mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-16 00:42:51 +08:00
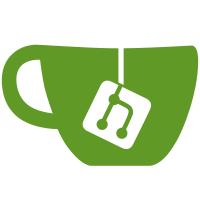
* Add constants for emojis * Move emoji shortcode rendering to Markup * Add documentation * Add example * Add tests
9301 lines
337 KiB
C#
9301 lines
337 KiB
C#
//------------------------------------------------------------------------------
|
|
// <auto-generated>
|
|
// This code was generated by a tool.
|
|
// Generated 2020-09-18 11:14
|
|
//
|
|
// Changes to this file may cause incorrect behavior and will be lost if
|
|
// the code is regenerated.
|
|
// </auto-generated>
|
|
//------------------------------------------------------------------------------
|
|
|
|
using System;
|
|
using System.Collections.Generic;
|
|
|
|
namespace Spectre.Console
|
|
{
|
|
/// <summary>
|
|
/// Utility for working with emojis.
|
|
/// </summary>
|
|
public static partial class Emoji
|
|
{
|
|
private static readonly Dictionary<string, string> _emojis
|
|
= new Dictionary<string, string>(StringComparer.InvariantCultureIgnoreCase)
|
|
{
|
|
{ "abacus", Emoji.Known.Abacus },
|
|
{ "ab_button_blood_type", Emoji.Known.AbButtonBloodType },
|
|
{ "a_button_blood_type", Emoji.Known.AButtonBloodType },
|
|
{ "accordion", Emoji.Known.Accordion },
|
|
{ "adhesive_bandage", Emoji.Known.AdhesiveBandage },
|
|
{ "admission_tickets", Emoji.Known.AdmissionTickets },
|
|
{ "aerial_tramway", Emoji.Known.AerialTramway },
|
|
{ "airplane", Emoji.Known.Airplane },
|
|
{ "airplane_arrival", Emoji.Known.AirplaneArrival },
|
|
{ "airplane_departure", Emoji.Known.AirplaneDeparture },
|
|
{ "alarm_clock", Emoji.Known.AlarmClock },
|
|
{ "alembic", Emoji.Known.Alembic },
|
|
{ "alien", Emoji.Known.Alien },
|
|
{ "alien_monster", Emoji.Known.AlienMonster },
|
|
{ "ambulance", Emoji.Known.Ambulance },
|
|
{ "american_football", Emoji.Known.AmericanFootball },
|
|
{ "amphora", Emoji.Known.Amphora },
|
|
{ "anatomical_heart", Emoji.Known.AnatomicalHeart },
|
|
{ "anchor", Emoji.Known.Anchor },
|
|
{ "anger_symbol", Emoji.Known.AngerSymbol },
|
|
{ "angry_face", Emoji.Known.AngryFace },
|
|
{ "angry_face_with_horns", Emoji.Known.AngryFaceWithHorns },
|
|
{ "anguished_face", Emoji.Known.AnguishedFace },
|
|
{ "ant", Emoji.Known.Ant },
|
|
{ "antenna_bars", Emoji.Known.AntennaBars },
|
|
{ "anxious_face_with_sweat", Emoji.Known.AnxiousFaceWithSweat },
|
|
{ "aquarius", Emoji.Known.Aquarius },
|
|
{ "aries", Emoji.Known.Aries },
|
|
{ "articulated_lorry", Emoji.Known.ArticulatedLorry },
|
|
{ "artist_palette", Emoji.Known.ArtistPalette },
|
|
{ "astonished_face", Emoji.Known.AstonishedFace },
|
|
{ "atm_sign", Emoji.Known.AtmSign },
|
|
{ "atom_symbol", Emoji.Known.AtomSymbol },
|
|
{ "automobile", Emoji.Known.Automobile },
|
|
{ "auto_rickshaw", Emoji.Known.AutoRickshaw },
|
|
{ "avocado", Emoji.Known.Avocado },
|
|
{ "axe", Emoji.Known.Axe },
|
|
{ "baby", Emoji.Known.Baby },
|
|
{ "baby_angel", Emoji.Known.BabyAngel },
|
|
{ "baby_bottle", Emoji.Known.BabyBottle },
|
|
{ "baby_chick", Emoji.Known.BabyChick },
|
|
{ "baby_symbol", Emoji.Known.BabySymbol },
|
|
{ "back_arrow", Emoji.Known.BackArrow },
|
|
{ "backhand_index_pointing_down", Emoji.Known.BackhandIndexPointingDown },
|
|
{ "backhand_index_pointing_left", Emoji.Known.BackhandIndexPointingLeft },
|
|
{ "backhand_index_pointing_right", Emoji.Known.BackhandIndexPointingRight },
|
|
{ "backhand_index_pointing_up", Emoji.Known.BackhandIndexPointingUp },
|
|
{ "backpack", Emoji.Known.Backpack },
|
|
{ "bacon", Emoji.Known.Bacon },
|
|
{ "badger", Emoji.Known.Badger },
|
|
{ "badminton", Emoji.Known.Badminton },
|
|
{ "bagel", Emoji.Known.Bagel },
|
|
{ "baggage_claim", Emoji.Known.BaggageClaim },
|
|
{ "baguette_bread", Emoji.Known.BaguetteBread },
|
|
{ "balance_scale", Emoji.Known.BalanceScale },
|
|
{ "bald", Emoji.Known.Bald },
|
|
{ "ballet_shoes", Emoji.Known.BalletShoes },
|
|
{ "balloon", Emoji.Known.Balloon },
|
|
{ "ballot_box_with_ballot", Emoji.Known.BallotBoxWithBallot },
|
|
{ "banana", Emoji.Known.Banana },
|
|
{ "banjo", Emoji.Known.Banjo },
|
|
{ "bank", Emoji.Known.Bank },
|
|
{ "barber_pole", Emoji.Known.BarberPole },
|
|
{ "bar_chart", Emoji.Known.BarChart },
|
|
{ "baseball", Emoji.Known.Baseball },
|
|
{ "basket", Emoji.Known.Basket },
|
|
{ "basketball", Emoji.Known.Basketball },
|
|
{ "bat", Emoji.Known.Bat },
|
|
{ "bathtub", Emoji.Known.Bathtub },
|
|
{ "battery", Emoji.Known.Battery },
|
|
{ "b_button_blood_type", Emoji.Known.BButtonBloodType },
|
|
{ "beach_with_umbrella", Emoji.Known.BeachWithUmbrella },
|
|
{ "beaming_face_with_smiling_eyes", Emoji.Known.BeamingFaceWithSmilingEyes },
|
|
{ "bear", Emoji.Known.Bear },
|
|
{ "beating_heart", Emoji.Known.BeatingHeart },
|
|
{ "beaver", Emoji.Known.Beaver },
|
|
{ "bed", Emoji.Known.Bed },
|
|
{ "beer_mug", Emoji.Known.BeerMug },
|
|
{ "beetle", Emoji.Known.Beetle },
|
|
{ "bell", Emoji.Known.Bell },
|
|
{ "bellhop_bell", Emoji.Known.BellhopBell },
|
|
{ "bell_pepper", Emoji.Known.BellPepper },
|
|
{ "bell_with_slash", Emoji.Known.BellWithSlash },
|
|
{ "bento_box", Emoji.Known.BentoBox },
|
|
{ "beverage_box", Emoji.Known.BeverageBox },
|
|
{ "bicycle", Emoji.Known.Bicycle },
|
|
{ "bikini", Emoji.Known.Bikini },
|
|
{ "billed_cap", Emoji.Known.BilledCap },
|
|
{ "biohazard", Emoji.Known.Biohazard },
|
|
{ "bird", Emoji.Known.Bird },
|
|
{ "birthday_cake", Emoji.Known.BirthdayCake },
|
|
{ "bison", Emoji.Known.Bison },
|
|
{ "black_circle", Emoji.Known.BlackCircle },
|
|
{ "black_flag", Emoji.Known.BlackFlag },
|
|
{ "black_heart", Emoji.Known.BlackHeart },
|
|
{ "black_large_square", Emoji.Known.BlackLargeSquare },
|
|
{ "black_medium_small_square", Emoji.Known.BlackMediumSmallSquare },
|
|
{ "black_medium_square", Emoji.Known.BlackMediumSquare },
|
|
{ "black_nib", Emoji.Known.BlackNib },
|
|
{ "black_small_square", Emoji.Known.BlackSmallSquare },
|
|
{ "black_square_button", Emoji.Known.BlackSquareButton },
|
|
{ "blossom", Emoji.Known.Blossom },
|
|
{ "blowfish", Emoji.Known.Blowfish },
|
|
{ "blueberries", Emoji.Known.Blueberries },
|
|
{ "blue_book", Emoji.Known.BlueBook },
|
|
{ "blue_circle", Emoji.Known.BlueCircle },
|
|
{ "blue_heart", Emoji.Known.BlueHeart },
|
|
{ "blue_square", Emoji.Known.BlueSquare },
|
|
{ "boar", Emoji.Known.Boar },
|
|
{ "bomb", Emoji.Known.Bomb },
|
|
{ "bone", Emoji.Known.Bone },
|
|
{ "bookmark", Emoji.Known.Bookmark },
|
|
{ "bookmark_tabs", Emoji.Known.BookmarkTabs },
|
|
{ "books", Emoji.Known.Books },
|
|
{ "boomerang", Emoji.Known.Boomerang },
|
|
{ "bottle_with_popping_cork", Emoji.Known.BottleWithPoppingCork },
|
|
{ "bouquet", Emoji.Known.Bouquet },
|
|
{ "bow_and_arrow", Emoji.Known.BowAndArrow },
|
|
{ "bowling", Emoji.Known.Bowling },
|
|
{ "bowl_with_spoon", Emoji.Known.BowlWithSpoon },
|
|
{ "boxing_glove", Emoji.Known.BoxingGlove },
|
|
{ "boy", Emoji.Known.Boy },
|
|
{ "brain", Emoji.Known.Brain },
|
|
{ "bread", Emoji.Known.Bread },
|
|
{ "breast_feeding", Emoji.Known.BreastFeeding },
|
|
{ "brick", Emoji.Known.Brick },
|
|
{ "bridge_at_night", Emoji.Known.BridgeAtNight },
|
|
{ "briefcase", Emoji.Known.Briefcase },
|
|
{ "briefs", Emoji.Known.Briefs },
|
|
{ "bright_button", Emoji.Known.BrightButton },
|
|
{ "broccoli", Emoji.Known.Broccoli },
|
|
{ "broken_heart", Emoji.Known.BrokenHeart },
|
|
{ "broom", Emoji.Known.Broom },
|
|
{ "brown_circle", Emoji.Known.BrownCircle },
|
|
{ "brown_heart", Emoji.Known.BrownHeart },
|
|
{ "brown_square", Emoji.Known.BrownSquare },
|
|
{ "bubble_tea", Emoji.Known.BubbleTea },
|
|
{ "bucket", Emoji.Known.Bucket },
|
|
{ "bug", Emoji.Known.Bug },
|
|
{ "building_construction", Emoji.Known.BuildingConstruction },
|
|
{ "bullet_train", Emoji.Known.BulletTrain },
|
|
{ "bullseye", Emoji.Known.Bullseye },
|
|
{ "burrito", Emoji.Known.Burrito },
|
|
{ "bus", Emoji.Known.Bus },
|
|
{ "bus_stop", Emoji.Known.BusStop },
|
|
{ "bust_in_silhouette", Emoji.Known.BustInSilhouette },
|
|
{ "busts_in_silhouette", Emoji.Known.BustsInSilhouette },
|
|
{ "butter", Emoji.Known.Butter },
|
|
{ "butterfly", Emoji.Known.Butterfly },
|
|
{ "cactus", Emoji.Known.Cactus },
|
|
{ "calendar", Emoji.Known.Calendar },
|
|
{ "call_me_hand", Emoji.Known.CallMeHand },
|
|
{ "camel", Emoji.Known.Camel },
|
|
{ "camera", Emoji.Known.Camera },
|
|
{ "camera_with_flash", Emoji.Known.CameraWithFlash },
|
|
{ "camping", Emoji.Known.Camping },
|
|
{ "cancer", Emoji.Known.Cancer },
|
|
{ "candle", Emoji.Known.Candle },
|
|
{ "candy", Emoji.Known.Candy },
|
|
{ "canned_food", Emoji.Known.CannedFood },
|
|
{ "canoe", Emoji.Known.Canoe },
|
|
{ "capricorn", Emoji.Known.Capricorn },
|
|
{ "card_file_box", Emoji.Known.CardFileBox },
|
|
{ "card_index", Emoji.Known.CardIndex },
|
|
{ "card_index_dividers", Emoji.Known.CardIndexDividers },
|
|
{ "carousel_horse", Emoji.Known.CarouselHorse },
|
|
{ "carpentry_saw", Emoji.Known.CarpentrySaw },
|
|
{ "carp_streamer", Emoji.Known.CarpStreamer },
|
|
{ "carrot", Emoji.Known.Carrot },
|
|
{ "castle", Emoji.Known.Castle },
|
|
{ "cat", Emoji.Known.Cat },
|
|
{ "cat_face", Emoji.Known.CatFace },
|
|
{ "cat_with_tears_of_joy", Emoji.Known.CatWithTearsOfJoy },
|
|
{ "cat_with_wry_smile", Emoji.Known.CatWithWrySmile },
|
|
{ "chains", Emoji.Known.Chains },
|
|
{ "chair", Emoji.Known.Chair },
|
|
{ "chart_decreasing", Emoji.Known.ChartDecreasing },
|
|
{ "chart_increasing", Emoji.Known.ChartIncreasing },
|
|
{ "chart_increasing_with_yen", Emoji.Known.ChartIncreasingWithYen },
|
|
{ "check_box_with_check", Emoji.Known.CheckBoxWithCheck },
|
|
{ "check_mark", Emoji.Known.CheckMark },
|
|
{ "check_mark_button", Emoji.Known.CheckMarkButton },
|
|
{ "cheese_wedge", Emoji.Known.CheeseWedge },
|
|
{ "chequered_flag", Emoji.Known.ChequeredFlag },
|
|
{ "cherries", Emoji.Known.Cherries },
|
|
{ "cherry_blossom", Emoji.Known.CherryBlossom },
|
|
{ "chess_pawn", Emoji.Known.ChessPawn },
|
|
{ "chestnut", Emoji.Known.Chestnut },
|
|
{ "chicken", Emoji.Known.Chicken },
|
|
{ "child", Emoji.Known.Child },
|
|
{ "children_crossing", Emoji.Known.ChildrenCrossing },
|
|
{ "chipmunk", Emoji.Known.Chipmunk },
|
|
{ "chocolate_bar", Emoji.Known.ChocolateBar },
|
|
{ "chopsticks", Emoji.Known.Chopsticks },
|
|
{ "christmas_tree", Emoji.Known.ChristmasTree },
|
|
{ "church", Emoji.Known.Church },
|
|
{ "cigarette", Emoji.Known.Cigarette },
|
|
{ "cinema", Emoji.Known.Cinema },
|
|
{ "circled_m", Emoji.Known.CircledM },
|
|
{ "circus_tent", Emoji.Known.CircusTent },
|
|
{ "cityscape", Emoji.Known.Cityscape },
|
|
{ "cityscape_at_dusk", Emoji.Known.CityscapeAtDusk },
|
|
{ "clamp", Emoji.Known.Clamp },
|
|
{ "clapper_board", Emoji.Known.ClapperBoard },
|
|
{ "clapping_hands", Emoji.Known.ClappingHands },
|
|
{ "classical_building", Emoji.Known.ClassicalBuilding },
|
|
{ "cl_button", Emoji.Known.ClButton },
|
|
{ "clinking_beer_mugs", Emoji.Known.ClinkingBeerMugs },
|
|
{ "clinking_glasses", Emoji.Known.ClinkingGlasses },
|
|
{ "clipboard", Emoji.Known.Clipboard },
|
|
{ "clockwise_vertical_arrows", Emoji.Known.ClockwiseVerticalArrows },
|
|
{ "closed_book", Emoji.Known.ClosedBook },
|
|
{ "closed_mailbox_with_lowered_flag", Emoji.Known.ClosedMailboxWithLoweredFlag },
|
|
{ "closed_mailbox_with_raised_flag", Emoji.Known.ClosedMailboxWithRaisedFlag },
|
|
{ "closed_umbrella", Emoji.Known.ClosedUmbrella },
|
|
{ "cloud", Emoji.Known.Cloud },
|
|
{ "cloud_with_lightning", Emoji.Known.CloudWithLightning },
|
|
{ "cloud_with_lightning_and_rain", Emoji.Known.CloudWithLightningAndRain },
|
|
{ "cloud_with_rain", Emoji.Known.CloudWithRain },
|
|
{ "cloud_with_snow", Emoji.Known.CloudWithSnow },
|
|
{ "clown_face", Emoji.Known.ClownFace },
|
|
{ "club_suit", Emoji.Known.ClubSuit },
|
|
{ "clutch_bag", Emoji.Known.ClutchBag },
|
|
{ "coat", Emoji.Known.Coat },
|
|
{ "cockroach", Emoji.Known.Cockroach },
|
|
{ "cocktail_glass", Emoji.Known.CocktailGlass },
|
|
{ "coconut", Emoji.Known.Coconut },
|
|
{ "coffin", Emoji.Known.Coffin },
|
|
{ "coin", Emoji.Known.Coin },
|
|
{ "cold_face", Emoji.Known.ColdFace },
|
|
{ "collision", Emoji.Known.Collision },
|
|
{ "comet", Emoji.Known.Comet },
|
|
{ "compass", Emoji.Known.Compass },
|
|
{ "computer_disk", Emoji.Known.ComputerDisk },
|
|
{ "computer_mouse", Emoji.Known.ComputerMouse },
|
|
{ "confetti_ball", Emoji.Known.ConfettiBall },
|
|
{ "confounded_face", Emoji.Known.ConfoundedFace },
|
|
{ "confused_face", Emoji.Known.ConfusedFace },
|
|
{ "construction", Emoji.Known.Construction },
|
|
{ "construction_worker", Emoji.Known.ConstructionWorker },
|
|
{ "control_knobs", Emoji.Known.ControlKnobs },
|
|
{ "convenience_store", Emoji.Known.ConvenienceStore },
|
|
{ "cooked_rice", Emoji.Known.CookedRice },
|
|
{ "cookie", Emoji.Known.Cookie },
|
|
{ "cooking", Emoji.Known.Cooking },
|
|
{ "cool_button", Emoji.Known.CoolButton },
|
|
{ "copyright", Emoji.Known.Copyright },
|
|
{ "couch_and_lamp", Emoji.Known.CouchAndLamp },
|
|
{ "counterclockwise_arrows_button", Emoji.Known.CounterclockwiseArrowsButton },
|
|
{ "couple_with_heart", Emoji.Known.CoupleWithHeart },
|
|
{ "cow", Emoji.Known.Cow },
|
|
{ "cowboy_hat_face", Emoji.Known.CowboyHatFace },
|
|
{ "cow_face", Emoji.Known.CowFace },
|
|
{ "crab", Emoji.Known.Crab },
|
|
{ "crayon", Emoji.Known.Crayon },
|
|
{ "credit_card", Emoji.Known.CreditCard },
|
|
{ "crescent_moon", Emoji.Known.CrescentMoon },
|
|
{ "cricket", Emoji.Known.Cricket },
|
|
{ "cricket_game", Emoji.Known.CricketGame },
|
|
{ "crocodile", Emoji.Known.Crocodile },
|
|
{ "croissant", Emoji.Known.Croissant },
|
|
{ "crossed_fingers", Emoji.Known.CrossedFingers },
|
|
{ "crossed_flags", Emoji.Known.CrossedFlags },
|
|
{ "crossed_swords", Emoji.Known.CrossedSwords },
|
|
{ "cross_mark", Emoji.Known.CrossMark },
|
|
{ "cross_mark_button", Emoji.Known.CrossMarkButton },
|
|
{ "crown", Emoji.Known.Crown },
|
|
{ "crying_cat", Emoji.Known.CryingCat },
|
|
{ "crying_face", Emoji.Known.CryingFace },
|
|
{ "crystal_ball", Emoji.Known.CrystalBall },
|
|
{ "cucumber", Emoji.Known.Cucumber },
|
|
{ "cupcake", Emoji.Known.Cupcake },
|
|
{ "cup_with_straw", Emoji.Known.CupWithStraw },
|
|
{ "curling_stone", Emoji.Known.CurlingStone },
|
|
{ "curly_hair", Emoji.Known.CurlyHair },
|
|
{ "curly_loop", Emoji.Known.CurlyLoop },
|
|
{ "currency_exchange", Emoji.Known.CurrencyExchange },
|
|
{ "curry_rice", Emoji.Known.CurryRice },
|
|
{ "custard", Emoji.Known.Custard },
|
|
{ "customs", Emoji.Known.Customs },
|
|
{ "cut_of_meat", Emoji.Known.CutOfMeat },
|
|
{ "cyclone", Emoji.Known.Cyclone },
|
|
{ "dagger", Emoji.Known.Dagger },
|
|
{ "dango", Emoji.Known.Dango },
|
|
{ "dashing_away", Emoji.Known.DashingAway },
|
|
{ "deaf_person", Emoji.Known.DeafPerson },
|
|
{ "deciduous_tree", Emoji.Known.DeciduousTree },
|
|
{ "deer", Emoji.Known.Deer },
|
|
{ "delivery_truck", Emoji.Known.DeliveryTruck },
|
|
{ "department_store", Emoji.Known.DepartmentStore },
|
|
{ "derelict_house", Emoji.Known.DerelictHouse },
|
|
{ "desert", Emoji.Known.Desert },
|
|
{ "desert_island", Emoji.Known.DesertIsland },
|
|
{ "desktop_computer", Emoji.Known.DesktopComputer },
|
|
{ "detective", Emoji.Known.Detective },
|
|
{ "diamond_suit", Emoji.Known.DiamondSuit },
|
|
{ "diamond_with_a_dot", Emoji.Known.DiamondWithADot },
|
|
{ "dim_button", Emoji.Known.DimButton },
|
|
{ "disappointed_face", Emoji.Known.DisappointedFace },
|
|
{ "disguised_face", Emoji.Known.DisguisedFace },
|
|
{ "divide", Emoji.Known.Divide },
|
|
{ "diving_mask", Emoji.Known.DivingMask },
|
|
{ "diya_lamp", Emoji.Known.DiyaLamp },
|
|
{ "dizzy", Emoji.Known.Dizzy },
|
|
{ "dna", Emoji.Known.Dna },
|
|
{ "dodo", Emoji.Known.Dodo },
|
|
{ "dog", Emoji.Known.Dog },
|
|
{ "dog_face", Emoji.Known.DogFace },
|
|
{ "dollar_banknote", Emoji.Known.DollarBanknote },
|
|
{ "dolphin", Emoji.Known.Dolphin },
|
|
{ "door", Emoji.Known.Door },
|
|
{ "dotted_six_pointed_star", Emoji.Known.DottedSixPointedStar },
|
|
{ "double_curly_loop", Emoji.Known.DoubleCurlyLoop },
|
|
{ "double_exclamation_mark", Emoji.Known.DoubleExclamationMark },
|
|
{ "doughnut", Emoji.Known.Doughnut },
|
|
{ "dove", Emoji.Known.Dove },
|
|
{ "down_arrow", Emoji.Known.DownArrow },
|
|
{ "downcast_face_with_sweat", Emoji.Known.DowncastFaceWithSweat },
|
|
{ "down_left_arrow", Emoji.Known.DownLeftArrow },
|
|
{ "down_right_arrow", Emoji.Known.DownRightArrow },
|
|
{ "downwards_button", Emoji.Known.DownwardsButton },
|
|
{ "dragon", Emoji.Known.Dragon },
|
|
{ "dragon_face", Emoji.Known.DragonFace },
|
|
{ "dress", Emoji.Known.Dress },
|
|
{ "drooling_face", Emoji.Known.DroolingFace },
|
|
{ "droplet", Emoji.Known.Droplet },
|
|
{ "drop_of_blood", Emoji.Known.DropOfBlood },
|
|
{ "drum", Emoji.Known.Drum },
|
|
{ "duck", Emoji.Known.Duck },
|
|
{ "dumpling", Emoji.Known.Dumpling },
|
|
{ "dvd", Emoji.Known.Dvd },
|
|
{ "eagle", Emoji.Known.Eagle },
|
|
{ "ear", Emoji.Known.Ear },
|
|
{ "ear_of_corn", Emoji.Known.EarOfCorn },
|
|
{ "ear_with_hearing_aid", Emoji.Known.EarWithHearingAid },
|
|
{ "egg", Emoji.Known.Egg },
|
|
{ "eggplant", Emoji.Known.Eggplant },
|
|
{ "eight_o_clock", Emoji.Known.EightOClock },
|
|
{ "eight_pointed_star", Emoji.Known.EightPointedStar },
|
|
{ "eight_spoked_asterisk", Emoji.Known.EightSpokedAsterisk },
|
|
{ "eight_thirty", Emoji.Known.EightThirty },
|
|
{ "eject_button", Emoji.Known.EjectButton },
|
|
{ "electric_plug", Emoji.Known.ElectricPlug },
|
|
{ "elephant", Emoji.Known.Elephant },
|
|
{ "elevator", Emoji.Known.Elevator },
|
|
{ "eleven_o_clock", Emoji.Known.ElevenOClock },
|
|
{ "eleven_thirty", Emoji.Known.ElevenThirty },
|
|
{ "elf", Emoji.Known.Elf },
|
|
{ "e_mail", Emoji.Known.EMail },
|
|
{ "end_arrow", Emoji.Known.EndArrow },
|
|
{ "envelope", Emoji.Known.Envelope },
|
|
{ "envelope_with_arrow", Emoji.Known.EnvelopeWithArrow },
|
|
{ "euro_banknote", Emoji.Known.EuroBanknote },
|
|
{ "evergreen_tree", Emoji.Known.EvergreenTree },
|
|
{ "ewe", Emoji.Known.Ewe },
|
|
{ "exclamation_question_mark", Emoji.Known.ExclamationQuestionMark },
|
|
{ "exploding_head", Emoji.Known.ExplodingHead },
|
|
{ "expressionless_face", Emoji.Known.ExpressionlessFace },
|
|
{ "eye", Emoji.Known.Eye },
|
|
{ "eyes", Emoji.Known.Eyes },
|
|
{ "face_blowing_a_kiss", Emoji.Known.FaceBlowingAKiss },
|
|
{ "face_savoring_food", Emoji.Known.FaceSavoringFood },
|
|
{ "face_screaming_in_fear", Emoji.Known.FaceScreamingInFear },
|
|
{ "face_vomiting", Emoji.Known.FaceVomiting },
|
|
{ "face_with_hand_over_mouth", Emoji.Known.FaceWithHandOverMouth },
|
|
{ "face_with_head_bandage", Emoji.Known.FaceWithHeadBandage },
|
|
{ "face_with_medical_mask", Emoji.Known.FaceWithMedicalMask },
|
|
{ "face_with_monocle", Emoji.Known.FaceWithMonocle },
|
|
{ "face_with_open_mouth", Emoji.Known.FaceWithOpenMouth },
|
|
{ "face_without_mouth", Emoji.Known.FaceWithoutMouth },
|
|
{ "face_with_raised_eyebrow", Emoji.Known.FaceWithRaisedEyebrow },
|
|
{ "face_with_rolling_eyes", Emoji.Known.FaceWithRollingEyes },
|
|
{ "face_with_steam_from_nose", Emoji.Known.FaceWithSteamFromNose },
|
|
{ "face_with_symbols_on_mouth", Emoji.Known.FaceWithSymbolsOnMouth },
|
|
{ "face_with_tears_of_joy", Emoji.Known.FaceWithTearsOfJoy },
|
|
{ "face_with_thermometer", Emoji.Known.FaceWithThermometer },
|
|
{ "face_with_tongue", Emoji.Known.FaceWithTongue },
|
|
{ "factory", Emoji.Known.Factory },
|
|
{ "fairy", Emoji.Known.Fairy },
|
|
{ "falafel", Emoji.Known.Falafel },
|
|
{ "fallen_leaf", Emoji.Known.FallenLeaf },
|
|
{ "family", Emoji.Known.Family },
|
|
{ "fast_down_button", Emoji.Known.FastDownButton },
|
|
{ "fast_forward_button", Emoji.Known.FastForwardButton },
|
|
{ "fast_reverse_button", Emoji.Known.FastReverseButton },
|
|
{ "fast_up_button", Emoji.Known.FastUpButton },
|
|
{ "fax_machine", Emoji.Known.FaxMachine },
|
|
{ "fearful_face", Emoji.Known.FearfulFace },
|
|
{ "feather", Emoji.Known.Feather },
|
|
{ "female_sign", Emoji.Known.FemaleSign },
|
|
{ "ferris_wheel", Emoji.Known.FerrisWheel },
|
|
{ "ferry", Emoji.Known.Ferry },
|
|
{ "field_hockey", Emoji.Known.FieldHockey },
|
|
{ "file_cabinet", Emoji.Known.FileCabinet },
|
|
{ "file_folder", Emoji.Known.FileFolder },
|
|
{ "film_frames", Emoji.Known.FilmFrames },
|
|
{ "film_projector", Emoji.Known.FilmProjector },
|
|
{ "fire", Emoji.Known.Fire },
|
|
{ "firecracker", Emoji.Known.Firecracker },
|
|
{ "fire_engine", Emoji.Known.FireEngine },
|
|
{ "fire_extinguisher", Emoji.Known.FireExtinguisher },
|
|
{ "fireworks", Emoji.Known.Fireworks },
|
|
{ "1st_place_medal", Emoji.Known.FirstPlaceMedal },
|
|
{ "first_quarter_moon", Emoji.Known.FirstQuarterMoon },
|
|
{ "first_quarter_moon_face", Emoji.Known.FirstQuarterMoonFace },
|
|
{ "fish", Emoji.Known.Fish },
|
|
{ "fish_cake_with_swirl", Emoji.Known.FishCakeWithSwirl },
|
|
{ "fishing_pole", Emoji.Known.FishingPole },
|
|
{ "five_o_clock", Emoji.Known.FiveOClock },
|
|
{ "five_thirty", Emoji.Known.FiveThirty },
|
|
{ "flag_in_hole", Emoji.Known.FlagInHole },
|
|
{ "flamingo", Emoji.Known.Flamingo },
|
|
{ "flashlight", Emoji.Known.Flashlight },
|
|
{ "flatbread", Emoji.Known.Flatbread },
|
|
{ "flat_shoe", Emoji.Known.FlatShoe },
|
|
{ "fleur_de_lis", Emoji.Known.FleurDeLis },
|
|
{ "flexed_biceps", Emoji.Known.FlexedBiceps },
|
|
{ "floppy_disk", Emoji.Known.FloppyDisk },
|
|
{ "flower_playing_cards", Emoji.Known.FlowerPlayingCards },
|
|
{ "flushed_face", Emoji.Known.FlushedFace },
|
|
{ "fly", Emoji.Known.Fly },
|
|
{ "flying_disc", Emoji.Known.FlyingDisc },
|
|
{ "flying_saucer", Emoji.Known.FlyingSaucer },
|
|
{ "fog", Emoji.Known.Fog },
|
|
{ "foggy", Emoji.Known.Foggy },
|
|
{ "folded_hands", Emoji.Known.FoldedHands },
|
|
{ "fondue", Emoji.Known.Fondue },
|
|
{ "foot", Emoji.Known.Foot },
|
|
{ "footprints", Emoji.Known.Footprints },
|
|
{ "fork_and_knife", Emoji.Known.ForkAndKnife },
|
|
{ "fork_and_knife_with_plate", Emoji.Known.ForkAndKnifeWithPlate },
|
|
{ "fortune_cookie", Emoji.Known.FortuneCookie },
|
|
{ "fountain", Emoji.Known.Fountain },
|
|
{ "fountain_pen", Emoji.Known.FountainPen },
|
|
{ "four_leaf_clover", Emoji.Known.FourLeafClover },
|
|
{ "four_o_clock", Emoji.Known.FourOClock },
|
|
{ "four_thirty", Emoji.Known.FourThirty },
|
|
{ "fox", Emoji.Known.Fox },
|
|
{ "framed_picture", Emoji.Known.FramedPicture },
|
|
{ "free_button", Emoji.Known.FreeButton },
|
|
{ "french_fries", Emoji.Known.FrenchFries },
|
|
{ "fried_shrimp", Emoji.Known.FriedShrimp },
|
|
{ "frog", Emoji.Known.Frog },
|
|
{ "front_facing_baby_chick", Emoji.Known.FrontFacingBabyChick },
|
|
{ "frowning_face", Emoji.Known.FrowningFace },
|
|
{ "frowning_face_with_open_mouth", Emoji.Known.FrowningFaceWithOpenMouth },
|
|
{ "fuel_pump", Emoji.Known.FuelPump },
|
|
{ "full_moon", Emoji.Known.FullMoon },
|
|
{ "full_moon_face", Emoji.Known.FullMoonFace },
|
|
{ "funeral_urn", Emoji.Known.FuneralUrn },
|
|
{ "game_die", Emoji.Known.GameDie },
|
|
{ "garlic", Emoji.Known.Garlic },
|
|
{ "gear", Emoji.Known.Gear },
|
|
{ "gemini", Emoji.Known.Gemini },
|
|
{ "gem_stone", Emoji.Known.GemStone },
|
|
{ "genie", Emoji.Known.Genie },
|
|
{ "ghost", Emoji.Known.Ghost },
|
|
{ "giraffe", Emoji.Known.Giraffe },
|
|
{ "girl", Emoji.Known.Girl },
|
|
{ "glasses", Emoji.Known.Glasses },
|
|
{ "glass_of_milk", Emoji.Known.GlassOfMilk },
|
|
{ "globe_showing_americas", Emoji.Known.GlobeShowingAmericas },
|
|
{ "globe_showing_asia_australia", Emoji.Known.GlobeShowingAsiaAustralia },
|
|
{ "globe_showing_europe_africa", Emoji.Known.GlobeShowingEuropeAfrica },
|
|
{ "globe_with_meridians", Emoji.Known.GlobeWithMeridians },
|
|
{ "gloves", Emoji.Known.Gloves },
|
|
{ "glowing_star", Emoji.Known.GlowingStar },
|
|
{ "goal_net", Emoji.Known.GoalNet },
|
|
{ "goat", Emoji.Known.Goat },
|
|
{ "goblin", Emoji.Known.Goblin },
|
|
{ "goggles", Emoji.Known.Goggles },
|
|
{ "gorilla", Emoji.Known.Gorilla },
|
|
{ "graduation_cap", Emoji.Known.GraduationCap },
|
|
{ "grapes", Emoji.Known.Grapes },
|
|
{ "green_apple", Emoji.Known.GreenApple },
|
|
{ "green_book", Emoji.Known.GreenBook },
|
|
{ "green_circle", Emoji.Known.GreenCircle },
|
|
{ "green_heart", Emoji.Known.GreenHeart },
|
|
{ "green_salad", Emoji.Known.GreenSalad },
|
|
{ "green_square", Emoji.Known.GreenSquare },
|
|
{ "grimacing_face", Emoji.Known.GrimacingFace },
|
|
{ "grinning_cat", Emoji.Known.GrinningCat },
|
|
{ "grinning_cat_with_smiling_eyes", Emoji.Known.GrinningCatWithSmilingEyes },
|
|
{ "grinning_face", Emoji.Known.GrinningFace },
|
|
{ "grinning_face_with_big_eyes", Emoji.Known.GrinningFaceWithBigEyes },
|
|
{ "grinning_face_with_smiling_eyes", Emoji.Known.GrinningFaceWithSmilingEyes },
|
|
{ "grinning_face_with_sweat", Emoji.Known.GrinningFaceWithSweat },
|
|
{ "grinning_squinting_face", Emoji.Known.GrinningSquintingFace },
|
|
{ "growing_heart", Emoji.Known.GrowingHeart },
|
|
{ "guard", Emoji.Known.Guard },
|
|
{ "guide_dog", Emoji.Known.GuideDog },
|
|
{ "guitar", Emoji.Known.Guitar },
|
|
{ "hamburger", Emoji.Known.Hamburger },
|
|
{ "hammer", Emoji.Known.Hammer },
|
|
{ "hammer_and_pick", Emoji.Known.HammerAndPick },
|
|
{ "hammer_and_wrench", Emoji.Known.HammerAndWrench },
|
|
{ "hamster", Emoji.Known.Hamster },
|
|
{ "handbag", Emoji.Known.Handbag },
|
|
{ "handshake", Emoji.Known.Handshake },
|
|
{ "hand_with_fingers_splayed", Emoji.Known.HandWithFingersSplayed },
|
|
{ "hatching_chick", Emoji.Known.HatchingChick },
|
|
{ "headphone", Emoji.Known.Headphone },
|
|
{ "headstone", Emoji.Known.Headstone },
|
|
{ "hear_no_evil_monkey", Emoji.Known.HearNoEvilMonkey },
|
|
{ "heart_decoration", Emoji.Known.HeartDecoration },
|
|
{ "heart_exclamation", Emoji.Known.HeartExclamation },
|
|
{ "heart_suit", Emoji.Known.HeartSuit },
|
|
{ "heart_with_arrow", Emoji.Known.HeartWithArrow },
|
|
{ "heart_with_ribbon", Emoji.Known.HeartWithRibbon },
|
|
{ "heavy_dollar_sign", Emoji.Known.HeavyDollarSign },
|
|
{ "hedgehog", Emoji.Known.Hedgehog },
|
|
{ "helicopter", Emoji.Known.Helicopter },
|
|
{ "herb", Emoji.Known.Herb },
|
|
{ "hibiscus", Emoji.Known.Hibiscus },
|
|
{ "high_heeled_shoe", Emoji.Known.HighHeeledShoe },
|
|
{ "high_speed_train", Emoji.Known.HighSpeedTrain },
|
|
{ "high_voltage", Emoji.Known.HighVoltage },
|
|
{ "hiking_boot", Emoji.Known.HikingBoot },
|
|
{ "hindu_temple", Emoji.Known.HinduTemple },
|
|
{ "hippopotamus", Emoji.Known.Hippopotamus },
|
|
{ "hole", Emoji.Known.Hole },
|
|
{ "hollow_red_circle", Emoji.Known.HollowRedCircle },
|
|
{ "honeybee", Emoji.Known.Honeybee },
|
|
{ "honey_pot", Emoji.Known.HoneyPot },
|
|
{ "hook", Emoji.Known.Hook },
|
|
{ "horizontal_traffic_light", Emoji.Known.HorizontalTrafficLight },
|
|
{ "horse", Emoji.Known.Horse },
|
|
{ "horse_face", Emoji.Known.HorseFace },
|
|
{ "horse_racing", Emoji.Known.HorseRacing },
|
|
{ "hospital", Emoji.Known.Hospital },
|
|
{ "hot_beverage", Emoji.Known.HotBeverage },
|
|
{ "hot_dog", Emoji.Known.HotDog },
|
|
{ "hotel", Emoji.Known.Hotel },
|
|
{ "hot_face", Emoji.Known.HotFace },
|
|
{ "hot_pepper", Emoji.Known.HotPepper },
|
|
{ "hot_springs", Emoji.Known.HotSprings },
|
|
{ "hourglass_done", Emoji.Known.HourglassDone },
|
|
{ "hourglass_not_done", Emoji.Known.HourglassNotDone },
|
|
{ "house", Emoji.Known.House },
|
|
{ "houses", Emoji.Known.Houses },
|
|
{ "house_with_garden", Emoji.Known.HouseWithGarden },
|
|
{ "hugging_face", Emoji.Known.HuggingFace },
|
|
{ "hundred_points", Emoji.Known.HundredPoints },
|
|
{ "hushed_face", Emoji.Known.HushedFace },
|
|
{ "hut", Emoji.Known.Hut },
|
|
{ "ice", Emoji.Known.Ice },
|
|
{ "ice_cream", Emoji.Known.IceCream },
|
|
{ "ice_hockey", Emoji.Known.IceHockey },
|
|
{ "ice_skate", Emoji.Known.IceSkate },
|
|
{ "id_button", Emoji.Known.IdButton },
|
|
{ "inbox_tray", Emoji.Known.InboxTray },
|
|
{ "incoming_envelope", Emoji.Known.IncomingEnvelope },
|
|
{ "index_pointing_up", Emoji.Known.IndexPointingUp },
|
|
{ "infinity", Emoji.Known.Infinity },
|
|
{ "information", Emoji.Known.Information },
|
|
{ "input_latin_letters", Emoji.Known.InputLatinLetters },
|
|
