||||
Default = new Color(0, 0, 0, 0, true);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
@ -36,11 +35,6 @@ namespace Spectre.Console
|
||||
/// </summary>
|
||||
public byte B { get; }
|
||||
|
||||
/// <summary>
|
||||
/// Gets the name of the color, if any.
|
||||
/// </summary>
|
||||
public string Name { get; }
|
||||
|
||||
/// <summary>
|
||||
/// Gets the number of the color, if any.
|
||||
/// </summary>
|
||||
@ -63,7 +57,6 @@ namespace Spectre.Console
|
||||
G = green;
|
||||
B = blue;
|
||||
IsDefault = false;
|
||||
Name = null;
|
||||
Number = null;
|
||||
}
|
||||
|
||||
@ -89,7 +82,7 @@ namespace Spectre.Console
|
||||
/// <inheritdoc/>
|
||||
public bool Equals(Color other)
|
||||
{
|
||||
return Number == other.Number || (R == other.R && G == other.G && B == other.B);
|
||||
return R == other.R && G == other.G && B == other.B;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
@ -114,6 +107,15 @@ namespace Spectre.Console
|
||||
return !(left == right);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Convers a <see cref="int"/> to a <see cref="Color"/>.
|
||||
/// </summary>
|
||||
/// <param name="number">The color number to convert.</param>
|
||||
public static implicit operator Color(int number)
|
||||
{
|
||||
return FromInt32(number);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Convers a <see cref="ConsoleColor"/> to a <see cref="Color"/>.
|
||||
/// </summary>
|
||||
@ -124,18 +126,12 @@ namespace Spectre.Console
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Convers a color number into a <see cref="Color"/>.
|
||||
/// Convers a <see cref="Color"/> to a <see cref="ConsoleColor"/>.
|
||||
/// </summary>
|
||||
/// <param name="number">The color number.</param>
|
||||
/// <returns>The color representing the specified color number.</returns>
|
||||
public static Color FromColorNumber(int number)
|
||||
/// <param name="color">The console color to convert.</param>
|
||||
public static implicit operator ConsoleColor(Color color)
|
||||
{
|
||||
if (number < 0 || number > 255)
|
||||
{
|
||||
throw new InvalidOperationException("Color number must be between 0 and 255");
|
||||
}
|
||||
|
||||
return ColorPalette.EightBit.First(x => x.Number == number);
|
||||
return ToConsoleColor(color);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
@ -180,6 +176,16 @@ namespace Spectre.Console
|
||||
};
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Convers a color number into a <see cref="Color"/>.
|
||||
/// </summary>
|
||||
/// <param name="number">The color number.</param>
|
||||
/// <returns>The color representing the specified color number.</returns>
|
||||
public static Color FromInt32(int number)
|
||||
{
|
||||
return ColorTable.GetColor(number);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Convers a <see cref="ConsoleColor"/> to a <see cref="Color"/>.
|
||||
/// </summary>
|
||||
@ -212,7 +218,16 @@ namespace Spectre.Console
|
||||
/// <inheritdoc/>
|
||||
public override string ToString()
|
||||
{
|
||||
return Name ?? string.Format(CultureInfo.InvariantCulture, "#{0:2X}{1:2X}{2:2X}", R, G, B);
|
||||
if (Number != null)
|
||||
{
|
||||
var name = ColorTable.GetName(Number.Value);
|
||||
if (!string.IsNullOrWhiteSpace(name))
|
||||
{
|
||||
return name;
|
||||
}
|
||||
}
|
||||
|
||||
