mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-31 17:25:58 +08:00
Add convenience methods for tree nodes
This commit is contained in:
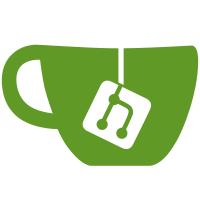
committed by
Patrik Svensson
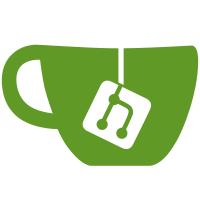
parent
87e6b42409
commit
1f211d3e1f
@ -9,17 +9,17 @@ namespace TableExample
|
||||
var tree = new Tree();
|
||||
|
||||
tree.AddNode(new FigletText("Dec 2020"));
|
||||
tree.AddNode(new Markup("[link]Click to go to summary[/]"));
|
||||
tree.AddNode("[link]Click to go to summary[/]");
|
||||
|
||||
// Add the calendar nodes
|
||||
tree.AddNode(new Markup("[blue]Calendar[/]"),
|
||||
tree.AddNode("[blue]Calendar[/]",
|
||||
node => node.AddNode(
|
||||
new Calendar(2020, 12)
|
||||
.AddCalendarEvent(2020, 12, 12)
|
||||
.HideHeader()));
|
||||
|
||||
// Add video games node
|
||||
tree.AddNode(new Markup("[red]Played video games[/]"),
|
||||
tree.AddNode("[red]Played video games[/]",
|
||||
node => node.AddNode(
|
||||
new Table()
|
||||
.RoundedBorder()
|
||||
@ -31,8 +31,8 @@ namespace TableExample
|
||||
|
||||
|
||||
// Add the fruit nodes
|
||||
tree.AddNode(new Markup("[green]Fruits[/]"), fruits =>
|
||||
fruits.AddNode(new Markup("Eaten"),
|
||||
tree.AddNode("[green]Fruits[/]", fruits =>
|
||||
fruits.AddNode("Eaten",
|
||||
node => node.AddNode(
|
||||
new BarChart().Width(40)
|
||||
.AddItem("Apple", 12, Color.Red)
|
||||
|
@ -1,4 +1,5 @@
|
||||
using System;
|
||||
using System.Linq;
|
||||
using Spectre.Console.Rendering;
|
||||
|
||||
namespace Spectre.Console
|
||||
@ -9,7 +10,49 @@ namespace Spectre.Console
|
||||
public static class HasTreeNodeExtensions
|
||||
{
|
||||
/// <summary>
|
||||
/// Adds a child tree node.
|
||||
/// Adds a tree node.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with tree nodes.</typeparam>
|
||||
/// <param name="obj">The object that has tree nodes.</param>
|
||||
/// <param name="markup">The node's markup text.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T AddNode<T>(this T obj, string markup)
|
||||
where T : class, IHasTreeNodes
|
||||
{
|
||||
if (obj is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(obj));
|
||||
}
|
||||
|
||||
if (markup is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(markup));
|
||||
}
|
||||
|
||||
return AddNode(obj, new Markup(markup));
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Adds a tree node.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with tree nodes.</typeparam>
|
||||
/// <param name="obj">The object that has tree nodes.</param>
|
||||
/// <param name="markup">The node's markup text.</param>
|
||||
/// <param name="action">An action that can be used to configure the created node further.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T AddNode<T>(this T obj, string markup, Action<TreeNode> action)
|
||||
where T : class, IHasTreeNodes
|
||||
{
|
||||
if (markup is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(markup));
|
||||
}
|
||||
|
||||
return AddNode(obj, new Markup(markup), action);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Adds a tree node.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with tree nodes.</typeparam>
|
||||
/// <param name="obj">The object that has tree nodes.</param>
|
||||
@ -23,19 +66,24 @@ namespace Spectre.Console
|
||||
throw new ArgumentNullException(nameof(obj));
|
||||
}
|
||||
|
||||
if (renderable is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(renderable));
|
||||
}
|
||||
|
||||
obj.Children.Add(new TreeNode(renderable));
|
||||
return obj;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Adds a child tree node.
|
||||
/// Adds a tree node.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with tree nodes.</typeparam>
|
||||
/// <param name="obj">The object that has tree nodes.</param>
|
||||
/// <param name="renderable">The renderable to add.</param>
|
||||
/// <param name="config">An action that can be used to configure the created node further.</param>
|
||||
/// <param name="action">An action that can be used to configure the created node further.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T AddNode<T>(this T obj, IRenderable renderable, Action<TreeNode> config)
|
||||
public static T AddNode<T>(this T obj, IRenderable renderable, Action<TreeNode> action)
|
||||
where T : class, IHasTreeNodes
|
||||
{
|
||||
if (obj is null)
|
||||
@ -43,20 +91,25 @@ namespace Spectre.Console
|
||||
throw new ArgumentNullException(nameof(obj));
|
||||
}
|
||||
|
||||
if (config is null)
|
||||
if (renderable is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(config));
|
||||
throw new ArgumentNullException(nameof(renderable));
|
||||
}
|
||||
|
||||
if (action is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(action));
|
||||
}
|
||||
|
||||
var node = new TreeNode(renderable);
|
||||
config(node);
|
||||
action(node);
|
||||
|
||||
obj.Children.Add(node);
|
||||
return obj;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Adds a child tree node.
|
||||
/// Adds a tree node.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with tree nodes.</typeparam>
|
||||
/// <param name="obj">The object that has tree nodes.</param>
|
||||
@ -70,8 +123,61 @@ namespace Spectre.Console
|
||||
throw new ArgumentNullException(nameof(obj));
|
||||
}
|
||||
|
||||
if (node is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(node));
|
||||
}
|
||||
|
||||
obj.Children.Add(node);
|
||||
return obj;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Add multiple tree nodes.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with tree nodes.</typeparam>
|
||||
/// <param name="obj">The object that has tree nodes.</param>
|
||||
/// <param name="nodes">The tree nodes to add.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T AddNodes<T>(this T obj, params string[] nodes)
|
||||
where T : class, IHasTreeNodes
|
||||
{
|
||||
if (obj is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(obj));
|
||||
}
|
||||
|
||||
if (nodes is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(nodes));
|
||||
}
|
||||
|
||||
obj.Children.AddRange(nodes.Select(node => new TreeNode(new Markup(node))));
|
||||
return obj;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Add multiple tree nodes.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with tree nodes.</typeparam>
|
||||
/// <param name="obj">The object that has tree nodes.</param>
|
||||
/// <param name="nodes">The tree nodes to add.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T AddNodes<T>(this T obj, params TreeNode[] nodes)
|
||||
where T : class, IHasTreeNodes
|
||||
{
|
||||
if (obj is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(obj));
|
||||
}
|
||||
|
||||
if (nodes is null)
|
||||
{
|
||||
throw new ArgumentNullException(nameof(nodes));
|
||||
}
|
||||
|
||||
obj.Children.AddRange(nodes);
|
||||
return obj;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
Reference in New Issue
Block a user