{ "input_latin_lowercase", Emoji.Known.InputLatinLowercase },
|
|
{ "input_latin_uppercase", Emoji.Known.InputLatinUppercase },
|
|
{ "input_numbers", Emoji.Known.InputNumbers },
|
|
{ "input_symbols", Emoji.Known.InputSymbols },
|
|
{ "jack_o_lantern", Emoji.Known.JackOLantern },
|
|
{ "japanese_acceptable_button", Emoji.Known.JapaneseAcceptableButton },
|
|
{ "japanese_application_button", Emoji.Known.JapaneseApplicationButton },
|
|
{ "japanese_bargain_button", Emoji.Known.JapaneseBargainButton },
|
|
{ "japanese_castle", Emoji.Known.JapaneseCastle },
|
|
{ "japanese_congratulations_button", Emoji.Known.JapaneseCongratulationsButton },
|
|
{ "japanese_discount_button", Emoji.Known.JapaneseDiscountButton },
|
|
{ "japanese_dolls", Emoji.Known.JapaneseDolls },
|
|
{ "japanese_free_of_charge_button", Emoji.Known.JapaneseFreeOfChargeButton },
|
|
{ "japanese_here_button", Emoji.Known.JapaneseHereButton },
|
|
{ "japanese_monthly_amount_button", Emoji.Known.JapaneseMonthlyAmountButton },
|
|
{ "japanese_not_free_of_charge_button", Emoji.Known.JapaneseNotFreeOfChargeButton },
|
|
{ "japanese_no_vacancy_button", Emoji.Known.JapaneseNoVacancyButton },
|
|
{ "japanese_open_for_business_button", Emoji.Known.JapaneseOpenForBusinessButton },
|
|
{ "japanese_passing_grade_button", Emoji.Known.JapanesePassingGradeButton },
|
|
{ "japanese_post_office", Emoji.Known.JapanesePostOffice },
|
|
{ "japanese_prohibited_button", Emoji.Known.JapaneseProhibitedButton },
|
|
{ "japanese_reserved_button", Emoji.Known.JapaneseReservedButton },
|
|
{ "japanese_secret_button", Emoji.Known.JapaneseSecretButton },
|
|
{ "japanese_service_charge_button", Emoji.Known.JapaneseServiceChargeButton },
|
|
{ "japanese_symbol_for_beginner", Emoji.Known.JapaneseSymbolForBeginner },
|
|
{ "japanese_vacancy_button", Emoji.Known.JapaneseVacancyButton },
|
|
{ "jeans", Emoji.Known.Jeans },
|
|
{ "joker", Emoji.Known.Joker },
|
|
{ "joystick", Emoji.Known.Joystick },
|
|
{ "kaaba", Emoji.Known.Kaaba },
|
|
{ "kangaroo", Emoji.Known.Kangaroo },
|
|
{ "key", Emoji.Known.Key },
|
|
{ "keyboard", Emoji.Known.Keyboard },
|
|
{ "keycap_10", Emoji.Known.Keycap10 },
|
|
{ "kick_scooter", Emoji.Known.KickScooter },
|
|
{ "kimono", Emoji.Known.Kimono },
|
|
{ "kiss", Emoji.Known.Kiss },
|
|
{ "kissing_cat", Emoji.Known.KissingCat },
|
|
{ "kissing_face", Emoji.Known.KissingFace },
|
|
{ "kissing_face_with_closed_eyes", Emoji.Known.KissingFaceWithClosedEyes },
|
|
{ "kissing_face_with_smiling_eyes", Emoji.Known.KissingFaceWithSmilingEyes },
|
|
{ "kiss_mark", Emoji.Known.KissMark },
|
|
{ "kitchen_knife", Emoji.Known.KitchenKnife },
|
|
{ "kite", Emoji.Known.Kite },
|
|
{ "kiwi_fruit", Emoji.Known.KiwiFruit },
|
|
{ "knocked_out_face", Emoji.Known.KnockedOutFace },
|
|
{ "knot", Emoji.Known.Knot },
|
|
{ "koala", Emoji.Known.Koala },
|
|
{ "lab_coat", Emoji.Known.LabCoat },
|
|
{ "label", Emoji.Known.Label },
|
|
{ "lacrosse", Emoji.Known.Lacrosse },
|
|
{ "ladder", Emoji.Known.Ladder },
|
|
{ "lady_beetle", Emoji.Known.LadyBeetle },
|
|
{ "laptop", Emoji.Known.Laptop },
|
|
{ "large_blue_diamond", Emoji.Known.LargeBlueDiamond },
|
|
{ "large_orange_diamond", Emoji.Known.LargeOrangeDiamond },
|
|
{ "last_quarter_moon", Emoji.Known.LastQuarterMoon },
|
|
{ "last_quarter_moon_face", Emoji.Known.LastQuarterMoonFace },
|
|
{ "last_track_button", Emoji.Known.LastTrackButton },
|
|
{ "latin_cross", Emoji.Known.LatinCross },
|
|
{ "leaf_fluttering_in_wind", Emoji.Known.LeafFlutteringInWind },
|
|
{ "leafy_green", Emoji.Known.LeafyGreen },
|
|
{ "ledger", Emoji.Known.Ledger },
|
|
{ "left_arrow", Emoji.Known.LeftArrow },
|
|
{ "left_arrow_curving_right", Emoji.Known.LeftArrowCurvingRight },
|
|
{ "left_facing_fist", Emoji.Known.LeftFacingFist },
|
|
{ "left_luggage", Emoji.Known.LeftLuggage },
|
|
{ "left_right_arrow", Emoji.Known.LeftRightArrow },
|
|
{ "left_speech_bubble", Emoji.Known.LeftSpeechBubble },
|
|
{ "leg", Emoji.Known.Leg },
|
|
{ "lemon", Emoji.Known.Lemon },
|
|
{ "leo", Emoji.Known.Leo },
|
|
{ "leopard", Emoji.Known.Leopard },
|
|
{ "level_slider", Emoji.Known.LevelSlider },
|
|
{ "libra", Emoji.Known.Libra },
|
|
{ "light_bulb", Emoji.Known.LightBulb },
|
|
{ "light_rail", Emoji.Known.LightRail },
|
|
{ "link", Emoji.Known.Link },
|
|
{ "linked_paperclips", Emoji.Known.LinkedPaperclips },
|
|
{ "lion", Emoji.Known.Lion },
|
|
{ "lipstick", Emoji.Known.Lipstick },
|
|
{ "litter_in_bin_sign", Emoji.Known.LitterInBinSign },
|
|
{ "lizard", Emoji.Known.Lizard },
|
|
{ "llama", Emoji.Known.Llama },
|
|
{ "lobster", Emoji.Known.Lobster },
|
|
{ "locked", Emoji.Known.Locked },
|
|
{ "locked_with_key", Emoji.Known.LockedWithKey },
|
|
{ "locked_with_pen", Emoji.Known.LockedWithPen },
|
|
{ "locomotive", Emoji.Known.Locomotive },
|
|
{ "lollipop", Emoji.Known.Lollipop },
|
|
{ "long_drum", Emoji.Known.LongDrum },
|
|
{ "lotion_bottle", Emoji.Known.LotionBottle },
|
|
{ "loudly_crying_face", Emoji.Known.LoudlyCryingFace },
|
|
{ "loudspeaker", Emoji.Known.Loudspeaker },
|
|
{ "love_hotel", Emoji.Known.LoveHotel },
|
|
{ "love_letter", Emoji.Known.LoveLetter },
|
|
{ "love_you_gesture", Emoji.Known.LoveYouGesture },
|
|
{ "luggage", Emoji.Known.Luggage },
|
|
{ "lungs", Emoji.Known.Lungs },
|
|
{ "lying_face", Emoji.Known.LyingFace },
|
|
{ "mage", Emoji.Known.Mage },
|
|
{ "magic_wand", Emoji.Known.MagicWand },
|
|
{ "magnet", Emoji.Known.Magnet },
|
|
{ "magnifying_glass_tilted_left", Emoji.Known.MagnifyingGlassTiltedLeft },
|
|
{ "magnifying_glass_tilted_right", Emoji.Known.MagnifyingGlassTiltedRight },
|
|
{ "mahjong_red_dragon", Emoji.Known.MahjongRedDragon },
|
|
{ "male_sign", Emoji.Known.MaleSign },
|
|
{ "mammoth", Emoji.Known.Mammoth },
|
|
{ "man", Emoji.Known.Man },
|
|
{ "man_dancing", Emoji.Known.ManDancing },
|
|
{ "mango", Emoji.Known.Mango },
|
|
{ "mans_shoe", Emoji.Known.MansShoe },
|
|
{ "mantelpiece_clock", Emoji.Known.MantelpieceClock },
|
|
{ "manual_wheelchair", Emoji.Known.ManualWheelchair },
|
|
{ "maple_leaf", Emoji.Known.MapleLeaf },
|
|
{ "map_of_japan", Emoji.Known.MapOfJapan },
|
|
{ "martial_arts_uniform", Emoji.Known.MartialArtsUniform },
|
|
{ "mate", Emoji.Known.Mate },
|
|
{ "meat_on_bone", Emoji.Known.MeatOnBone },
|
|
{ "mechanical_arm", Emoji.Known.MechanicalArm },
|
|
{ "mechanical_leg", Emoji.Known.MechanicalLeg },
|
|
{ "medical_symbol", Emoji.Known.MedicalSymbol },
|
|
{ "megaphone", Emoji.Known.Megaphone },
|
|
{ "melon", Emoji.Known.Melon },
|
|
{ "memo", Emoji.Known.Memo },
|
|
{ "men_holding_hands", Emoji.Known.MenHoldingHands },
|
|
{ "menorah", Emoji.Known.Menorah },
|
|
{ "mens_room", Emoji.Known.MensRoom },
|
|
{ "merperson", Emoji.Known.Merperson },
|
|
{ "metro", Emoji.Known.Metro },
|
|
{ "microbe", Emoji.Known.Microbe },
|
|
{ "microphone", Emoji.Known.Microphone },
|
|
{ "microscope", Emoji.Known.Microscope },
|
|
{ "middle_finger", Emoji.Known.MiddleFinger },
|
|
{ "military_helmet", Emoji.Known.MilitaryHelmet },
|
|
{ "military_medal", Emoji.Known.MilitaryMedal },
|
|
{ "milky_way", Emoji.Known.MilkyWay },
|
|
{ "minibus", Emoji.Known.Minibus },
|
|
{ "minus", Emoji.Known.Minus },
|
|
{ "mirror", Emoji.Known.Mirror },
|
|
{ "moai", Emoji.Known.Moai },
|
|
{ "mobile_phone", Emoji.Known.MobilePhone },
|
|
{ "mobile_phone_off", Emoji.Known.MobilePhoneOff },
|
|
{ "mobile_phone_with_arrow", Emoji.Known.MobilePhoneWithArrow },
|
|
{ "money_bag", Emoji.Known.MoneyBag },
|
|
{ "money_mouth_face", Emoji.Known.MoneyMouthFace },
|
|
{ "money_with_wings", Emoji.Known.MoneyWithWings },
|
|
{ "monkey", Emoji.Known.Monkey },
|
|
{ "monkey_face", Emoji.Known.MonkeyFace },
|
|
{ "monorail", Emoji.Known.Monorail },
|
|
{ "moon_cake", Emoji.Known.MoonCake },
|
|
{ "moon_viewing_ceremony", Emoji.Known.MoonViewingCeremony },
|
|
{ "mosque", Emoji.Known.Mosque },
|
|
{ "mosquito", Emoji.Known.Mosquito },
|
|
{ "motor_boat", Emoji.Known.MotorBoat },
|
|
{ "motorcycle", Emoji.Known.Motorcycle },
|
|
{ "motorized_wheelchair", Emoji.Known.MotorizedWheelchair },
|
|
{ "motor_scooter", Emoji.Known.MotorScooter },
|
|
{ "motorway", Emoji.Known.Motorway },
|
|
{ "mountain", Emoji.Known.Mountain },
|
|
{ "mountain_cableway", Emoji.Known.MountainCableway },
|
|
{ "mountain_railway", Emoji.Known.MountainRailway },
|
|
{ "mount_fuji", Emoji.Known.MountFuji },
|
|
{ "mouse", Emoji.Known.Mouse },
|
|
{ "mouse_face", Emoji.Known.MouseFace },
|
|
{ "mouse_trap", Emoji.Known.MouseTrap },
|
|
{ "mouth", Emoji.Known.Mouth },
|
|
{ "movie_camera", Emoji.Known.MovieCamera },
|
|
{ "mrs_claus", Emoji.Known.MrsClaus },
|
|
{ "multiply", Emoji.Known.Multiply },
|
|
{ "mushroom", Emoji.Known.Mushroom },
|
|
{ "musical_keyboard", Emoji.Known.MusicalKeyboard },
|
|
{ "musical_note", Emoji.Known.MusicalNote },
|
|
{ "musical_notes", Emoji.Known.MusicalNotes },
|
|
{ "musical_score", Emoji.Known.MusicalScore },
|
|
{ "muted_speaker", Emoji.Known.MutedSpeaker },
|
|
{ "nail_polish", Emoji.Known.NailPolish },
|
|
{ "name_badge", Emoji.Known.NameBadge },
|
|
{ "national_park", Emoji.Known.NationalPark },
|
|
{ "nauseated_face", Emoji.Known.NauseatedFace },
|
|
{ "nazar_amulet", Emoji.Known.NazarAmulet },
|
|
{ "necktie", Emoji.Known.Necktie },
|
|
{ "nerd_face", Emoji.Known.NerdFace },
|
|
{ "nesting_dolls", Emoji.Known.NestingDolls },
|
|
{ "neutral_face", Emoji.Known.NeutralFace },
|
|
{ "new_button", Emoji.Known.NewButton },
|
|
{ "new_moon", Emoji.Known.NewMoon },
|
|
{ "new_moon_face", Emoji.Known.NewMoonFace },
|
|
{ "newspaper", Emoji.Known.Newspaper },
|
|
{ "next_track_button", Emoji.Known.NextTrackButton },
|
|
{ "ng_button", Emoji.Known.NgButton },
|
|
{ "night_with_stars", Emoji.Known.NightWithStars },
|
|
{ "nine_o_clock", Emoji.Known.NineOClock },
|
|
{ "nine_thirty", Emoji.Known.NineThirty },
|
|
{ "ninja", Emoji.Known.Ninja },
|
|
{ "no_bicycles", Emoji.Known.NoBicycles },
|
|
{ "no_entry", Emoji.Known.NoEntry },
|
|
{ "no_littering", Emoji.Known.NoLittering },
|
|
{ "no_mobile_phones", Emoji.Known.NoMobilePhones },
|
|
{ "non_potable_water", Emoji.Known.NonPotableWater },
|
|
{ "no_one_under_eighteen", Emoji.Known.NoOneUnderEighteen },
|
|
{ "no_pedestrians", Emoji.Known.NoPedestrians },
|
|
{ "nose", Emoji.Known.Nose },
|
|
{ "no_smoking", Emoji.Known.NoSmoking },
|
|
{ "notebook", Emoji.Known.Notebook },
|
|
{ "notebook_with_decorative_cover", Emoji.Known.NotebookWithDecorativeCover },
|
|
{ "nut_and_bolt", Emoji.Known.NutAndBolt },
|
|
{ "o_button_blood_type", Emoji.Known.OButtonBloodType },
|
|
{ "octopus", Emoji.Known.Octopus },
|
|
{ "oden", Emoji.Known.Oden },
|
|
{ "office_building", Emoji.Known.OfficeBuilding },
|
|
{ "ogre", Emoji.Known.Ogre },
|
|
{ "oil_drum", Emoji.Known.OilDrum },
|
|
{ "ok_button", Emoji.Known.OkButton },
|
|
{ "ok_hand", Emoji.Known.OkHand },
|
|
{ "older_person", Emoji.Known.OlderPerson },
|
|
{ "old_key", Emoji.Known.OldKey },
|
|
{ "old_man", Emoji.Known.OldMan },
|
|
{ "old_woman", Emoji.Known.OldWoman },
|
|
{ "olive", Emoji.Known.Olive },
|
|
{ "om", Emoji.Known.Om },
|
|
{ "on_arrow", Emoji.Known.OnArrow },
|
|
{ "oncoming_automobile", Emoji.Known.OncomingAutomobile },
|
|
{ "oncoming_bus", Emoji.Known.OncomingBus },
|
|
{ "oncoming_fist", Emoji.Known.OncomingFist },
|
|
{ "oncoming_police_car", Emoji.Known.OncomingPoliceCar },
|
|
{ "oncoming_taxi", Emoji.Known.OncomingTaxi },
|
|
{ "one_o_clock", Emoji.Known.OneOClock },
|
|
{ "one_piece_swimsuit", Emoji.Known.OnePieceSwimsuit },
|
|
{ "one_thirty", Emoji.Known.OneThirty },
|
|
{ "onion", Emoji.Known.Onion },
|
|
{ "open_book", Emoji.Known.OpenBook },
|
|
{ "open_file_folder", Emoji.Known.OpenFileFolder },
|
|
{ "open_hands", Emoji.Known.OpenHands },
|
|
{ "open_mailbox_with_lowered_flag", Emoji.Known.OpenMailboxWithLoweredFlag },
|
|
{ "open_mailbox_with_raised_flag", Emoji.Known.OpenMailboxWithRaisedFlag },
|
|
{ "ophiuchus", Emoji.Known.Ophiuchus },
|
|
{ "optical_disk", Emoji.Known.OpticalDisk },
|
|
{ "orange_book", Emoji.Known.OrangeBook },
|
|
{ "orange_circle", Emoji.Known.OrangeCircle },
|
|
{ "orange_heart", Emoji.Known.OrangeHeart },
|
|
{ "orange_square", Emoji.Known.OrangeSquare },
|
|
{ "orangutan", Emoji.Known.Orangutan },
|
|
{ "orthodox_cross", Emoji.Known.OrthodoxCross },
|
|
{ "otter", Emoji.Known.Otter },
|
|
{ "outbox_tray", Emoji.Known.OutboxTray },
|
|
{ "owl", Emoji.Known.Owl },
|
|
{ "ox", Emoji.Known.Ox },
|
|
{ "oyster", Emoji.Known.Oyster },
|
|
{ "package", Emoji.Known.Package },
|
|
{ "page_facing_up", Emoji.Known.PageFacingUp },
|
|
{ "pager", Emoji.Known.Pager },
|
|
{ "page_with_curl", Emoji.Known.PageWithCurl },
|
|
{ "paintbrush", Emoji.Known.Paintbrush },
|
|
{ "palms_up_together", Emoji.Known.PalmsUpTogether },
|
|
{ "palm_tree", Emoji.Known.PalmTree },
|
|
{ "pancakes", Emoji.Known.Pancakes },
|
|
{ "panda", Emoji.Known.Panda },
|
|
{ "paperclip", Emoji.Known.Paperclip },
|
|
{ "parachute", Emoji.Known.Parachute },
|
|
{ "parrot", Emoji.Known.Parrot },
|
|
{ "part_alternation_mark", Emoji.Known.PartAlternationMark },
|
|
{ "partying_face", Emoji.Known.PartyingFace },
|
|
{ "party_popper", Emoji.Known.PartyPopper },
|
|
{ "passenger_ship", Emoji.Known.PassengerShip },
|
|
{ "passport_control", Emoji.Known.PassportControl },
|
|
{ "pause_button", Emoji.Known.PauseButton },
|
|
{ "paw_prints", Emoji.Known.PawPrints },
|
|
{ "p_button", Emoji.Known.PButton },
|
|
{ "peace_symbol", Emoji.Known.PeaceSymbol },
|
|
{ "peach", Emoji.Known.Peach },
|
|
{ "peacock", Emoji.Known.Peacock },
|
|
{ "peanuts", Emoji.Known.Peanuts },
|
|
{ "pear", Emoji.Known.Pear },
|
|
{ "pen", Emoji.Known.Pen },
|
|
{ "pencil", Emoji.Known.Pencil },
|
|
{ "penguin", Emoji.Known.Penguin },
|
|
{ "pensive_face", Emoji.Known.PensiveFace },
|
|
{ "people_hugging", Emoji.Known.PeopleHugging },
|
|
{ "people_with_bunny_ears", Emoji.Known.PeopleWithBunnyEars },
|
|
{ "people_wrestling", Emoji.Known.PeopleWrestling },
|
|
{ "performing_arts", Emoji.Known.PerformingArts },
|
|
{ "persevering_face", Emoji.Known.PerseveringFace },
|
|
{ "person", Emoji.Known.Person },
|
|
{ "person_beard", Emoji.Known.PersonBeard },
|
|
{ "person_biking", Emoji.Known.PersonBiking },
|
|
{ "person_blond_hair", Emoji.Known.PersonBlondHair },
|
|
{ "person_bouncing_ball", Emoji.Known.PersonBouncingBall },
|
|
{ "person_bowing", Emoji.Known.PersonBowing },
|
|
{ "person_cartwheeling", Emoji.Known.PersonCartwheeling },
|
|
{ "person_climbing", Emoji.Known.PersonClimbing },
|
|
{ "person_facepalming", Emoji.Known.PersonFacepalming },
|
|
{ "person_fencing", Emoji.Known.PersonFencing },
|
|
{ "person_frowning", Emoji.Known.PersonFrowning },
|
|
{ "person_gesturing_no", Emoji.Known.PersonGesturingNo },
|
|
{ "person_gesturing_ok", Emoji.Known.PersonGesturingOk },
|
|
{ "person_getting_haircut", Emoji.Known.PersonGettingHaircut },
|
|
{ "person_getting_massage", Emoji.Known.PersonGettingMassage },
|
|
{ "person_golfing", Emoji.Known.PersonGolfing },
|
|
{ "person_in_bed", Emoji.Known.PersonInBed },
|
|
{ "person_in_lotus_position", Emoji.Known.PersonInLotusPosition },
|
|
{ "person_in_steamy_room", Emoji.Known.PersonInSteamyRoom },
|
|
{ "person_in_suit_levitating", Emoji.Known.PersonInSuitLevitating },
|
|
{ "person_in_tuxedo", Emoji.Known.PersonInTuxedo },
|
|
{ "person_juggling", Emoji.Known.PersonJuggling },
|
|
{ "person_kneeling", Emoji.Known.PersonKneeling },
|
|
{ "person_lifting_weights", Emoji.Known.PersonLiftingWeights },
|
|
{ "person_mountain_biking", Emoji.Known.PersonMountainBiking },
|
|
{ "person_playing_handball", Emoji.Known.PersonPlayingHandball },
|
|
{ "person_playing_water_polo", Emoji.Known.PersonPlayingWaterPolo },
|
|
{ "person_pouting", Emoji.Known.PersonPouting },
|
|
{ "person_raising_hand", Emoji.Known.PersonRaisingHand },
|
|
{ "person_rowing_boat", Emoji.Known.PersonRowingBoat },
|
|
{ "person_running", Emoji.Known.PersonRunning },
|
|
{ "person_shrugging", Emoji.Known.PersonShrugging },
|
|
{ "person_standing", Emoji.Known.PersonStanding },
|
|
{ "person_surfing", Emoji.Known.PersonSurfing },
|
|
{ "person_swimming", Emoji.Known.PersonSwimming },
|
|
{ "person_taking_bath", Emoji.Known.PersonTakingBath },
|
|
{ "person_tipping_hand", Emoji.Known.PersonTippingHand },
|
|
{ "person_walking", Emoji.Known.PersonWalking },
|
|
{ "person_wearing_turban", Emoji.Known.PersonWearingTurban },
|
|
{ "person_with_skullcap", Emoji.Known.PersonWithSkullcap },
|
|
{ "person_with_veil", Emoji.Known.PersonWithVeil },
|
|
{ "petri_dish", Emoji.Known.PetriDish },
|
|
{ "pick", Emoji.Known.Pick },
|
|
{ "pickup_truck", Emoji.Known.PickupTruck },
|
|
{ "pie", Emoji.Known.Pie },
|
|
{ "pig", Emoji.Known.Pig },
|
|
{ "pig_face", Emoji.Known.PigFace },
|
|
{ "pig_nose", Emoji.Known.PigNose },
|
|
{ "pile_of_poo", Emoji.Known.PileOfPoo },
|
|
{ "pill", Emoji.Known.Pill },
|
|
{ "piñata", Emoji.Known.Piñata },
|
|
{ "pinched_fingers", Emoji.Known.PinchedFingers },
|
|
{ "pinching_hand", Emoji.Known.PinchingHand },
|
|
{ "pineapple", Emoji.Known.Pineapple },
|
|
{ "pine_decoration", Emoji.Known.PineDecoration },
|
|
{ "ping_pong", Emoji.Known.PingPong },
|
|
{ "pisces", Emoji.Known.Pisces },
|
|
{ "pizza", Emoji.Known.Pizza },
|
|
{ "placard", Emoji.Known.Placard },
|
|
{ "place_of_worship", Emoji.Known.PlaceOfWorship },
|
|
{ "play_button", Emoji.Known.PlayButton },
|
|
{ "play_or_pause_button", Emoji.Known.PlayOrPauseButton },
|
|
{ "pleading_face", Emoji.Known.PleadingFace },
|
|
{ "plunger", Emoji.Known.Plunger },
|
|
{ "plus", Emoji.Known.Plus },
|
|
{ "police_car", Emoji.Known.PoliceCar },
|
|
{ "police_car_light", Emoji.Known.PoliceCarLight },
|
|
{ "police_officer", Emoji.Known.PoliceOfficer },
|
|
{ "poodle", Emoji.Known.Poodle },
|
|
{ "pool_8_ball", Emoji.Known.Pool8Ball },
|
|
{ "popcorn", Emoji.Known.Popcorn },
|
|
{ "postal_horn", Emoji.Known.PostalHorn },
|
|
{ "postbox", Emoji.Known.Postbox },
|
|
{ "post_office", Emoji.Known.PostOffice },
|
|
{ "potable_water", Emoji.Known.PotableWater },
|
|
{ "potato", Emoji.Known.Potato },
|
|
{ "pot_of_food", Emoji.Known.PotOfFood },
|
|
{ "potted_plant", Emoji.Known.PottedPlant },
|
|
{ "poultry_leg", Emoji.Known.PoultryLeg },
|
|
{ "pound_banknote", Emoji.Known.PoundBanknote },
|
|
{ "pouting_cat", Emoji.Known.PoutingCat },
|
|
{ "pouting_face", Emoji.Known.PoutingFace },
|
|
{ "prayer_beads", Emoji.Known.PrayerBeads },
|
|
{ "pregnant_woman", Emoji.Known.PregnantWoman },
|
|
{ "pretzel", Emoji.Known.Pretzel },
|
|
{ "prince", Emoji.Known.Prince },
|
|
{ "princess", Emoji.Known.Princess },
|
|
{ "printer", Emoji.Known.Printer },
|
|
{ "prohibited", Emoji.Known.Prohibited },
|
|
{ "purple_circle", Emoji.Known.PurpleCircle },
|
|
{ "purple_heart", Emoji.Known.PurpleHeart },
|
|
{ "purple_square", Emoji.Known.PurpleSquare },
|
|
{ "purse", Emoji.Known.Purse },
|
|
{ "pushpin", Emoji.Known.Pushpin },
|
|
{ "puzzle_piece", Emoji.Known.PuzzlePiece },
|
|
{ "rabbit", Emoji.Known.Rabbit },
|
|
{ "rabbit_face", Emoji.Known.RabbitFace },
|
|
{ "raccoon", Emoji.Known.Raccoon },
|
|
{ "racing_car", Emoji.Known.RacingCar },
|
|
{ "radio", Emoji.Known.Radio },
|
|
{ "radioactive", Emoji.Known.Radioactive },
|
|
{ "radio_button", Emoji.Known.RadioButton },
|
|
{ "railway_car", Emoji.Known.RailwayCar },
|
|
{ "railway_track", Emoji.Known.RailwayTrack },
|
|
{ "rainbow", Emoji.Known.Rainbow },
|
|
{ "raised_back_of_hand", Emoji.Known.RaisedBackOfHand },
|
|
{ "raised_fist", Emoji.Known.RaisedFist },
|
|
{ "raised_hand", Emoji.Known.RaisedHand },
|
|
{ "raising_hands", Emoji.Known.RaisingHands },
|
|
{ "ram", Emoji.Known.Ram },
|
|
{ "rat", Emoji.Known.Rat },
|
|
{ "razor", Emoji.Known.Razor },
|
|
{ "receipt", Emoji.Known.Receipt },
|
|
{ "record_button", Emoji.Known.RecordButton },
|
|
{ "recycling_symbol", Emoji.Known.RecyclingSymbol },
|
|
{ "red_apple", Emoji.Known.RedApple },
|
|
{ "red_circle", Emoji.Known.RedCircle },
|
|
{ "red_envelope", Emoji.Known.RedEnvelope },
|
|
{ "red_exclamation_mark", Emoji.Known.RedExclamationMark },
|
|
{ "red_hair", Emoji.Known.RedHair },
|
|
{ "red_heart", Emoji.Known.RedHeart },
|
|
{ "red_paper_lantern", Emoji.Known.RedPaperLantern },
|
|
{ "red_question_mark", Emoji.Known.RedQuestionMark },
|
|
{ "red_square", Emoji.Known.RedSquare },
|
|
{ "red_triangle_pointed_down", Emoji.Known.RedTrianglePointedDown },
|
|
{ "red_triangle_pointed_up", Emoji.Known.RedTrianglePointedUp },
|
|
{ "registered", Emoji.Known.Registered },
|
|
{ "relieved_face", Emoji.Known.RelievedFace },
|
|
{ "reminder_ribbon", Emoji.Known.ReminderRibbon },
|
|
{ "repeat_button", Emoji.Known.RepeatButton },
|
|
{ "repeat_single_button", Emoji.Known.RepeatSingleButton },
|
|
{ "rescue_workers_helmet", Emoji.Known.RescueWorkersHelmet },
|
|
{ "restroom", Emoji.Known.Restroom },
|
|
{ "reverse_button", Emoji.Known.ReverseButton },
|
|
{ "revolving_hearts", Emoji.Known.RevolvingHearts },
|
|
{ "rhinoceros", Emoji.Known.Rhinoceros },
|
|
{ "ribbon", Emoji.Known.Ribbon },
|
|
{ "rice_ball", Emoji.Known.RiceBall },
|
|
{ "rice_cracker", Emoji.Known.RiceCracker },
|
|
{ "right_anger_bubble", Emoji.Known.RightAngerBubble },
|
|
{ "right_arrow", Emoji.Known.RightArrow },
|
|
{ "right_arrow_curving_down", Emoji.Known.RightArrowCurvingDown },
|
|
{ "right_arrow_curving_left", Emoji.Known.RightArrowCurvingLeft },
|
|
{ "right_arrow_curving_up", Emoji.Known.RightArrowCurvingUp },
|
|
{ "right_facing_fist", Emoji.Known.RightFacingFist },
|
|
{ "ring", Emoji.Known.Ring },
|
|
{ "ringed_planet", Emoji.Known.RingedPlanet },
|
|
{ "roasted_sweet_potato", Emoji.Known.RoastedSweetPotato },
|
|
{ "robot", Emoji.Known.Robot },
|
|
{ "rock", Emoji.Known.Rock },
|
|
{ "rocket", Emoji.Known.Rocket },
|
|
{ "rolled_up_newspaper", Emoji.Known.RolledUpNewspaper },
|
|
{ "roller_coaster", Emoji.Known.RollerCoaster },
|
|
{ "roller_skate", Emoji.Known.RollerSkate },
|
|
{ "rolling_on_the_floor_laughing", Emoji.Known.RollingOnTheFloorLaughing },
|
|
{ "roll_of_paper", Emoji.Known.RollOfPaper },
|
|
{ "rooster", Emoji.Known.Rooster },
|
|
{ "rose", Emoji.Known.Rose },
|
|
{ "rosette", Emoji.Known.Rosette },
|
|
{ "round_pushpin", Emoji.Known.RoundPushpin },
|
|
{ "rugby_football", Emoji.Known.RugbyFootball },
|
|
{ "running_shirt", Emoji.Known.RunningShirt },
|
|
{ "running_shoe", Emoji.Known.RunningShoe },
|
|
{ "sad_but_relieved_face", Emoji.Known.SadButRelievedFace },
|
|
{ "safety_pin", Emoji.Known.SafetyPin },
|
|
{ "safety_vest", Emoji.Known.SafetyVest },
|
|
{ "sagittarius", Emoji.Known.Sagittarius },
|
|
{ "sailboat", Emoji.Known.Sailboat },
|
|
{ "sake", Emoji.Known.Sake },
|
|
{ "salt", Emoji.Known.Salt },
|
|
{ "sandwich", Emoji.Known.Sandwich },
|
|
{ "santa_claus", Emoji.Known.SantaClaus },
|
|
{ "sari", Emoji.Known.Sari },
|
|
{ "satellite", Emoji.Known.Satellite },
|
|
{ "satellite_antenna", Emoji.Known.SatelliteAntenna },
|
|
{ "sauropod", Emoji.Known.Sauropod },
|
|
{ "saxophone", Emoji.Known.Saxophone },
|
|
{ "scarf", Emoji.Known.Scarf },
|
|
{ "school", Emoji.Known.School },
|
|
{ "scissors", Emoji.Known.Scissors },
|
|
{ "scorpio", Emoji.Known.Scorpio },
|
|
{ "scorpion", Emoji.Known.Scorpion },
|
|
{ "screwdriver", Emoji.Known.Screwdriver },
|
|
{ "scroll", Emoji.Known.Scroll },
|
|
{ "seal", Emoji.Known.Seal },
|
|
{ "seat", Emoji.Known.Seat },
|
|
{ "2nd_place_medal", Emoji.Known.SecondPlaceMedal },
|
|
{ "seedling", Emoji.Known.Seedling },
|
|
{ "see_no_evil_monkey", Emoji.Known.SeeNoEvilMonkey },
|
|
{ "selfie", Emoji.Known.Selfie },
|
|
{ "seven_o_clock", Emoji.Known.SevenOClock },
|
|
{ "seven_thirty", Emoji.Known.SevenThirty },
|
|
{ "sewing_needle", Emoji.Known.SewingNeedle },
|
|
{ "shallow_pan_of_food", Emoji.Known.ShallowPanOfFood },
|
|
{ "shamrock", Emoji.Known.Shamrock },
|
|
{ "shark", Emoji.Known.Shark },
|
|
{ "shaved_ice", Emoji.Known.ShavedIce },
|
|
{ "sheaf_of_rice", Emoji.Known.SheafOfRice },
|
|
{ "shield", Emoji.Known.Shield },
|
|
{ "shinto_shrine", Emoji.Known.ShintoShrine },
|
|
{ "ship", Emoji.Known.Ship },
|
|
{ "shooting_star", Emoji.Known.ShootingStar },
|
|
{ "shopping_bags", Emoji.Known.ShoppingBags },
|
|
{ "shopping_cart", Emoji.Known.ShoppingCart },
|
|
{ "shortcake", Emoji.Known.Shortcake },
|
|
{ "shorts", Emoji.Known.Shorts },
|
|
{ "shower", Emoji.Known.Shower },
|
|
{ "shrimp", Emoji.Known.Shrimp },
|
|
{ "shuffle_tracks_button", Emoji.Known.ShuffleTracksButton },
|
|
{ "shushing_face", Emoji.Known.ShushingFace },
|
|
{ "sign_of_the_horns", Emoji.Known.SignOfTheHorns },
|
|
{ "six_o_clock", Emoji.Known.SixOClock },
|
|
{ "six_thirty", Emoji.Known.SixThirty },
|
|
{ "skateboard", Emoji.Known.Skateboard },
|
|
{ "skier", Emoji.Known.Skier },
|
|
{ "skis", Emoji.Known.Skis },
|
|
{ "skull", Emoji.Known.Skull },
|
|
{ "skull_and_crossbones", Emoji.Known.SkullAndCrossbones },
|
|
{ "skunk", Emoji.Known.Skunk },
|
|
{ "sled", Emoji.Known.Sled },
|
|
{ "sleeping_face", Emoji.Known.SleepingFace },
|
|
{ "sleepy_face", Emoji.Known.SleepyFace },
|
|
{ "slightly_frowning_face", Emoji.Known.SlightlyFrowningFace },
|
|
{ "slightly_smiling_face", Emoji.Known.SlightlySmilingFace },
|
|
{ "sloth", Emoji.Known.Sloth },
|
|
{ "slot_machine", Emoji.Known.SlotMachine },
|
|
{ "small_airplane", Emoji.Known.SmallAirplane },
|
|
{ "small_blue_diamond", Emoji.Known.SmallBlueDiamond },
|
|
{ "small_orange_diamond", Emoji.Known.SmallOrangeDiamond },
|
|
{ "smiling_cat_with_heart_eyes", Emoji.Known.SmilingCatWithHeartEyes },
|
|
{ "smiling_face", Emoji.Known.SmilingFace },
|
|
{ "smiling_face_with_halo", Emoji.Known.SmilingFaceWithHalo },
|
|
{ "smiling_face_with_heart_eyes", Emoji.Known.SmilingFaceWithHeartEyes },
|
|
{ "smiling_face_with_hearts", Emoji.Known.SmilingFaceWithHearts },
|
|
{ "smiling_face_with_horns", Emoji.Known.SmilingFaceWithHorns },
|
|
{ "smiling_face_with_smiling_eyes", Emoji.Known.SmilingFaceWithSmilingEyes },
|
|
{ "smiling_face_with_sunglasses", Emoji.Known.SmilingFaceWithSunglasses },
|
|
{ "smiling_face_with_tear", Emoji.Known.SmilingFaceWithTear },
|
|
{ "smirking_face", Emoji.Known.SmirkingFace },
|
|
{ "snail", Emoji.Known.Snail },
|
|
{ "snake", Emoji.Known.Snake },
|
|
{ "sneezing_face", Emoji.Known.SneezingFace },
|
|
{ "snowboarder", Emoji.Known.Snowboarder },
|
|
{ "snow_capped_mountain", Emoji.Known.SnowCappedMountain },
|
|
{ "snowflake", Emoji.Known.Snowflake },
|
|
{ "snowman", Emoji.Known.Snowman },
|
|
{ "snowman_without_snow", Emoji.Known.SnowmanWithoutSnow },
|
|
{ "soap", Emoji.Known.Soap },
|
|
{ "soccer_ball", Emoji.Known.SoccerBall },
|
|
{ "socks", Emoji.Known.Socks },
|
|
{ "softball", Emoji.Known.Softball },
|
|
{ "soft_ice_cream", Emoji.Known.SoftIceCream },
|
|
{ "soon_arrow", Emoji.Known.SoonArrow },
|
|
{ "sos_button", Emoji.Known.SosButton },
|
|
{ "spade_suit", Emoji.Known.SpadeSuit },
|
|
{ "spaghetti", Emoji.Known.Spaghetti },
|
|
{ "sparkle", Emoji.Known.Sparkle },
|
|
{ "sparkler", Emoji.Known.Sparkler },
|
|
{ "sparkles", Emoji.Known.Sparkles },
|
|
{ "sparkling_heart", Emoji.Known.SparklingHeart },
|
|
{ "speaker_high_volume", Emoji.Known.SpeakerHighVolume },
|
|
{ "speaker_low_volume", Emoji.Known.SpeakerLowVolume },
|
|
{ "speaker_medium_volume", Emoji.Known.SpeakerMediumVolume },
|
|
{ "speaking_head", Emoji.Known.SpeakingHead },
|
|
{ "speak_no_evil_monkey", Emoji.Known.SpeakNoEvilMonkey },
|
|
{ "speech_balloon", Emoji.Known.SpeechBalloon },
|
|
{ "speedboat", Emoji.Known.Speedboat },
|
|
{ "spider", Emoji.Known.Spider },
|
|
{ "spider_web", Emoji.Known.SpiderWeb },
|
|
{ "spiral_calendar", Emoji.Known.SpiralCalendar },
|
|
{ "spiral_notepad", Emoji.Known.SpiralNotepad },
|
|
{ "spiral_shell", Emoji.Known.SpiralShell },
|
|
{ "sponge", Emoji.Known.Sponge },
|
|
{ "spoon", Emoji.Known.Spoon },
|
|
{ "sports_medal", Emoji.Known.SportsMedal },
|
|
{ "sport_utility_vehicle", Emoji.Known.SportUtilityVehicle },
|
|
{ "spouting_whale", Emoji.Known.SpoutingWhale },
|
|
{ "squid", Emoji.Known.Squid },
|
|
{ "squinting_face_with_tongue", Emoji.Known.SquintingFaceWithTongue },