return string.Format(CultureInfo.InvariantCulture, "#{0:X2}{1:X2}{2:X2} (RGB={0},{1},{2})", R, G, B);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
120
src/Spectre.Console/Internal/Colors/ColorTable.cs
Normal file
120
src/Spectre.Console/Internal/Colors/ColorTable.cs
Normal file
@ -0,0 +1,120 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Diagnostics.CodeAnalysis;
|
||||
|
||||
namespace Spectre.Console.Internal
|
||||
{
|
||||
internal static class ColorTable
|
||||
{
|
||||
private static readonly Dictionary<int, string> _nameLookup;
|
||||
private static readonly Dictionary<string, int> _numberLookup;
|
||||
|
||||
[SuppressMessage("Performance", "CA1810:Initialize reference type static fields inline")]
|
||||
static ColorTable()
|
||||
{
|
||||
_numberLookup = new Dictionary<string, int>(StringComparer.OrdinalIgnoreCase)
|
||||
{
|
||||
{ "black", 0 }, { "maroon", 1 }, { "green", 2 }, { "olive", 3 }, { "navy", 4 },
|
||||
{ "purple", 5 }, { "teal", 6 }, { "silver", 7 }, { "grey", 8 },
|
||||
{ "red", 9 }, { "lime", 10 }, { "yellow", 11 }, { "blue", 12 },
|
||||
{ "fuchsia", 13 }, { "aqua", 14 }, { "white", 15 }, { "grey0", 16 },
|
||||
{ "navyblue", 17 }, { "darkblue", 18 }, { "blue3", 19 }, { "blue3_1", 20 },
|
||||
{ "blue1", 21 }, { "darkgreen", 22 }, { "deepskyblue4", 23 }, { "deepskyblue4_1", 24 },
|
||||
{ "deepskyblue4_2", 25 }, { "dodgerblue3", 26 }, { "dodgerblue2", 27 }, { "green4", 28 },
|
||||
{ "springgreen4", 29 }, { "turquoise4", 30 }, { "deepskyblue3", 31 }, { "deepskyblue3_1", 32 },
|
||||
{ "dodgerblue1", 33 }, { "green3", 34 }, { "springgreen3", 35 }, { "darkcyan", 36 },
|
||||
{ "lightseagreen", 37 }, { "deepskyblue2", 38 }, { "deepskyblue1", 39 }, { "green3_1", 40 },
|
||||
{ "springgreen3_1", 41 }, { "springgreen2", 42 }, { "cyan3", 43 }, { "darkturquoise", 44 },
|
||||
{ "turquoise2", 45 }, { "green1", 46 }, { "springgreen2_1", 47 }, { "springgreen1", 48 },
|
||||
{ "mediumspringgreen", 49 }, { "cyan2", 50 }, { "cyan1", 51 }, { "darkred", 52 },
|
||||
{ "deeppink4", 53 }, { "purple4", 54 }, { "purple4_1", 55 }, { "purple3", 56 },
|
||||
{ "blueviolet", 57 }, { "orange4", 58 }, { "grey37", 59 }, { "mediumpurple4", 60 },
|
||||
{ "slateblue3", 61 }, { "slateblue3_1", 62 }, { "royalblue1", 63 }, { "chartreuse4", 64 },
|
||||
{ "darkseagreen4", 65 }, { "paleturquoise4", 66 }, { "steelblue", 67 }, { "steelblue3", 68 },
|
||||
{ "cornflowerblue", 69 }, { "chartreuse3", 70 }, { "darkseagreen4_1", 71 }, { "cadetblue", 72 },
|
||||
{ "cadetblue_1", 73 }, { "skyblue3", 74 }, { "steelblue1", 75 }, { "chartreuse3_1", 76 },
|
||||
{ "palegreen3", 77 }, { "seagreen3", 78 }, { "aquamarine3", 79 }, { "mediumturquoise", 80 },
|
||||
{ "steelblue1_1", 81 }, { "chartreuse2", 82 }, { "seagreen2", 83 }, { "seagreen1", 84 },
|
||||
{ "seagreen1_1", 85 }, { "aquamarine1", 86 }, { "darkslategray2", 87 }, { "darkred_1", 88 },
|
||||
{ "deeppink4_1", 89 }, { "darkmagenta", 90 }, { "darkmagenta_1", 91 }, { "darkviolet", 92 },
|
||||
{ "purple_1", 93 }, { "orange4_1", 94 }, { "lightpink4", 95 }, { "plum4", 96 },
|
||||
{ "mediumpurple3", 97 }, { "mediumpurple3_1", 98 }, { "slateblue1", 99 }, { "yellow4", 100 },
|
||||
{ "wheat4", 101 }, { "grey53", 102 }, { "lightslategrey", 103 }, { "mediumpurple", 104 },
|
||||
{ "lightslateblue", 105 }, { "yellow4_1", 106 }, { "darkolivegreen3", 107 }, { "darkseagreen", 108 },
