mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-08-01 09:45:58 +08:00
Update Canvas tests
This commit is contained in:
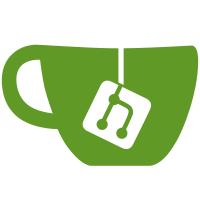
committed by
Patrik Svensson
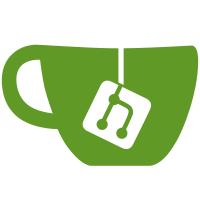
parent
8901450283
commit
11e192e750
@ -0,0 +1,5 @@
|
||||
[101m [0m [42m [0m
|
||||
|
||||
|
||||
|
||||
[104m [0m [103m [0m
|
@ -0,0 +1,5 @@
|
||||
[106m [0m
|
||||
|
||||
|
||||
|
||||
|
@ -0,0 +1,2 @@
|
||||
[106m [0m
|
||||
|
@ -0,0 +1,4 @@
|
||||
┌──────┐
|
||||
│ [106m [0m │
|
||||
│ [100m [0m │
|
||||
└──────┘
|
@ -1,57 +1,81 @@
|
||||
using System;
|
||||
using System.Linq;
|
||||
using System.Text;
|
||||
using System.Threading.Tasks;
|
||||
using Shouldly;
|
||||
using Spectre.Console.Rendering;
|
||||
using Spectre.Console.Testing;
|
||||
using Spectre.Verify.Extensions;
|
||||
using VerifyXunit;
|
||||
using Xunit;
|
||||
|
||||
namespace Spectre.Console.Tests.Unit
|
||||
{
|
||||
[UsesVerify]
|
||||
[ExpectationPath("Widgets/Canvas")]
|
||||
public class CanvasTests
|
||||
{
|
||||
[Fact]
|
||||
public void Canvas_Must_Have_Proper_Size()
|
||||
public sealed class TheConstructor
|
||||
{
|
||||
Should.Throw<ArgumentException>(() => new Canvas(1, 0));
|
||||
[Fact]
|
||||
public void Should_Throw_If_Width_Is_Less_Than_Zero()
|
||||
{
|
||||
// Given, When
|
||||
var result = Record.Exception(() => new Canvas(0, 1));
|
||||
|
||||
Should.Throw<ArgumentException>(() => new Canvas(0, 1));
|
||||
// Then
|
||||
result.ShouldBeOfType<ArgumentException>()
|
||||
.And(ex => ex.ParamName.ShouldBe("width"));
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Throw_If_Height_Is_Less_Than_Zero()
|
||||
{
|
||||
// Given, When
|
||||
var result = Record.Exception(() => new Canvas(1, 0));
|
||||
|
||||
// Then
|
||||
result.ShouldBeOfType<ArgumentException>()
|
||||
.And(ex => ex.ParamName.ShouldBe("height"));
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Simple_Render()
|
||||
[Expectation("Render")]
|
||||
public async Task Should_Render_Canvas_Correctly()
|
||||
{
|
||||
// Given
|
||||
var console = new FakeAnsiConsole(ColorSystem.Standard);
|
||||
var canvas = new Canvas(width: 2, height: 2);
|
||||
canvas.SetPixel(0, 0, Color.Aqua);
|
||||
canvas.SetPixel(1, 1, Color.Grey);
|
||||
var canvas = new Canvas(width: 5, height: 5);
|
||||
canvas.SetPixel(0, 0, Color.Red);
|
||||
canvas.SetPixel(4, 0, Color.Green);
|
||||
canvas.SetPixel(0, 4, Color.Blue);
|
||||
canvas.SetPixel(4, 4, Color.Yellow);
|
||||
|
||||
// When
|
||||
console.Render(canvas);
|
||||
|
||||
// Then
|
||||
console.Output.ShouldBe($"\u001b[106m \u001b[0m {Environment.NewLine} \u001b[100m \u001b[0m{Environment.NewLine}");
|
||||
await Verifier.Verify(console.Output);
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Render_Wider_Than_Terminal_Cannot_Be_Reduced_Further()
|
||||
[Expectation("Render_Nested")]
|
||||
public async Task Simple_Measure()
|
||||
{
|
||||
// Given
|
||||
var console = new FakeAnsiConsole(ColorSystem.Standard, width: 10);
|
||||
var canvas = new Canvas(width: 20, height: 2);
|
||||
canvas.SetPixel(0, 0, Color.Aqua);
|
||||
canvas.SetPixel(19, 1, Color.Grey);
|
||||
var console = new FakeAnsiConsole(ColorSystem.Standard);