|
|
{ "stadium", Emoji.Known.Stadium },
|
|
{ "star", Emoji.Known.Star },
|
|
{ "star_and_crescent", Emoji.Known.StarAndCrescent },
|
|
{ "star_of_david", Emoji.Known.StarOfDavid },
|
|
{ "star_struck", Emoji.Known.StarStruck },
|
|
{ "station", Emoji.Known.Station },
|
|
{ "statue_of_liberty", Emoji.Known.StatueOfLiberty },
|
|
{ "steaming_bowl", Emoji.Known.SteamingBowl },
|
|
{ "stethoscope", Emoji.Known.Stethoscope },
|
|
{ "stop_button", Emoji.Known.StopButton },
|
|
{ "stop_sign", Emoji.Known.StopSign },
|
|
{ "stopwatch", Emoji.Known.Stopwatch },
|
|
{ "straight_ruler", Emoji.Known.StraightRuler },
|
|
{ "strawberry", Emoji.Known.Strawberry },
|
|
{ "studio_microphone", Emoji.Known.StudioMicrophone },
|
|
{ "stuffed_flatbread", Emoji.Known.StuffedFlatbread },
|
|
{ "sun", Emoji.Known.Sun },
|
|
{ "sun_behind_cloud", Emoji.Known.SunBehindCloud },
|
|
{ "sun_behind_large_cloud", Emoji.Known.SunBehindLargeCloud },
|
|
{ "sun_behind_rain_cloud", Emoji.Known.SunBehindRainCloud },
|
|
{ "sun_behind_small_cloud", Emoji.Known.SunBehindSmallCloud },
|
|
{ "sunflower", Emoji.Known.Sunflower },
|
|
{ "sunglasses", Emoji.Known.Sunglasses },
|
|
{ "sunrise", Emoji.Known.Sunrise },
|
|
{ "sunrise_over_mountains", Emoji.Known.SunriseOverMountains },
|
|
{ "sunset", Emoji.Known.Sunset },
|
|
{ "sun_with_face", Emoji.Known.SunWithFace },
|
|
{ "superhero", Emoji.Known.Superhero },
|
|
{ "supervillain", Emoji.Known.Supervillain },
|
|
{ "sushi", Emoji.Known.Sushi },
|
|
{ "suspension_railway", Emoji.Known.SuspensionRailway },
|
|
{ "swan", Emoji.Known.Swan },
|
|
{ "sweat_droplets", Emoji.Known.SweatDroplets },
|
|
{ "synagogue", Emoji.Known.Synagogue },
|
|
{ "syringe", Emoji.Known.Syringe },
|
|
{ "taco", Emoji.Known.Taco },
|
|
{ "takeout_box", Emoji.Known.TakeoutBox },
|
|
{ "tamale", Emoji.Known.Tamale },
|
|
{ "tanabata_tree", Emoji.Known.TanabataTree },
|
|
{ "tangerine", Emoji.Known.Tangerine },
|
|
{ "taurus", Emoji.Known.Taurus },
|
|
{ "taxi", Emoji.Known.Taxi },
|
|
{ "teacup_without_handle", Emoji.Known.TeacupWithoutHandle },
|
|
{ "teapot", Emoji.Known.Teapot },
|
|
{ "tear_off_calendar", Emoji.Known.TearOffCalendar },
|
|
{ "teddy_bear", Emoji.Known.TeddyBear },
|
|
{ "telephone", Emoji.Known.Telephone },
|
|
{ "telephone_receiver", Emoji.Known.TelephoneReceiver },
|
|
{ "telescope", Emoji.Known.Telescope },
|
|
{ "television", Emoji.Known.Television },
|
|
{ "tennis", Emoji.Known.Tennis },
|
|
{ "ten_o_clock", Emoji.Known.TenOClock },
|
|
{ "tent", Emoji.Known.Tent },
|
|
{ "ten_thirty", Emoji.Known.TenThirty },
|
|
{ "test_tube", Emoji.Known.TestTube },
|
|
{ "thermometer", Emoji.Known.Thermometer },
|
|
{ "thinking_face", Emoji.Known.ThinkingFace },
|
|
{ "3rd_place_medal", Emoji.Known.ThirdPlaceMedal },
|
|
{ "thong_sandal", Emoji.Known.ThongSandal },
|
|
{ "thought_balloon", Emoji.Known.ThoughtBalloon },
|
|
{ "thread", Emoji.Known.Thread },
|
|
{ "three_o_clock", Emoji.Known.ThreeOClock },
|
|
{ "three_thirty", Emoji.Known.ThreeThirty },
|
|
{ "thumbs_down", Emoji.Known.ThumbsDown },
|
|
{ "thumbs_up", Emoji.Known.ThumbsUp },
|
|
{ "ticket", Emoji.Known.Ticket },
|
|
{ "tiger", Emoji.Known.Tiger },
|
|
{ "tiger_face", Emoji.Known.TigerFace },
|
|
{ "timer_clock", Emoji.Known.TimerClock },
|
|
{ "tired_face", Emoji.Known.TiredFace },
|
|
{ "toilet", Emoji.Known.Toilet },
|
|
{ "tokyo_tower", Emoji.Known.TokyoTower },
|
|
{ "tomato", Emoji.Known.Tomato },
|
|
{ "tongue", Emoji.Known.Tongue },
|
|
{ "toolbox", Emoji.Known.Toolbox },
|
|
{ "tooth", Emoji.Known.Tooth },
|
|
{ "toothbrush", Emoji.Known.Toothbrush },
|
|
{ "top_arrow", Emoji.Known.TopArrow },
|
|
{ "top_hat", Emoji.Known.TopHat },
|
|
{ "tornado", Emoji.Known.Tornado },
|
|
{ "trackball", Emoji.Known.Trackball },
|
|
{ "tractor", Emoji.Known.Tractor },
|
|
{ "trade_mark", Emoji.Known.TradeMark },
|
|
{ "train", Emoji.Known.Train },
|
|
{ "tram", Emoji.Known.Tram },
|
|
{ "tram_car", Emoji.Known.TramCar },
|
|
{ "transgender_symbol", Emoji.Known.TransgenderSymbol },
|
|
{ "t_rex", Emoji.Known.TRex },
|
|
{ "triangular_flag", Emoji.Known.TriangularFlag },
|
|
{ "triangular_ruler", Emoji.Known.TriangularRuler },
|
|
{ "trident_emblem", Emoji.Known.TridentEmblem },
|
|
{ "trolleybus", Emoji.Known.Trolleybus },
|
|
{ "trophy", Emoji.Known.Trophy },
|
|
{ "tropical_drink", Emoji.Known.TropicalDrink },
|
|
{ "tropical_fish", Emoji.Known.TropicalFish },
|
|
{ "trumpet", Emoji.Known.Trumpet },
|
|
{ "t_shirt", Emoji.Known.TShirt },
|
|
{ "tulip", Emoji.Known.Tulip },
|
|
{ "tumbler_glass", Emoji.Known.TumblerGlass },
|
|
{ "turkey", Emoji.Known.Turkey },
|
|
{ "turtle", Emoji.Known.Turtle },
|
|
{ "twelve_o_clock", Emoji.Known.TwelveOClock },
|
|
{ "twelve_thirty", Emoji.Known.TwelveThirty },
|
|
{ "two_hearts", Emoji.Known.TwoHearts },
|
|
{ "two_hump_camel", Emoji.Known.TwoHumpCamel },
|
|
{ "two_o_clock", Emoji.Known.TwoOClock },
|
|
{ "two_thirty", Emoji.Known.TwoThirty },
|
|
{ "umbrella", Emoji.Known.Umbrella },
|
|
{ "umbrella_on_ground", Emoji.Known.UmbrellaOnGround },
|
|
{ "umbrella_with_rain_drops", Emoji.Known.UmbrellaWithRainDrops },
|
|
{ "unamused_face", Emoji.Known.UnamusedFace },
|
|
{ "unicorn", Emoji.Known.Unicorn },
|
|
{ "unlocked", Emoji.Known.Unlocked },
|
|
{ "up_arrow", Emoji.Known.UpArrow },
|
|
{ "up_button", Emoji.Known.UpButton },
|
|
{ "up_down_arrow", Emoji.Known.UpDownArrow },
|
|
{ "up_left_arrow", Emoji.Known.UpLeftArrow },
|
|
{ "up_right_arrow", Emoji.Known.UpRightArrow },
|
|
{ "upside_down_face", Emoji.Known.UpsideDownFace },
|
|
{ "upwards_button", Emoji.Known.UpwardsButton },
|
|
{ "vampire", Emoji.Known.Vampire },
|
|
{ "vertical_traffic_light", Emoji.Known.VerticalTrafficLight },
|
|
{ "vibration_mode", Emoji.Known.VibrationMode },
|
|
{ "victory_hand", Emoji.Known.VictoryHand },
|
|
{ "video_camera", Emoji.Known.VideoCamera },
|
|
{ "videocassette", Emoji.Known.Videocassette },
|
|
{ "video_game", Emoji.Known.VideoGame },
|
|
{ "violin", Emoji.Known.Violin },
|
|
{ "virgo", Emoji.Known.Virgo },
|
|
{ "volcano", Emoji.Known.Volcano },
|
|
{ "volleyball", Emoji.Known.Volleyball },
|
|
{ "vs_button", Emoji.Known.VsButton },
|
|
{ "vulcan_salute", Emoji.Known.VulcanSalute },
|
|
{ "waffle", Emoji.Known.Waffle },
|
|
{ "waning_crescent_moon", Emoji.Known.WaningCrescentMoon },
|
|
{ "waning_gibbous_moon", Emoji.Known.WaningGibbousMoon },
|
|
{ "warning", Emoji.Known.Warning },
|
|
{ "wastebasket", Emoji.Known.Wastebasket },
|
|
{ "watch", Emoji.Known.Watch },
|
|
{ "water_buffalo", Emoji.Known.WaterBuffalo },
|
|
{ "water_closet", Emoji.Known.WaterCloset },
|
|
{ "watermelon", Emoji.Known.Watermelon },
|
|
{ "water_pistol", Emoji.Known.WaterPistol },
|
|
{ "water_wave", Emoji.Known.WaterWave },
|
|
{ "waving_hand", Emoji.Known.WavingHand },
|
|
{ "wavy_dash", Emoji.Known.WavyDash },
|
|
{ "waxing_crescent_moon", Emoji.Known.WaxingCrescentMoon },
|
|
{ "waxing_gibbous_moon", Emoji.Known.WaxingGibbousMoon },
|
|
{ "weary_cat", Emoji.Known.WearyCat },
|
|
{ "weary_face", Emoji.Known.WearyFace },
|
|
{ "wedding", Emoji.Known.Wedding },
|
|
{ "whale", Emoji.Known.Whale },
|
|
{ "wheelchair_symbol", Emoji.Known.WheelchairSymbol },
|
|
{ "wheel_of_dharma", Emoji.Known.WheelOfDharma },
|
|
{ "white_cane", Emoji.Known.WhiteCane },
|
|
{ "white_circle", Emoji.Known.WhiteCircle },
|
|
{ "white_exclamation_mark", Emoji.Known.WhiteExclamationMark },
|
|
{ "white_flag", Emoji.Known.WhiteFlag },
|
|
{ "white_flower", Emoji.Known.WhiteFlower },
|
|
{ "white_hair", Emoji.Known.WhiteHair },
|
|
{ "white_heart", Emoji.Known.WhiteHeart },
|
|
{ "white_large_square", Emoji.Known.WhiteLargeSquare },
|
|
{ "white_medium_small_square", Emoji.Known.WhiteMediumSmallSquare },
|
|
{ "white_medium_square", Emoji.Known.WhiteMediumSquare },
|
|
{ "white_question_mark", Emoji.Known.WhiteQuestionMark },
|
|
{ "white_small_square", Emoji.Known.WhiteSmallSquare },
|
|
{ "white_square_button", Emoji.Known.WhiteSquareButton },
|
|
{ "wilted_flower", Emoji.Known.WiltedFlower },
|
|
{ "wind_chime", Emoji.Known.WindChime },
|
|
{ "wind_face", Emoji.Known.WindFace },
|
|
{ "window", Emoji.Known.Window },
|
|
{ "wine_glass", Emoji.Known.WineGlass },
|
|
{ "winking_face", Emoji.Known.WinkingFace },
|
|
{ "winking_face_with_tongue", Emoji.Known.WinkingFaceWithTongue },
|
|
{ "wolf", Emoji.Known.Wolf },
|
|
{ "woman", Emoji.Known.Woman },
|
|
{ "woman_and_man_holding_hands", Emoji.Known.WomanAndManHoldingHands },
|
|
{ "woman_dancing", Emoji.Known.WomanDancing },
|
|
{ "womans_boot", Emoji.Known.WomansBoot },
|
|
{ "womans_clothes", Emoji.Known.WomansClothes },
|
|
{ "womans_hat", Emoji.Known.WomansHat },
|
|
{ "womans_sandal", Emoji.Known.WomansSandal },
|
|
{ "woman_with_headscarf", Emoji.Known.WomanWithHeadscarf },
|
|
{ "women_holding_hands", Emoji.Known.WomenHoldingHands },
|
|
{ "womens_room", Emoji.Known.WomensRoom },
|
|
{ "wood", Emoji.Known.Wood },
|
|
{ "woozy_face", Emoji.Known.WoozyFace },
|
|
{ "world_map", Emoji.Known.WorldMap },
|
|
{ "worm", Emoji.Known.Worm },
|
|
{ "worried_face", Emoji.Known.WorriedFace },
|
|
{ "wrapped_gift", Emoji.Known.WrappedGift },
|
|
{ "wrench", Emoji.Known.Wrench },
|
|
{ "writing_hand", Emoji.Known.WritingHand },
|
|
{ "yarn", Emoji.Known.Yarn },
|
|
{ "yawning_face", Emoji.Known.YawningFace },
|
|
{ "yellow_circle", Emoji.Known.YellowCircle },
|
|
{ "yellow_heart", Emoji.Known.YellowHeart },
|
|
{ "yellow_square", Emoji.Known.YellowSquare },
|
|
{ "yen_banknote", Emoji.Known.YenBanknote },
|
|
{ "yin_yang", Emoji.Known.YinYang },
|
|
{ "yo_yo", Emoji.Known.YoYo },
|
|
{ "zany_face", Emoji.Known.ZanyFace },
|
|
{ "zebra", Emoji.Known.Zebra },
|
|
{ "zipper_mouth_face", Emoji.Known.ZipperMouthFace },
|
|
{ "zombie", Emoji.Known.Zombie },
|
|
{ "zzz", Emoji.Known.Zzz },
|
|
};
|
|
|
|
/// <summary>
|
|
/// Contains all predefined emojis.
|
|
/// </summary>
|
|
public static class Known
|
|
{
|
|
/// <summary>
|
|
/// Gets the "abacus" emoji.
|
|
/// Description: Abacus.
|
|
/// </summary>
|
|
public const string Abacus = "\U0001F9EE";
|
|
|
|
/// <summary>
|
|
/// Gets the "ab_button_blood_type" emoji.
|
|
/// Description: AB button blood type.
|
|
/// </summary>
|
|
public const string AbButtonBloodType = "\U0001F18E";
|
|
|
|
/// <summary>
|
|
/// Gets the "a_button_blood_type" emoji.
|
|
/// Description: A button blood type.
|
|
/// </summary>
|
|
public const string AButtonBloodType = "\U0001F170";
|
|
|
|
/// <summary>
|
|
/// Gets the "accordion" emoji.
|
|
/// Description: Accordion.
|
|
/// </summary>
|
|
public const string Accordion = "\U0001FA97";
|
|
|
|
/// <summary>
|
|
/// Gets the "adhesive_bandage" emoji.
|
|
/// Description: Adhesive bandage.
|
|
/// </summary>
|
|
public const string AdhesiveBandage = "\U0001FA79";
|
|
|
|
/// <summary>
|
|
/// Gets the "admission_tickets" emoji.
|
|
/// Description: Admission tickets.
|
|
/// </summary>
|
|
public const string AdmissionTickets = "\U0001F39F";
|
|
|
|
/// <summary>
|
|
/// Gets the "aerial_tramway" emoji.
|
|
/// Description: Aerial tramway.
|
|
/// </summary>
|
|
public const string AerialTramway = "\U0001F6A1";
|
|
|
|
/// <summary>
|
|
/// Gets the "airplane" emoji.
|
|
/// Description: Airplane.
|
|
/// </summary>
|
|
public const string Airplane = "\U00002708";
|
|
|
|
/// <summary>
|
|
/// Gets the "airplane_arrival" emoji.
|
|
/// Description: Airplane arrival.
|
|
/// </summary>
|
|
public const string AirplaneArrival = "\U0001F6EC";
|
|
|
|
/// <summary>
|
|
/// Gets the "airplane_departure" emoji.
|
|
/// Description: Airplane departure.
|
|
/// </summary>
|
|
public const string AirplaneDeparture = "\U0001F6EB";
|
|
|
|
/// <summary>
|
|
/// Gets the "alarm_clock" emoji.
|
|
/// Description: Alarm clock.
|
|
/// </summary>
|
|
public const string AlarmClock = "\U000023F0";
|
|
|
|
/// <summary>
|
|
/// Gets the "alembic" emoji.
|
|
/// Description: Alembic.
|
|
/// </summary>
|
|
public const string Alembic = "\U00002697";
|
|
|
|
/// <summary>
|
|
/// Gets the "alien" emoji.
|
|
/// Description: Alien.
|
|
/// </summary>
|
|
public const string Alien = "\U0001F47D";
|
|
|
|
/// <summary>
|
|
/// Gets the "alien_monster" emoji.
|
|
/// Description: Alien monster.
|
|
/// </summary>
|
|
public const string AlienMonster = "\U0001F47E";
|
|
|
|
/// <summary>
|
|
/// Gets the "ambulance" emoji.
|
|
/// Description: Ambulance.
|
|
/// </summary>
|
|
public const string Ambulance = "\U0001F691";
|
|
|
|
/// <summary>
|
|
/// Gets the "american_football" emoji.
|
|
/// Description: American football.
|
|
/// </summary>
|
|
public const string AmericanFootball = "\U0001F3C8";
|
|
|
|
/// <summary>
|
|
/// Gets the "amphora" emoji.
|
|
/// Description: Amphora.
|
|
/// </summary>
|
|
public const string Amphora = "\U0001F3FA";
|
|
|
|
/// <summary>
|
|
/// Gets the "anatomical_heart" emoji.
|
|
/// Description: Anatomical heart.
|
|
/// </summary>
|
|
public const string AnatomicalHeart = "\U0001FAC0";
|
|
|
|
/// <summary>
|
|
/// Gets the "anchor" emoji.
|
|
/// Description: Anchor.
|
|
/// </summary>
|
|
public const string Anchor = "\U00002693";
|
|
|
|
/// <summary>
|
|
/// Gets the "anger_symbol" emoji.
|
|
/// Description: Anger symbol.
|
|
/// </summary>
|
|
public const string AngerSymbol = "\U0001F4A2";
|
|
|
|
/// <summary>
|
|
/// Gets the "angry_face" emoji.
|
|
/// Description: Angry face.
|
|
/// </summary>
|
|
public const string AngryFace = "\U0001F620";
|
|
|
|
/// <summary>
|
|
/// Gets the "angry_face_with_horns" emoji.
|
|
/// Description: Angry face with horns.
|
|
/// </summary>
|
|
public const string AngryFaceWithHorns = "\U0001F47F";
|
|
|
|
/// <summary>
|
|
/// Gets the "anguished_face" emoji.
|
|
/// Description: Anguished face.
|
|
/// </summary>
|
|
public const string AnguishedFace = "\U0001F627";
|
|
|
|
/// <summary>
|
|
/// Gets the "ant" emoji.
|
|
/// Description: Ant.
|
|
/// </summary>
|
|
public const string Ant = "\U0001F41C";
|
|
|
|
/// <summary>
|
|
/// Gets the "antenna_bars" emoji.
|
|
/// Description: Antenna bars.
|
|
/// </summary>
|
|
public const string AntennaBars = "\U0001F4F6";
|
|
|
|
/// <summary>
|
|
/// Gets the "anxious_face_with_sweat" emoji.
|
|
/// Description: Anxious face with sweat.
|
|
/// </summary>
|
|
public const string AnxiousFaceWithSweat = "\U0001F630";
|
|
|
|
/// <summary>
|
|
/// Gets the "aquarius" emoji.
|
|
/// Description: Aquarius.
|
|
/// </summary>
|
|
public const string Aquarius = "\U00002652";
|
|
|
|
/// <summary>
|
|
/// Gets the "aries" emoji.
|
|
/// Description: Aries.
|
|
/// </summary>
|
|
public const string Aries = "\U00002648";
|
|
|
|
/// <summary>
|
|
/// Gets the "articulated_lorry" emoji.
|
|
/// Description: Articulated lorry.
|
|
/// </summary>
|
|
public const string ArticulatedLorry = "\U0001F69B";
|
|
|
|
/// <summary>
|
|
/// Gets the "artist_palette" emoji.
|
|
/// Description: Artist palette.
|
|
/// </summary>
|
|
public const string ArtistPalette = "\U0001F3A8";
|
|
|
|
/// <summary>
|
|
/// Gets the "astonished_face" emoji.
|
|
/// Description: Astonished face.
|
|
/// </summary>
|
|
public const string AstonishedFace = "\U0001F632";
|
|
|
|
/// <summary>
|
|
/// Gets the "atm_sign" emoji.
|
|
/// Description: ATM sign.
|
|
/// </summary>
|
|
public const string AtmSign = "\U0001F3E7";
|
|
|
|
/// <summary>
|
|
/// Gets the "atom_symbol" emoji.
|
|
/// Description: Atom symbol.
|
|
/// </summary>
|
|
public const string AtomSymbol = "\U0000269B";
|
|
|
|
/// <summary>
|
|
/// Gets the "automobile" emoji.
|
|
/// Description: Automobile.
|
|
/// </summary>
|
|
public const string Automobile = "\U0001F697";
|
|
|
|
/// <summary>
|
|
/// Gets the "auto_rickshaw" emoji.
|
|
/// Description: Auto rickshaw.
|
|
/// </summary>
|
|
public const string AutoRickshaw = "\U0001F6FA";
|
|
|
|
/// <summary>
|
|
/// Gets the "avocado" emoji.
|
|
/// Description: Avocado.
|
|
/// </summary>
|
|
public const string Avocado = "\U0001F951";
|
|
|
|
/// <summary>
|
|
/// Gets the "axe" emoji.
|
|
/// Description: Axe.
|
|
/// </summary>
|
|
public const string Axe = "\U0001FA93";
|
|
|
|
/// <summary>
|
|
/// Gets the "baby" emoji.
|
|
/// Description: Baby.
|
|
/// </summary>
|
|
public const string Baby = "\U0001F476";
|
|
|
|
/// <summary>
|
|
/// Gets the "baby_angel" emoji.
|
|
/// Description: Baby angel.
|
|
/// </summary>
|
|
public const string BabyAngel = "\U0001F47C";
|
|
|
|
/// <summary>
|
|
/// Gets the "baby_bottle" emoji.
|
|
/// Description: Baby bottle.
|
|
/// </summary>
|
|
public const string BabyBottle = "\U0001F37C";
|
|
|
|
/// <summary>
|
|
/// Gets the "baby_chick" emoji.
|
|
/// Description: Baby chick.
|
|
/// </summary>
|
|
public const string BabyChick = "\U0001F424";
|
|
|
|
/// <summary>
|
|
/// Gets the "baby_symbol" emoji.
|
|
/// Description: Baby symbol.
|
|
/// </summary>
|
|
public const string BabySymbol = "\U0001F6BC";
|
|
|
|
/// <summary>
|
|
/// Gets the "back_arrow" emoji.
|
|
/// Description: BACK arrow.
|
|
/// </summary>
|
|
public const string BackArrow = "\U0001F519";
|
|
|
|
/// <summary>
|
|
/// Gets the "backhand_index_pointing_down" emoji.
|
|
/// Description: Backhand index pointing down.
|
|
/// </summary>
|
|
public const string BackhandIndexPointingDown = "\U0001F447";
|
|
|
|
/// <summary>
|
|
/// Gets the "backhand_index_pointing_left" emoji.
|
|
/// Description: Backhand index pointing left.
|
|
/// </summary>
|
|
public const string BackhandIndexPointingLeft = "\U0001F448";
|
|
|
|
/// <summary>
|
|
/// Gets the "backhand_index_pointing_right" emoji.
|
|
/// Description: Backhand index pointing right.
|
|
/// </summary>
|
|
public const string BackhandIndexPointingRight = "\U0001F449";
|
|
|
|
/// <summary>
|
|
/// Gets the "backhand_index_pointing_up" emoji.
|
|
/// Description: Backhand index pointing up.
|
|
/// </summary>
|
|
public const string BackhandIndexPointingUp = "\U0001F446";
|
|
|
|
/// <summary>
|
|
/// Gets the "backpack" emoji.
|
|
/// Description: Backpack.
|
|
/// </summary>
|
|
public const string Backpack = "\U0001F392";
|
|
|
|
/// <summary>
|
|
/// Gets the "bacon" emoji.
|
|
/// Description: Bacon.
|
|
/// </summary>
|
|
public const string Bacon = "\U0001F953";
|
|
|
|
/// <summary>
|
|
/// Gets the "badger" emoji.
|
|
/// Description: Badger.
|
|
/// </summary>
|
|
public const string Badger = "\U0001F9A1";
|
|
|
|
/// <summary>
|
|
/// Gets the "badminton" emoji.
|
|
/// Description: Badminton.
|
|
/// </summary>
|
|
public const string Badminton = "\U0001F3F8";
|
|
|
|
/// <summary>
|
|
/// Gets the "bagel" emoji.
|
|
/// Description: Bagel.
|
|
/// </summary>
|
|
public const string Bagel = "\U0001F96F";
|
|
|
|
/// <summary>
|
|
/// Gets the "baggage_claim" emoji.
|
|
/// Description: Baggage claim.
|
|
/// </summary>
|
|
public const string BaggageClaim = "\U0001F6C4";
|
|
|
|
/// <summary>
|
|
/// Gets the "baguette_bread" emoji.
|
|
/// Description: Baguette bread.
|
|
/// </summary>
|
|
public const string BaguetteBread = "\U0001F956";
|
|
|
|
/// <summary>
|
|
/// Gets the "balance_scale" emoji.
|
|
/// Description: Balance scale.
|
|
/// </summary>
|
|
public const string BalanceScale = "\U00002696";
|
|
|
|
/// <summary>
|
|
/// Gets the "bald" emoji.
|
|
/// Description: Bald.
|
|
/// </summary>
|
|
public const string Bald = "\U0001F9B2";
|
|
|
|
/// <summary>
|
|
/// Gets the "ballet_shoes" emoji.
|
|
/// Description: Ballet shoes.
|
|
/// </summary>
|
|
public const string BalletShoes = "\U0001FA70";
|
|
|
|
/// <summary>
|
|
/// Gets the "balloon" emoji.
|
|
/// Description: Balloon.
|
|
/// </summary>
|
|
public const string Balloon = "\U0001F388";
|
|
|
|
/// <summary>
|
|
/// Gets the "ballot_box_with_ballot" emoji.
|
|
/// Description: Ballot box with ballot.
|
|
/// </summary>
|
|
public const string BallotBoxWithBallot = "\U0001F5F3";
|
|
|
|
/// <summary>
|
|
/// Gets the "banana" emoji.
|
|
/// Description: Banana.
|
|
/// </summary>
|
|
public const string Banana = "\U0001F34C";
|
|
|
|
/// <summary>
|
|
/// Gets the "banjo" emoji.
|
|
/// Description: Banjo.
|
|
/// </summary>
|
|
public const string Banjo = "\U0001FA95";
|
|
|
|
/// <summary>
|
|
/// Gets the "bank" emoji.
|
|
/// Description: Bank.
|
|
/// </summary>
|
|
public const string Bank = "\U0001F3E6";
|
|
|
|
/// <summary>
|
|
/// Gets the "barber_pole" emoji.
|
|
/// Description: Barber pole.
|
|
/// </summary>
|
|
public const string BarberPole = "\U0001F488";
|
|
|
|
/// <summary>
|
|
/// Gets the "bar_chart" emoji.
|
|
/// Description: Bar chart.
|
|
/// </summary>
|
|
public const string BarChart = "\U0001F4CA";
|
|
|
|
/// <summary>
|
|
/// Gets the "baseball" emoji.
|
|
/// Description: Baseball.
|
|
/// </summary>
|
|
public const string Baseball = "\U000026BE";
|
|
|
|
/// <summary>
|
|
/// Gets the "basket" emoji.
|
|
/// Description: Basket.
|
|
/// </summary>
|
|
public const string Basket = "\U0001F9FA";
|
|
|
|
/// <summary>
|
|
/// Gets the "basketball" emoji.
|
|
/// Description: Basketball.
|
|
/// </summary>
|
|
public const string Basketball = "\U0001F3C0";
|
|
|
|
/// <summary>
|
|
/// Gets the "bat" emoji.
|
|
/// Description: Bat.
|
|
/// </summary>
|
|
public const string Bat = "\U0001F987";
|
|
|
|
/// <summary>
|
|
/// Gets the "bathtub" emoji.
|
|
/// Description: Bathtub.
|
|
/// </summary>
|
|
public const string Bathtub = "\U0001F6C1";
|
|
|
|
/// <summary>
|
|
/// Gets the "battery" emoji.
|
|
/// Description: Battery.
|
|
/// </summary>
|
|
public const string Battery = "\U0001F50B";
|
|
|
|
/// <summary>
|
|
/// Gets the "b_button_blood_type" emoji.
|
|
/// Description: B button blood type.
|
|
/// </summary>
|
|
public const string BButtonBloodType = "\U0001F171";
|
|
|
|
/// <summary>
|
|
/// Gets the "beach_with_umbrella" emoji.
|
|
/// Description: Beach with umbrella.
|
|
/// </summary>
|
|
public const string BeachWithUmbrella = "\U0001F3D6";
|
|
|
|
/// <summary>
|
|
/// Gets the "beaming_face_with_smiling_eyes" emoji.
|
|
/// Description: Beaming face with smiling eyes.
|
|
/// </summary>
|
|
public const string BeamingFaceWithSmilingEyes = "\U0001F601";
|
|
|
|
/// <summary>
|
|
/// Gets the "bear" emoji.
|
|
/// Description: Bear.
|
|
/// </summary>
|
|
public const string Bear = "\U0001F43B";
|
|
|
|
/// <summary>
|
|
/// Gets the "beating_heart" emoji.
|
|
/// Description: Beating heart.
|
|
/// </summary>
|
|
public const string BeatingHeart = "\U0001F493";
|
|
|
|
/// <summary>
|
|
/// Gets the "beaver" emoji.
|
|
/// Description: Beaver.
|
|
/// </summary>
|
|
public const string Beaver = "\U0001F9AB";
|
|
|
|
/// <summary>
|
|
/// Gets the "bed" emoji.
|
|
/// Description: Bed.
|
|
/// </summary>
|
|
public const string Bed = "\U0001F6CF";
|
|
|
|
/// <summary>
|
|
/// Gets the "beer_mug" emoji.
|
|
/// Description: Beer mug.
|
|
/// </summary>
|
|
public const string BeerMug = "\U0001F37A";
|
|
|
|
/// <summary>
|
|
/// Gets the "beetle" emoji.
|
|
/// Description: Beetle.
|
|
/// </summary>
|
|
public const string Beetle = "\U0001FAB2";
|
|
|
|
/// <summary>
|
|
/// Gets the "bell" emoji.
|
|
/// Description: Bell.
|
|
/// </summary>
|
|
public const string Bell = "\U0001F514";
|
|
|
|
/// <summary>
|
|
/// Gets the "bellhop_bell" emoji.
|
|
/// Description: Bellhop bell.
|
|
/// </summary>
|
|
public const string BellhopBell = "\U0001F6CE";
|
|
|
|
/// <summary>
|
|
/// Gets the "bell_pepper" emoji.
|
|
/// Description: Bell pepper.
|
|
/// </summary>
|
|
public const string BellPepper = "\U0001FAD1";
|
|
|
|
/// <summary>
|
|
/// Gets the "bell_with_slash" emoji.
|
|
/// Description: Bell with slash.
|
|
/// </summary>
|
|
public const string BellWithSlash = "\U0001F515";
|
|
|
|
/// <summary>
|
|
/// Gets the "bento_box" emoji.
|
|
/// Description: Bento box.
|
|
/// </summary>
|
|
public const string BentoBox = "\U0001F371";
|
|
|
|
/// <summary>
|
|
/// Gets the "beverage_box" emoji.
|
|
/// Description: Beverage box.
|
|
/// </summary>
|
|
public const string BeverageBox = "\U0001F9C3";
|
|
|
|
/// <summary>
|
|
/// Gets the "bicycle" emoji.
|
|
/// Description: Bicycle.
|
|
/// </summary>
|
|
public const string Bicycle = "\U0001F6B2";
|
|
|
|
/// <summary>
|
|
/// Gets the "bikini" emoji.
|
|
/// Description: Bikini.
|
|
/// </summary>
|
|
public const string Bikini = "\U0001F459";
|
|
|
|
/// <summary>
|
|
/// Gets the "billed_cap" emoji.
|
|
/// Description: Billed cap.
|
|
/// </summary>
|
|
public const string BilledCap = "\U0001F9E2";
|
|
|
|
/// <summary>
|
|
/// Gets the "biohazard" emoji.
|
|
/// Description: Biohazard.
|
|
/// </summary>
|
|
public const string Biohazard = "\U00002623";
|
|
|
|
/// <summary>
|
|
/// Gets the "bird" emoji.
|
|
/// Description: Bird.
|
|
/// </summary>
|
|
public const string Bird = "\U0001F426";
|
|
|
|
/// <summary>
|
|
/// Gets the "birthday_cake" emoji.
|
|
/// Description: Birthday cake.
|
|
/// </summary>
|
|
public const string BirthdayCake = "\U0001F382";
|
|
|
|
/// <summary>
|
|
/// Gets the "bison" emoji.
|
|
/// Description: Bison.
|
|
/// </summary>
|
|
public const string Bison = "\U0001F9AC";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_circle" emoji.
|
|
/// Description: Black circle.
|
|
/// </summary>
|
|
public const string BlackCircle = "\U000026AB";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_flag" emoji.
|
|
/// Description: Black flag.
|
|
/// </summary>
|
|
public const string BlackFlag = "\U0001F3F4";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_heart" emoji.
|
|
/// Description: Black heart.
|
|
/// </summary>
|
|
public const string BlackHeart = "\U0001F5A4";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_large_square" emoji.
|
|
/// Description: Black large square.
|
|
/// </summary>
|
|
public const string BlackLargeSquare = "\U00002B1B";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_medium_small_square" emoji.
|
|
/// Description: black medium small square.
|
|
/// </summary>
|
|
public const string BlackMediumSmallSquare = "\U000025FE";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_medium_square" emoji.
|
|
/// Description: Black medium square.
|
|
/// </summary>
|
|
public const string BlackMediumSquare = "\U000025FC";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_nib" emoji.
|
|
/// Description: Black nib.
|
|
/// </summary>
|
|
public const string BlackNib = "\U00002712";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_small_square" emoji.
|
|
/// Description: Black small square.
|
|
/// </summary>
|
|
public const string BlackSmallSquare = "\U000025AA";
|
|
|
|
/// <summary>
|
|
/// Gets the "black_square_button" emoji.
|
|
/// Description: Black square button.
|
|
/// </summary>
|
|
public const string BlackSquareButton = "\U0001F532";
|
|
|
|
/// <summary>
|
|
/// Gets the "blossom" emoji.
|
|
/// Description: Blossom.
|
|
/// </summary>
|
|
public const string Blossom = "\U0001F33C";
|
|
|
|
/// <summary>
|
|
/// Gets the "blowfish" emoji.
|
|
/// Description: Blowfish.
|
|
/// </summary>
|
|
public const string Blowfish = "\U0001F421";
|
|
|
|
/// <summary>
|
|
/// Gets the "blueberries" emoji.
|
|
/// Description: Blueberries.
|
|
/// </summary>
|
|
public const string Blueberries = "\U0001FAD0";
|
|
|
|
/// <summary>
|
|
/// Gets the "blue_book" emoji.
|
|
/// Description: Blue book.
|
|
/// </summary>
|
|
public const string BlueBook = "\U0001F4D8";
|
|
|
|
/// <summary>
|
|
/// Gets the "blue_circle" emoji.
|
|
/// Description: Blue circle.
|
|
/// </summary>
|
|
public const string BlueCircle = "\U0001F535";
|
|
|
|
/// <summary>
|
|
/// Gets the "blue_heart" emoji.
|
|
/// Description: Blue heart.
|
|
/// </summary>
|
|
public const string BlueHeart = "\U0001F499";
|
|
|
|
/// <summary>
|
|
/// Gets the "blue_square" emoji.
|
|
/// Description: Blue square.
|
|
/// </summary>
|
|
public const string BlueSquare = "\U0001F7E6";
|
|
|
|
/// <summary>
|
|
/// Gets the "boar" emoji.