|
||||
{ "lightskyblue3", 109 }, { "lightskyblue3_1", 110 }, { "skyblue2", 111 }, { "chartreuse2_1", 112 },
|
||||
{ "darkolivegreen3_1", 113 }, { "palegreen3_1", 114 }, { "darkseagreen3", 115 }, { "darkslategray3", 116 },
|
||||
{ "skyblue1", 117 }, { "chartreuse1", 118 }, { "lightgreen", 119 }, { "lightgreen_1", 120 },
|
||||
{ "palegreen1", 121 }, { "aquamarine1_1", 122 }, { "darkslategray1", 123 }, { "red3", 124 },
|
||||
{ "deeppink4_2", 125 }, { "mediumvioletred", 126 }, { "magenta3", 127 }, { "darkviolet_1", 128 },
|
||||
{ "purple_2", 129 }, { "darkorange3", 130 }, { "indianred", 131 }, { "hotpink3", 132 },
|
||||
{ "mediumorchid3", 133 }, { "mediumorchid", 134 }, { "mediumpurple2", 135 }, { "darkgoldenrod", 136 },
|
||||
{ "lightsalmon3", 137 }, { "rosybrown", 138 }, { "grey63", 139 }, { "mediumpurple2_1", 140 },
|
||||
{ "mediumpurple1", 141 }, { "gold3", 142 }, { "darkkhaki", 143 }, { "navajowhite3", 144 },
|
||||
{ "grey69", 145 }, { "lightsteelblue3", 146 }, { "lightsteelblue", 147 }, { "yellow3", 148 },
|
||||
{ "darkolivegreen3_2", 149 }, { "darkseagreen3_1", 150 }, { "darkseagreen2", 151 }, { "lightcyan3", 152 },
|
||||
{ "lightskyblue1", 153 }, { "greenyellow", 154 }, { "darkolivegreen2", 155 }, { "palegreen1_1", 156 },
|
||||
{ "darkseagreen2_1", 157 }, { "darkseagreen1", 158 }, { "paleturquoise1", 159 }, { "red3_1", 160 },
|
||||
{ "deeppink3", 161 }, { "deeppink3_1", 162 }, { "magenta3_1", 163 }, { "magenta3_2", 164 },
|
||||
{ "magenta2", 165 }, { "darkorange3_1", 166 }, { "indianred_1", 167 }, { "hotpink3_1", 168 },
|
||||
{ "hotpink2", 169 }, { "orchid", 170 }, { "mediumorchid1", 171 }, { "orange3", 172 },
|
||||
{ "lightsalmon3_1", 173 }, { "lightpink3", 174 }, { "pink3", 175 }, { "plum3", 176 },
|
||||
{ "violet", 177 }, { "gold3_1", 178 }, { "lightgoldenrod3", 179 }, { "tan", 180 },
|
||||
{ "mistyrose3", 181 }, { "thistle3", 182 }, { "plum2", 183 }, { "yellow3_1", 184 },
|
||||
{ "khaki3", 185 }, { "lightgoldenrod2", 186 }, { "lightyellow3", 187 }, { "grey84", 188 },
|
||||
{ "lightsteelblue1", 189 }, { "yellow2", 190 }, { "darkolivegreen1", 191 }, { "darkolivegreen1_1", 192 },
|
||||
{ "darkseagreen1_1", 193 }, { "honeydew2", 194 }, { "lightcyan1", 195 }, { "red1", 196 },
|
||||
{ "deeppink2", 197 }, { "deeppink1", 198 }, { "deeppink1_1", 199 }, { "magenta2_1", 200 },
|
||||
{ "magenta1", 201 }, { "orangered1", 202 }, { "indianred1", 203 }, { "indianred1_1", 204 },
|
||||
{ "hotpink", 205 }, { "hotpink_1", 206 }, { "mediumorchid1_1", 207 }, { "darkorange", 208 },
|
||||
{ "salmon1", 209 }, { "lightcoral", 210 }, { "palevioletred1", 211 }, { "orchid2", 212 },
|
||||
{ "orchid1", 213 }, { "orange1", 214 }, { "sandybrown", 215 }, { "lightsalmon1", 216 },
|
||||
{ "lightpink1", 217 }, { "pink1", 218 }, { "plum1", 219 }, { "gold1", 220 },
|
||||
{ "lightgoldenrod2_1", 221 }, { "lightgoldenrod2_2", 222 }, { "navajowhite1", 223 }, { "mistyrose1", 224 },
|
||||
{ "thistle1", 225 }, { "yellow1", 226 }, { "lightgoldenrod1", 227 }, { "khaki1", 228 },
|
||||
{ "wheat1", 229 }, { "cornsilk1", 230 }, { "grey100", 231 }, { "grey3", 232 },
|
||||
{ "grey7", 233 }, { "grey11", 234 }, { "grey15", 235 }, { "grey19", 236 },
|
||||
{ "grey23", 237 }, { "grey27", 238 }, { "grey30", 239 }, { "grey35", 240 },
|
||||