|
||||
var panel = new Panel(new Canvas(width: 2, height: 2)
|
||||
.SetPixel(0, 0, Color.Aqua)
|
||||
.SetPixel(1, 1, Color.Grey));
|
||||
|
||||
// When
|
||||
console.Render(canvas);
|
||||
console.Render(panel);
|
||||
|
||||
// Then
|
||||
console.Output.ShouldBe(string.Empty);
|
||||
await Verifier.Verify(console.Output);
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Render_Wider_Than_Terminal()
|
||||
[Expectation("Render_NarrowTerminal")]
|
||||
public async Task Should_Scale_Down_Canvas_Is_Bigger_Than_Terminal()
|
||||
{
|
||||
// Given
|
||||
var console = new FakeAnsiConsole(ColorSystem.Standard, width: 10);
|
||||
@ -63,46 +87,40 @@ namespace Spectre.Console.Tests.Unit
|
||||
console.Render(canvas);
|
||||
|
||||
// Then
|
||||
var numNewlines = console.Output.Count(x => x == '\n');
|
||||
|
||||
// Small terminal shrinks the canvas
|
||||
numNewlines.ShouldBe(expected: 2);
|
||||
await Verifier.Verify(console.Output);
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Render_Wider_Configured_With_Max_Width()
|
||||
[Expectation("Render_MaxWidth")]
|
||||
public async Task Should_Scale_Down_Canvas_If_MaxWidth_Is_Set()
|
||||
{
|
||||
// Given
|
||||
var console = new FakeAnsiConsole(ColorSystem.Standard, width: 80);
|
||||
var canvas = new Canvas(width: 20, height: 10) { MaxWidth = 10 };
|
||||
canvas.SetPixel(0, 0, Color.Aqua);
|
||||
canvas.SetPixel(19, 9, Color.Grey);
|
||||
canvas.SetPixel(19, 9, Color.Aqua);
|
||||
|
||||
// When
|
||||
console.Render(canvas);
|
||||
|
||||
// Then
|
||||
var numNewlines = console.Output.Count(x => x == '\n');
|
||||
|
||||
// MaxWidth truncates the canvas
|
||||
numNewlines.ShouldBe(expected: 5);
|
||||
await Verifier.Verify(console.Output);
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Simple_Measure()
|
||||
public void Should_Not_Render_Canvas_If_Canvas_Cannot_Be_Scaled_Down()
|
||||
{
|
||||
// Given
|
||||
var console = new FakeAnsiConsole(ColorSystem.Standard);
|
||||
var canvas = new Canvas(width: 2, height: 2);
|
||||
var console = new FakeAnsiConsole(ColorSystem.Standard, width: 10);
|
||||
var canvas = new Canvas(width: 20, height: 2);
|
||||
canvas.SetPixel(0, 0, Color.Aqua);
|
||||
canvas.SetPixel(1, 1, Color.Grey);
|
||||
canvas.SetPixel(19, 1, Color.Grey);
|
||||
|
||||
// When
|
||||
var measurement = ((IRenderable)canvas).Measure(new RenderContext(Encoding.Unicode, false), 80);
|
||||
console.Render(canvas);
|
||||
|
||||
// Then
|
||||
measurement.Max.ShouldBe(expected: 4);
|
||||
measurement.Min.ShouldBe(expected: 4);
|
||||
console.Output.ShouldBeEmpty();
|
||||
}
|
||||
}
|
||||
}
|
@ -66,9 +66,11 @@ namespace Spectre.Console
|
||||
/// <param name="x">The X coordinate for the pixel.</param>
|
||||
/// <param name="y">The Y coordinate for the pixel.</param>
|
||||
/// <param name="color">The pixel color.</param>
|
||||
public void SetPixel(int x, int y, Color color)
|
||||
/// <returns>The same <see cref="Canvas"/> instance so that multiple calls can be chained.</returns>
|
||||
public Canvas SetPixel(int x, int y, Color color)
|
||||
{
|
||||
_pixels[x, y] = color;
|
||||
return this;
|
||||
}
|
||||
|
||||
/// <inheritdoc/>
|
||||
|
Reference in New Issue
Block a user