|
|
/// Description: Boar.
|
|
/// </summary>
|
|
public const string Boar = "\U0001F417";
|
|
|
|
/// <summary>
|
|
/// Gets the "bomb" emoji.
|
|
/// Description: Bomb.
|
|
/// </summary>
|
|
public const string Bomb = "\U0001F4A3";
|
|
|
|
/// <summary>
|
|
/// Gets the "bone" emoji.
|
|
/// Description: Bone.
|
|
/// </summary>
|
|
public const string Bone = "\U0001F9B4";
|
|
|
|
/// <summary>
|
|
/// Gets the "bookmark" emoji.
|
|
/// Description: Bookmark.
|
|
/// </summary>
|
|
public const string Bookmark = "\U0001F516";
|
|
|
|
/// <summary>
|
|
/// Gets the "bookmark_tabs" emoji.
|
|
/// Description: Bookmark tabs.
|
|
/// </summary>
|
|
public const string BookmarkTabs = "\U0001F4D1";
|
|
|
|
/// <summary>
|
|
/// Gets the "books" emoji.
|
|
/// Description: Books.
|
|
/// </summary>
|
|
public const string Books = "\U0001F4DA";
|
|
|
|
/// <summary>
|
|
/// Gets the "boomerang" emoji.
|
|
/// Description: Boomerang.
|
|
/// </summary>
|
|
public const string Boomerang = "\U0001FA83";
|
|
|
|
/// <summary>
|
|
/// Gets the "bottle_with_popping_cork" emoji.
|
|
/// Description: Bottle with popping cork.
|
|
/// </summary>
|
|
public const string BottleWithPoppingCork = "\U0001F37E";
|
|
|
|
/// <summary>
|
|
/// Gets the "bouquet" emoji.
|
|
/// Description: Bouquet.
|
|
/// </summary>
|
|
public const string Bouquet = "\U0001F490";
|
|
|
|
/// <summary>
|
|
/// Gets the "bow_and_arrow" emoji.
|
|
/// Description: Bow and arrow.
|
|
/// </summary>
|
|
public const string BowAndArrow = "\U0001F3F9";
|
|
|
|
/// <summary>
|
|
/// Gets the "bowling" emoji.
|
|
/// Description: Bowling.
|
|
/// </summary>
|
|
public const string Bowling = "\U0001F3B3";
|
|
|
|
/// <summary>
|
|
/// Gets the "bowl_with_spoon" emoji.
|
|
/// Description: Bowl with spoon.
|
|
/// </summary>
|
|
public const string BowlWithSpoon = "\U0001F963";
|
|
|
|
/// <summary>
|
|
/// Gets the "boxing_glove" emoji.
|
|
/// Description: Boxing glove.
|
|
/// </summary>
|
|
public const string BoxingGlove = "\U0001F94A";
|
|
|
|
/// <summary>
|
|
/// Gets the "boy" emoji.
|
|
/// Description: Boy.
|
|
/// </summary>
|
|
public const string Boy = "\U0001F466";
|
|
|
|
/// <summary>
|
|
/// Gets the "brain" emoji.
|
|
/// Description: Brain.
|
|
/// </summary>
|
|
public const string Brain = "\U0001F9E0";
|
|
|
|
/// <summary>
|
|
/// Gets the "bread" emoji.
|
|
/// Description: Bread.
|
|
/// </summary>
|
|
public const string Bread = "\U0001F35E";
|
|
|
|
/// <summary>
|
|
/// Gets the "breast_feeding" emoji.
|
|
/// Description: breast feeding.
|
|
/// </summary>
|
|
public const string BreastFeeding = "\U0001F931";
|
|
|
|
/// <summary>
|
|
/// Gets the "brick" emoji.
|
|
/// Description: Brick.
|
|
/// </summary>
|
|
public const string Brick = "\U0001F9F1";
|
|
|
|
/// <summary>
|
|
/// Gets the "bridge_at_night" emoji.
|
|
/// Description: Bridge at night.
|
|
/// </summary>
|
|
public const string BridgeAtNight = "\U0001F309";
|
|
|
|
/// <summary>
|
|
/// Gets the "briefcase" emoji.
|
|
/// Description: Briefcase.
|
|
/// </summary>
|
|
public const string Briefcase = "\U0001F4BC";
|
|
|
|
/// <summary>
|
|
/// Gets the "briefs" emoji.
|
|
/// Description: Briefs.
|
|
/// </summary>
|
|
public const string Briefs = "\U0001FA72";
|
|
|
|
/// <summary>
|
|
/// Gets the "bright_button" emoji.
|
|
/// Description: Bright button.
|
|
/// </summary>
|
|
public const string BrightButton = "\U0001F506";
|
|
|
|
/// <summary>
|
|
/// Gets the "broccoli" emoji.
|
|
/// Description: Broccoli.
|
|
/// </summary>
|
|
public const string Broccoli = "\U0001F966";
|
|
|
|
/// <summary>
|
|
/// Gets the "broken_heart" emoji.
|
|
/// Description: Broken heart.
|
|
/// </summary>
|
|
public const string BrokenHeart = "\U0001F494";
|
|
|
|
/// <summary>
|
|
/// Gets the "broom" emoji.
|
|
/// Description: Broom.
|
|
/// </summary>
|
|
public const string Broom = "\U0001F9F9";
|
|
|
|
/// <summary>
|
|
/// Gets the "brown_circle" emoji.
|
|
/// Description: Brown circle.
|
|
/// </summary>
|
|
public const string BrownCircle = "\U0001F7E4";
|
|
|
|
/// <summary>
|
|
/// Gets the "brown_heart" emoji.
|
|
/// Description: Brown heart.
|
|
/// </summary>
|
|
public const string BrownHeart = "\U0001F90E";
|
|
|
|
/// <summary>
|
|
/// Gets the "brown_square" emoji.
|
|
/// Description: Brown square.
|
|
/// </summary>
|
|
public const string BrownSquare = "\U0001F7EB";
|
|
|
|
/// <summary>
|
|
/// Gets the "bubble_tea" emoji.
|
|
/// Description: Bubble tea.
|
|
/// </summary>
|
|
public const string BubbleTea = "\U0001F9CB";
|
|
|
|
/// <summary>
|
|
/// Gets the "bucket" emoji.
|
|
/// Description: Bucket.
|
|
/// </summary>
|
|
public const string Bucket = "\U0001FAA3";
|
|
|
|
/// <summary>
|
|
/// Gets the "bug" emoji.
|
|
/// Description: Bug.
|
|
/// </summary>
|
|
public const string Bug = "\U0001F41B";
|
|
|
|
/// <summary>
|
|
/// Gets the "building_construction" emoji.
|
|
/// Description: Building construction.
|
|
/// </summary>
|
|
public const string BuildingConstruction = "\U0001F3D7";
|
|
|
|
/// <summary>
|
|
/// Gets the "bullet_train" emoji.
|
|
/// Description: Bullet train.
|
|
/// </summary>
|
|
public const string BulletTrain = "\U0001F685";
|
|
|
|
/// <summary>
|
|
/// Gets the "bullseye" emoji.
|
|
/// Description: Bullseye.
|
|
/// </summary>
|
|
public const string Bullseye = "\U0001F3AF";
|
|
|
|
/// <summary>
|
|
/// Gets the "burrito" emoji.
|
|
/// Description: Burrito.
|
|
/// </summary>
|
|
public const string Burrito = "\U0001F32F";
|
|
|
|
/// <summary>
|
|
/// Gets the "bus" emoji.
|
|
/// Description: Bus.
|
|
/// </summary>
|
|
public const string Bus = "\U0001F68C";
|
|
|
|
/// <summary>
|
|
/// Gets the "bus_stop" emoji.
|
|
/// Description: Bus stop.
|
|
/// </summary>
|
|
public const string BusStop = "\U0001F68F";
|
|
|
|
/// <summary>
|
|
/// Gets the "bust_in_silhouette" emoji.
|
|
/// Description: Bust in silhouette.
|
|
/// </summary>
|
|
public const string BustInSilhouette = "\U0001F464";
|
|
|
|
/// <summary>
|
|
/// Gets the "busts_in_silhouette" emoji.
|
|
/// Description: Busts in silhouette.
|
|
/// </summary>
|
|
public const string BustsInSilhouette = "\U0001F465";
|
|
|
|
/// <summary>
|
|
/// Gets the "butter" emoji.
|
|
/// Description: Butter.
|
|
/// </summary>
|
|
public const string Butter = "\U0001F9C8";
|
|
|
|
/// <summary>
|
|
/// Gets the "butterfly" emoji.
|
|
/// Description: Butterfly.
|
|
/// </summary>
|
|
public const string Butterfly = "\U0001F98B";
|
|
|
|
/// <summary>
|
|
/// Gets the "cactus" emoji.
|
|
/// Description: Cactus.
|
|
/// </summary>
|
|
public const string Cactus = "\U0001F335";
|
|
|
|
/// <summary>
|
|
/// Gets the "calendar" emoji.
|
|
/// Description: Calendar.
|
|
/// </summary>
|
|
public const string Calendar = "\U0001F4C5";
|
|
|
|
/// <summary>
|
|
/// Gets the "call_me_hand" emoji.
|
|
/// Description: Call me hand.
|
|
/// </summary>
|
|
public const string CallMeHand = "\U0001F919";
|
|
|
|
/// <summary>
|
|
/// Gets the "camel" emoji.
|
|
/// Description: Camel.
|
|
/// </summary>
|
|
public const string Camel = "\U0001F42A";
|
|
|
|
/// <summary>
|
|
/// Gets the "camera" emoji.
|
|
/// Description: Camera.
|
|
/// </summary>
|
|
public const string Camera = "\U0001F4F7";
|
|
|
|
/// <summary>
|
|
/// Gets the "camera_with_flash" emoji.
|
|
/// Description: Camera with flash.
|
|
/// </summary>
|
|
public const string CameraWithFlash = "\U0001F4F8";
|
|
|
|
/// <summary>
|
|
/// Gets the "camping" emoji.
|
|
/// Description: Camping.
|
|
/// </summary>
|
|
public const string Camping = "\U0001F3D5";
|
|
|
|
/// <summary>
|
|
/// Gets the "cancer" emoji.
|
|
/// Description: Cancer.
|
|
/// </summary>
|
|
public const string Cancer = "\U0000264B";
|
|
|
|
/// <summary>
|
|
/// Gets the "candle" emoji.
|
|
/// Description: Candle.
|
|
/// </summary>
|
|
public const string Candle = "\U0001F56F";
|
|
|
|
/// <summary>
|
|
/// Gets the "candy" emoji.
|
|
/// Description: Candy.
|
|
/// </summary>
|
|
public const string Candy = "\U0001F36C";
|
|
|
|
/// <summary>
|
|
/// Gets the "canned_food" emoji.
|
|
/// Description: Canned food.
|
|
/// </summary>
|
|
public const string CannedFood = "\U0001F96B";
|
|
|
|
/// <summary>
|
|
/// Gets the "canoe" emoji.
|
|
/// Description: Canoe.
|
|
/// </summary>
|
|
public const string Canoe = "\U0001F6F6";
|
|
|
|
/// <summary>
|
|
/// Gets the "capricorn" emoji.
|
|
/// Description: Capricorn.
|
|
/// </summary>
|
|
public const string Capricorn = "\U00002651";
|
|
|
|
/// <summary>
|
|
/// Gets the "card_file_box" emoji.
|
|
/// Description: Card file box.
|
|
/// </summary>
|
|
public const string CardFileBox = "\U0001F5C3";
|
|
|
|
/// <summary>
|
|
/// Gets the "card_index" emoji.
|
|
/// Description: Card index.
|
|
/// </summary>
|
|
public const string CardIndex = "\U0001F4C7";
|
|
|
|
/// <summary>
|
|
/// Gets the "card_index_dividers" emoji.
|
|
/// Description: Card index dividers.
|
|
/// </summary>
|
|
public const string CardIndexDividers = "\U0001F5C2";
|
|
|
|
/// <summary>
|
|
/// Gets the "carousel_horse" emoji.
|
|
/// Description: Carousel horse.
|
|
/// </summary>
|
|
public const string CarouselHorse = "\U0001F3A0";
|
|
|
|
/// <summary>
|
|
/// Gets the "carpentry_saw" emoji.
|
|
/// Description: Carpentry saw.
|
|
/// </summary>
|
|
public const string CarpentrySaw = "\U0001FA9A";
|
|
|
|
/// <summary>
|
|
/// Gets the "carp_streamer" emoji.
|
|
/// Description: Carp streamer.
|
|
/// </summary>
|
|
public const string CarpStreamer = "\U0001F38F";
|
|
|
|
/// <summary>
|
|
/// Gets the "carrot" emoji.
|
|
/// Description: Carrot.
|
|
/// </summary>
|
|
public const string Carrot = "\U0001F955";
|
|
|
|
/// <summary>
|
|
/// Gets the "castle" emoji.
|
|
/// Description: Castle.
|
|
/// </summary>
|
|
public const string Castle = "\U0001F3F0";
|
|
|
|
/// <summary>
|
|
/// Gets the "cat" emoji.
|
|
/// Description: Cat.
|
|
/// </summary>
|
|
public const string Cat = "\U0001F408";
|
|
|
|
/// <summary>
|
|
/// Gets the "cat_face" emoji.
|
|
/// Description: Cat face.
|
|
/// </summary>
|
|
public const string CatFace = "\U0001F431";
|
|
|
|
/// <summary>
|
|
/// Gets the "cat_with_tears_of_joy" emoji.
|
|
/// Description: Cat with tears of joy.
|
|
/// </summary>
|
|
public const string CatWithTearsOfJoy = "\U0001F639";
|
|
|
|
/// <summary>
|
|
/// Gets the "cat_with_wry_smile" emoji.
|
|
/// Description: Cat with wry smile.
|
|
/// </summary>
|
|
public const string CatWithWrySmile = "\U0001F63C";
|
|
|
|
/// <summary>
|
|
/// Gets the "chains" emoji.
|
|
/// Description: Chains.
|
|
/// </summary>
|
|
public const string Chains = "\U000026D3";
|
|
|
|
/// <summary>
|
|
/// Gets the "chair" emoji.
|
|
/// Description: Chair.
|
|
/// </summary>
|
|
public const string Chair = "\U0001FA91";
|
|
|
|
/// <summary>
|
|
/// Gets the "chart_decreasing" emoji.
|
|
/// Description: Chart decreasing.
|
|
/// </summary>
|
|
public const string ChartDecreasing = "\U0001F4C9";
|
|
|
|
/// <summary>
|
|
/// Gets the "chart_increasing" emoji.
|
|
/// Description: Chart increasing.
|
|
/// </summary>
|
|
public const string ChartIncreasing = "\U0001F4C8";
|
|
|
|
/// <summary>
|
|
/// Gets the "chart_increasing_with_yen" emoji.
|
|
/// Description: Chart increasing with yen.
|
|
/// </summary>
|
|
public const string ChartIncreasingWithYen = "\U0001F4B9";
|
|
|
|
/// <summary>
|
|
/// Gets the "check_box_with_check" emoji.
|
|
/// Description: Check box with check.
|
|
/// </summary>
|
|
public const string CheckBoxWithCheck = "\U00002611";
|
|
|
|
/// <summary>
|
|
/// Gets the "check_mark" emoji.
|
|
/// Description: Check mark.
|
|
/// </summary>
|
|
public const string CheckMark = "\U00002714";
|
|
|
|
/// <summary>
|
|
/// Gets the "check_mark_button" emoji.
|
|
/// Description: Check mark button.
|
|
/// </summary>
|
|
public const string CheckMarkButton = "\U00002705";
|
|
|
|
/// <summary>
|
|
/// Gets the "cheese_wedge" emoji.
|
|
/// Description: Cheese wedge.
|
|
/// </summary>
|
|
public const string CheeseWedge = "\U0001F9C0";
|
|
|
|
/// <summary>
|
|
/// Gets the "chequered_flag" emoji.
|
|
/// Description: Chequered flag.
|
|
/// </summary>
|
|
public const string ChequeredFlag = "\U0001F3C1";
|
|
|
|
/// <summary>
|
|
/// Gets the "cherries" emoji.
|
|
/// Description: Cherries.
|
|
/// </summary>
|
|
public const string Cherries = "\U0001F352";
|
|
|
|
/// <summary>
|
|
/// Gets the "cherry_blossom" emoji.
|
|
/// Description: Cherry blossom.
|
|
/// </summary>
|
|
public const string CherryBlossom = "\U0001F338";
|
|
|
|
/// <summary>
|
|
/// Gets the "chess_pawn" emoji.
|
|
/// Description: Chess pawn.
|
|
/// </summary>
|
|
public const string ChessPawn = "\U0000265F";
|
|
|
|
/// <summary>
|
|
/// Gets the "chestnut" emoji.
|
|
/// Description: Chestnut.
|
|
/// </summary>
|
|
public const string Chestnut = "\U0001F330";
|
|
|
|
/// <summary>
|
|
/// Gets the "chicken" emoji.
|
|
/// Description: Chicken.
|
|
/// </summary>
|
|
public const string Chicken = "\U0001F414";
|
|
|
|
/// <summary>
|
|
/// Gets the "child" emoji.
|
|
/// Description: Child.
|
|
/// </summary>
|
|
public const string Child = "\U0001F9D2";
|
|
|
|
/// <summary>
|
|
/// Gets the "children_crossing" emoji.
|
|
/// Description: Children crossing.
|
|
/// </summary>
|
|
public const string ChildrenCrossing = "\U0001F6B8";
|
|
|
|
/// <summary>
|
|
/// Gets the "chipmunk" emoji.
|
|
/// Description: Chipmunk.
|
|
/// </summary>
|
|
public const string Chipmunk = "\U0001F43F";
|
|
|
|
/// <summary>
|
|
/// Gets the "chocolate_bar" emoji.
|
|
/// Description: Chocolate bar.
|
|
/// </summary>
|
|
public const string ChocolateBar = "\U0001F36B";
|
|
|
|
/// <summary>
|
|
/// Gets the "chopsticks" emoji.
|
|
/// Description: Chopsticks.
|
|
/// </summary>
|
|
public const string Chopsticks = "\U0001F962";
|
|
|
|
/// <summary>
|
|
/// Gets the "christmas_tree" emoji.
|
|
/// Description: Christmas tree.
|
|
/// </summary>
|
|
public const string ChristmasTree = "\U0001F384";
|
|
|
|
/// <summary>
|
|
/// Gets the "church" emoji.
|
|
/// Description: Church.
|
|
/// </summary>
|
|
public const string Church = "\U000026EA";
|
|
|
|
/// <summary>
|
|
/// Gets the "cigarette" emoji.
|
|
/// Description: Cigarette.
|
|
/// </summary>
|
|
public const string Cigarette = "\U0001F6AC";
|
|
|
|
/// <summary>
|
|
/// Gets the "cinema" emoji.
|
|
/// Description: Cinema.
|
|
/// </summary>
|
|
public const string Cinema = "\U0001F3A6";
|
|
|
|
/// <summary>
|
|
/// Gets the "circled_m" emoji.
|
|
/// Description: Circled M.
|
|
/// </summary>
|
|
public const string CircledM = "\U000024C2";
|
|
|
|
/// <summary>
|
|
/// Gets the "circus_tent" emoji.
|
|
/// Description: Circus tent.
|
|
/// </summary>
|
|
public const string CircusTent = "\U0001F3AA";
|
|
|
|
/// <summary>
|
|
/// Gets the "cityscape" emoji.
|
|
/// Description: Cityscape.
|
|
/// </summary>
|
|
public const string Cityscape = "\U0001F3D9";
|
|
|
|
/// <summary>
|
|
/// Gets the "cityscape_at_dusk" emoji.
|
|
/// Description: Cityscape at dusk.
|
|
/// </summary>
|
|
public const string CityscapeAtDusk = "\U0001F306";
|
|
|
|
/// <summary>
|
|
/// Gets the "clamp" emoji.
|
|
/// Description: Clamp.
|
|
/// </summary>
|
|
public const string Clamp = "\U0001F5DC";
|
|
|
|
/// <summary>
|
|
/// Gets the "clapper_board" emoji.
|
|
/// Description: Clapper board.
|
|
/// </summary>
|
|
public const string ClapperBoard = "\U0001F3AC";
|
|
|
|
/// <summary>
|
|
/// Gets the "clapping_hands" emoji.
|
|
/// Description: Clapping hands.
|
|
/// </summary>
|
|
public const string ClappingHands = "\U0001F44F";
|
|
|
|
/// <summary>
|
|
/// Gets the "classical_building" emoji.
|
|
/// Description: Classical building.
|
|
/// </summary>
|
|
public const string ClassicalBuilding = "\U0001F3DB";
|
|
|
|
/// <summary>
|
|
/// Gets the "cl_button" emoji.
|
|
/// Description: CL button.
|
|
/// </summary>
|
|
public const string ClButton = "\U0001F191";
|
|
|
|
/// <summary>
|
|
/// Gets the "clinking_beer_mugs" emoji.
|
|
/// Description: Clinking beer mugs.
|
|
/// </summary>
|
|
public const string ClinkingBeerMugs = "\U0001F37B";
|
|
|
|
/// <summary>
|
|
/// Gets the "clinking_glasses" emoji.
|
|
/// Description: Clinking glasses.
|
|
/// </summary>
|
|
public const string ClinkingGlasses = "\U0001F942";
|
|
|
|
/// <summary>
|
|
/// Gets the "clipboard" emoji.
|
|
/// Description: Clipboard.
|
|
/// </summary>
|
|
public const string Clipboard = "\U0001F4CB";
|
|
|
|
/// <summary>
|
|
/// Gets the "clockwise_vertical_arrows" emoji.
|
|
/// Description: Clockwise vertical arrows.
|
|
/// </summary>
|
|
public const string ClockwiseVerticalArrows = "\U0001F503";
|
|
|
|
/// <summary>
|
|
/// Gets the "closed_book" emoji.
|
|
/// Description: Closed book.
|
|
/// </summary>
|
|
public const string ClosedBook = "\U0001F4D5";
|
|
|
|
/// <summary>
|
|
/// Gets the "closed_mailbox_with_lowered_flag" emoji.
|
|
/// Description: Closed mailbox with lowered flag.
|
|
/// </summary>
|
|
public const string ClosedMailboxWithLoweredFlag = "\U0001F4EA";
|
|
|
|
/// <summary>
|
|
/// Gets the "closed_mailbox_with_raised_flag" emoji.
|
|
/// Description: Closed mailbox with raised flag.
|
|
/// </summary>
|
|
public const string ClosedMailboxWithRaisedFlag = "\U0001F4EB";
|
|
|
|
/// <summary>
|
|
/// Gets the "closed_umbrella" emoji.
|
|
/// Description: Closed umbrella.
|
|
/// </summary>
|
|
public const string ClosedUmbrella = "\U0001F302";
|
|
|
|
/// <summary>
|
|
/// Gets the "cloud" emoji.
|
|
/// Description: Cloud.
|
|
/// </summary>
|
|
public const string Cloud = "\U00002601";
|
|
|
|
/// <summary>
|
|
/// Gets the "cloud_with_lightning" emoji.
|
|
/// Description: Cloud with lightning.
|
|
/// </summary>
|
|
public const string CloudWithLightning = "\U0001F329";
|
|
|
|
/// <summary>
|
|
/// Gets the "cloud_with_lightning_and_rain" emoji.
|
|
/// Description: Cloud with lightning and rain.
|
|
/// </summary>
|
|
public const string CloudWithLightningAndRain = "\U000026C8";
|
|
|
|
/// <summary>
|
|
/// Gets the "cloud_with_rain" emoji.
|
|
/// Description: Cloud with rain.
|
|
/// </summary>
|
|
public const string CloudWithRain = "\U0001F327";
|
|
|
|
/// <summary>
|
|
/// Gets the "cloud_with_snow" emoji.
|
|
/// Description: Cloud with snow.
|
|
/// </summary>
|
|
public const string CloudWithSnow = "\U0001F328";
|
|
|
|
/// <summary>
|
|
/// Gets the "clown_face" emoji.
|
|
/// Description: Clown face.
|
|
/// </summary>
|
|
public const string ClownFace = "\U0001F921";
|
|
|
|
/// <summary>
|
|
/// Gets the "club_suit" emoji.
|
|
/// Description: Club suit.
|
|
/// </summary>
|
|
public const string ClubSuit = "\U00002663";
|
|
|
|
/// <summary>
|
|
/// Gets the "clutch_bag" emoji.
|
|
/// Description: Clutch bag.
|
|
/// </summary>
|
|
public const string ClutchBag = "\U0001F45D";
|
|
|
|
/// <summary>
|
|
/// Gets the "coat" emoji.
|
|
/// Description: Coat.
|
|
/// </summary>
|
|
public const string Coat = "\U0001F9E5";
|
|
|
|
/// <summary>
|
|
/// Gets the "cockroach" emoji.
|
|
/// Description: Cockroach.
|
|
/// </summary>
|
|
public const string Cockroach = "\U0001FAB3";
|
|
|
|
/// <summary>
|
|
/// Gets the "cocktail_glass" emoji.
|
|
/// Description: Cocktail glass.
|
|
/// </summary>
|
|
public const string CocktailGlass = "\U0001F378";
|
|
|
|
/// <summary>
|
|
/// Gets the "coconut" emoji.
|
|
/// Description: Coconut.
|
|
/// </summary>
|
|
public const string Coconut = "\U0001F965";
|
|
|
|
/// <summary>
|
|
/// Gets the "coffin" emoji.
|
|
/// Description: Coffin.
|
|
/// </summary>
|
|
public const string Coffin = "\U000026B0";
|
|
|
|
/// <summary>
|
|
/// Gets the "coin" emoji.
|
|
/// Description: Coin.
|
|
/// </summary>
|
|
public const string Coin = "\U0001FA99";
|
|
|
|
/// <summary>
|
|
/// Gets the "cold_face" emoji.
|
|
/// Description: Cold face.
|
|
/// </summary>
|
|
public const string ColdFace = "\U0001F976";
|
|
|
|
/// <summary>
|
|
/// Gets the "collision" emoji.
|
|
/// Description: Collision.
|
|
/// </summary>
|
|
public const string Collision = "\U0001F4A5";
|
|
|
|
/// <summary>
|
|
/// Gets the "comet" emoji.
|
|
/// Description: Comet.
|
|
/// </summary>
|
|
public const string Comet = "\U00002604";
|
|
|
|
/// <summary>
|
|
/// Gets the "compass" emoji.
|
|
/// Description: Compass.
|
|
/// </summary>
|
|
public const string Compass = "\U0001F9ED";
|
|
|
|
/// <summary>
|
|
/// Gets the "computer_disk" emoji.
|
|
/// Description: Computer disk.
|
|
/// </summary>
|
|
public const string ComputerDisk = "\U0001F4BD";
|
|
|
|
/// <summary>
|
|
/// Gets the "computer_mouse" emoji.
|
|
/// Description: Computer mouse.
|
|
/// </summary>
|
|
public const string ComputerMouse = "\U0001F5B1";
|
|
|
|
/// <summary>
|
|
/// Gets the "confetti_ball" emoji.
|
|
/// Description: Confetti ball.
|
|
/// </summary>
|
|
public const string ConfettiBall = "\U0001F38A";
|
|
|
|
/// <summary>
|
|
/// Gets the "confounded_face" emoji.
|
|
/// Description: Confounded face.
|
|
/// </summary>
|
|
public const string ConfoundedFace = "\U0001F616";
|
|
|
|
/// <summary>
|
|
/// Gets the "confused_face" emoji.
|
|
/// Description: Confused face.
|
|
/// </summary>
|
|
public const string ConfusedFace = "\U0001F615";
|
|
|
|
/// <summary>
|
|
/// Gets the "construction" emoji.
|
|
/// Description: Construction.
|
|
/// </summary>
|
|
public const string Construction = "\U0001F6A7";
|
|
|
|
/// <summary>
|
|
/// Gets the "construction_worker" emoji.
|
|
/// Description: Construction worker.
|
|
/// </summary>
|
|
public const string ConstructionWorker = "\U0001F477";
|
|
|
|
/// <summary>
|
|
/// Gets the "control_knobs" emoji.
|
|
/// Description: Control knobs.
|
|
/// </summary>
|
|
public const string ControlKnobs = "\U0001F39B";
|
|
|
|
/// <summary>
|
|
/// Gets the "convenience_store" emoji.
|
|
/// Description: Convenience store.
|
|
/// </summary>
|
|
public const string ConvenienceStore = "\U0001F3EA";
|
|
|
|
/// <summary>
|
|
/// Gets the "cooked_rice" emoji.
|
|
/// Description: Cooked rice.
|
|
/// </summary>
|
|
public const string CookedRice = "\U0001F35A";
|
|
|
|
/// <summary>
|
|
/// Gets the "cookie" emoji.
|
|
/// Description: Cookie.
|
|
/// </summary>
|
|
public const string Cookie = "\U0001F36A";
|
|
|
|
/// <summary>
|
|
/// Gets the "cooking" emoji.
|
|
/// Description: Cooking.
|
|
/// </summary>
|
|
public const string Cooking = "\U0001F373";
|
|
|
|
/// <summary>
|
|
/// Gets the "cool_button" emoji.
|
|
/// Description: COOL button.
|
|
/// </summary>
|
|
public const string CoolButton = "\U0001F192";
|
|
|
|
/// <summary>
|
|
/// Gets the "copyright" emoji.
|
|
/// Description: Copyright.
|
|
/// </summary>
|
|
public const string Copyright = "\U000000A9";
|
|
|
|
/// <summary>
|
|
/// Gets the "couch_and_lamp" emoji.
|
|
/// Description: Couch and lamp.
|
|
/// </summary>
|
|
public const string CouchAndLamp = "\U0001F6CB";
|
|
|
|
/// <summary>
|
|
/// Gets the "counterclockwise_arrows_button" emoji.
|
|
/// Description: Counterclockwise arrows button.
|
|
/// </summary>
|
|
public const string CounterclockwiseArrowsButton = "\U0001F504";
|
|
|
|
/// <summary>
|
|
/// Gets the "couple_with_heart" emoji.
|
|
/// Description: Couple with heart.
|
|
/// </summary>
|
|
public const string CoupleWithHeart = "\U0001F491";
|
|
|
|
/// <summary>
|
|
/// Gets the "cow" emoji.
|
|
/// Description: Cow.
|
|
/// </summary>
|
|
public const string Cow = "\U0001F404";
|
|
|
|
/// <summary>
|
|
/// Gets the "cowboy_hat_face" emoji.
|
|
/// Description: Cowboy hat face.
|
|
/// </summary>
|
|
public const string CowboyHatFace = "\U0001F920";
|
|
|
|
/// <summary>
|
|
/// Gets the "cow_face" emoji.
|
|
/// Description: Cow face.
|
|
/// </summary>
|
|
public const string CowFace = "\U0001F42E";
|
|
|
|
/// <summary>
|
|
/// Gets the "crab" emoji.
|
|
/// Description: Crab.
|
|
/// </summary>
|
|
public const string Crab = "\U0001F980";
|
|
|
|
/// <summary>
|
|
/// Gets the "crayon" emoji.
|
|
/// Description: Crayon.
|
|
/// </summary>
|
|
public const string Crayon = "\U0001F58D";
|
|
|
|
/// <summary>
|
|
/// Gets the "credit_card" emoji.
|
|
/// Description: Credit card.
|
|
/// </summary>
|
|
public const string CreditCard = "\U0001F4B3";
|
|
|
|
/// <summary>
|
|
/// Gets the "crescent_moon" emoji.
|
|
/// Description: Crescent moon.
|
|
/// </summary>
|
|
public const string CrescentMoon = "\U0001F319";
|
|
|
|
/// <summary>
|
|
/// Gets the "cricket" emoji.
|
|
/// Description: Cricket.
|
|
/// </summary>
|
|
public const string Cricket = "\U0001F997";
|
|
|
|
/// <summary>
|
|
/// Gets the "cricket_game" emoji.
|
|
/// Description: Cricket game.
|
|
/// </summary>
|
|
public const string CricketGame = "\U0001F3CF";
|
|
|
|
/// <summary>
|
|
/// Gets the "crocodile" emoji.
|
|
/// Description: Crocodile.
|
|
/// </summary>
|
|
public const string Crocodile = "\U0001F40A";
|
|
|
|
/// <summary>
|
|
/// Gets the "croissant" emoji.
|
|
/// Description: Croissant.
|
|
/// </summary>
|
|
public const string Croissant = "\U0001F950";
|
|
|
|
/// <summary>
|
|
/// Gets the "crossed_fingers" emoji.
|
|
/// Description: Crossed fingers.
|
|
/// </summary>
|
|
public const string CrossedFingers = "\U0001F91E";
|
|
|
|
/// <summary>
|
|
/// Gets the "crossed_flags" emoji.
|
|
/// Description: Crossed flags.
|
|
/// </summary>
|
|
public const string CrossedFlags = "\U0001F38C";
|
|
|
|
/// <summary>
|
|
/// Gets the "crossed_swords" emoji.
|
|
/// Description: Crossed swords.
|
|
/// </summary>
|
|
public const string CrossedSwords = "\U00002694";
|
|
|
|
/// <summary>
|
|
/// Gets the "cross_mark" emoji.
|
|
/// Description: Cross mark.
|
|
/// </summary>
|
|
public const string CrossMark = "\U0000274C";
|
|
|
|
/// <summary>
|
|
/// Gets the "cross_mark_button" emoji.
|
|
/// Description: Cross mark button.
|
|
/// </summary>
|
|
public const string CrossMarkButton = "\U0000274E";
|
|
|
|
/// <summary>
|
|
/// Gets the "crown" emoji.
|
|
/// Description: Crown.
|
|
/// </summary>
|
|
public const string Crown = "\U0001F451";
|
|
|
|
/// <summary>
|
|
/// Gets the "crying_cat" emoji.
|
|
/// Description: Crying cat.
|
|
/// </summary>
|
|
public const string CryingCat = "\U0001F63F";
|
|
|
|
/// <summary>
|
|
/// Gets the "crying_face" emoji.
|
|
/// Description: Crying face.
|
|
/// </summary>
|
|
public const string CryingFace = "\U0001F622";
|
|
|
|
/// <summary>
|
|
/// Gets the "crystal_ball" emoji.
|
|
/// Description: Crystal ball.
|
|
/// </summary>
|
|
public const string CrystalBall = "\U0001F52E";
|
|
|
|
/// <summary>
|
|
/// Gets the "cucumber" emoji.
|
|
/// Description: Cucumber.
|
|
/// </summary>
|
|
public const string Cucumber = "\U0001F952";
|
|
|
|
/// <summary>
|
|
/// Gets the "cupcake" emoji.
|
|
/// Description: Cupcake.
|
|
/// </summary>
|
|
public const string Cupcake = "\U0001F9C1";
|
|
|
|
/// <summary>
|
|
/// Gets the "cup_with_straw" emoji.
|
|
/// Description: Cup with straw.
|
|
/// </summary>
|
|
public const string CupWithStraw = "\U0001F964";
|
|
|
|
/// <summary>
|
|
/// Gets the "curling_stone" emoji.
|
|
/// Description: Curling stone.
|
|
/// </summary>
|
|
public const string CurlingStone = "\U0001F94C";
|
|
|
|
/// <summary>
|
|
/// Gets the "curly_hair" emoji.
|
|
/// Description: Curly hair.
|
|
/// </summary>
|
|
public const string CurlyHair = "\U0001F9B1";
|
|
|
|
/// <summary>
|
|
/// Gets the "curly_loop" emoji.
|
|
/// Description: Curly loop.
|
|
/// </summary>
|
|
public const string CurlyLoop = "\U000027B0";
|
|
|
|
/// <summary>
|
|
/// Gets the "currency_exchange" emoji.
|
|
/// Description: Currency exchange.
|
|
/// </summary>
|
|
public const string CurrencyExchange = "\U0001F4B1";
|
|
|
|
/// <summary>
|
|
/// Gets the "curry_rice" emoji.
|
|
/// Description: Curry rice.
|
|
/// </summary>
|
|
public const string CurryRice = "\U0001F35B";
|
|
|
|
/// <summary>
|
|
/// Gets the "custard" emoji.
|
|
/// Description: Custard.
|
|
/// </summary>
|
|
public const string Custard = "\U0001F36E";
|
|
|
|
/// <summary>
|
|
/// Gets the "customs" emoji.
|
|
/// Description: Customs.
|
|
/// </summary>
|
|
public const string Customs = "\U0001F6C3";
|
|
|
|
/// <summary>
|
|
/// Gets the "cut_of_meat" emoji.
|
|
/// Description: Cut of meat.
|
|
/// </summary>
|
|
public const string CutOfMeat = "\U0001F969";
|
|
|
|
/// <summary>
|
|
/// Gets the "cyclone" emoji.
|
|
/// Description: Cyclone.
|
|
/// </summary>
|
|
public const string Cyclone = "\U0001F300";
|
|
|
|
/// <summary>
|
|
/// Gets the "dagger" emoji.
|
|
/// Description: Dagger.
|
|
/// </summary>
|
|
public const string Dagger = "\U0001F5E1";
|
|
|
|
/// <summary>
|
|
/// Gets the "dango" emoji.
|
|
/// Description: Dango.
|
|
/// </summary>
|
|
public const string Dango = "\U0001F361";
|
|
|
|
/// <summary>
|
|
/// Gets the "dashing_away" emoji.
|
|
/// Description: Dashing away.
|
|
/// </summary>
|
|
public const string DashingAway = "\U0001F4A8";
|
|
|
|
/// <summary>
|
|
/// Gets the "deaf_person" emoji.
|
|
/// Description: Deaf person.
|
|
/// </summary>
|
|
public const string DeafPerson = "\U0001F9CF";
|
|
|
|
/// <summary>
|
|
/// Gets the "deciduous_tree" emoji.
|
|
/// Description: Deciduous tree.
|
|
/// </summary>
|
|
public const string DeciduousTree = "\U0001F333";
|
|
|
|
/// <summary>
|
|
/// Gets the "deer" emoji.
|
|
/// Description: Deer.
|
|
/// </summary>
|
|
public const string Deer = "\U0001F98C";
|
|
|
|
/// <summary>
|
|
/// Gets the "delivery_truck" emoji.
|
|
/// Description: Delivery truck.
|
|
/// </summary>
|
|
public const string DeliveryTruck = "\U0001F69A";
|
|
|
|
/// <summary>
|
|
/// Gets the "department_store" emoji.
|
|
/// Description: Department store.
|
|
/// </summary>
|
|
public const string DepartmentStore = "\U0001F3EC";
|
|
|
|
/// <summary>
|
|
/// Gets the "derelict_house" emoji.
|
|
/// Description: Derelict house.
|
|
/// </summary>
|
|
public const string DerelictHouse = "\U0001F3DA";
|
|
|
|
/// <summary>
|
|
/// Gets the "desert" emoji.
|
|
/// Description: Desert.
|
|
/// </summary>
|
|
public const string Desert = "\U0001F3DC";
|
|
|
|
/// <summary>
|
|
/// Gets the "desert_island" emoji.
|
|
/// Description: Desert island.
|
|
/// </summary>
|
|
public const string DesertIsland = "\U0001F3DD";
|
|
|
|
/// <summary>
|
|
/// Gets the "desktop_computer" emoji.
|
|
/// Description: Desktop computer.