{ "grey39", 241 }, { "grey42", 242 }, { "grey46", 243 }, { "grey50", 244 },
|
||||
{ "grey54", 245 }, { "grey58", 246 }, { "grey62", 247 }, { "grey66", 248 },
|
||||
{ "grey70", 249 }, { "grey74", 250 }, { "grey78", 251 }, { "grey82", 252 },
|
||||
};
|
||||
|
||||
_nameLookup = new Dictionary<int, string>();
|
||||
foreach (var pair in _numberLookup)
|
||||
{
|
||||
_nameLookup.Add(pair.Value, pair.Key);
|
||||
}
|
||||
}
|
||||
|
||||
public static Color GetColor(int number)
|
||||
{
|
||||
if (number < 0 || number > 255)
|
||||
{
|
||||
throw new InvalidOperationException("Color number must be between 0 and 255");
|
||||
}
|
||||
|
||||
return ColorPalette.EightBit[number];
|
||||
}
|
||||
|
||||
public static Color? GetColor(string name)
|
||||
{
|
||||
if (!_numberLookup.TryGetValue(name, out var number))
|
||||
{
|
||||
return null;
|
||||
}
|
||||
|
||||
if (number > ColorPalette.EightBit.Count - 1)
|
||||
{
|
||||
return null;
|
||||
}
|
||||
|
||||
return ColorPalette.EightBit[number];
|
||||
}
|
||||
|
||||
public static string GetName(int number)
|
||||
{
|
||||
_nameLookup.TryGetValue(number, out var name);
|
||||
return name;
|
||||
}
|
||||
}
|
||||
}
|
@ -1,17 +1,15 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Diagnostics.CodeAnalysis;
|
||||
|
||||
namespace Spectre.Console.Internal
|
||||
{
|
||||
internal sealed class Lookup
|
||||
internal static class StyleTable
|
||||
{
|
||||
private readonly Dictionary<string, Styles?> _styles;
|
||||
private readonly Dictionary<string, Color?> _colors;
|
||||
private static readonly Dictionary<string, Styles?> _styles;
|
||||
|
||||
private static readonly Lazy<Lookup> _lazy = new Lazy<Lookup>(() => new Lookup());
|
||||
public static Lookup Instance => _lazy.Value;
|
||||
|
||||
private Lookup()
|
||||
[SuppressMessage("Performance", "CA1810:Initialize reference type static fields inline")]
|
||||
static StyleTable()
|
||||
{
|
||||
_styles = new Dictionary<string, Styles?>(StringComparer.OrdinalIgnoreCase)
|
||||
{
|
||||
@ -25,24 +23,12 @@ namespace Spectre.Console.Internal
|
||||
{ "rapidblink", Styles.RapidBlink },
|
||||
{ "strikethrough", Styles.Strikethrough },
|
||||
};
|
||||
|
||||
_colors = new Dictionary<string, Color?>(StringComparer.OrdinalIgnoreCase);
|
||||
foreach (var color in ColorPalette.EightBit)
|
||||
{
|
||||
_colors.Add(color.Name, color);
|
||||
}
|
||||
}
|
||||
|
||||
public Styles? GetStyle(string name)
|
||||
public static Styles? GetStyle(string name)
|
||||
{
|
||||
_styles.TryGetValue(name, out var style);
|
||||
return style;
|
||||
}
|
||||
|
||||
public Color? GetColor(string name)
|
||||
{
|
||||
_colors.TryGetValue(name, out var color);
|
||||
return color;
|
||||
}
|
||||
}
|
||||
}
|
@ -20,7 +20,7 @@ namespace Spectre.Console.Internal
|
||||
continue;
|
||||
}
|
||||
|
||||
var style = Lookup.Instance.GetStyle(part);
|
||||
var style = StyleTable.GetStyle(part);
|
||||
if (style != null)
|
||||
{
|
||||
if (effectiveStyle == null)
|
||||
@ -32,7 +32,7 @@ namespace Spectre.Console.Internal
|
||||
}
|
||||
else
|
||||
{
|
||||
var color = Lookup.Instance.GetColor(part);
|
||||
var color = ColorTable.GetColor(part);
|
||||
if (color == null)
|
||||
{
|
||||
throw new InvalidOperationException("Could not find color..");
|
||||
|
Reference in New Issue
Block a user