|
|
/// </summary>
|
|
public const string DesktopComputer = "\U0001F5A5";
|
|
|
|
/// <summary>
|
|
/// Gets the "detective" emoji.
|
|
/// Description: Detective.
|
|
/// </summary>
|
|
public const string Detective = "\U0001F575";
|
|
|
|
/// <summary>
|
|
/// Gets the "diamond_suit" emoji.
|
|
/// Description: Diamond suit.
|
|
/// </summary>
|
|
public const string DiamondSuit = "\U00002666";
|
|
|
|
/// <summary>
|
|
/// Gets the "diamond_with_a_dot" emoji.
|
|
/// Description: Diamond with a dot.
|
|
/// </summary>
|
|
public const string DiamondWithADot = "\U0001F4A0";
|
|
|
|
/// <summary>
|
|
/// Gets the "dim_button" emoji.
|
|
/// Description: Dim button.
|
|
/// </summary>
|
|
public const string DimButton = "\U0001F505";
|
|
|
|
/// <summary>
|
|
/// Gets the "disappointed_face" emoji.
|
|
/// Description: Disappointed face.
|
|
/// </summary>
|
|
public const string DisappointedFace = "\U0001F61E";
|
|
|
|
/// <summary>
|
|
/// Gets the "disguised_face" emoji.
|
|
/// Description: Disguised face.
|
|
/// </summary>
|
|
public const string DisguisedFace = "\U0001F978";
|
|
|
|
/// <summary>
|
|
/// Gets the "divide" emoji.
|
|
/// Description: Divide.
|
|
/// </summary>
|
|
public const string Divide = "\U00002797";
|
|
|
|
/// <summary>
|
|
/// Gets the "diving_mask" emoji.
|
|
/// Description: Diving mask.
|
|
/// </summary>
|
|
public const string DivingMask = "\U0001F93F";
|
|
|
|
/// <summary>
|
|
/// Gets the "diya_lamp" emoji.
|
|
/// Description: Diya lamp.
|
|
/// </summary>
|
|
public const string DiyaLamp = "\U0001FA94";
|
|
|
|
/// <summary>
|
|
/// Gets the "dizzy" emoji.
|
|
/// Description: Dizzy.
|
|
/// </summary>
|
|
public const string Dizzy = "\U0001F4AB";
|
|
|
|
/// <summary>
|
|
/// Gets the "dna" emoji.
|
|
/// Description: Dna.
|
|
/// </summary>
|
|
public const string Dna = "\U0001F9EC";
|
|
|
|
/// <summary>
|
|
/// Gets the "dodo" emoji.
|
|
/// Description: Dodo.
|
|
/// </summary>
|
|
public const string Dodo = "\U0001F9A4";
|
|
|
|
/// <summary>
|
|
/// Gets the "dog" emoji.
|
|
/// Description: Dog.
|
|
/// </summary>
|
|
public const string Dog = "\U0001F415";
|
|
|
|
/// <summary>
|
|
/// Gets the "dog_face" emoji.
|
|
/// Description: Dog face.
|
|
/// </summary>
|
|
public const string DogFace = "\U0001F436";
|
|
|
|
/// <summary>
|
|
/// Gets the "dollar_banknote" emoji.
|
|
/// Description: Dollar banknote.
|
|
/// </summary>
|
|
public const string DollarBanknote = "\U0001F4B5";
|
|
|
|
/// <summary>
|
|
/// Gets the "dolphin" emoji.
|
|
/// Description: Dolphin.
|
|
/// </summary>
|
|
public const string Dolphin = "\U0001F42C";
|
|
|
|
/// <summary>
|
|
/// Gets the "door" emoji.
|
|
/// Description: Door.
|
|
/// </summary>
|
|
public const string Door = "\U0001F6AA";
|
|
|
|
/// <summary>
|
|
/// Gets the "dotted_six_pointed_star" emoji.
|
|
/// Description: dotted six pointed star.
|
|
/// </summary>
|
|
public const string DottedSixPointedStar = "\U0001F52F";
|
|
|
|
/// <summary>
|
|
/// Gets the "double_curly_loop" emoji.
|
|
/// Description: Double curly loop.
|
|
/// </summary>
|
|
public const string DoubleCurlyLoop = "\U000027BF";
|
|
|
|
/// <summary>
|
|
/// Gets the "double_exclamation_mark" emoji.
|
|
/// Description: Double exclamation mark.
|
|
/// </summary>
|
|
public const string DoubleExclamationMark = "\U0000203C";
|
|
|
|
/// <summary>
|
|
/// Gets the "doughnut" emoji.
|
|
/// Description: Doughnut.
|
|
/// </summary>
|
|
public const string Doughnut = "\U0001F369";
|
|
|
|
/// <summary>
|
|
/// Gets the "dove" emoji.
|
|
/// Description: Dove.
|
|
/// </summary>
|
|
public const string Dove = "\U0001F54A";
|
|
|
|
/// <summary>
|
|
/// Gets the "down_arrow" emoji.
|
|
/// Description: Down arrow.
|
|
/// </summary>
|
|
public const string DownArrow = "\U00002B07";
|
|
|
|
/// <summary>
|
|
/// Gets the "downcast_face_with_sweat" emoji.
|
|
/// Description: Downcast face with sweat.
|
|
/// </summary>
|
|
public const string DowncastFaceWithSweat = "\U0001F613";
|
|
|
|
/// <summary>
|
|
/// Gets the "down_left_arrow" emoji.
|
|
/// Description: down left arrow.
|
|
/// </summary>
|
|
public const string DownLeftArrow = "\U00002199";
|
|
|
|
/// <summary>
|
|
/// Gets the "down_right_arrow" emoji.
|
|
/// Description: down right arrow.
|
|
/// </summary>
|
|
public const string DownRightArrow = "\U00002198";
|
|
|
|
/// <summary>
|
|
/// Gets the "downwards_button" emoji.
|
|
/// Description: Downwards button.
|
|
/// </summary>
|
|
public const string DownwardsButton = "\U0001F53D";
|
|
|
|
/// <summary>
|
|
/// Gets the "dragon" emoji.
|
|
/// Description: Dragon.
|
|
/// </summary>
|
|
public const string Dragon = "\U0001F409";
|
|
|
|
/// <summary>
|
|
/// Gets the "dragon_face" emoji.
|
|
/// Description: Dragon face.
|
|
/// </summary>
|
|
public const string DragonFace = "\U0001F432";
|
|
|
|
/// <summary>
|
|
/// Gets the "dress" emoji.
|
|
/// Description: Dress.
|
|
/// </summary>
|
|
public const string Dress = "\U0001F457";
|
|
|
|
/// <summary>
|
|
/// Gets the "drooling_face" emoji.
|
|
/// Description: Drooling face.
|
|
/// </summary>
|
|
public const string DroolingFace = "\U0001F924";
|
|
|
|
/// <summary>
|
|
/// Gets the "droplet" emoji.
|
|
/// Description: Droplet.
|
|
/// </summary>
|
|
public const string Droplet = "\U0001F4A7";
|
|
|
|
/// <summary>
|
|
/// Gets the "drop_of_blood" emoji.
|
|
/// Description: Drop of blood.
|
|
/// </summary>
|
|
public const string DropOfBlood = "\U0001FA78";
|
|
|
|
/// <summary>
|
|
/// Gets the "drum" emoji.
|
|
/// Description: Drum.
|
|
/// </summary>
|
|
public const string Drum = "\U0001F941";
|
|
|
|
/// <summary>
|
|
/// Gets the "duck" emoji.
|
|
/// Description: Duck.
|
|
/// </summary>
|
|
public const string Duck = "\U0001F986";
|
|
|
|
/// <summary>
|
|
/// Gets the "dumpling" emoji.
|
|
/// Description: Dumpling.
|
|
/// </summary>
|
|
public const string Dumpling = "\U0001F95F";
|
|
|
|
/// <summary>
|
|
/// Gets the "dvd" emoji.
|
|
/// Description: Dvd.
|
|
/// </summary>
|
|
public const string Dvd = "\U0001F4C0";
|
|
|
|
/// <summary>
|
|
/// Gets the "eagle" emoji.
|
|
/// Description: Eagle.
|
|
/// </summary>
|
|
public const string Eagle = "\U0001F985";
|
|
|
|
/// <summary>
|
|
/// Gets the "ear" emoji.
|
|
/// Description: Ear.
|
|
/// </summary>
|
|
public const string Ear = "\U0001F442";
|
|
|
|
/// <summary>
|
|
/// Gets the "ear_of_corn" emoji.
|
|
/// Description: Ear of corn.
|
|
/// </summary>
|
|
public const string EarOfCorn = "\U0001F33D";
|
|
|
|
/// <summary>
|
|
/// Gets the "ear_with_hearing_aid" emoji.
|
|
/// Description: Ear with hearing aid.
|
|
/// </summary>
|
|
public const string EarWithHearingAid = "\U0001F9BB";
|
|
|
|
/// <summary>
|
|
/// Gets the "egg" emoji.
|
|
/// Description: Egg.
|
|
/// </summary>
|
|
public const string Egg = "\U0001F95A";
|
|
|
|
/// <summary>
|
|
/// Gets the "eggplant" emoji.
|
|
/// Description: Eggplant.
|
|
/// </summary>
|
|
public const string Eggplant = "\U0001F346";
|
|
|
|
/// <summary>
|
|
/// Gets the "eight_o_clock" emoji.
|
|
/// Description: Eight o clock.
|
|
/// </summary>
|
|
public const string EightOClock = "\U0001F557";
|
|
|
|
/// <summary>
|
|
/// Gets the "eight_pointed_star" emoji.
|
|
/// Description: eight pointed star.
|
|
/// </summary>
|
|
public const string EightPointedStar = "\U00002734";
|
|
|
|
/// <summary>
|
|
/// Gets the "eight_spoked_asterisk" emoji.
|
|
/// Description: eight spoked asterisk.
|
|
/// </summary>
|
|
public const string EightSpokedAsterisk = "\U00002733";
|
|
|
|
/// <summary>
|
|
/// Gets the "eight_thirty" emoji.
|
|
/// Description: eight thirty.
|
|
/// </summary>
|
|
public const string EightThirty = "\U0001F563";
|
|
|
|
/// <summary>
|
|
/// Gets the "eject_button" emoji.
|
|
/// Description: Eject button.
|
|
/// </summary>
|
|
public const string EjectButton = "\U000023CF";
|
|
|
|
/// <summary>
|
|
/// Gets the "electric_plug" emoji.
|
|
/// Description: Electric plug.
|
|
/// </summary>
|
|
public const string ElectricPlug = "\U0001F50C";
|
|
|
|
/// <summary>
|
|
/// Gets the "elephant" emoji.
|
|
/// Description: Elephant.
|
|
/// </summary>
|
|
public const string Elephant = "\U0001F418";
|
|
|
|
/// <summary>
|
|
/// Gets the "elevator" emoji.
|
|
/// Description: Elevator.
|
|
/// </summary>
|
|
public const string Elevator = "\U0001F6D7";
|
|
|
|
/// <summary>
|
|
/// Gets the "eleven_o_clock" emoji.
|
|
/// Description: Eleven o clock.
|
|
/// </summary>
|
|
public const string ElevenOClock = "\U0001F55A";
|
|
|
|
/// <summary>
|
|
/// Gets the "eleven_thirty" emoji.
|
|
/// Description: eleven thirty.
|
|
/// </summary>
|
|
public const string ElevenThirty = "\U0001F566";
|
|
|
|
/// <summary>
|
|
/// Gets the "elf" emoji.
|
|
/// Description: Elf.
|
|
/// </summary>
|
|
public const string Elf = "\U0001F9DD";
|
|
|
|
/// <summary>
|
|
/// Gets the "e_mail" emoji.
|
|
/// Description: e mail.
|
|
/// </summary>
|
|
public const string EMail = "\U0001F4E7";
|
|
|
|
/// <summary>
|
|
/// Gets the "end_arrow" emoji.
|
|
/// Description: END arrow.
|
|
/// </summary>
|
|
public const string EndArrow = "\U0001F51A";
|
|
|
|
/// <summary>
|
|
/// Gets the "envelope" emoji.
|
|
/// Description: Envelope.
|
|
/// </summary>
|
|
public const string Envelope = "\U00002709";
|
|
|
|
/// <summary>
|
|
/// Gets the "envelope_with_arrow" emoji.
|
|
/// Description: Envelope with arrow.
|
|
/// </summary>
|
|
public const string EnvelopeWithArrow = "\U0001F4E9";
|
|
|
|
/// <summary>
|
|
/// Gets the "euro_banknote" emoji.
|
|
/// Description: Euro banknote.
|
|
/// </summary>
|
|
public const string EuroBanknote = "\U0001F4B6";
|
|
|
|
/// <summary>
|
|
/// Gets the "evergreen_tree" emoji.
|
|
/// Description: Evergreen tree.
|
|
/// </summary>
|
|
public const string EvergreenTree = "\U0001F332";
|
|
|
|
/// <summary>
|
|
/// Gets the "ewe" emoji.
|
|
/// Description: Ewe.
|
|
/// </summary>
|
|
public const string Ewe = "\U0001F411";
|
|
|
|
/// <summary>
|
|
/// Gets the "exclamation_question_mark" emoji.
|
|
/// Description: Exclamation question mark.
|
|
/// </summary>
|
|
public const string ExclamationQuestionMark = "\U00002049";
|
|
|
|
/// <summary>
|
|
/// Gets the "exploding_head" emoji.
|
|
/// Description: Exploding head.
|
|
/// </summary>
|
|
public const string ExplodingHead = "\U0001F92F";
|
|
|
|
/// <summary>
|
|
/// Gets the "expressionless_face" emoji.
|
|
/// Description: Expressionless face.
|
|
/// </summary>
|
|
public const string ExpressionlessFace = "\U0001F611";
|
|
|
|
/// <summary>
|
|
/// Gets the "eye" emoji.
|
|
/// Description: Eye.
|
|
/// </summary>
|
|
public const string Eye = "\U0001F441";
|
|
|
|
/// <summary>
|
|
/// Gets the "eyes" emoji.
|
|
/// Description: Eyes.
|
|
/// </summary>
|
|
public const string Eyes = "\U0001F440";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_blowing_a_kiss" emoji.
|
|
/// Description: Face blowing a kiss.
|
|
/// </summary>
|
|
public const string FaceBlowingAKiss = "\U0001F618";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_savoring_food" emoji.
|
|
/// Description: Face savoring food.
|
|
/// </summary>
|
|
public const string FaceSavoringFood = "\U0001F60B";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_screaming_in_fear" emoji.
|
|
/// Description: Face screaming in fear.
|
|
/// </summary>
|
|
public const string FaceScreamingInFear = "\U0001F631";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_vomiting" emoji.
|
|
/// Description: Face vomiting.
|
|
/// </summary>
|
|
public const string FaceVomiting = "\U0001F92E";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_hand_over_mouth" emoji.
|
|
/// Description: Face with hand over mouth.
|
|
/// </summary>
|
|
public const string FaceWithHandOverMouth = "\U0001F92D";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_head_bandage" emoji.
|
|
/// Description: face with head bandage.
|
|
/// </summary>
|
|
public const string FaceWithHeadBandage = "\U0001F915";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_medical_mask" emoji.
|
|
/// Description: Face with medical mask.
|
|
/// </summary>
|
|
public const string FaceWithMedicalMask = "\U0001F637";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_monocle" emoji.
|
|
/// Description: Face with monocle.
|
|
/// </summary>
|
|
public const string FaceWithMonocle = "\U0001F9D0";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_open_mouth" emoji.
|
|
/// Description: Face with open mouth.
|
|
/// </summary>
|
|
public const string FaceWithOpenMouth = "\U0001F62E";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_without_mouth" emoji.
|
|
/// Description: Face without mouth.
|
|
/// </summary>
|
|
public const string FaceWithoutMouth = "\U0001F636";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_raised_eyebrow" emoji.
|
|
/// Description: Face with raised eyebrow.
|
|
/// </summary>
|
|
public const string FaceWithRaisedEyebrow = "\U0001F928";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_rolling_eyes" emoji.
|
|
/// Description: Face with rolling eyes.
|
|
/// </summary>
|
|
public const string FaceWithRollingEyes = "\U0001F644";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_steam_from_nose" emoji.
|
|
/// Description: Face with steam from nose.
|
|
/// </summary>
|
|
public const string FaceWithSteamFromNose = "\U0001F624";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_symbols_on_mouth" emoji.
|
|
/// Description: Face with symbols on mouth.
|
|
/// </summary>
|
|
public const string FaceWithSymbolsOnMouth = "\U0001F92C";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_tears_of_joy" emoji.
|
|
/// Description: Face with tears of joy.
|
|
/// </summary>
|
|
public const string FaceWithTearsOfJoy = "\U0001F602";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_thermometer" emoji.
|
|
/// Description: Face with thermometer.
|
|
/// </summary>
|
|
public const string FaceWithThermometer = "\U0001F912";
|
|
|
|
/// <summary>
|
|
/// Gets the "face_with_tongue" emoji.
|
|
/// Description: Face with tongue.
|
|
/// </summary>
|
|
public const string FaceWithTongue = "\U0001F61B";
|
|
|
|
/// <summary>
|
|
/// Gets the "factory" emoji.
|
|
/// Description: Factory.
|
|
/// </summary>
|
|
public const string Factory = "\U0001F3ED";
|
|
|
|
/// <summary>
|
|
/// Gets the "fairy" emoji.
|
|
/// Description: Fairy.
|
|
/// </summary>
|
|
public const string Fairy = "\U0001F9DA";
|
|
|
|
/// <summary>
|
|
/// Gets the "falafel" emoji.
|
|
/// Description: Falafel.
|
|
/// </summary>
|
|
public const string Falafel = "\U0001F9C6";
|
|
|
|
/// <summary>
|
|
/// Gets the "fallen_leaf" emoji.
|
|
/// Description: Fallen leaf.
|
|
/// </summary>
|
|
public const string FallenLeaf = "\U0001F342";
|
|
|
|
/// <summary>
|
|
/// Gets the "family" emoji.
|
|
/// Description: Family.
|
|
/// </summary>
|
|
public const string Family = "\U0001F46A";
|
|
|
|
/// <summary>
|
|
/// Gets the "fast_down_button" emoji.
|
|
/// Description: Fast down button.
|
|
/// </summary>
|
|
public const string FastDownButton = "\U000023EC";
|
|
|
|
/// <summary>
|
|
/// Gets the "fast_forward_button" emoji.
|
|
/// Description: fast forward button.
|
|
/// </summary>
|
|
public const string FastForwardButton = "\U000023E9";
|
|
|
|
/// <summary>
|
|
/// Gets the "fast_reverse_button" emoji.
|
|
/// Description: Fast reverse button.
|
|
/// </summary>
|
|
public const string FastReverseButton = "\U000023EA";
|
|
|
|
/// <summary>
|
|
/// Gets the "fast_up_button" emoji.
|
|
/// Description: Fast up button.
|
|
/// </summary>
|
|
public const string FastUpButton = "\U000023EB";
|
|
|
|
/// <summary>
|
|
/// Gets the "fax_machine" emoji.
|
|
/// Description: Fax machine.
|
|
/// </summary>
|
|
public const string FaxMachine = "\U0001F4E0";
|
|
|
|
/// <summary>
|
|
/// Gets the "fearful_face" emoji.
|
|
/// Description: Fearful face.
|
|
/// </summary>
|
|
public const string FearfulFace = "\U0001F628";
|
|
|
|
/// <summary>
|
|
/// Gets the "feather" emoji.
|
|
/// Description: Feather.
|
|
/// </summary>
|
|
public const string Feather = "\U0001FAB6";
|
|
|
|
/// <summary>
|
|
/// Gets the "female_sign" emoji.
|
|
/// Description: Female sign.
|
|
/// </summary>
|
|
public const string FemaleSign = "\U00002640";
|
|
|
|
/// <summary>
|
|
/// Gets the "ferris_wheel" emoji.
|
|
/// Description: Ferris wheel.
|
|
/// </summary>
|
|
public const string FerrisWheel = "\U0001F3A1";
|
|
|
|
/// <summary>
|
|
/// Gets the "ferry" emoji.
|
|
/// Description: Ferry.
|
|
/// </summary>
|
|
public const string Ferry = "\U000026F4";
|
|
|
|
/// <summary>
|
|
/// Gets the "field_hockey" emoji.
|
|
/// Description: Field hockey.
|
|
/// </summary>
|
|
public const string FieldHockey = "\U0001F3D1";
|
|
|
|
/// <summary>
|
|
/// Gets the "file_cabinet" emoji.
|
|
/// Description: File cabinet.
|
|
/// </summary>
|
|
public const string FileCabinet = "\U0001F5C4";
|
|
|
|
/// <summary>
|
|
/// Gets the "file_folder" emoji.
|
|
/// Description: File folder.
|
|
/// </summary>
|
|
public const string FileFolder = "\U0001F4C1";
|
|
|
|
/// <summary>
|
|
/// Gets the "film_frames" emoji.
|
|
/// Description: Film frames.
|
|
/// </summary>
|
|
public const string FilmFrames = "\U0001F39E";
|
|
|
|
/// <summary>
|
|
/// Gets the "film_projector" emoji.
|
|
/// Description: Film projector.
|
|
/// </summary>
|
|
public const string FilmProjector = "\U0001F4FD";
|
|
|
|
/// <summary>
|
|
/// Gets the "fire" emoji.
|
|
/// Description: Fire.
|
|
/// </summary>
|
|
public const string Fire = "\U0001F525";
|
|
|
|
/// <summary>
|
|
/// Gets the "firecracker" emoji.
|
|
/// Description: Firecracker.
|
|
/// </summary>
|
|
public const string Firecracker = "\U0001F9E8";
|
|
|
|
/// <summary>
|
|
/// Gets the "fire_engine" emoji.
|
|
/// Description: Fire engine.
|
|
/// </summary>
|
|
public const string FireEngine = "\U0001F692";
|
|
|
|
/// <summary>
|
|
/// Gets the "fire_extinguisher" emoji.
|
|
/// Description: Fire extinguisher.
|
|
/// </summary>
|
|
public const string FireExtinguisher = "\U0001F9EF";
|
|
|
|
/// <summary>
|
|
/// Gets the "fireworks" emoji.
|
|
/// Description: Fireworks.
|
|
/// </summary>
|
|
public const string Fireworks = "\U0001F386";
|
|
|
|
/// <summary>
|
|
/// Gets the "1st_place_medal" emoji.
|
|
/// Description: 1st place medal.
|
|
/// </summary>
|
|
public const string FirstPlaceMedal = "\U0001F947";
|
|
|
|
/// <summary>
|
|
/// Gets the "first_quarter_moon" emoji.
|
|
/// Description: First quarter moon.
|
|
/// </summary>
|
|
public const string FirstQuarterMoon = "\U0001F313";
|
|
|
|
/// <summary>
|
|
/// Gets the "first_quarter_moon_face" emoji.
|
|
/// Description: First quarter moon face.
|
|
/// </summary>
|
|
public const string FirstQuarterMoonFace = "\U0001F31B";
|
|
|
|
/// <summary>
|
|
/// Gets the "fish" emoji.
|
|
/// Description: Fish.
|
|
/// </summary>
|
|
public const string Fish = "\U0001F41F";
|
|
|
|
/// <summary>
|
|
/// Gets the "fish_cake_with_swirl" emoji.
|
|
/// Description: Fish cake with swirl.
|
|
/// </summary>
|
|
public const string FishCakeWithSwirl = "\U0001F365";
|
|
|
|
/// <summary>
|
|
/// Gets the "fishing_pole" emoji.
|
|
/// Description: Fishing pole.
|
|
/// </summary>
|
|
public const string FishingPole = "\U0001F3A3";
|
|
|
|
/// <summary>
|
|
/// Gets the "five_o_clock" emoji.
|
|
/// Description: Five o clock.
|
|
/// </summary>
|
|
public const string FiveOClock = "\U0001F554";
|
|
|
|
/// <summary>
|
|
/// Gets the "five_thirty" emoji.
|
|
/// Description: five thirty.
|
|
/// </summary>
|
|
public const string FiveThirty = "\U0001F560";
|
|
|
|
/// <summary>
|
|
/// Gets the "flag_in_hole" emoji.
|
|
/// Description: Flag in hole.
|
|
/// </summary>
|
|
public const string FlagInHole = "\U000026F3";
|
|
|
|
/// <summary>
|
|
/// Gets the "flamingo" emoji.
|
|
/// Description: Flamingo.
|
|
/// </summary>
|
|
public const string Flamingo = "\U0001F9A9";
|
|
|
|
/// <summary>
|
|
/// Gets the "flashlight" emoji.
|
|
/// Description: Flashlight.
|
|
/// </summary>
|
|
public const string Flashlight = "\U0001F526";
|
|
|
|
/// <summary>
|
|
/// Gets the "flatbread" emoji.
|
|
/// Description: Flatbread.
|
|
/// </summary>
|
|
public const string Flatbread = "\U0001FAD3";
|
|
|
|
/// <summary>
|
|
/// Gets the "flat_shoe" emoji.
|
|
/// Description: Flat shoe.
|
|
/// </summary>
|
|
public const string FlatShoe = "\U0001F97F";
|
|
|
|
/// <summary>
|
|
/// Gets the "fleur_de_lis" emoji.
|
|
/// Description: fleur de lis.
|
|
/// </summary>
|
|
public const string FleurDeLis = "\U0000269C";
|
|
|
|
/// <summary>
|
|
/// Gets the "flexed_biceps" emoji.
|
|
/// Description: Flexed biceps.
|
|
/// </summary>
|
|
public const string FlexedBiceps = "\U0001F4AA";
|
|
|
|
/// <summary>
|
|
/// Gets the "floppy_disk" emoji.
|
|
/// Description: Floppy disk.
|
|
/// </summary>
|
|
public const string FloppyDisk = "\U0001F4BE";
|
|
|
|
/// <summary>
|
|
/// Gets the "flower_playing_cards" emoji.
|
|
/// Description: Flower playing cards.
|
|
/// </summary>
|
|
public const string FlowerPlayingCards = "\U0001F3B4";
|
|
|
|
/// <summary>
|
|
/// Gets the "flushed_face" emoji.
|
|
/// Description: Flushed face.
|
|
/// </summary>
|
|
public const string FlushedFace = "\U0001F633";
|
|
|
|
/// <summary>
|
|
/// Gets the "fly" emoji.
|
|
/// Description: Fly.
|
|
/// </summary>
|
|
public const string Fly = "\U0001FAB0";
|
|
|
|
/// <summary>
|
|
/// Gets the "flying_disc" emoji.
|
|
/// Description: Flying disc.
|
|
/// </summary>
|
|
public const string FlyingDisc = "\U0001F94F";
|
|
|
|
/// <summary>
|
|
/// Gets the "flying_saucer" emoji.
|
|
/// Description: Flying saucer.
|
|
/// </summary>
|
|
public const string FlyingSaucer = "\U0001F6F8";
|
|
|
|
/// <summary>
|
|
/// Gets the "fog" emoji.
|
|
/// Description: Fog.
|
|
/// </summary>
|
|
public const string Fog = "\U0001F32B";
|
|
|
|
/// <summary>
|
|
/// Gets the "foggy" emoji.
|
|
/// Description: Foggy.
|
|
/// </summary>
|
|
public const string Foggy = "\U0001F301";
|
|
|
|
/// <summary>
|
|
/// Gets the "folded_hands" emoji.
|
|
/// Description: Folded hands.
|
|
/// </summary>
|
|
public const string FoldedHands = "\U0001F64F";
|
|
|
|
/// <summary>
|
|
/// Gets the "fondue" emoji.
|
|
/// Description: Fondue.
|
|
/// </summary>
|
|
public const string Fondue = "\U0001FAD5";
|
|
|
|
/// <summary>
|
|
/// Gets the "foot" emoji.
|
|
/// Description: Foot.
|
|
/// </summary>
|
|
public const string Foot = "\U0001F9B6";
|
|
|
|
/// <summary>
|
|
/// Gets the "footprints" emoji.
|
|
/// Description: Footprints.
|
|
/// </summary>
|
|
public const string Footprints = "\U0001F463";
|
|
|
|
/// <summary>
|
|
/// Gets the "fork_and_knife" emoji.
|
|
/// Description: Fork and knife.
|
|
/// </summary>
|
|
public const string ForkAndKnife = "\U0001F374";
|
|
|
|
/// <summary>
|
|
/// Gets the "fork_and_knife_with_plate" emoji.
|
|
/// Description: Fork and knife with plate.
|
|
/// </summary>
|
|
public const string ForkAndKnifeWithPlate = "\U0001F37D";
|
|
|
|
/// <summary>
|
|
/// Gets the "fortune_cookie" emoji.
|
|
/// Description: Fortune cookie.
|
|
/// </summary>
|
|
public const string FortuneCookie = "\U0001F960";
|
|
|
|
/// <summary>
|
|
/// Gets the "fountain" emoji.
|
|
/// Description: Fountain.
|
|
/// </summary>
|
|
public const string Fountain = "\U000026F2";
|
|
|
|
/// <summary>
|
|
/// Gets the "fountain_pen" emoji.
|
|
/// Description: Fountain pen.
|
|
/// </summary>
|
|
public const string FountainPen = "\U0001F58B";
|
|
|
|
/// <summary>
|
|
/// Gets the "four_leaf_clover" emoji.
|
|
/// Description: Four leaf clover.
|
|
/// </summary>
|
|
public const string FourLeafClover = "\U0001F340";
|
|
|
|
/// <summary>
|
|
/// Gets the "four_o_clock" emoji.
|
|
/// Description: Four o clock.
|
|
/// </summary>
|
|
public const string FourOClock = "\U0001F553";
|
|
|
|
/// <summary>
|
|
/// Gets the "four_thirty" emoji.
|
|
/// Description: four thirty.
|
|
/// </summary>
|
|
public const string FourThirty = "\U0001F55F";
|
|
|
|
/// <summary>
|
|
/// Gets the "fox" emoji.
|
|
/// Description: Fox.
|
|
/// </summary>
|
|
public const string Fox = "\U0001F98A";
|
|
|
|
/// <summary>
|
|
/// Gets the "framed_picture" emoji.
|
|
/// Description: Framed picture.
|
|
/// </summary>
|
|
public const string FramedPicture = "\U0001F5BC";
|
|
|
|
/// <summary>
|
|
/// Gets the "free_button" emoji.
|
|
/// Description: FREE button.
|
|
/// </summary>
|
|
public const string FreeButton = "\U0001F193";
|
|
|
|
/// <summary>
|
|
/// Gets the "french_fries" emoji.
|
|
/// Description: French fries.
|
|
/// </summary>
|
|
public const string FrenchFries = "\U0001F35F";
|
|
|
|
/// <summary>
|
|
/// Gets the "fried_shrimp" emoji.
|
|
/// Description: Fried shrimp.
|
|
/// </summary>
|
|
public const string FriedShrimp = "\U0001F364";
|
|
|
|
/// <summary>
|
|
/// Gets the "frog" emoji.
|
|
/// Description: Frog.
|
|
/// </summary>
|
|
public const string Frog = "\U0001F438";
|
|
|
|
/// <summary>
|
|
/// Gets the "front_facing_baby_chick" emoji.
|
|
/// Description: front facing baby chick.
|
|
/// </summary>
|
|
public const string FrontFacingBabyChick = "\U0001F425";
|
|
|
|
/// <summary>
|
|
/// Gets the "frowning_face" emoji.
|
|
/// Description: Frowning face.
|
|
/// </summary>
|
|
public const string FrowningFace = "\U00002639";
|
|
|
|
/// <summary>
|
|
/// Gets the "frowning_face_with_open_mouth" emoji.
|
|
/// Description: Frowning face with open mouth.
|
|
/// </summary>
|
|
public const string FrowningFaceWithOpenMouth = "\U0001F626";
|
|
|
|
/// <summary>
|
|
/// Gets the "fuel_pump" emoji.
|
|
/// Description: Fuel pump.
|
|
/// </summary>
|
|
public const string FuelPump = "\U000026FD";
|
|
|
|
/// <summary>
|
|
/// Gets the "full_moon" emoji.
|
|
/// Description: Full moon.
|
|
/// </summary>
|
|
public const string FullMoon = "\U0001F315";
|
|
|
|
/// <summary>
|
|
/// Gets the "full_moon_face" emoji.
|
|
/// Description: Full moon face.
|
|
/// </summary>
|
|
public const string FullMoonFace = "\U0001F31D";
|
|
|
|
/// <summary>
|
|
/// Gets the "funeral_urn" emoji.
|
|
/// Description: Funeral urn.
|
|
/// </summary>
|
|
public const string FuneralUrn = "\U000026B1";
|
|
|
|
/// <summary>
|
|
/// Gets the "game_die" emoji.
|
|
/// Description: Game die.
|
|
/// </summary>
|
|
public const string GameDie = "\U0001F3B2";
|
|
|
|
/// <summary>
|
|
/// Gets the "garlic" emoji.
|
|
/// Description: Garlic.
|
|
/// </summary>
|
|
public const string Garlic = "\U0001F9C4";
|
|
|
|
/// <summary>
|
|
/// Gets the "gear" emoji.
|
|
/// Description: Gear.
|
|
/// </summary>
|
|
public const string Gear = "\U00002699";
|
|
|
|
/// <summary>
|
|
/// Gets the "gemini" emoji.
|
|
/// Description: Gemini.
|
|
/// </summary>
|
|
public const string Gemini = "\U0000264A";
|
|
|
|
/// <summary>
|
|
/// Gets the "gem_stone" emoji.
|
|
/// Description: Gem stone.
|
|
/// </summary>
|
|
public const string GemStone = "\U0001F48E";
|
|
|
|
/// <summary>
|
|
/// Gets the "genie" emoji.
|
|
/// Description: Genie.
|
|
/// </summary>
|
|
public const string Genie = "\U0001F9DE";
|
|
|
|
/// <summary>
|
|
/// Gets the "ghost" emoji.
|
|
/// Description: Ghost.
|
|
/// </summary>
|
|
public const string Ghost = "\U0001F47B";
|
|
|
|
/// <summary>
|
|
/// Gets the "giraffe" emoji.
|
|
/// Description: Giraffe.
|
|
/// </summary>
|
|
public const string Giraffe = "\U0001F992";
|
|
|
|
/// <summary>
|
|
/// Gets the "girl" emoji.
|
|
/// Description: Girl.
|
|
/// </summary>
|
|
public const string Girl = "\U0001F467";
|
|
|
|
/// <summary>
|
|
/// Gets the "glasses" emoji.
|
|
/// Description: Glasses.
|
|
/// </summary>
|
|
public const string Glasses = "\U0001F453";
|
|
|
|
/// <summary>
|
|
/// Gets the "glass_of_milk" emoji.
|
|
/// Description: Glass of milk.
|
|
/// </summary>
|
|
public const string GlassOfMilk = "\U0001F95B";
|
|
|
|
/// <summary>
|
|
/// Gets the "globe_showing_americas" emoji.
|
|
/// Description: Globe showing americas.
|
|
/// </summary>
|
|
public const string GlobeShowingAmericas = "\U0001F30E";
|
|
|
|
/// <summary>
|
|
/// Gets the "globe_showing_asia_australia" emoji.
|
|
/// Description: globe showing Asia Australia.
|
|
/// </summary>
|
|
public const string GlobeShowingAsiaAustralia = "\U0001F30F";
|
|
|
|
/// <summary>
|
|
/// Gets the "globe_showing_europe_africa" emoji.
|
|
/// Description: globe showing Europe Africa.
|
|
/// </summary>
|
|
public const string GlobeShowingEuropeAfrica = "\U0001F30D";
|
|
|
|
/// <summary>
|
|
/// Gets the "globe_with_meridians" emoji.
|
|
/// Description: Globe with meridians.
|
|
/// </summary>
|
|
public const string GlobeWithMeridians = "\U0001F310";
|
|
|
|
/// <summary>
|
|
/// Gets the "gloves" emoji.
|
|
/// Description: Gloves.
|
|
/// </summary>
|
|
public const string Gloves = "\U0001F9E4";
|
|
|
|
/// <summary>
|
|
/// Gets the "glowing_star" emoji.
|
|
/// Description: Glowing star.
|
|
/// </summary>
|
|
public const string GlowingStar = "\U0001F31F";
|
|
|
|
/// <summary>
|
|
/// Gets the "goal_net" emoji.
|
|
/// Description: Goal net.
|
|
/// </summary>
|
|
public const string GoalNet = "\U0001F945";
|
|
|
|
/// <summary>
|
|
/// Gets the "goat" emoji.
|
|
/// Description: Goat.
|
|
/// </summary>
|
|
public const string Goat = "\U0001F410";
|
|
|
|
/// <summary>
|
|
/// Gets the "goblin" emoji.
|
|
/// Description: Goblin.
|
|
/// </summary>
|
|
public const string Goblin = "\U0001F47A";
|
|
|
|
/// <summary>
|
|
/// Gets the "goggles" emoji.
|
|
/// Description: Goggles.
|
|
/// </summary>
|
|
public const string Goggles = "\U0001F97D";
|
|
|
|
/// <summary>
|
|
/// Gets the "gorilla" emoji.
|
|
/// Description: Gorilla.
|
|
/// </summary>
|
|
public const string Gorilla = "\U0001F98D";
|
|
|
|
/// <summary>
|
|
/// Gets the "graduation_cap" emoji.
|
|
/// Description: Graduation cap.
|
|
/// </summary>
|
|
public const string GraduationCap = "\U0001F393";
|
|
|
|
/// <summary>
|
|
/// Gets the "grapes" emoji.
|
|
/// Description: Grapes.
|
|
/// </summary>
|
|
public const string Grapes = "\U0001F347";
|
|
|
|
/// <summary>
|
|
/// Gets the "green_apple" emoji.
|
|
/// Description: Green apple.
|
|
/// </summary>
|
|
public const string GreenApple = "\U0001F34F";
|
|
|
|
/// <summary>
|
|
/// Gets the "green_book" emoji.
|
|
/// Description: Green book.
|
|
/// </summary>
|
|
public const string GreenBook = "\U0001F4D7";
|
|
|
|
/// <summary>
|
|
/// Gets the "green_circle" emoji.
|
|
/// Description: Green circle.
|
|
/// </summary>
|
|
public const string GreenCircle = "\U0001F7E2";
|
|
|
|
/// <summary>
|
|
/// Gets the "green_heart" emoji.
|
|
/// Description: Green heart.
|
|
/// </summary>
|
|
public const string GreenHeart = "\U0001F49A";
|
|
|
|
/// <summary>
|
|
/// Gets the "green_salad" emoji.
|
|
/// Description: Green salad.
|
|
/// </summary>
|
|
public const string GreenSalad = "\U0001F957";
|
|
|
|
/// <summary>
|
|
/// Gets the "green_square" emoji.
|
|
/// Description: Green square.
|
|
/// </summary>
|
|
public const string GreenSquare = "\U0001F7E9";
|
|
|
|
/// <summary>
|
|
/// Gets the "grimacing_face" emoji.
|
|
/// Description: Grimacing face.
|
|
/// </summary>
|
|
public const string GrimacingFace = "\U0001F62C";
|
|
|
|
/// <summary>
|
|
/// Gets the "grinning_cat" emoji.
|
|
/// Description: Grinning cat.
|
|
/// </summary>
|
|
public const string GrinningCat = "\U0001F63A";
|
|
|
|
/// <summary>
|
|
/// Gets the "grinning_cat_with_smiling_eyes" emoji.
|
|
/// Description: Grinning cat with smiling eyes.
|
|
/// </summary>
|
|
public const string GrinningCatWithSmilingEyes = "\U0001F638";
|
|
|
|
/// <summary>
|
|
/// Gets the "grinning_face" emoji.
|
|
/// Description: Grinning face.
|
|
/// </summary>
|
|
public const string GrinningFace = "\U0001F600";
|
|
|
|
/// <summary>
|
|
/// Gets the "grinning_face_with_big_eyes" emoji.
|
|
/// Description: Grinning face with big eyes.
|
|
/// </summary>
|
|
public const string GrinningFaceWithBigEyes = "\U0001F603";
|
|
|
|
/// <summary>
|
|
/// Gets the "grinning_face_with_smiling_eyes" emoji.
|
|
/// Description: Grinning face with smiling eyes.
|
|
/// </summary>
|
|
public const string GrinningFaceWithSmilingEyes = "\U0001F604";
|
|
|
|
/// <summary>
|
|
/// Gets the "grinning_face_with_sweat" emoji.
|
|
/// Description: Grinning face with sweat.
|
|
/// </summary>
|
|
public const string GrinningFaceWithSweat = "\U0001F605";
|
|
|
|
/// <summary>
|
|
/// Gets the "grinning_squinting_face" emoji.
|
|
/// Description: Grinning squinting face.
|
|
/// </summary>
|
|
public const string GrinningSquintingFace = "\U0001F606";
|
|
|
|
/// <summary>
|
|
/// Gets the "growing_heart" emoji.
|
|
/// Description: Growing heart.
|
|
/// </summary>
|
|
public const string GrowingHeart = "\U0001F497";
|
|
|
|
/// <summary>
|
|
/// Gets the "guard" emoji.
|
|
/// Description: Guard.
|
|
/// </summary>
|
|
public const string Guard = "\U0001F482";
|
|
|
|
/// <summary>
|
|
/// Gets the "guide_dog" emoji.
|
|
/// Description: Guide dog.
|
|
/// </summary>
|
|
public const string GuideDog = "\U0001F9AE";
|
|
|
|
/// <summary>
|
|
/// Gets the "guitar" emoji.
|
|
/// Description: Guitar.
|
|
/// </summary>
|
|
public const string Guitar = "\U0001F3B8";
|
|
|
|
/// <summary>
|
|
/// Gets the "hamburger" emoji.
|
|
/// Description: Hamburger.
|
|
/// </summary>
|
|
public const string Hamburger = "\U0001F354";
|
|
|
|
/// <summary>
|
|
/// Gets the "hammer" emoji.
|
|
/// Description: Hammer.
|
|
/// </summary>
|
|
public const string Hammer = "\U0001F528";
|
|
|
|
/// <summary>
|
|
/// Gets the "hammer_and_pick" emoji.
|
|
/// Description: Hammer and pick.
|
|
/// </summary>
|
|
public const string HammerAndPick = "\U00002692";
|
|
|
|
/// <summary>
|
|
/// Gets the "hammer_and_wrench" emoji.
|
|
/// Description: Hammer and wrench.
|
|
/// </summary>
|
|
public const string HammerAndWrench = "\U0001F6E0";
|
|
|
|
/// <summary>
|
|
/// Gets the "hamster" emoji.
|
|
/// Description: Hamster.
|
|
/// </summary>
|
|
public const string Hamster = "\U0001F439";
|
|
|
|
/// <summary>
|
|
/// Gets the "handbag" emoji.
|
|
/// Description: Handbag.
|
|
/// </summary>
|
|
public const string Handbag = "\U0001F45C";
|
|
|
|
/// <summary>
|
|
/// Gets the "handshake" emoji.
|
|
/// Description: Handshake.
|
|
/// </summary>
|
|
public const string Handshake = "\U0001F91D";
|
|
|
|
/// <summary>
|
|
/// Gets the "hand_with_fingers_splayed" emoji.
|
|
/// Description: Hand with fingers splayed.
|
|
/// </summary>
|
|
public const string HandWithFingersSplayed = "\U0001F590";
|
|
|
|
/// <summary>
|
|
/// Gets the "hatching_chick" emoji.
|
|
/// Description: Hatching chick.
|
|
/// </summary>
|
|
public const string HatchingChick = "\U0001F423";
|
|
|
|
/// <summary>
|
|
/// Gets the "headphone" emoji.
|
|
/// Description: Headphone.
|
|
/// </summary>
|
|
public const string Headphone = "\U0001F3A7";
|
|
|
|
/// <summary>
|
|
/// Gets the "headstone" emoji.
|
|
/// Description: Headstone.
|
|
/// </summary>
|
|
public const string Headstone = "\U0001FAA6";
|
|
|
|
/// <summary>
|
|
/// Gets the "hear_no_evil_monkey" emoji.
|
|
/// Description: hear no evil monkey.
|
|
/// </summary>
|
|
public const string HearNoEvilMonkey = "\U0001F649";
|
|
|
|
/// <summary>
|
|
/// Gets the "heart_decoration" emoji.
|
|
/// Description: Heart decoration.
|
|
/// </summary>
|
|
public const string HeartDecoration = "\U0001F49F";
|
|
|
|
/// <summary>
|
|
/// Gets the "heart_exclamation" emoji.
|
|
/// Description: Heart exclamation.
|
|
/// </summary>
|
|
public const string HeartExclamation = "\U00002763";
|
|
|
|
/// <summary>
|
|
/// Gets the "heart_suit" emoji.
|
|
/// Description: Heart suit.
|
|
/// </summary>
|
|
public const string HeartSuit = "\U00002665";
|
|
|
|
/// <summary>
|
|
/// Gets the "heart_with_arrow" emoji.
|
|
/// Description: Heart with arrow.
|
|
/// </summary>
|
|
public const string HeartWithArrow = "\U0001F498";
|
|
|
|
/// <summary>
|
|
/// Gets the "heart_with_ribbon" emoji.
|
|
/// Description: Heart with ribbon.
|
|
/// </summary>
|
|
public const string HeartWithRibbon = "\U0001F49D";
|
|
|
|
/// <summary>
|
|
/// Gets the "heavy_dollar_sign" emoji.
|
|
/// Description: Heavy dollar sign.
|
|
/// </summary>
|
|
public const string HeavyDollarSign = "\U0001F4B2";
|
|
|
|
/// <summary>
|
|
/// Gets the "hedgehog" emoji.
|
|
/// Description: Hedgehog.
|
|
/// </summary>
|
|
public const string Hedgehog = "\U0001F994";
|
|
|
|
/// <summary>
|
|
/// Gets the "helicopter" emoji.
|
|
/// Description: Helicopter.
|
|
/// </summary>
|
|
public const string Helicopter = "\U0001F681";
|
|
|
|
/// <summary>
|
|
/// Gets the "herb" emoji.
|
|
/// Description: Herb.
|
|
/// </summary>
|
|
public const string Herb = "\U0001F33F";
|
|
|
|
/// <summary>
|
|
/// Gets the "hibiscus" emoji.
|
|
/// Description: Hibiscus.
|
|
/// </summary>
|
|
public const string Hibiscus = "\U0001F33A";
|
|
|
|
/// <summary>
|
|
/// Gets the "high_heeled_shoe" emoji.
|
|
/// Description: high heeled shoe.
|
|
/// </summary>
|
|
public const string HighHeeledShoe = "\U0001F460";
|
|
|
|
/// <summary>
|
|
/// Gets the "high_speed_train" emoji.
|
|
/// Description: high speed train.
|
|
/// </summary>
|
|
public const string HighSpeedTrain = "\U0001F684";
|
|
|
|
/// <summary>
|
|
/// Gets the "high_voltage" emoji.
|
|
/// Description: High voltage.
|
|
/// </summary>
|
|
public const string HighVoltage = "\U000026A1";
|
|
|
|
/// <summary>
|
|
/// Gets the "hiking_boot" emoji.
|
|
/// Description: Hiking boot.
|
|
/// </summary>
|
|
public const string HikingBoot = "\U0001F97E";
|
|
|
|
/// <summary>
|
|
/// Gets the "hindu_temple" emoji.
|
|
/// Description: Hindu temple.
|
|
/// </summary>
|
|
public const string HinduTemple = "\U0001F6D5";
|
|
|
|
/// <summary>
|
|
/// Gets the "hippopotamus" emoji.
|
|
/// Description: Hippopotamus.
|
|
/// </summary>
|
|
public const string Hippopotamus = "\U0001F99B";
|
|
|
|
/// <summary>
|
|
/// Gets the "hole" emoji.
|
|
/// Description: Hole.
|
|
/// </summary>
|
|
public const string Hole = "\U0001F573";
|
|
|
|
/// <summary>
|
|
/// Gets the "hollow_red_circle" emoji.
|
|
/// Description: Hollow red circle.
|
|
/// </summary>
|
|
public const string HollowRedCircle = "\U00002B55";
|
|
|
|
/// <summary>
|
|
/// Gets the "honeybee" emoji.
|
|
/// Description: Honeybee.
|
|
/// </summary>
|
|
public const string Honeybee = "\U0001F41D";
|
|
|
|
/// <summary>
|
|
/// Gets the "honey_pot" emoji.
|
|
/// Description: Honey pot.
|
|
/// </summary>
|
|
public const string HoneyPot = "\U0001F36F";
|
|
|
|
/// <summary>
|
|
/// Gets the "hook" emoji.
|
|
/// Description: Hook.
|
|
/// </summary>
|
|
public const string Hook = "\U0001FA9D";
|
|
|
|
/// <summary>
|
|
/// Gets the "horizontal_traffic_light" emoji.
|
|
/// Description: Horizontal traffic light.
|
|
/// </summary>
|
|
public const string HorizontalTrafficLight = "\U0001F6A5";
|
|
|
|
/// <summary>
|
|
/// Gets the "horse" emoji.
|
|
/// Description: Horse.
|
|
/// </summary>
|
|
public const string Horse = "\U0001F40E";
|
|
|
|
/// <summary>
|
|
/// Gets the "horse_face" emoji.
|
|
/// Description: Horse face.
|
|
/// </summary>
|
|
public const string HorseFace = "\U0001F434";
|
|
|
|
/// <summary>
|
|
/// Gets the "horse_racing" emoji.
|
|
/// Description: Horse racing.
|
|
/// </summary>
|
|
public const string HorseRacing = "\U0001F3C7";
|
|
|
|
/// <summary>
|
|
/// Gets the "hospital" emoji.
|
|
/// Description: Hospital.
|
|
/// </summary>
|
|
public const string Hospital = "\U0001F3E5";
|
|
|
|
/// <summary>
|
|
/// Gets the "hot_beverage" emoji.
|
|
/// Description: Hot beverage.
|
|
/// </summary>
|
|
public const string HotBeverage = "\U00002615";
|
|
|
|
/// <summary>
|
|
/// Gets the "hot_dog" emoji.
|
|
/// Description: Hot dog.
|
|
/// </summary>
|
|
public const string HotDog = "\U0001F32D";
|
|
|
|
/// <summary>
|
|
/// Gets the "hotel" emoji.
|
|
/// Description: Hotel.
|
|
/// </summary>
|
|
public const string Hotel = "\U0001F3E8";
|
|
|
|
/// <summary>
|
|
/// Gets the "hot_face" emoji.
|
|
/// Description: Hot face.
|
|
/// </summary>
|
|
public const string HotFace = "\U0001F975";
|
|
|
|
/// <summary>
|
|
/// Gets the "hot_pepper" emoji.
|
|
/// Description: Hot pepper.
|
|
/// </summary>
|
|
public const string HotPepper = "\U0001F336";
|
|
|
|
/// <summary>
|
|
/// Gets the "hot_springs" emoji.
|
|
/// Description: Hot springs.
|
|
/// </summary>
|
|
public const string HotSprings = "\U00002668";
|
|
|
|
/// <summary>
|
|
/// Gets the "hourglass_done" emoji.
|
|
/// Description: Hourglass done.
|
|
/// </summary>
|
|
public const string HourglassDone = "\U0000231B";
|
|
|
|
/// <summary>
|
|
/// Gets the "hourglass_not_done" emoji.
|
|
/// Description: Hourglass not done.
|
|
/// </summary>
|
|
public const string HourglassNotDone = "\U000023F3";
|
|
|
|
/// <summary>
|
|
/// Gets the "house" emoji.
|
|
/// Description: House.
|
|
/// </summary>
|
|
public const string House = "\U0001F3E0";
|
|
|
|
/// <summary>
|
|
/// Gets the "houses" emoji.
|
|
/// Description: Houses.
|
|
/// </summary>
|
|
public const string Houses = "\U0001F3D8";
|
|
|
|
/// <summary>
|
|
/// Gets the "house_with_garden" emoji.
|
|
/// Description: House with garden.
|
|
/// </summary>
|
|
public const string HouseWithGarden = "\U0001F3E1";
|
|
|
|
/// <summary>
|
|
/// Gets the "hugging_face" emoji.
|
|
/// Description: Hugging face.
|
|
/// </summary>
|
|
public const string HuggingFace = "\U0001F917";
|
|
|
|
/// <summary>
|
|
/// Gets the "hundred_points" emoji.
|
|
/// Description: Hundred points.
|
|
/// </summary>
|
|
public const string HundredPoints = "\U0001F4AF";
|
|
|
|
/// <summary>
|
|
/// Gets the "hushed_face" emoji.
|
|
/// Description: Hushed face.
|
|
/// </summary>
|
|
public const string HushedFace = "\U0001F62F";
|
|
|
|
/// <summary>
|
|
/// Gets the "hut" emoji.
|
|
/// Description: Hut.
|
|
/// </summary>
|
|
public const string Hut = "\U0001F6D6";
|
|
|
|
/// <summary>
|
|
/// Gets the "ice" emoji.
|
|
/// Description: Ice.
|
|
/// </summary>
|
|
public const string Ice = "\U0001F9CA";
|
|
|
|
/// <summary>
|
|
/// Gets the "ice_cream" emoji.
|
|
/// Description: Ice cream.
|
|
/// </summary>
|
|
public const string IceCream = "\U0001F368";
|
|
|
|
/// <summary>
|
|
/// Gets the "ice_hockey" emoji.
|
|
/// Description: Ice hockey.
|
|
/// </summary>
|
|
public const string IceHockey = "\U0001F3D2";
|
|
|
|
/// <summary>
|
|
/// Gets the "ice_skate" emoji.
|
|
/// Description: Ice skate.
|
|
/// </summary>
|
|
public const string IceSkate = "\U000026F8";
|
|
|
|
/// <summary>
|
|
/// Gets the "id_button" emoji.
|
|
/// Description: ID button.
|
|
/// </summary>
|
|
public const string IdButton = "\U0001F194";
|
|
|
|
/// <summary>
|
|
/// Gets the "inbox_tray" emoji.
|
|
/// Description: Inbox tray.
|
|
/// </summary>
|
|
public const string InboxTray = "\U0001F4E5";
|
|
|
|
/// <summary>
|
|
/// Gets the "incoming_envelope" emoji.
|
|
/// Description: Incoming envelope.
|
|
/// </summary>
|
|
public const string IncomingEnvelope = "\U0001F4E8";
|
|
|
|
/// <summary>
|
|
/// Gets the "index_pointing_up" emoji.
|
|
/// Description: Index pointing up.
|
|
/// </summary>
|
|
public const string IndexPointingUp = "\U0000261D";
|
|
|
|
/// <summary>
|
|
/// Gets the "infinity" emoji.
|
|
/// Description: Infinity.
|
|
/// </summary>
|
|
public const string Infinity = "\U0000267E";
|
|
|
|
/// <summary>
|
|
/// Gets the "information" emoji.
|
|
/// Description: Information.
|
|
/// </summary>
|
|
public const string Information = "\U00002139";
|
|
|
|
/// <summary>
|
|
/// Gets the "input_latin_letters" emoji.
|
|
/// Description: Input latin letters.
|
|
/// </summary>
|
|
public const string InputLatinLetters = "\U0001F524";
|
|
|
|
/// <summary>
|
|
/// Gets the "input_latin_lowercase" emoji.
|
|
/// Description: Input latin lowercase.
|
|
/// </summary>
|
|
public const string InputLatinLowercase = "\U0001F521";
|
|
|
|
/// <summary>
|
|
/// Gets the "input_latin_uppercase" emoji.
|
|
/// Description: Input latin uppercase.
|
|
/// </summary>
|
|
public const string InputLatinUppercase = "\U0001F520";
|
|
|
|
/// <summary>
|
|
/// Gets the "input_numbers" emoji.
|
|
/// Description: Input numbers.
|
|
/// </summary>
|
|
public const string InputNumbers = "\U0001F522";
|
|
|
|
/// <summary>
|
|
/// Gets the "input_symbols" emoji.
|
|
/// Description: Input symbols.
|
|
/// </summary>
|
|
public const string InputSymbols = "\U0001F523";
|
|
|
|
/// <summary>
|
|
/// Gets the "jack_o_lantern" emoji.
|
|
/// Description: jack o lantern.
|
|
/// </summary>
|
|
public const string JackOLantern = "\U0001F383";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_acceptable_button" emoji.
|
|
/// Description: Japanese acceptable button.
|
|
/// </summary>
|
|
public const string JapaneseAcceptableButton = "\U0001F251";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_application_button" emoji.
|
|
/// Description: Japanese application button.
|
|
/// </summary>
|
|
public const string JapaneseApplicationButton = "\U0001F238";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_bargain_button" emoji.
|
|
/// Description: Japanese bargain button.
|
|
/// </summary>
|
|
public const string JapaneseBargainButton = "\U0001F250";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_castle" emoji.
|
|
/// Description: Japanese castle.
|
|
/// </summary>
|
|
public const string JapaneseCastle = "\U0001F3EF";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_congratulations_button" emoji.
|
|
/// Description: Japanese congratulations button.
|
|
/// </summary>
|
|
public const string JapaneseCongratulationsButton = "\U00003297";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_discount_button" emoji.
|
|
/// Description: Japanese discount button.
|
|
/// </summary>
|
|
public const string JapaneseDiscountButton = "\U0001F239";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_dolls" emoji.
|
|
/// Description: Japanese dolls.
|
|
/// </summary>
|
|
public const string JapaneseDolls = "\U0001F38E";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_free_of_charge_button" emoji.
|
|
/// Description: Japanese free of charge button.
|
|
/// </summary>
|
|
public const string JapaneseFreeOfChargeButton = "\U0001F21A";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_here_button" emoji.
|
|
/// Description: Japanese here button.
|
|
/// </summary>
|
|
public const string JapaneseHereButton = "\U0001F201";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_monthly_amount_button" emoji.
|
|
/// Description: Japanese monthly amount button.
|
|
/// </summary>
|
|
public const string JapaneseMonthlyAmountButton = "\U0001F237";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_not_free_of_charge_button" emoji.
|
|
/// Description: Japanese not free of charge button.
|
|
/// </summary>
|
|
public const string JapaneseNotFreeOfChargeButton = "\U0001F236";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_no_vacancy_button" emoji.
|
|
/// Description: Japanese no vacancy button.
|
|
/// </summary>
|
|
public const string JapaneseNoVacancyButton = "\U0001F235";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_open_for_business_button" emoji.
|
|
/// Description: Japanese open for business button.
|
|
/// </summary>
|
|
public const string JapaneseOpenForBusinessButton = "\U0001F23A";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_passing_grade_button" emoji.
|
|
/// Description: Japanese passing grade button.
|
|
/// </summary>
|
|
public const string JapanesePassingGradeButton = "\U0001F234";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_post_office" emoji.
|
|
/// Description: Japanese post office.
|
|
/// </summary>
|
|
public const string JapanesePostOffice = "\U0001F3E3";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_prohibited_button" emoji.
|
|
/// Description: Japanese prohibited button.
|
|
/// </summary>
|
|
public const string JapaneseProhibitedButton = "\U0001F232";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_reserved_button" emoji.
|
|
/// Description: Japanese reserved button.
|
|
/// </summary>
|
|
public const string JapaneseReservedButton = "\U0001F22F";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_secret_button" emoji.
|
|
/// Description: Japanese secret button.
|
|
/// </summary>
|
|
public const string JapaneseSecretButton = "\U00003299";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_service_charge_button" emoji.
|
|
/// Description: Japanese service charge button.
|
|
/// </summary>
|
|
public const string JapaneseServiceChargeButton = "\U0001F202";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_symbol_for_beginner" emoji.
|
|
/// Description: Japanese symbol for beginner.
|
|
/// </summary>
|
|
public const string JapaneseSymbolForBeginner = "\U0001F530";
|
|
|
|
/// <summary>
|
|
/// Gets the "japanese_vacancy_button" emoji.
|
|
/// Description: Japanese vacancy button.
|
|
/// </summary>
|
|
public const string JapaneseVacancyButton = "\U0001F233";
|
|
|
|
/// <summary>
|
|
/// Gets the "jeans" emoji.
|
|
/// Description: Jeans.
|
|
/// </summary>
|
|
public const string Jeans = "\U0001F456";
|
|
|
|
/// <summary>
|
|
/// Gets the "joker" emoji.
|
|
/// Description: Joker.
|
|
/// </summary>
|
|
public const string Joker = "\U0001F0CF";
|
|
|
|
/// <summary>
|
|
/// Gets the "joystick" emoji.
|
|
/// Description: Joystick.
|
|
/// </summary>
|
|
public const string Joystick = "\U0001F579";
|
|
|
|
/// <summary>
|
|
/// Gets the "kaaba" emoji.
|
|
/// Description: Kaaba.
|
|
/// </summary>
|
|
public const string Kaaba = "\U0001F54B";
|
|
|
|
/// <summary>
|
|
/// Gets the "kangaroo" emoji.
|
|
/// Description: Kangaroo.
|
|
/// </summary>
|
|
public const string Kangaroo = "\U0001F998";
|
|
|
|
/// <summary>
|
|
/// Gets the "key" emoji.
|
|
/// Description: Key.
|
|
/// </summary>
|
|
public const string Key = "\U0001F511";
|
|
|
|
/// <summary>
|
|
/// Gets the "keyboard" emoji.
|
|
/// Description: Keyboard.
|
|
/// </summary>
|
|
public const string Keyboard = "\U00002328";
|
|
|
|
/// <summary>
|
|
/// Gets the "keycap_10" emoji.
|
|
/// Description: Keycap 10.
|
|
/// </summary>
|
|
public const string Keycap10 = "\U0001F51F";
|
|
|
|
/// <summary>
|
|
/// Gets the "kick_scooter" emoji.
|
|
/// Description: Kick scooter.
|
|
/// </summary>
|
|
public const string KickScooter = "\U0001F6F4";
|
|
|
|
/// <summary>
|
|
/// Gets the "kimono" emoji.
|
|
/// Description: Kimono.
|
|
/// </summary>
|
|
public const string Kimono = "\U0001F458";
|
|
|
|
/// <summary>
|
|
/// Gets the "kiss" emoji.
|
|
/// Description: Kiss.
|
|
/// </summary>
|
|
public const string Kiss = "\U0001F48F";
|
|
|
|
/// <summary>
|
|
/// Gets the "kissing_cat" emoji.
|
|
/// Description: Kissing cat.
|
|
/// </summary>
|
|
public const string KissingCat = "\U0001F63D";
|
|
|
|
/// <summary>
|
|
/// Gets the "kissing_face" emoji.
|
|
/// Description: Kissing face.
|
|
/// </summary>
|
|
public const string KissingFace = "\U0001F617";
|
|
|
|
/// <summary>
|
|
/// Gets the "kissing_face_with_closed_eyes" emoji.
|
|
/// Description: Kissing face with closed eyes.
|
|
/// </summary>
|
|
public const string KissingFaceWithClosedEyes = "\U0001F61A";
|
|
|
|
/// <summary>
|
|
/// Gets the "kissing_face_with_smiling_eyes" emoji.
|
|
/// Description: Kissing face with smiling eyes.
|
|
/// </summary>
|
|
public const string KissingFaceWithSmilingEyes = "\U0001F619";
|
|
|
|
/// <summary>
|
|
/// Gets the "kiss_mark" emoji.
|
|
/// Description: Kiss mark.
|
|
/// </summary>
|
|
public const string KissMark = "\U0001F48B";
|
|
|
|
/// <summary>
|
|
/// Gets the "kitchen_knife" emoji.
|
|
/// Description: Kitchen knife.
|
|
/// </summary>
|
|
public const string KitchenKnife = "\U0001F52A";
|
|
|
|
/// <summary>
|
|
/// Gets the "kite" emoji.
|
|
/// Description: Kite.
|
|
/// </summary>
|
|
public const string Kite = "\U0001FA81";
|
|
|
|
/// <summary>
|
|
/// Gets the "kiwi_fruit" emoji.
|
|
/// Description: Kiwi fruit.
|
|
/// </summary>
|
|
public const string KiwiFruit = "\U0001F95D";
|
|
|
|
/// <summary>
|
|
/// Gets the "knocked_out_face" emoji.
|
|
/// Description: knocked out face.
|
|
/// </summary>
|
|
public const string KnockedOutFace = "\U0001F635";
|
|
|
|
/// <summary>
|
|
/// Gets the "knot" emoji.
|
|
/// Description: Knot.
|
|
/// </summary>
|
|
public const string Knot = "\U0001FAA2";
|
|
|
|
/// <summary>
|
|
/// Gets the "koala" emoji.
|
|
/// Description: Koala.
|
|
/// </summary>
|
|
public const string Koala = "\U0001F428";
|
|
|
|
/// <summary>
|
|
/// Gets the "lab_coat" emoji.
|
|
/// Description: Lab coat.
|
|
/// </summary>
|
|
public const string LabCoat = "\U0001F97C";
|
|
|
|
/// <summary>
|
|
/// Gets the "label" emoji.
|
|
/// Description: Label.
|
|
/// </summary>
|
|
public const string Label = "\U0001F3F7";
|
|
|
|
/// <summary>
|
|
/// Gets the "lacrosse" emoji.
|
|
/// Description: Lacrosse.
|
|
/// </summary>
|
|
public const string Lacrosse = "\U0001F94D";
|
|
|
|
/// <summary>
|
|
/// Gets the "ladder" emoji.
|
|
/// Description: Ladder.
|
|
/// </summary>
|
|
public const string Ladder = "\U0001FA9C";
|
|
|
|
/// <summary>
|
|
/// Gets the "lady_beetle" emoji.
|
|
/// Description: Lady beetle.
|
|
/// </summary>
|
|
public const string LadyBeetle = "\U0001F41E";
|
|
|
|
/// <summary>
|
|
/// Gets the "laptop" emoji.
|
|
/// Description: Laptop.
|
|
/// </summary>
|
|
public const string Laptop = "\U0001F4BB";
|
|
|
|
/// <summary>
|
|
/// Gets the "large_blue_diamond" emoji.
|
|
/// Description: Large blue diamond.
|
|
/// </summary>
|
|
public const string LargeBlueDiamond = "\U0001F537";
|
|
|
|
/// <summary>
|
|
/// Gets the "large_orange_diamond" emoji.
|
|
/// Description: Large orange diamond.
|
|
/// </summary>
|
|
public const string LargeOrangeDiamond = "\U0001F536";
|
|
|
|
/// <summary>
|
|
/// Gets the "last_quarter_moon" emoji.
|
|
/// Description: Last quarter moon.
|
|
/// </summary>
|
|
public const string LastQuarterMoon = "\U0001F317";
|
|
|
|
/// <summary>
|
|
/// Gets the "last_quarter_moon_face" emoji.
|
|
/// Description: Last quarter moon face.
|
|
/// </summary>
|
|
public const string LastQuarterMoonFace = "\U0001F31C";
|
|
|
|
/// <summary>
|
|
/// Gets the "last_track_button" emoji.
|
|
/// Description: Last track button.
|
|
/// </summary>
|
|
public const string LastTrackButton = "\U000023EE";
|
|
|
|
/// <summary>
|
|
/// Gets the "latin_cross" emoji.
|
|
/// Description: Latin cross.
|
|
/// </summary>
|
|
public const string LatinCross = "\U0000271D";
|
|
|
|
/// <summary>
|
|
/// Gets the "leaf_fluttering_in_wind" emoji.
|
|
/// Description: Leaf fluttering in wind.
|
|
/// </summary>
|
|
public const string LeafFlutteringInWind = "\U0001F343";
|
|
|
|
/// <summary>
|
|
/// Gets the "leafy_green" emoji.
|
|
/// Description: Leafy green.
|
|
/// </summary>
|
|
public const string LeafyGreen = "\U0001F96C";
|
|
|
|
/// <summary>
|
|
/// Gets the "ledger" emoji.
|
|
/// Description: Ledger.
|
|
/// </summary>
|
|
public const string Ledger = "\U0001F4D2";
|
|
|
|
/// <summary>
|
|
/// Gets the "left_arrow" emoji.
|
|
/// Description: Left arrow.
|
|
/// </summary>
|
|
public const string LeftArrow = "\U00002B05";
|
|
|
|
/// <summary>
|
|
/// Gets the "left_arrow_curving_right" emoji.
|
|
/// Description: Left arrow curving right.
|
|
/// </summary>
|
|
public const string LeftArrowCurvingRight = "\U000021AA";
|
|
|
|
/// <summary>
|
|
/// Gets the "left_facing_fist" emoji.
|
|
/// Description: left facing fist.
|
|
/// </summary>
|
|
public const string LeftFacingFist = "\U0001F91B";
|
|
|
|
/// <summary>
|
|
/// Gets the "left_luggage" emoji.
|
|
/// Description: Left luggage.
|
|
/// </summary>
|
|
public const string LeftLuggage = "\U0001F6C5";
|
|
|
|
/// <summary>
|
|
/// Gets the "left_right_arrow" emoji.
|
|
/// Description: left right arrow.
|
|
/// </summary>
|
|
public const string LeftRightArrow = "\U00002194";
|
|
|
|
/// <summary>
|
|
/// Gets the "left_speech_bubble" emoji.
|
|
/// Description: Left speech bubble.
|
|
/// </summary>
|
|
public const string LeftSpeechBubble = "\U0001F5E8";
|
|
|
|
/// <summary>
|
|
/// Gets the "leg" emoji.
|
|
/// Description: Leg.
|
|
/// </summary>
|
|
public const string Leg = "\U0001F9B5";
|
|
|
|
/// <summary>
|
|
/// Gets the "lemon" emoji.
|
|
/// Description: Lemon.
|
|
/// </summary>
|
|
public const string Lemon = "\U0001F34B";
|
|
|
|
/// <summary>
|
|
/// Gets the "leo" emoji.
|
|
/// Description: Leo.
|
|
/// </summary>
|
|
public const string Leo = "\U0000264C";
|
|
|
|
/// <summary>
|
|
/// Gets the "leopard" emoji.
|
|
/// Description: Leopard.
|
|
/// </summary>
|
|
public const string Leopard = "\U0001F406";
|
|
|
|
/// <summary>
|
|
/// Gets the "level_slider" emoji.
|
|
/// Description: Level slider.
|
|
/// </summary>
|
|
public const string LevelSlider = "\U0001F39A";
|
|
|
|
/// <summary>
|
|
/// Gets the "libra" emoji.
|
|
/// Description: Libra.
|
|
/// </summary>
|
|
public const string Libra = "\U0000264E";
|
|
|
|
/// <summary>
|
|
/// Gets the "light_bulb" emoji.
|
|
/// Description: Light bulb.
|
|
/// </summary>
|
|
public const string LightBulb = "\U0001F4A1";
|
|
|
|
/// <summary>
|
|
/// Gets the "light_rail" emoji.
|
|
/// Description: Light rail.
|
|
/// </summary>
|
|
public const string LightRail = "\U0001F688";
|
|
|
|
/// <summary>
|
|
/// Gets the "link" emoji.
|
|
/// Description: Link.
|
|
/// </summary>
|
|
public const string Link = "\U0001F517";
|
|
|
|
/// <summary>
|
|
/// Gets the "linked_paperclips" emoji.
|
|
/// Description: Linked paperclips.
|
|
/// </summary>
|
|
public const string LinkedPaperclips = "\U0001F587";
|
|
|
|
/// <summary>
|
|
/// Gets the "lion" emoji.
|
|
/// Description: Lion.
|
|
/// </summary>
|
|
public const string Lion = "\U0001F981";
|
|
|
|
/// <summary>
|
|
/// Gets the "lipstick" emoji.
|
|
/// Description: Lipstick.
|
|
/// </summary>
|
|
public const string Lipstick = "\U0001F484";
|
|
|
|
/// <summary>
|
|
/// Gets the "litter_in_bin_sign" emoji.
|
|
/// Description: Litter in bin sign.
|
|
/// </summary>
|
|
public const string LitterInBinSign = "\U0001F6AE";
|
|
|
|
/// <summary>
|
|
/// Gets the "lizard" emoji.
|
|
/// Description: Lizard.
|
|
/// </summary>
|
|
public const string Lizard = "\U0001F98E";
|
|
|
|
/// <summary>
|
|
/// Gets the "llama" emoji.
|
|
/// Description: Llama.
|
|
/// </summary>
|
|
public const string Llama = "\U0001F999";
|
|
|
|
/// <summary>
|
|
/// Gets the "lobster" emoji.
|
|
/// Description: Lobster.
|
|
/// </summary>
|
|
public const string Lobster = "\U0001F99E";
|
|
|
|
/// <summary>
|
|
/// Gets the "locked" emoji.
|
|
/// Description: Locked.
|
|
/// </summary>
|
|
public const string Locked = "\U0001F512";
|
|
|
|
/// <summary>
|
|
/// Gets the "locked_with_key" emoji.
|
|
/// Description: Locked with key.
|
|
/// </summary>
|
|
public const string LockedWithKey = "\U0001F510";
|
|
|
|
/// <summary>
|
|
/// Gets the "locked_with_pen" emoji.
|
|
/// Description: Locked with pen.
|
|
/// </summary>
|
|
public const string LockedWithPen = "\U0001F50F";
|
|
|
|
/// <summary>
|
|
/// Gets the "locomotive" emoji.
|
|
/// Description: Locomotive.
|
|
/// </summary>
|
|
public const string Locomotive = "\U0001F682";
|
|
|
|
/// <summary>
|
|
/// Gets the "lollipop" emoji.
|
|
/// Description: Lollipop.
|
|
/// </summary>
|
|
public const string Lollipop = "\U0001F36D";
|
|
|
|
/// <summary>
|
|
/// Gets the "long_drum" emoji.
|
|
/// Description: Long drum.
|
|
/// </summary>
|
|
public const string LongDrum = "\U0001FA98";
|
|
|
|
/// <summary>
|
|
/// Gets the "lotion_bottle" emoji.
|
|
/// Description: Lotion bottle.
|
|
/// </summary>
|
|
public const string LotionBottle = "\U0001F9F4";
|
|
|
|
/// <summary>
|
|
/// Gets the "loudly_crying_face" emoji.
|
|
/// Description: Loudly crying face.
|
|
/// </summary>
|
|
public const string LoudlyCryingFace = "\U0001F62D";
|
|
|
|
/// <summary>
|
|
/// Gets the "loudspeaker" emoji.
|
|
/// Description: Loudspeaker.
|
|
/// </summary>
|
|
public const string Loudspeaker = "\U0001F4E2";
|
|
|
|
/// <summary>
|
|
/// Gets the "love_hotel" emoji.
|
|
/// Description: Love hotel.
|
|
/// </summary>
|
|
public const string LoveHotel = "\U0001F3E9";
|
|
|
|
/// <summary>
|
|
/// Gets the "love_letter" emoji.
|
|
/// Description: Love letter.
|
|
/// </summary>
|
|
public const string LoveLetter = "\U0001F48C";
|
|
|
|
/// <summary>
|
|
/// Gets the "love_you_gesture" emoji.
|
|
/// Description: love you gesture.
|
|
/// </summary>
|
|
public const string LoveYouGesture = "\U0001F91F";
|
|
|
|
/// <summary>
|
|
/// Gets the "luggage" emoji.
|
|
/// Description: Luggage.
|
|
/// </summary>
|
|
public const string Luggage = "\U0001F9F3";
|
|
|
|
/// <summary>
|
|
/// Gets the "lungs" emoji.
|
|
/// Description: Lungs.
|
|
/// </summary>
|
|
public const string Lungs = "\U0001FAC1";
|
|
|
|
/// <summary>
|
|
/// Gets the "lying_face" emoji.
|
|
/// Description: Lying face.
|
|
/// </summary>
|
|
public const string LyingFace = "\U0001F925";
|
|
|
|
/// <summary>
|
|
/// Gets the "mage" emoji.
|
|
/// Description: Mage.
|
|
/// </summary>
|
|
public const string Mage = "\U0001F9D9";
|
|
|
|
/// <summary>
|
|
/// Gets the "magic_wand" emoji.
|
|
/// Description: Magic wand.
|
|
/// </summary>
|
|
public const string MagicWand = "\U0001FA84";
|
|
|
|
/// <summary>
|
|
/// Gets the "magnet" emoji.
|
|
/// Description: Magnet.
|
|
/// </summary>
|
|
public const string Magnet = "\U0001F9F2";
|
|
|
|
/// <summary>
|
|
/// Gets the "magnifying_glass_tilted_left" emoji.
|
|
/// Description: Magnifying glass tilted left.
|
|
/// </summary>
|
|
public const string MagnifyingGlassTiltedLeft = "\U0001F50D";
|
|
|
|
/// <summary>
|
|
/// Gets the "magnifying_glass_tilted_right" emoji.
|
|
/// Description: Magnifying glass tilted right.
|
|
/// </summary>
|
|
public const string MagnifyingGlassTiltedRight = "\U0001F50E";
|
|
|
|
/// <summary>
|
|
/// Gets the "mahjong_red_dragon" emoji.
|
|
/// Description: Mahjong red dragon.
|
|
/// </summary>
|
|
public const string MahjongRedDragon = "\U0001F004";
|
|
|
|
/// <summary>
|
|
/// Gets the "male_sign" emoji.
|
|
/// Description: Male sign.
|
|
/// </summary>
|
|
public const string MaleSign = "\U00002642";
|
|
|
|
/// <summary>
|
|
/// Gets the "mammoth" emoji.
|
|
/// Description: Mammoth.
|
|
/// </summary>
|
|
public const string Mammoth = "\U0001F9A3";
|
|
|
|
/// <summary>
|
|
/// Gets the "man" emoji.
|
|
/// Description: Man.
|
|
/// </summary>
|
|
public const string Man = "\U0001F468";
|
|
|
|
/// <summary>
|
|
/// Gets the "man_dancing" emoji.
|
|
/// Description: Man dancing.
|
|
/// </summary>
|
|
public const string ManDancing = "\U0001F57A";
|
|
|
|
/// <summary>
|
|
/// Gets the "mango" emoji.
|
|
/// Description: Mango.
|
|
/// </summary>
|
|
public const string Mango = "\U0001F96D";
|
|
|
|
/// <summary>
|
|
/// Gets the "mans_shoe" emoji.
|
|
/// Description: Man s shoe.
|
|
/// </summary>
|
|
public const string MansShoe = "\U0001F45E";
|
|
|
|
/// <summary>
|
|
/// Gets the "mantelpiece_clock" emoji.
|
|
/// Description: Mantelpiece clock.
|
|
/// </summary>
|
|
public const string MantelpieceClock = "\U0001F570";
|
|
|
|
/// <summary>
|
|
/// Gets the "manual_wheelchair" emoji.
|
|
/// Description: Manual wheelchair.
|
|
/// </summary>
|
|
public const string ManualWheelchair = "\U0001F9BD";
|
|
|
|
/// <summary>
|
|
/// Gets the "maple_leaf" emoji.
|
|
/// Description: Maple leaf.
|
|
/// </summary>
|
|
public const string MapleLeaf = "\U0001F341";
|
|
|
|
/// <summary>
|
|
/// Gets the "map_of_japan" emoji.
|
|
/// Description: Map of japan.
|
|
/// </summary>
|
|
public const string MapOfJapan = "\U0001F5FE";
|
|
|
|
/// <summary>
|
|
/// Gets the "martial_arts_uniform" emoji.
|
|
/// Description: Martial arts uniform.
|
|
/// </summary>
|
|
public const string MartialArtsUniform = "\U0001F94B";
|
|
|
|
/// <summary>
|
|
/// Gets the "mate" emoji.
|
|
/// Description: Mate.
|
|
/// </summary>
|
|
public const string Mate = "\U0001F9C9";
|
|
|
|
/// <summary>
|
|
/// Gets the "meat_on_bone" emoji.
|
|
/// Description: Meat on bone.
|
|
/// </summary>
|
|
public const string MeatOnBone = "\U0001F356";
|
|
|
|
/// <summary>
|
|
/// Gets the "mechanical_arm" emoji.
|
|
/// Description: Mechanical arm.
|
|
/// </summary>
|
|
public const string MechanicalArm = "\U0001F9BE";
|
|
|
|
/// <summary>
|
|
/// Gets the "mechanical_leg" emoji.
|
|
/// Description: Mechanical leg.
|
|
/// </summary>
|
|
public const string MechanicalLeg = "\U0001F9BF";
|
|
|
|
/// <summary>
|
|
/// Gets the "medical_symbol" emoji.
|
|
/// Description: Medical symbol.
|
|
/// </summary>
|
|
public const string MedicalSymbol = "\U00002695";
|
|
|
|
/// <summary>
|
|
/// Gets the "megaphone" emoji.
|
|
/// Description: Megaphone.
|
|
/// </summary>
|
|
public const string Megaphone = "\U0001F4E3";
|
|
|
|
/// <summary>
|
|
/// Gets the "melon" emoji.
|
|
/// Description: Melon.
|
|
/// </summary>
|
|
public const string Melon = "\U0001F348";
|
|
|
|
/// <summary>
|
|
/// Gets the "memo" emoji.
|
|
/// Description: Memo.
|
|
/// </summary>
|
|
public const string Memo = "\U0001F4DD";
|
|
|
|
/// <summary>
|
|
/// Gets the "men_holding_hands" emoji.
|
|
/// Description: Men holding hands.
|
|
/// </summary>
|
|
public const string MenHoldingHands = "\U0001F46C";
|
|
|
|
/// <summary>
|
|
/// Gets the "menorah" emoji.
|
|
/// Description: Menorah.
|
|
/// </summary>
|
|
public const string Menorah = "\U0001F54E";
|
|
|
|
/// <summary>
|
|
/// Gets the "mens_room" emoji.
|
|
/// Description: Men s room.
|
|
/// </summary>
|
|
public const string MensRoom = "\U0001F6B9";
|
|
|
|
/// <summary>
|
|
/// Gets the "merperson" emoji.
|
|
/// Description: Merperson.
|
|
/// </summary>
|
|
public const string Merperson = "\U0001F9DC";
|
|
|
|
/// <summary>
|
|
/// Gets the "metro" emoji.
|
|
/// Description: Metro.
|
|
/// </summary>
|
|
public const string Metro = "\U0001F687";
|
|
|
|
/// <summary>
|
|
/// Gets the "microbe" emoji.
|
|
/// Description: Microbe.
|
|
/// </summary>
|
|
public const string Microbe = "\U0001F9A0";
|
|
|
|
/// <summary>
|
|
/// Gets the "microphone" emoji.
|
|
/// Description: Microphone.
|
|
/// </summary>
|
|
public const string Microphone = "\U0001F3A4";
|
|
|
|
/// <summary>
|
|
/// Gets the "microscope" emoji.
|
|
/// Description: Microscope.
|
|
/// </summary>
|
|
public const string Microscope = "\U0001F52C";
|
|
|
|
/// <summary>
|
|
/// Gets the "middle_finger" emoji.
|
|
/// Description: Middle finger.
|
|
/// </summary>
|
|
public const string MiddleFinger = "\U0001F595";
|
|
|
|
/// <summary>
|
|
/// Gets the "military_helmet" emoji.
|
|
/// Description: Military helmet.
|
|
/// </summary>
|
|
public const string MilitaryHelmet = "\U0001FA96";
|
|
|
|
/// <summary>
|
|
/// Gets the "military_medal" emoji.
|
|
/// Description: Military medal.
|
|
/// </summary>
|
|
public const string MilitaryMedal = "\U0001F396";
|
|
|
|
/// <summary>
|
|
/// Gets the "milky_way" emoji.
|
|
/// Description: Milky way.
|
|
/// </summary>
|
|
public const string MilkyWay = "\U0001F30C";
|
|
|
|
/// <summary>
|
|
/// Gets the "minibus" emoji.
|
|
/// Description: Minibus.
|
|
/// </summary>
|
|
public const string Minibus = "\U0001F690";
|
|
|
|
/// <summary>
|
|
/// Gets the "minus" emoji.
|
|
/// Description: Minus.
|
|
/// </summary>
|
|
public const string Minus = "\U00002796";
|
|
|
|
/// <summary>
|
|
/// Gets the "mirror" emoji.
|
|
/// Description: Mirror.
|
|
/// </summary>
|
|
public const string Mirror = "\U0001FA9E";
|
|
|
|
/// <summary>
|
|
/// Gets the "moai" emoji.
|
|
/// Description: Moai.
|
|
/// </summary>
|
|
public const string Moai = "\U0001F5FF";
|
|
|
|
/// <summary>
|
|
/// Gets the "mobile_phone" emoji.
|
|
/// Description: Mobile phone.
|
|
/// </summary>
|
|
public const string MobilePhone = "\U0001F4F1";
|
|
|
|
/// <summary>
|
|
/// Gets the "mobile_phone_off" emoji.
|
|
/// Description: Mobile phone off.
|
|
/// </summary>
|
|
public const string MobilePhoneOff = "\U0001F4F4";
|
|
|
|
/// <summary>
|
|
/// Gets the "mobile_phone_with_arrow" emoji.
|
|
/// Description: Mobile phone with arrow.
|
|
/// </summary>
|
|
public const string MobilePhoneWithArrow = "\U0001F4F2";
|
|
|
|
/// <summary>
|
|
/// Gets the "money_bag" emoji.
|
|
/// Description: Money bag.
|
|
/// </summary>
|
|
public const string MoneyBag = "\U0001F4B0";
|
|
|
|
/// <summary>
|
|
/// Gets the "money_mouth_face" emoji.
|
|
/// Description: money mouth face.
|
|
/// </summary>
|
|
public const string MoneyMouthFace = "\U0001F911";
|
|
|
|
/// <summary>
|
|
/// Gets the "money_with_wings" emoji.
|
|
/// Description: Money with wings.
|
|
/// </summary>
|
|
public const string MoneyWithWings = "\U0001F4B8";
|
|
|
|
/// <summary>
|
|
/// Gets the "monkey" emoji.
|
|
/// Description: Monkey.
|
|
/// </summary>
|
|
public const string Monkey = "\U0001F412";
|
|
|
|
/// <summary>
|
|
/// Gets the "monkey_face" emoji.
|
|
/// Description: Monkey face.
|
|
/// </summary>
|
|
public const string MonkeyFace = "\U0001F435";
|
|
|
|
/// <summary>
|
|
/// Gets the "monorail" emoji.
|
|
/// Description: Monorail.
|
|
/// </summary>
|
|
public const string Monorail = "\U0001F69D";
|
|
|
|
/// <summary>
|
|
/// Gets the "moon_cake" emoji.
|
|
/// Description: Moon cake.
|
|
/// </summary>
|
|
public const string MoonCake = "\U0001F96E";
|
|
|
|
/// <summary>
|
|
/// Gets the "moon_viewing_ceremony" emoji.
|
|
/// Description: Moon viewing ceremony.
|
|
/// </summary>
|
|
public const string MoonViewingCeremony = "\U0001F391";
|
|
|
|
/// <summary>
|
|
/// Gets the "mosque" emoji.
|
|
/// Description: Mosque.
|
|
/// </summary>
|
|
public const string Mosque = "\U0001F54C";
|
|
|
|
/// <summary>
|
|
/// Gets the "mosquito" emoji.
|
|
/// Description: Mosquito.
|
|
/// </summary>
|
|
public const string Mosquito = "\U0001F99F";
|
|
|
|
/// <summary>
|
|
/// Gets the "motor_boat" emoji.
|
|
/// Description: Motor boat.
|
|
/// </summary>
|
|
public const string MotorBoat = "\U0001F6E5";
|
|
|
|
/// <summary>
|
|
/// Gets the "motorcycle" emoji.
|
|
/// Description: Motorcycle.
|
|
/// </summary>
|
|
public const string Motorcycle = "\U0001F3CD";
|
|
|
|
/// <summary>
|
|
/// Gets the "motorized_wheelchair" emoji.
|
|
/// Description: Motorized wheelchair.
|
|
/// </summary>
|
|
public const string MotorizedWheelchair = "\U0001F9BC";
|
|
|
|
/// <summary>
|
|
/// Gets the "motor_scooter" emoji.
|
|
/// Description: Motor scooter.
|
|
/// </summary>
|
|
public const string MotorScooter = "\U0001F6F5";
|
|
|
|
/// <summary>
|
|
/// Gets the "motorway" emoji.
|
|
/// Description: Motorway.
|
|
/// </summary>
|
|
public const string Motorway = "\U0001F6E3";
|
|
|
|
/// <summary>
|
|
/// Gets the "mountain" emoji.
|
|
/// Description: Mountain.
|
|
/// </summary>
|
|
public const string Mountain = "\U000026F0";
|
|
|
|
/// <summary>
|
|
/// Gets the "mountain_cableway" emoji.
|
|
/// Description: Mountain cableway.
|
|
/// </summary>
|
|
public const string MountainCableway = "\U0001F6A0";
|
|
|
|
/// <summary>
|
|
/// Gets the "mountain_railway" emoji.
|
|
/// Description: Mountain railway.
|
|
/// </summary>
|
|
public const string MountainRailway = "\U0001F69E";
|
|
|
|
/// <summary>
|
|
/// Gets the "mount_fuji" emoji.
|
|
/// Description: Mount fuji.
|
|
/// </summary>
|
|
public const string MountFuji = "\U0001F5FB";
|
|
|
|
/// <summary>
|
|
/// Gets the "mouse" emoji.
|
|
/// Description: Mouse.
|
|
/// </summary>
|
|
public const string Mouse = "\U0001F401";
|
|
|
|
/// <summary>
|
|
/// Gets the "mouse_face" emoji.
|
|
/// Description: Mouse face.
|
|
/// </summary>
|
|
public const string MouseFace = "\U0001F42D";
|
|
|
|
/// <summary>
|
|
/// Gets the "mouse_trap" emoji.
|
|
/// Description: Mouse trap.
|
|
/// </summary>
|
|
public const string MouseTrap = "\U0001FAA4";
|
|
|
|
/// <summary>
|
|
/// Gets the "mouth" emoji.
|
|
/// Description: Mouth.
|
|
/// </summary>
|
|
public const string Mouth = "\U0001F444";
|
|
|
|
/// <summary>
|
|
/// Gets the "movie_camera" emoji.
|
|
/// Description: Movie camera.
|
|
/// </summary>
|
|
public const string MovieCamera = "\U0001F3A5";
|
|
|
|
/// <summary>
|
|
/// Gets the "mrs_claus" emoji.
|
|
/// Description: Mrs claus.
|
|
/// </summary>
|
|
public const string MrsClaus = "\U0001F936";
|
|
|
|
/// <summary>
|
|
/// Gets the "multiply" emoji.
|
|
/// Description: Multiply.
|
|
/// </summary>
|
|
public const string Multiply = "\U00002716";
|
|
|
|
/// <summary>
|
|
/// Gets the "mushroom" emoji.
|
|
/// Description: Mushroom.
|
|
/// </summary>
|
|
public const string Mushroom = "\U0001F344";
|
|
|
|
/// <summary>
|
|
/// Gets the "musical_keyboard" emoji.
|
|
/// Description: Musical keyboard.
|
|
/// </summary>
|
|
public const string MusicalKeyboard = "\U0001F3B9";
|
|
|
|
/// <summary>
|
|
/// Gets the "musical_note" emoji.
|
|
/// Description: Musical note.
|
|
/// </summary>
|
|
public const string MusicalNote = "\U0001F3B5";
|
|
|
|
/// <summary>
|
|
/// Gets the "musical_notes" emoji.
|
|
/// Description: Musical notes.
|
|
/// </summary>
|
|
public const string MusicalNotes = "\U0001F3B6";
|
|
|
|
/// <summary>
|
|
/// Gets the "musical_score" emoji.
|
|
/// Description: Musical score.
|
|
/// </summary>
|
|
public const string MusicalScore = "\U0001F3BC";
|
|
|
|
/// <summary>
|
|
/// Gets the "muted_speaker" emoji.
|
|
/// Description: Muted speaker.
|
|
/// </summary>
|
|
public const string MutedSpeaker = "\U0001F507";
|
|
|
|
/// <summary>
|
|
/// Gets the "nail_polish" emoji.
|
|
/// Description: Nail polish.
|
|
/// </summary>
|
|
public const string NailPolish = "\U0001F485";
|
|
|
|
/// <summary>
|
|
/// Gets the "name_badge" emoji.
|
|
/// Description: Name badge.
|
|
/// </summary>
|
|
public const string NameBadge = "\U0001F4DB";
|
|
|
|
/// <summary>
|
|
/// Gets the "national_park" emoji.
|
|
/// Description: National park.
|
|
/// </summary>
|
|
public const string NationalPark = "\U0001F3DE";
|
|
|
|
/// <summary>
|
|
/// Gets the "nauseated_face" emoji.
|
|
/// Description: Nauseated face.
|
|
/// </summary>
|
|
public const string NauseatedFace = "\U0001F922";
|
|
|
|
/// <summary>
|
|
/// Gets the "nazar_amulet" emoji.
|
|
/// Description: Nazar amulet.
|
|
/// </summary>
|
|
public const string NazarAmulet = "\U0001F9FF";
|
|
|
|
/// <summary>
|
|
/// Gets the "necktie" emoji.
|
|
/// Description: Necktie.
|
|
/// </summary>
|
|
public const string Necktie = "\U0001F454";
|
|
|
|
/// <summary>
|
|
/// Gets the "nerd_face" emoji.
|
|
/// Description: Nerd face.
|
|
/// </summary>
|
|
public const string NerdFace = "\U0001F913";
|
|
|
|
/// <summary>
|
|
/// Gets the "nesting_dolls" emoji.
|
|
/// Description: Nesting dolls.
|
|
/// </summary>
|
|
public const string NestingDolls = "\U0001FA86";
|
|
|
|
/// <summary>
|
|
/// Gets the "neutral_face" emoji.
|
|
/// Description: Neutral face.
|
|
/// </summary>
|
|
public const string NeutralFace = "\U0001F610";
|
|
|
|
/// <summary>
|
|
/// Gets the "new_button" emoji.
|
|
/// Description: NEW button.
|
|
/// </summary>
|
|
public const string NewButton = "\U0001F195";
|
|
|
|
/// <summary>
|
|
/// Gets the "new_moon" emoji.
|
|
/// Description: New moon.
|
|
/// </summary>
|
|
public const string NewMoon = "\U0001F311";
|
|
|
|
/// <summary>
|
|
/// Gets the "new_moon_face" emoji.
|
|
/// Description: New moon face.
|
|
/// </summary>
|
|
public const string NewMoonFace = "\U0001F31A";
|
|
|
|
/// <summary>
|
|
/// Gets the "newspaper" emoji.
|
|
/// Description: Newspaper.
|
|
/// </summary>
|
|
public const string Newspaper = "\U0001F4F0";
|
|
|
|
/// <summary>
|
|
/// Gets the "next_track_button" emoji.
|
|
/// Description: Next track button.
|
|
/// </summary>
|
|
public const string NextTrackButton = "\U000023ED";
|
|
|
|
/// <summary>
|
|
/// Gets the "ng_button" emoji.
|
|
/// Description: NG button.
|
|
/// </summary>
|
|
public const string NgButton = "\U0001F196";
|
|
|
|
/// <summary>
|
|
/// Gets the "night_with_stars" emoji.
|
|
/// Description: Night with stars.
|
|
/// </summary>
|
|
public const string NightWithStars = "\U0001F303";
|
|
|
|
/// <summary>
|
|
/// Gets the "nine_o_clock" emoji.
|
|
/// Description: Nine o clock.
|
|
/// </summary>
|
|
public const string NineOClock = "\U0001F558";
|
|
|
|
/// <summary>
|
|
/// Gets the "nine_thirty" emoji.
|
|
/// Description: nine thirty.
|
|
/// </summary>
|
|
public const string NineThirty = "\U0001F564";
|
|
|
|
/// <summary>
|
|
/// Gets the "ninja" emoji.
|
|
/// Description: Ninja.
|
|
/// </summary>
|
|
public const string Ninja = "\U0001F977";
|
|
|
|
/// <summary>
|
|
/// Gets the "no_bicycles" emoji.
|
|
/// Description: No bicycles.
|
|
/// </summary>
|
|
public const string NoBicycles = "\U0001F6B3";
|
|
|
|
/// <summary>
|
|
/// Gets the "no_entry" emoji.
|
|
/// Description: No entry.
|
|
/// </summary>
|
|
public const string NoEntry = "\U000026D4";
|
|
|
|
/// <summary>
|
|
/// Gets the "no_littering" emoji.
|
|
/// Description: No littering.
|
|
/// </summary>
|
|
public const string NoLittering = "\U0001F6AF";
|
|
|
|
/// <summary>
|
|
/// Gets the "no_mobile_phones" emoji.
|
|
/// Description: No mobile phones.
|
|
/// </summary>
|
|
public const string NoMobilePhones = "\U0001F4F5";
|
|
|
|
/// <summary>
|
|
/// Gets the "non_potable_water" emoji.
|
|
/// Description: non potable water.
|
|
/// </summary>
|
|
public const string NonPotableWater = "\U0001F6B1";
|
|
|
|
/// <summary>
|
|
/// Gets the "no_one_under_eighteen" emoji.
|
|
/// Description: No one under eighteen.
|
|
/// </summary>
|
|
public const string NoOneUnderEighteen = "\U0001F51E";
|
|
|
|
/// <summary>
|
|
/// Gets the "no_pedestrians" emoji.
|
|
/// Description: No pedestrians.
|
|
/// </summary>
|
|
public const string NoPedestrians = "\U0001F6B7";
|
|
|
|
/// <summary>
|
|
/// Gets the "nose" emoji.
|
|
/// Description: Nose.
|
|
/// </summary>
|
|
public const string Nose = "\U0001F443";
|
|
|
|
/// <summary>
|
|
/// Gets the "no_smoking" emoji.
|
|
/// Description: No smoking.
|
|
/// </summary>
|
|
public const string NoSmoking = "\U0001F6AD";
|
|
|
|
/// <summary>
|
|
/// Gets the "notebook" emoji.
|
|
/// Description: Notebook.
|
|
/// </summary>
|
|
public const string Notebook = "\U0001F4D3";
|
|
|
|
/// <summary>
|
|
/// Gets the "notebook_with_decorative_cover" emoji.
|
|
/// Description: Notebook with decorative cover.
|
|
/// </summary>
|
|
public const string NotebookWithDecorativeCover = "\U0001F4D4";
|
|
|
|
/// <summary>
|
|
/// Gets the "nut_and_bolt" emoji.
|
|
/// Description: Nut and bolt.
|
|
/// </summary>
|
|
public const string NutAndBolt = "\U0001F529";
|
|
|
|
/// <summary>
|
|
/// Gets the "o_button_blood_type" emoji.
|
|
/// Description: O button blood type.
|
|
/// </summary>
|
|
public const string OButtonBloodType = "\U0001F17E";
|
|
|
|
/// <summary>
|
|
/// Gets the "octopus" emoji.
|
|
/// Description: Octopus.
|
|
/// </summary>
|
|
public const string Octopus = "\U0001F419";
|
|
|
|
/// <summary>
|
|
/// Gets the "oden" emoji.
|
|
/// Description: Oden.
|
|
/// </summary>
|
|
public const string Oden = "\U0001F362";
|
|
|
|
/// <summary>
|
|
/// Gets the "office_building" emoji.
|
|
/// Description: Office building.
|
|
/// </summary>
|
|
public const string OfficeBuilding = "\U0001F3E2";
|
|
|
|
/// <summary>
|
|
/// Gets the "ogre" emoji.
|
|
/// Description: Ogre.
|
|
/// </summary>
|
|
public const string Ogre = "\U0001F479";
|
|
|
|
/// <summary>
|
|
/// Gets the "oil_drum" emoji.
|
|
/// Description: Oil drum.
|
|
/// </summary>
|
|
public const string OilDrum = "\U0001F6E2";
|
|
|
|
/// <summary>
|
|
/// Gets the "ok_button" emoji.
|
|
/// Description: OK button.
|
|
/// </summary>
|
|
public const string OkButton = "\U0001F197";
|
|
|
|
/// <summary>
|
|
/// Gets the "ok_hand" emoji.
|
|
/// Description: OK hand.
|
|
/// </summary>
|
|
public const string OkHand = "\U0001F44C";
|
|
|
|
/// <summary>
|
|
/// Gets the "older_person" emoji.
|
|
/// Description: Older person.
|
|
/// </summary>
|
|
public const string OlderPerson = "\U0001F9D3";
|
|
|
|
/// <summary>
|
|
/// Gets the "old_key" emoji.
|
|
/// Description: Old key.
|
|
/// </summary>
|
|
public const string OldKey = "\U0001F5DD";
|
|
|
|
/// <summary>
|
|
/// Gets the "old_man" emoji.
|
|
/// Description: Old man.
|
|
/// </summary>
|
|
public const string OldMan = "\U0001F474";
|
|
|
|
/// <summary>
|
|
/// Gets the "old_woman" emoji.
|
|
/// Description: Old woman.
|
|
/// </summary>
|
|
public const string OldWoman = "\U0001F475";
|
|
|
|
/// <summary>
|
|
/// Gets the "olive" emoji.
|
|
/// Description: Olive.
|
|
/// </summary>
|
|
public const string Olive = "\U0001FAD2";
|
|
|
|
/// <summary>
|
|
/// Gets the "om" emoji.
|
|
/// Description: Om.
|
|
/// </summary>
|
|
public const string Om = "\U0001F549";
|
|
|
|
/// <summary>
|
|
/// Gets the "on_arrow" emoji.
|
|
/// Description: ON arrow.
|
|
/// </summary>
|
|
public const string OnArrow = "\U0001F51B";
|
|
|
|
/// <summary>
|
|
/// Gets the "oncoming_automobile" emoji.
|
|
/// Description: Oncoming automobile.
|
|
/// </summary>
|
|
public const string OncomingAutomobile = "\U0001F698";
|
|
|
|
/// <summary>
|
|
/// Gets the "oncoming_bus" emoji.
|
|
/// Description: Oncoming bus.
|
|
/// </summary>
|
|
public const string OncomingBus = "\U0001F68D";
|
|
|
|
/// <summary>
|
|
/// Gets the "oncoming_fist" emoji.
|
|
/// Description: Oncoming fist.
|
|
/// </summary>
|
|
public const string OncomingFist = "\U0001F44A";
|
|
|
|
/// <summary>
|
|
/// Gets the "oncoming_police_car" emoji.
|
|
/// Description: Oncoming police car.
|
|
/// </summary>
|
|
public const string OncomingPoliceCar = "\U0001F694";
|
|
|
|
/// <summary>
|
|
/// Gets the "oncoming_taxi" emoji.
|
|
/// Description: Oncoming taxi.
|
|
/// </summary>
|
|
public const string OncomingTaxi = "\U0001F696";
|
|
|
|
/// <summary>
|
|
/// Gets the "one_o_clock" emoji.
|
|
/// Description: One o clock.
|
|
/// </summary>
|
|
public const string OneOClock = "\U0001F550";
|
|
|
|
/// <summary>
|
|
/// Gets the "one_piece_swimsuit" emoji.
|
|
/// Description: one piece swimsuit.
|
|
/// </summary>
|
|
public const string OnePieceSwimsuit = "\U0001FA71";
|
|
|
|
/// <summary>
|
|
/// Gets the "one_thirty" emoji.
|
|
/// Description: one thirty.
|
|
/// </summary>
|
|
public const string OneThirty = "\U0001F55C";
|
|
|
|
/// <summary>
|
|
/// Gets the "onion" emoji.
|
|
/// Description: Onion.
|
|
/// </summary>
|
|
public const string Onion = "\U0001F9C5";
|
|
|
|
/// <summary>
|
|
/// Gets the "open_book" emoji.
|
|
/// Description: Open book.
|
|
/// </summary>
|
|
public const string OpenBook = "\U0001F4D6";
|
|
|
|
/// <summary>
|
|
/// Gets the "open_file_folder" emoji.
|
|
/// Description: Open file folder.
|
|
/// </summary>
|
|
public const string OpenFileFolder = "\U0001F4C2";
|
|
|
|
/// <summary>
|
|
/// Gets the "open_hands" emoji.
|
|
/// Description: Open hands.
|
|
/// </summary>
|
|
public const string OpenHands = "\U0001F450";
|
|
|
|
/// <summary>
|
|
/// Gets the "open_mailbox_with_lowered_flag" emoji.
|
|
/// Description: Open mailbox with lowered flag.
|
|
/// </summary>
|
|
public const string OpenMailboxWithLoweredFlag = "\U0001F4ED";
|
|
|
|
/// <summary>
|
|
/// Gets the "open_mailbox_with_raised_flag" emoji.
|
|
/// Description: Open mailbox with raised flag.
|
|
/// </summary>
|
|
public const string OpenMailboxWithRaisedFlag = "\U0001F4EC";
|
|
|
|
/// <summary>
|
|
/// Gets the "ophiuchus" emoji.
|
|
/// Description: Ophiuchus.
|
|
/// </summary>
|
|
public const string Ophiuchus = "\U000026CE";
|
|
|
|
/// <summary>
|
|
/// Gets the "optical_disk" emoji.
|
|
/// Description: Optical disk.
|
|
/// </summary>
|
|
public const string OpticalDisk = "\U0001F4BF";
|
|
|
|
/// <summary>
|
|
/// Gets the "orange_book" emoji.
|
|
/// Description: Orange book.
|
|
/// </summary>
|
|
public const string OrangeBook = "\U0001F4D9";
|
|
|
|
/// <summary>
|
|
/// Gets the "orange_circle" emoji.
|
|
/// Description: Orange circle.
|
|
/// </summary>
|
|
public const string OrangeCircle = "\U0001F7E0";
|
|
|
|
/// <summary>
|
|
/// Gets the "orange_heart" emoji.
|
|
/// Description: Orange heart.
|
|
/// </summary>
|
|
public const string OrangeHeart = "\U0001F9E1";
|
|
|
|
/// <summary>
|
|
/// Gets the "orange_square" emoji.
|
|
/// Description: Orange square.
|
|
/// </summary>
|
|
public const string OrangeSquare = "\U0001F7E7";
|
|
|
|
/// <summary>
|
|
/// Gets the "orangutan" emoji.
|
|
/// Description: Orangutan.
|
|
/// </summary>
|
|
public const string Orangutan = "\U0001F9A7";
|
|
|
|
/// <summary>
|
|
/// Gets the "orthodox_cross" emoji.
|
|
/// Description: Orthodox cross.
|
|
/// </summary>
|
|
public const string OrthodoxCross = "\U00002626";
|
|
|
|
/// <summary>
|
|
/// Gets the "otter" emoji.
|
|
/// Description: Otter.
|
|
/// </summary>
|
|
public const string Otter = "\U0001F9A6";
|
|
|
|
/// <summary>
|
|
/// Gets the "outbox_tray" emoji.
|
|
/// Description: Outbox tray.
|
|
/// </summary>
|
|
public const string OutboxTray = "\U0001F4E4";
|
|
|
|
/// <summary>
|
|
/// Gets the "owl" emoji.
|
|
/// Description: Owl.
|
|
/// </summary>
|
|
public const string Owl = "\U0001F989";
|
|
|
|
/// <summary>
|
|
/// Gets the "ox" emoji.
|
|
/// Description: Ox.
|
|
/// </summary>
|
|
public const string Ox = "\U0001F402";
|
|
|
|
/// <summary>
|
|
/// Gets the "oyster" emoji.
|
|
/// Description: Oyster.
|
|
/// </summary>
|
|
public const string Oyster = "\U0001F9AA";
|
|
|
|
/// <summary>
|
|
/// Gets the "package" emoji.
|
|
/// Description: Package.
|
|
/// </summary>
|
|
public const string Package = "\U0001F4E6";
|
|
|
|
/// <summary>
|
|
/// Gets the "page_facing_up" emoji.
|
|
/// Description: Page facing up.
|
|
/// </summary>
|
|
public const string PageFacingUp = "\U0001F4C4";
|
|
|
|
/// <summary>
|
|
/// Gets the "pager" emoji.
|
|
/// Description: Pager.
|
|
/// </summary>
|
|
public const string Pager = "\U0001F4DF";
|
|
|
|
/// <summary>
|
|
/// Gets the "page_with_curl" emoji.
|
|
/// Description: Page with curl.
|
|
/// </summary>
|
|
public const string PageWithCurl = "\U0001F4C3";
|
|
|
|
/// <summary>
|
|
/// Gets the "paintbrush" emoji.
|
|
/// Description: Paintbrush.
|
|
/// </summary>
|
|
public const string Paintbrush = "\U0001F58C";
|
|
|
|
/// <summary>
|
|
/// Gets the "palms_up_together" emoji.
|
|
/// Description: Palms up together.
|
|
/// </summary>
|
|
public const string PalmsUpTogether = "\U0001F932";
|
|
|
|
/// <summary>
|
|
/// Gets the "palm_tree" emoji.
|
|
/// Description: Palm tree.
|
|
/// </summary>
|
|
public const string PalmTree = "\U0001F334";
|
|
|
|
/// <summary>
|
|
/// Gets the "pancakes" emoji.
|
|
/// Description: Pancakes.
|
|
/// </summary>
|
|
public const string Pancakes = "\U0001F95E";
|
|
|
|
/// <summary>
|
|
/// Gets the "panda" emoji.
|
|
/// Description: Panda.
|
|
/// </summary>
|
|
public const string Panda = "\U0001F43C";
|
|
|
|
/// <summary>
|
|
/// Gets the "paperclip" emoji.
|
|
/// Description: Paperclip.
|
|
/// </summary>
|
|
public const string Paperclip = "\U0001F4CE";
|
|
|
|
/// <summary>
|
|
/// Gets the "parachute" emoji.
|
|
/// Description: Parachute.
|
|
/// </summary>
|
|
public const string Parachute = "\U0001FA82";
|
|
|
|
/// <summary>
|
|
/// Gets the "parrot" emoji.
|
|
/// Description: Parrot.
|
|
/// </summary>
|
|
public const string Parrot = "\U0001F99C";
|
|
|
|
/// <summary>
|
|
/// Gets the "part_alternation_mark" emoji.
|
|
/// Description: Part alternation mark.
|
|
/// </summary>
|
|
public const string PartAlternationMark = "\U0000303D";
|
|
|
|
/// <summary>
|
|
/// Gets the "partying_face" emoji.
|
|
/// Description: Partying face.
|
|
/// </summary>
|
|
public const string PartyingFace = "\U0001F973";
|
|
|
|
/// <summary>
|
|
/// Gets the "party_popper" emoji.
|
|
/// Description: Party popper.
|
|
/// </summary>
|
|
public const string PartyPopper = "\U0001F389";
|
|
|
|
/// <summary>
|
|
/// Gets the "passenger_ship" emoji.
|
|
/// Description: Passenger ship.
|
|
/// </summary>
|
|
public const string PassengerShip = "\U0001F6F3";
|
|
|
|
/// <summary>
|
|
/// Gets the "passport_control" emoji.
|
|
/// Description: Passport control.
|
|
/// </summary>
|
|
public const string PassportControl = "\U0001F6C2";
|
|
|
|
/// <summary>
|
|
/// Gets the "pause_button" emoji.
|
|
/// Description: Pause button.
|
|
/// </summary>
|
|
public const string PauseButton = "\U000023F8";
|
|
|
|
/// <summary>
|
|
/// Gets the "paw_prints" emoji.
|
|
/// Description: Paw prints.
|
|
/// </summary>
|
|
public const string PawPrints = "\U0001F43E";
|
|
|
|
/// <summary>
|
|
/// Gets the "p_button" emoji.
|
|
/// Description: P button.
|
|
/// </summary>
|
|
public const string PButton = "\U0001F17F";
|
|
|
|
/// <summary>
|
|
/// Gets the "peace_symbol" emoji.
|
|
/// Description: Peace symbol.
|
|
/// </summary>
|
|
public const string PeaceSymbol = "\U0000262E";
|
|
|
|
/// <summary>
|
|
/// Gets the "peach" emoji.
|
|
/// Description: Peach.
|
|
/// </summary>
|
|
public const string Peach = "\U0001F351";
|
|
|
|
/// <summary>
|
|
/// Gets the "peacock" emoji.
|
|
/// Description: Peacock.
|
|
/// </summary>
|
|
public const string Peacock = "\U0001F99A";
|
|
|
|
/// <summary>
|
|
/// Gets the "peanuts" emoji.
|
|
/// Description: Peanuts.
|
|
/// </summary>
|
|
public const string Peanuts = "\U0001F95C";
|
|
|
|
/// <summary>
|
|
/// Gets the "pear" emoji.
|
|
/// Description: Pear.
|
|
/// </summary>
|
|
public const string Pear = "\U0001F350";
|
|
|
|
/// <summary>
|
|
/// Gets the "pen" emoji.
|
|
/// Description: Pen.
|
|
/// </summary>
|
|
public const string Pen = "\U0001F58A";
|
|
|
|
/// <summary>
|
|
/// Gets the "pencil" emoji.
|
|
/// Description: Pencil.
|
|
/// </summary>
|
|
public const string Pencil = "\U0000270F";
|
|
|
|
/// <summary>
|
|
/// Gets the "penguin" emoji.
|
|
/// Description: Penguin.
|
|
/// </summary>
|
|
public const string Penguin = "\U0001F427";
|
|
|
|
/// <summary>
|
|
/// Gets the "pensive_face" emoji.
|
|
/// Description: Pensive face.
|
|
/// </summary>
|
|
public const string PensiveFace = "\U0001F614";
|
|
|
|
/// <summary>
|
|
/// Gets the "people_hugging" emoji.
|
|
/// Description: People hugging.
|
|
/// </summary>
|
|
public const string PeopleHugging = "\U0001FAC2";
|
|
|
|
/// <summary>
|
|
/// Gets the "people_with_bunny_ears" emoji.
|
|
/// Description: People with bunny ears.
|
|
/// </summary>
|
|
public const string PeopleWithBunnyEars = "\U0001F46F";
|
|
|
|
/// <summary>
|
|
/// Gets the "people_wrestling" emoji.
|
|
/// Description: People wrestling.
|
|
/// </summary>
|
|
public const string PeopleWrestling = "\U0001F93C";
|
|
|
|
/// <summary>
|
|
/// Gets the "performing_arts" emoji.
|
|
/// Description: Performing arts.
|
|
/// </summary>
|
|
public const string PerformingArts = "\U0001F3AD";
|
|
|
|
/// <summary>
|
|
/// Gets the "persevering_face" emoji.
|
|
/// Description: Persevering face.
|
|
/// </summary>
|
|
public const string PerseveringFace = "\U0001F623";
|
|
|
|
/// <summary>
|
|
/// Gets the "person" emoji.
|
|
/// Description: Person.
|
|
/// </summary>
|
|
public const string Person = "\U0001F9D1";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_beard" emoji.
|
|
/// Description: Person beard.
|
|
/// </summary>
|
|
public const string PersonBeard = "\U0001F9D4";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_biking" emoji.
|
|
/// Description: Person biking.
|
|
/// </summary>
|
|
public const string PersonBiking = "\U0001F6B4";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_blond_hair" emoji.
|
|
/// Description: Person blond hair.
|
|
/// </summary>
|
|
public const string PersonBlondHair = "\U0001F471";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_bouncing_ball" emoji.
|
|
/// Description: Person bouncing ball.
|
|
/// </summary>
|
|
public const string PersonBouncingBall = "\U000026F9";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_bowing" emoji.
|
|
/// Description: Person bowing.
|
|
/// </summary>
|
|
public const string PersonBowing = "\U0001F647";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_cartwheeling" emoji.
|
|
/// Description: Person cartwheeling.
|
|
/// </summary>
|
|
public const string PersonCartwheeling = "\U0001F938";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_climbing" emoji.
|
|
/// Description: Person climbing.
|
|
/// </summary>
|
|
public const string PersonClimbing = "\U0001F9D7";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_facepalming" emoji.
|
|
/// Description: Person facepalming.
|
|
/// </summary>
|
|
public const string PersonFacepalming = "\U0001F926";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_fencing" emoji.
|
|
/// Description: Person fencing.
|
|
/// </summary>
|
|
public const string PersonFencing = "\U0001F93A";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_frowning" emoji.
|
|
/// Description: Person frowning.
|
|
/// </summary>
|
|
public const string PersonFrowning = "\U0001F64D";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_gesturing_no" emoji.
|
|
/// Description: Person gesturing NO.
|
|
/// </summary>
|
|
public const string PersonGesturingNo = "\U0001F645";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_gesturing_ok" emoji.
|
|
/// Description: Person gesturing OK.
|
|
/// </summary>
|
|
public const string PersonGesturingOk = "\U0001F646";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_getting_haircut" emoji.
|
|
/// Description: Person getting haircut.
|
|
/// </summary>
|
|
public const string PersonGettingHaircut = "\U0001F487";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_getting_massage" emoji.
|
|
/// Description: Person getting massage.
|
|
/// </summary>
|
|
public const string PersonGettingMassage = "\U0001F486";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_golfing" emoji.
|
|
/// Description: Person golfing.
|
|
/// </summary>
|
|
public const string PersonGolfing = "\U0001F3CC";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_in_bed" emoji.
|
|
/// Description: Person in bed.
|
|
/// </summary>
|
|
public const string PersonInBed = "\U0001F6CC";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_in_lotus_position" emoji.
|
|
/// Description: Person in lotus position.
|
|
/// </summary>
|
|
public const string PersonInLotusPosition = "\U0001F9D8";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_in_steamy_room" emoji.
|
|
/// Description: Person in steamy room.
|
|
/// </summary>
|
|
public const string PersonInSteamyRoom = "\U0001F9D6";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_in_suit_levitating" emoji.
|
|
/// Description: Person in suit levitating.
|
|
/// </summary>
|
|
public const string PersonInSuitLevitating = "\U0001F574";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_in_tuxedo" emoji.
|
|
/// Description: Person in tuxedo.
|
|
/// </summary>
|
|
public const string PersonInTuxedo = "\U0001F935";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_juggling" emoji.
|
|
/// Description: Person juggling.
|
|
/// </summary>
|
|
public const string PersonJuggling = "\U0001F939";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_kneeling" emoji.
|
|
/// Description: Person kneeling.
|
|
/// </summary>
|
|
public const string PersonKneeling = "\U0001F9CE";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_lifting_weights" emoji.
|
|
/// Description: Person lifting weights.
|
|
/// </summary>
|
|
public const string PersonLiftingWeights = "\U0001F3CB";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_mountain_biking" emoji.
|
|
/// Description: Person mountain biking.
|
|
/// </summary>
|
|
public const string PersonMountainBiking = "\U0001F6B5";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_playing_handball" emoji.
|
|
/// Description: Person playing handball.
|
|
/// </summary>
|
|
public const string PersonPlayingHandball = "\U0001F93E";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_playing_water_polo" emoji.
|
|
/// Description: Person playing water polo.
|
|
/// </summary>
|
|
public const string PersonPlayingWaterPolo = "\U0001F93D";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_pouting" emoji.
|
|
/// Description: Person pouting.
|
|
/// </summary>
|
|
public const string PersonPouting = "\U0001F64E";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_raising_hand" emoji.
|
|
/// Description: Person raising hand.
|
|
/// </summary>
|
|
public const string PersonRaisingHand = "\U0001F64B";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_rowing_boat" emoji.
|
|
/// Description: Person rowing boat.
|
|
/// </summary>
|
|
public const string PersonRowingBoat = "\U0001F6A3";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_running" emoji.
|
|
/// Description: Person running.
|
|
/// </summary>
|
|
public const string PersonRunning = "\U0001F3C3";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_shrugging" emoji.
|
|
/// Description: Person shrugging.
|
|
/// </summary>
|
|
public const string PersonShrugging = "\U0001F937";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_standing" emoji.
|
|
/// Description: Person standing.
|
|
/// </summary>
|
|
public const string PersonStanding = "\U0001F9CD";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_surfing" emoji.
|
|
/// Description: Person surfing.
|
|
/// </summary>
|
|
public const string PersonSurfing = "\U0001F3C4";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_swimming" emoji.
|
|
/// Description: Person swimming.
|
|
/// </summary>
|
|
public const string PersonSwimming = "\U0001F3CA";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_taking_bath" emoji.
|
|
/// Description: Person taking bath.
|
|
/// </summary>
|
|
public const string PersonTakingBath = "\U0001F6C0";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_tipping_hand" emoji.
|
|
/// Description: Person tipping hand.
|
|
/// </summary>
|
|
public const string PersonTippingHand = "\U0001F481";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_walking" emoji.
|
|
/// Description: Person walking.
|
|
/// </summary>
|
|
public const string PersonWalking = "\U0001F6B6";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_wearing_turban" emoji.
|
|
/// Description: Person wearing turban.
|
|
/// </summary>
|
|
public const string PersonWearingTurban = "\U0001F473";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_with_skullcap" emoji.
|
|
/// Description: Person with skullcap.
|
|
/// </summary>
|
|
public const string PersonWithSkullcap = "\U0001F472";
|
|
|
|
/// <summary>
|
|
/// Gets the "person_with_veil" emoji.
|
|
/// Description: Person with veil.
|
|
/// </summary>
|
|
public const string PersonWithVeil = "\U0001F470";
|
|
|
|
/// <summary>
|
|
/// Gets the "petri_dish" emoji.
|
|
/// Description: Petri dish.
|
|
/// </summary>
|
|
public const string PetriDish = "\U0001F9EB";
|
|
|
|
/// <summary>
|
|
/// Gets the "pick" emoji.
|
|
/// Description: Pick.
|
|
/// </summary>
|
|
public const string Pick = "\U000026CF";
|
|
|
|
/// <summary>
|
|
/// Gets the "pickup_truck" emoji.
|
|
/// Description: Pickup truck.
|
|
/// </summary>
|
|
public const string PickupTruck = "\U0001F6FB";
|
|
|
|
/// <summary>
|
|
/// Gets the "pie" emoji.
|
|
/// Description: Pie.
|
|
/// </summary>
|
|
public const string Pie = "\U0001F967";
|
|
|
|
/// <summary>
|
|
/// Gets the "pig" emoji.
|
|
/// Description: Pig.
|
|
/// </summary>
|
|
public const string Pig = "\U0001F416";
|
|
|
|
/// <summary>
|
|
/// Gets the "pig_face" emoji.
|
|
/// Description: Pig face.
|
|
/// </summary>
|
|
public const string PigFace = "\U0001F437";
|
|
|
|
/// <summary>
|
|
/// Gets the "pig_nose" emoji.
|
|
/// Description: Pig nose.
|
|
/// </summary>
|
|
public const string PigNose = "\U0001F43D";
|
|
|
|
/// <summary>
|
|
/// Gets the "pile_of_poo" emoji.
|
|
/// Description: Pile of poo.
|
|
/// </summary>
|
|
public const string PileOfPoo = "\U0001F4A9";
|
|
|
|
/// <summary>
|
|
/// Gets the "pill" emoji.
|
|
/// Description: Pill.
|
|
/// </summary>
|
|
public const string Pill = "\U0001F48A";
|
|
|
|
/// <summary>
|
|
/// Gets the "piñata" emoji.
|
|
/// Description: Piñata.
|
|
/// </summary>
|
|
public const string Piñata = "\U0001FA85";
|
|
|
|
/// <summary>
|
|
/// Gets the "pinched_fingers" emoji.
|
|
/// Description: Pinched fingers.
|
|
/// </summary>
|
|
public const string PinchedFingers = "\U0001F90C";
|
|
|
|
/// <summary>
|
|
/// Gets the "pinching_hand" emoji.
|
|
/// Description: Pinching hand.
|
|
/// </summary>
|
|
public const string PinchingHand = "\U0001F90F";
|
|
|
|
/// <summary>
|
|
/// Gets the "pineapple" emoji.
|
|
/// Description: Pineapple.
|
|
/// </summary>
|
|
public const string Pineapple = "\U0001F34D";
|
|
|
|
/// <summary>
|
|
/// Gets the "pine_decoration" emoji.
|
|
/// Description: Pine decoration.
|
|
/// </summary>
|
|
public const string PineDecoration = "\U0001F38D";
|
|
|
|
/// <summary>
|
|
/// Gets the "ping_pong" emoji.
|
|
/// Description: Ping pong.
|
|
/// </summary>
|
|
public const string PingPong = "\U0001F3D3";
|
|
|
|
/// <summary>
|
|
/// Gets the "pisces" emoji.
|
|
/// Description: Pisces.
|
|
/// </summary>
|
|
public const string Pisces = "\U00002653";
|
|
|
|
/// <summary>
|
|
/// Gets the "pizza" emoji.
|
|
/// Description: Pizza.
|
|
/// </summary>
|
|
public const string Pizza = "\U0001F355";
|
|
|
|
/// <summary>
|
|
/// Gets the "placard" emoji.
|
|
/// Description: Placard.
|
|
/// </summary>
|
|
public const string Placard = "\U0001FAA7";
|
|
|
|
/// <summary>
|
|
/// Gets the "place_of_worship" emoji.
|
|
/// Description: Place of worship.
|
|
/// </summary>
|
|
public const string PlaceOfWorship = "\U0001F6D0";
|
|
|
|
/// <summary>
|
|
/// Gets the "play_button" emoji.
|
|
/// Description: Play button.
|
|
/// </summary>
|
|
public const string PlayButton = "\U000025B6";
|
|
|
|
/// <summary>
|
|
/// Gets the "play_or_pause_button" emoji.
|
|
/// Description: Play or pause button.
|
|
/// </summary>
|
|
public const string PlayOrPauseButton = "\U000023EF";
|
|
|
|
/// <summary>
|
|
/// Gets the "pleading_face" emoji.
|
|
/// Description: Pleading face.
|
|
/// </summary>
|
|
public const string PleadingFace = "\U0001F97A";
|
|
|
|
/// <summary>
|
|
/// Gets the "plunger" emoji.
|
|
/// Description: Plunger.
|
|
/// </summary>
|
|
public const string Plunger = "\U0001FAA0";
|
|
|
|
/// <summary>
|
|
/// Gets the "plus" emoji.
|
|
/// Description: Plus.
|
|
/// </summary>
|
|
public const string Plus = "\U00002795";
|
|
|
|
/// <summary>
|
|
/// Gets the "police_car" emoji.
|
|
/// Description: Police car.
|
|
/// </summary>
|
|
public const string PoliceCar = "\U0001F693";
|
|
|
|
/// <summary>
|
|
/// Gets the "police_car_light" emoji.
|
|
/// Description: Police car light.
|
|
/// </summary>
|
|
public const string PoliceCarLight = "\U0001F6A8";
|
|
|
|
/// <summary>
|
|
/// Gets the "police_officer" emoji.
|
|
/// Description: Police officer.
|
|
/// </summary>
|
|
public const string PoliceOfficer = "\U0001F46E";
|
|
|
|
/// <summary>
|
|
/// Gets the "poodle" emoji.
|
|
/// Description: Poodle.
|
|
/// </summary>
|
|
public const string Poodle = "\U0001F429";
|
|
|
|
/// <summary>
|
|
/// Gets the "pool_8_ball" emoji.
|
|
/// Description: Pool 8 ball.
|
|
/// </summary>
|
|
public const string Pool8Ball = "\U0001F3B1";
|
|
|
|
/// <summary>
|
|
/// Gets the "popcorn" emoji.
|
|
/// Description: Popcorn.
|
|
/// </summary>
|
|
public const string Popcorn = "\U0001F37F";
|
|
|
|
/// <summary>
|
|
/// Gets the "postal_horn" emoji.
|
|
/// Description: Postal horn.
|
|
/// </summary>
|
|
public const string PostalHorn = "\U0001F4EF";
|
|
|
|
/// <summary>
|
|
/// Gets the "postbox" emoji.
|
|
/// Description: Postbox.
|
|
/// </summary>
|
|
public const string Postbox = "\U0001F4EE";
|
|
|
|
/// <summary>
|
|
/// Gets the "post_office" emoji.
|
|
/// Description: Post office.
|
|
/// </summary>
|
|
public const string PostOffice = "\U0001F3E4";
|
|
|
|
/// <summary>
|
|
/// Gets the "potable_water" emoji.
|
|
/// Description: Potable water.
|
|
/// </summary>
|
|
public const string PotableWater = "\U0001F6B0";
|
|
|
|
/// <summary>
|
|
/// Gets the "potato" emoji.
|
|
/// Description: Potato.
|
|
/// </summary>
|
|
public const string Potato = "\U0001F954";
|
|
|
|
/// <summary>
|
|
/// Gets the "pot_of_food" emoji.
|
|
/// Description: Pot of food.
|
|
/// </summary>
|
|
public const string PotOfFood = "\U0001F372";
|
|
|
|
/// <summary>
|
|
/// Gets the "potted_plant" emoji.
|
|
/// Description: Potted plant.
|
|
/// </summary>
|
|
public const string PottedPlant = "\U0001FAB4";
|
|
|
|
/// <summary>
|
|
/// Gets the "poultry_leg" emoji.
|
|
/// Description: Poultry leg.
|
|
/// </summary>
|
|
public const string PoultryLeg = "\U0001F357";
|
|
|
|
/// <summary>
|
|
/// Gets the "pound_banknote" emoji.
|
|
/// Description: Pound banknote.
|
|
/// </summary>
|
|
public const string PoundBanknote = "\U0001F4B7";
|
|
|
|
/// <summary>
|
|
/// Gets the "pouting_cat" emoji.
|
|
/// Description: Pouting cat.
|
|
/// </summary>
|
|
public const string PoutingCat = "\U0001F63E";
|
|
|
|
/// <summary>
|
|
/// Gets the "pouting_face" emoji.
|
|
/// Description: Pouting face.
|
|
/// </summary>
|
|
public const string PoutingFace = "\U0001F621";
|
|
|
|
/// <summary>
|
|
/// Gets the "prayer_beads" emoji.
|
|
/// Description: Prayer beads.
|
|
/// </summary>
|
|
public const string PrayerBeads = "\U0001F4FF";
|
|
|
|
/// <summary>
|
|
/// Gets the "pregnant_woman" emoji.
|
|
/// Description: Pregnant woman.
|
|
/// </summary>
|
|
public const string PregnantWoman = "\U0001F930";
|
|
|
|
/// <summary>
|
|
/// Gets the "pretzel" emoji.
|
|
/// Description: Pretzel.
|
|
/// </summary>
|
|
public const string Pretzel = "\U0001F968";
|
|
|
|
/// <summary>
|
|
/// Gets the "prince" emoji.
|
|
/// Description: Prince.
|
|
/// </summary>
|
|
public const string Prince = "\U0001F934";
|
|
|
|
/// <summary>
|
|
/// Gets the "princess" emoji.
|
|
/// Description: Princess.
|
|
/// </summary>
|
|
public const string Princess = "\U0001F478";
|
|
|
|
/// <summary>
|
|
/// Gets the "printer" emoji.
|
|
/// Description: Printer.
|
|
/// </summary>
|
|
public const string Printer = "\U0001F5A8";
|
|
|
|
/// <summary>
|
|
/// Gets the "prohibited" emoji.
|
|
/// Description: Prohibited.
|
|
/// </summary>
|
|
public const string Prohibited = "\U0001F6AB";
|
|
|
|
/// <summary>
|
|
/// Gets the "purple_circle" emoji.
|
|
/// Description: Purple circle.
|
|
/// </summary>
|
|
public const string PurpleCircle = "\U0001F7E3";
|
|
|
|
/// <summary>
|
|
/// Gets the "purple_heart" emoji.
|
|
/// Description: Purple heart.
|
|
/// </summary>
|
|
public const string PurpleHeart = "\U0001F49C";
|
|
|
|
/// <summary>
|
|
/// Gets the "purple_square" emoji.
|
|
/// Description: Purple square.
|
|
/// </summary>
|
|
public const string PurpleSquare = "\U0001F7EA";
|
|
|
|
/// <summary>
|
|
/// Gets the "purse" emoji.
|
|
/// Description: Purse.
|
|
/// </summary>
|
|
public const string Purse = "\U0001F45B";
|
|
|
|
/// <summary>
|
|
/// Gets the "pushpin" emoji.
|
|
/// Description: Pushpin.
|
|
/// </summary>
|
|
public const string Pushpin = "\U0001F4CC";
|
|
|
|
/// <summary>
|
|
/// Gets the "puzzle_piece" emoji.
|
|
/// Description: Puzzle piece.
|
|
/// </summary>
|
|
public const string PuzzlePiece = "\U0001F9E9";
|
|
|
|
/// <summary>
|
|
/// Gets the "rabbit" emoji.
|
|
/// Description: Rabbit.
|
|
/// </summary>
|
|
public const string Rabbit = "\U0001F407";
|
|
|
|
/// <summary>
|
|
/// Gets the "rabbit_face" emoji.
|
|
/// Description: Rabbit face.
|
|
/// </summary>
|
|
public const string RabbitFace = "\U0001F430";
|
|
|
|
/// <summary>
|
|
/// Gets the "raccoon" emoji.
|
|
/// Description: Raccoon.
|
|
/// </summary>
|
|
public const string Raccoon = "\U0001F99D";
|
|
|
|
/// <summary>
|
|
/// Gets the "racing_car" emoji.
|
|
/// Description: Racing car.
|
|
/// </summary>
|
|
public const string RacingCar = "\U0001F3CE";
|
|
|
|
/// <summary>
|
|
/// Gets the "radio" emoji.
|
|
/// Description: Radio.
|
|
/// </summary>
|
|
public const string Radio = "\U0001F4FB";
|
|
|
|
/// <summary>
|
|
/// Gets the "radioactive" emoji.
|
|
/// Description: Radioactive.
|
|
/// </summary>
|
|
public const string Radioactive = "\U00002622";
|
|
|
|
/// <summary>
|
|
/// Gets the "radio_button" emoji.
|
|
/// Description: Radio button.
|
|
/// </summary>
|
|
public const string RadioButton = "\U0001F518";
|
|
|
|
/// <summary>
|
|
/// Gets the "railway_car" emoji.
|
|
/// Description: Railway car.
|
|
/// </summary>
|
|
public const string RailwayCar = "\U0001F683";
|
|
|
|
/// <summary>
|
|
/// Gets the "railway_track" emoji.
|
|
/// Description: Railway track.
|
|
/// </summary>
|
|
public const string RailwayTrack = "\U0001F6E4";
|
|
|
|
/// <summary>
|
|
/// Gets the "rainbow" emoji.
|
|
/// Description: Rainbow.
|
|
/// </summary>
|
|
public const string Rainbow = "\U0001F308";
|
|
|
|
/// <summary>
|
|
/// Gets the "raised_back_of_hand" emoji.
|
|
/// Description: Raised back of hand.
|
|
/// </summary>
|
|
public const string RaisedBackOfHand = "\U0001F91A";
|
|
|
|
/// <summary>
|
|
/// Gets the "raised_fist" emoji.
|
|
/// Description: Raised fist.
|
|
/// </summary>
|
|
public const string RaisedFist = "\U0000270A";
|
|
|
|
/// <summary>
|
|
/// Gets the "raised_hand" emoji.
|
|
/// Description: Raised hand.
|
|
/// </summary>
|
|
public const string RaisedHand = "\U0000270B";
|
|
|
|
/// <summary>
|
|
/// Gets the "raising_hands" emoji.
|
|
/// Description: Raising hands.
|
|
/// </summary>
|
|
public const string RaisingHands = "\U0001F64C";
|
|
|
|
/// <summary>
|
|
/// Gets the "ram" emoji.
|
|
/// Description: Ram.
|
|
/// </summary>
|
|
public const string Ram = "\U0001F40F";
|
|
|
|
/// <summary>
|
|
/// Gets the "rat" emoji.
|
|
/// Description: Rat.
|
|
/// </summary>
|
|
public const string Rat = "\U0001F400";
|
|
|
|
/// <summary>
|
|
/// Gets the "razor" emoji.
|
|
/// Description: Razor.
|
|
/// </summary>
|
|
public const string Razor = "\U0001FA92";
|
|
|
|
/// <summary>
|
|
/// Gets the "receipt" emoji.
|
|
/// Description: Receipt.
|
|
/// </summary>
|
|
public const string Receipt = "\U0001F9FE";
|
|
|
|
/// <summary>
|
|
/// Gets the "record_button" emoji.
|
|
/// Description: Record button.
|
|
/// </summary>
|
|
public const string RecordButton = "\U000023FA";
|
|
|
|
/// <summary>
|
|
/// Gets the "recycling_symbol" emoji.
|
|
/// Description: Recycling symbol.
|
|
/// </summary>
|
|
public const string RecyclingSymbol = "\U0000267B";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_apple" emoji.
|
|
/// Description: Red apple.
|
|
/// </summary>
|
|
public const string RedApple = "\U0001F34E";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_circle" emoji.
|
|
/// Description: Red circle.
|
|
/// </summary>
|
|
public const string RedCircle = "\U0001F534";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_envelope" emoji.
|
|
/// Description: Red envelope.
|
|
/// </summary>
|
|
public const string RedEnvelope = "\U0001F9E7";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_exclamation_mark" emoji.
|
|
/// Description: Red exclamation mark.
|
|
/// </summary>
|
|
public const string RedExclamationMark = "\U00002757";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_hair" emoji.
|
|
/// Description: Red hair.
|
|
/// </summary>
|
|
public const string RedHair = "\U0001F9B0";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_heart" emoji.
|
|
/// Description: Red heart.
|
|
/// </summary>
|
|
public const string RedHeart = "\U00002764";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_paper_lantern" emoji.
|
|
/// Description: Red paper lantern.
|
|
/// </summary>
|
|
public const string RedPaperLantern = "\U0001F3EE";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_question_mark" emoji.
|
|
/// Description: Red question mark.
|
|
/// </summary>
|
|
public const string RedQuestionMark = "\U00002753";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_square" emoji.
|
|
/// Description: Red square.
|
|
/// </summary>
|
|
public const string RedSquare = "\U0001F7E5";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_triangle_pointed_down" emoji.
|
|
/// Description: Red triangle pointed down.
|
|
/// </summary>
|
|
public const string RedTrianglePointedDown = "\U0001F53B";
|
|
|
|
/// <summary>
|
|
/// Gets the "red_triangle_pointed_up" emoji.
|
|
/// Description: Red triangle pointed up.
|
|
/// </summary>
|
|
public const string RedTrianglePointedUp = "\U0001F53A";
|
|
|
|
/// <summary>
|
|
/// Gets the "registered" emoji.
|
|
/// Description: Registered.
|
|
/// </summary>
|
|
public const string Registered = "\U000000AE";
|
|
|
|
/// <summary>
|
|
/// Gets the "relieved_face" emoji.
|
|
/// Description: Relieved face.
|
|
/// </summary>
|
|
public const string RelievedFace = "\U0001F60C";
|
|
|
|
/// <summary>
|
|
/// Gets the "reminder_ribbon" emoji.
|
|
/// Description: Reminder ribbon.
|
|
/// </summary>
|
|
public const string ReminderRibbon = "\U0001F397";
|
|
|
|
/// <summary>
|
|
/// Gets the "repeat_button" emoji.
|
|
/// Description: Repeat button.
|
|
/// </summary>
|
|
public const string RepeatButton = "\U0001F501";
|
|
|
|
/// <summary>
|
|
/// Gets the "repeat_single_button" emoji.
|
|
/// Description: Repeat single button.
|
|
/// </summary>
|
|
public const string RepeatSingleButton = "\U0001F502";
|
|
|
|
/// <summary>
|
|
/// Gets the "rescue_workers_helmet" emoji.
|
|
/// Description: Rescue worker s helmet.
|
|
/// </summary>
|
|
public const string RescueWorkersHelmet = "\U000026D1";
|
|
|
|
/// <summary>
|
|
/// Gets the "restroom" emoji.
|
|
/// Description: Restroom.
|
|
/// </summary>
|
|
public const string Restroom = "\U0001F6BB";
|
|
|
|
/// <summary>
|
|
/// Gets the "reverse_button" emoji.
|
|
/// Description: Reverse button.
|
|
/// </summary>
|
|
public const string ReverseButton = "\U000025C0";
|
|
|
|
/// <summary>
|
|
/// Gets the "revolving_hearts" emoji.
|
|
/// Description: Revolving hearts.
|
|
/// </summary>
|
|
public const string RevolvingHearts = "\U0001F49E";
|
|
|
|
/// <summary>
|
|
/// Gets the "rhinoceros" emoji.
|
|
/// Description: Rhinoceros.
|
|
/// </summary>
|
|
public const string Rhinoceros = "\U0001F98F";
|
|
|
|
/// <summary>
|
|
/// Gets the "ribbon" emoji.
|
|
/// Description: Ribbon.
|
|
/// </summary>
|
|
public const string Ribbon = "\U0001F380";
|
|
|
|
/// <summary>
|
|
/// Gets the "rice_ball" emoji.
|
|
/// Description: Rice ball.
|
|
/// </summary>
|
|
public const string RiceBall = "\U0001F359";
|
|
|
|
/// <summary>
|
|
/// Gets the "rice_cracker" emoji.
|
|
/// Description: Rice cracker.
|
|
/// </summary>
|
|
public const string RiceCracker = "\U0001F358";
|
|
|
|
/// <summary>
|
|
/// Gets the "right_anger_bubble" emoji.
|
|
/// Description: Right anger bubble.
|
|
/// </summary>
|
|
public const string RightAngerBubble = "\U0001F5EF";
|
|
|
|
/// <summary>
|
|
/// Gets the "right_arrow" emoji.
|
|
/// Description: Right arrow.
|
|
/// </summary>
|
|
public const string RightArrow = "\U000027A1";
|
|
|
|
/// <summary>
|
|
/// Gets the "right_arrow_curving_down" emoji.
|
|
/// Description: Right arrow curving down.
|
|
/// </summary>
|
|
public const string RightArrowCurvingDown = "\U00002935";
|
|
|
|
/// <summary>
|
|
/// Gets the "right_arrow_curving_left" emoji.
|
|
/// Description: Right arrow curving left.
|
|
/// </summary>
|
|
public const string RightArrowCurvingLeft = "\U000021A9";
|
|
|
|
/// <summary>
|
|
/// Gets the "right_arrow_curving_up" emoji.
|
|
/// Description: Right arrow curving up.
|
|
/// </summary>
|
|
public const string RightArrowCurvingUp = "\U00002934";
|
|
|
|
/// <summary>
|
|
/// Gets the "right_facing_fist" emoji.
|
|
/// Description: right facing fist.
|
|
/// </summary>
|
|
public const string RightFacingFist = "\U0001F91C";
|
|
|
|
/// <summary>
|
|
/// Gets the "ring" emoji.
|
|
/// Description: Ring.
|
|
/// </summary>
|
|
public const string Ring = "\U0001F48D";
|
|
|
|
/// <summary>
|
|
/// Gets the "ringed_planet" emoji.
|
|
/// Description: Ringed planet.
|
|
/// </summary>
|
|
public const string RingedPlanet = "\U0001FA90";
|
|
|
|
/// <summary>
|
|
/// Gets the "roasted_sweet_potato" emoji.
|
|
/// Description: Roasted sweet potato.
|
|
/// </summary>
|
|
public const string RoastedSweetPotato = "\U0001F360";
|
|
|
|
/// <summary>
|
|
/// Gets the "robot" emoji.
|
|
/// Description: Robot.
|
|
/// </summary>
|
|
public const string Robot = "\U0001F916";
|
|
|
|
/// <summary>
|
|
/// Gets the "rock" emoji.
|
|
/// Description: Rock.
|
|
/// </summary>
|
|
public const string Rock = "\U0001FAA8";
|
|
|
|
/// <summary>
|
|
/// Gets the "rocket" emoji.
|
|
/// Description: Rocket.
|
|
/// </summary>
|
|
public const string Rocket = "\U0001F680";
|
|
|
|
/// <summary>
|
|
/// Gets the "rolled_up_newspaper" emoji.
|
|
/// Description: rolled up newspaper.
|
|
/// </summary>
|
|
public const string RolledUpNewspaper = "\U0001F5DE";
|
|
|
|
/// <summary>
|
|
/// Gets the "roller_coaster" emoji.
|
|
/// Description: Roller coaster.
|
|
/// </summary>
|
|
public const string RollerCoaster = "\U0001F3A2";
|
|
|
|
/// <summary>
|
|
/// Gets the "roller_skate" emoji.
|
|
/// Description: Roller skate.
|
|
/// </summary>
|
|
public const string RollerSkate = "\U0001F6FC";
|
|
|
|
/// <summary>
|
|
/// Gets the "rolling_on_the_floor_laughing" emoji.
|
|
/// Description: Rolling on the floor laughing.
|
|
/// </summary>
|
|
public const string RollingOnTheFloorLaughing = "\U0001F923";
|
|
|
|
/// <summary>
|
|
/// Gets the "roll_of_paper" emoji.
|
|
/// Description: Roll of paper.
|
|
/// </summary>
|
|
public const string RollOfPaper = "\U0001F9FB";
|
|
|
|
/// <summary>
|
|
/// Gets the "rooster" emoji.
|
|
/// Description: Rooster.
|
|
/// </summary>
|
|
public const string Rooster = "\U0001F413";
|
|
|
|
/// <summary>
|
|
/// Gets the "rose" emoji.
|
|
/// Description: Rose.
|
|
/// </summary>
|
|
public const string Rose = "\U0001F339";
|
|
|
|
/// <summary>
|
|
/// Gets the "rosette" emoji.
|
|
/// Description: Rosette.
|
|
/// </summary>
|
|
public const string Rosette = "\U0001F3F5";
|
|
|
|
/// <summary>
|
|
/// Gets the "round_pushpin" emoji.
|
|
/// Description: Round pushpin.
|
|
/// </summary>
|
|
public const string RoundPushpin = "\U0001F4CD";
|
|
|
|
/// <summary>
|
|
/// Gets the "rugby_football" emoji.
|
|
/// Description: Rugby football.
|
|
/// </summary>
|
|
public const string RugbyFootball = "\U0001F3C9";
|
|
|
|
/// <summary>
|
|
/// Gets the "running_shirt" emoji.
|
|
/// Description: Running shirt.
|
|
/// </summary>
|
|
public const string RunningShirt = "\U0001F3BD";
|
|
|
|
/// <summary>
|
|
/// Gets the "running_shoe" emoji.
|
|
/// Description: Running shoe.
|
|
/// </summary>
|
|
public const string RunningShoe = "\U0001F45F";
|
|
|
|
/// <summary>
|
|
/// Gets the "sad_but_relieved_face" emoji.
|
|
/// Description: Sad but relieved face.
|
|
/// </summary>
|
|
public const string SadButRelievedFace = "\U0001F625";
|
|
|
|
/// <summary>
|
|
/// Gets the "safety_pin" emoji.
|
|
/// Description: Safety pin.
|
|
/// </summary>
|
|
public const string SafetyPin = "\U0001F9F7";
|
|
|
|
/// <summary>
|
|
/// Gets the "safety_vest" emoji.
|
|
/// Description: Safety vest.
|
|
/// </summary>
|
|
public const string SafetyVest = "\U0001F9BA";
|
|
|
|
/// <summary>
|
|
/// Gets the "sagittarius" emoji.
|
|
/// Description: Sagittarius.
|
|
/// </summary>
|
|
public const string Sagittarius = "\U00002650";
|
|
|
|
/// <summary>
|
|
/// Gets the "sailboat" emoji.
|
|
/// Description: Sailboat.
|
|
/// </summary>
|
|
public const string Sailboat = "\U000026F5";
|
|
|
|
/// <summary>
|
|
/// Gets the "sake" emoji.
|
|
/// Description: Sake.
|
|
/// </summary>
|
|
public const string Sake = "\U0001F376";
|
|
|
|
/// <summary>
|
|
/// Gets the "salt" emoji.
|
|
/// Description: Salt.
|
|
/// </summary>
|
|
public const string Salt = "\U0001F9C2";
|
|
|
|
/// <summary>
|
|
/// Gets the "sandwich" emoji.
|
|
/// Description: Sandwich.
|
|
/// </summary>
|
|
public const string Sandwich = "\U0001F96A";
|
|
|
|
/// <summary>
|
|
/// Gets the "santa_claus" emoji.
|
|
/// Description: Santa claus.
|
|
/// </summary>
|
|
public const string SantaClaus = "\U0001F385";
|
|
|
|
/// <summary>
|
|
/// Gets the "sari" emoji.
|
|
/// Description: Sari.
|
|
/// </summary>
|
|
public const string Sari = "\U0001F97B";
|
|
|
|
/// <summary>
|
|
/// Gets the "satellite" emoji.
|
|
/// Description: Satellite.
|
|
/// </summary>
|
|
public const string Satellite = "\U0001F6F0";
|
|
|
|
/// <summary>
|
|
/// Gets the "satellite_antenna" emoji.
|
|
/// Description: Satellite antenna.
|
|
/// </summary>
|
|
public const string SatelliteAntenna = "\U0001F4E1";
|
|
|
|
/// <summary>
|
|
/// Gets the "sauropod" emoji.
|
|
/// Description: Sauropod.
|
|
/// </summary>
|
|
public const string Sauropod = "\U0001F995";
|
|
|
|
/// <summary>
|
|
/// Gets the "saxophone" emoji.
|
|
/// Description: Saxophone.
|
|
/// </summary>
|
|
public const string Saxophone = "\U0001F3B7";
|
|
|
|
/// <summary>
|
|
/// Gets the "scarf" emoji.
|
|
/// Description: Scarf.
|
|
/// </summary>
|
|
public const string Scarf = "\U0001F9E3";
|
|
|
|
/// <summary>
|
|
/// Gets the "school" emoji.
|
|
/// Description: School.
|
|
/// </summary>
|
|
public const string School = "\U0001F3EB";
|
|
|
|
/// <summary>
|
|
/// Gets the "scissors" emoji.
|
|
/// Description: Scissors.
|
|
/// </summary>
|
|
public const string Scissors = "\U00002702";
|
|
|
|
/// <summary>
|
|
/// Gets the "scorpio" emoji.
|
|
/// Description: Scorpio.
|
|
/// </summary>
|
|
public const string Scorpio = "\U0000264F";
|
|
|
|
/// <summary>
|
|
/// Gets the "scorpion" emoji.
|
|
/// Description: Scorpion.
|
|
/// </summary>
|
|
public const string Scorpion = "\U0001F982";
|
|
|
|
/// <summary>
|
|
/// Gets the "screwdriver" emoji.
|
|
/// Description: Screwdriver.
|
|
/// </summary>
|
|
public const string Screwdriver = "\U0001FA9B";
|
|
|
|
/// <summary>
|
|
/// Gets the "scroll" emoji.
|
|
/// Description: Scroll.
|
|
/// </summary>
|
|
public const string Scroll = "\U0001F4DC";
|
|
|
|
/// <summary>
|
|
/// Gets the "seal" emoji.
|
|
/// Description: Seal.
|
|
/// </summary>
|
|
public const string Seal = "\U0001F9AD";
|
|
|
|
/// <summary>
|
|
/// Gets the "seat" emoji.
|
|
/// Description: Seat.
|
|
/// </summary>
|
|
public const string Seat = "\U0001F4BA";
|
|
|
|
/// <summary>
|
|
/// Gets the "2nd_place_medal" emoji.
|
|
/// Description: 2nd place medal.
|
|
/// </summary>
|
|
public const string SecondPlaceMedal = "\U0001F948";
|
|
|
|
/// <summary>
|
|
/// Gets the "seedling" emoji.
|
|
/// Description: Seedling.
|
|
/// </summary>
|
|
public const string Seedling = "\U0001F331";
|
|
|
|
/// <summary>
|
|
/// Gets the "see_no_evil_monkey" emoji.
|
|
/// Description: see no evil monkey.
|
|
/// </summary>
|
|
public const string SeeNoEvilMonkey = "\U0001F648";
|
|
|
|
/// <summary>
|
|
/// Gets the "selfie" emoji.
|
|
/// Description: Selfie.
|
|
/// </summary>
|
|
public const string Selfie = "\U0001F933";
|
|
|
|
/// <summary>
|
|
/// Gets the "seven_o_clock" emoji.
|
|
/// Description: Seven o clock.
|
|
/// </summary>
|
|
public const string SevenOClock = "\U0001F556";
|
|
|
|
/// <summary>
|
|
/// Gets the "seven_thirty" emoji.
|
|
/// Description: seven thirty.
|
|
/// </summary>
|
|
public const string SevenThirty = "\U0001F562";
|
|
|
|
/// <summary>
|
|
/// Gets the "sewing_needle" emoji.
|
|
/// Description: Sewing needle.
|
|
/// </summary>
|
|
public const string SewingNeedle = "\U0001FAA1";
|
|
|
|
/// <summary>
|
|
/// Gets the "shallow_pan_of_food" emoji.
|
|
/// Description: Shallow pan of food.
|
|
/// </summary>
|
|
public const string ShallowPanOfFood = "\U0001F958";
|
|
|
|
/// <summary>
|
|
/// Gets the "shamrock" emoji.
|
|
/// Description: Shamrock.
|
|
/// </summary>
|
|
public const string Shamrock = "\U00002618";
|
|
|
|
/// <summary>
|
|
/// Gets the "shark" emoji.
|
|
/// Description: Shark.
|
|
/// </summary>
|
|
public const string Shark = "\U0001F988";
|
|
|
|
/// <summary>
|
|
/// Gets the "shaved_ice" emoji.
|
|
/// Description: Shaved ice.
|
|
/// </summary>
|
|
public const string ShavedIce = "\U0001F367";
|
|
|
|
/// <summary>
|
|
/// Gets the "sheaf_of_rice" emoji.
|
|
/// Description: Sheaf of rice.
|
|
/// </summary>
|
|
public const string SheafOfRice = "\U0001F33E";
|
|
|
|
/// <summary>
|
|
/// Gets the "shield" emoji.
|
|
/// Description: Shield.
|
|
/// </summary>
|
|
public const string Shield = "\U0001F6E1";
|
|
|
|
/// <summary>
|
|
/// Gets the "shinto_shrine" emoji.
|
|
/// Description: Shinto shrine.
|
|
/// </summary>
|
|
public const string ShintoShrine = "\U000026E9";
|
|
|
|
/// <summary>
|
|
/// Gets the "ship" emoji.
|
|
/// Description: Ship.
|
|
/// </summary>
|
|
public const string Ship = "\U0001F6A2";
|
|
|
|
/// <summary>
|
|
/// Gets the "shooting_star" emoji.
|
|
/// Description: Shooting star.
|
|
/// </summary>
|
|
public const string ShootingStar = "\U0001F320";
|
|
|
|
/// <summary>
|
|
/// Gets the "shopping_bags" emoji.
|
|
/// Description: Shopping bags.
|
|
/// </summary>
|
|
public const string ShoppingBags = "\U0001F6CD";
|
|
|
|
/// <summary>
|
|
/// Gets the "shopping_cart" emoji.
|
|
/// Description: Shopping cart.
|
|
/// </summary>
|
|
public const string ShoppingCart = "\U0001F6D2";
|
|
|
|
/// <summary>
|
|
/// Gets the "shortcake" emoji.
|
|
/// Description: Shortcake.
|
|
/// </summary>
|
|
public const string Shortcake = "\U0001F370";
|
|
|
|
/// <summary>
|
|
/// Gets the "shorts" emoji.
|
|
/// Description: Shorts.
|
|
/// </summary>
|
|
public const string Shorts = "\U0001FA73";
|
|
|
|
/// <summary>
|
|
/// Gets the "shower" emoji.
|
|
/// Description: Shower.
|
|
/// </summary>
|
|
public const string Shower = "\U0001F6BF";
|
|
|
|
/// <summary>
|
|
/// Gets the "shrimp" emoji.
|
|
/// Description: Shrimp.
|
|
/// </summary>
|
|
public const string Shrimp = "\U0001F990";
|
|
|
|
/// <summary>
|
|
/// Gets the "shuffle_tracks_button" emoji.
|
|
/// Description: Shuffle tracks button.
|
|
/// </summary>
|
|
public const string ShuffleTracksButton = "\U0001F500";
|
|
|
|
/// <summary>
|
|
/// Gets the "shushing_face" emoji.
|
|
/// Description: Shushing face.
|
|
/// </summary>
|
|
public const string ShushingFace = "\U0001F92B";
|
|
|
|
/// <summary>
|
|
/// Gets the "sign_of_the_horns" emoji.
|
|
/// Description: Sign of the horns.
|
|
/// </summary>
|
|
public const string SignOfTheHorns = "\U0001F918";
|
|
|
|
/// <summary>
|
|
/// Gets the "six_o_clock" emoji.
|
|
/// Description: Six o clock.
|
|
/// </summary>
|
|
public const string SixOClock = "\U0001F555";
|
|
|
|
/// <summary>
|
|
/// Gets the "six_thirty" emoji.
|
|
/// Description: six thirty.
|
|
/// </summary>
|
|
public const string SixThirty = "\U0001F561";
|
|
|
|
/// <summary>
|
|
/// Gets the "skateboard" emoji.
|
|
/// Description: Skateboard.
|
|
/// </summary>
|
|
public const string Skateboard = "\U0001F6F9";
|
|
|
|
/// <summary>
|
|
/// Gets the "skier" emoji.
|
|
/// Description: Skier.
|
|
/// </summary>
|
|
public const string Skier = "\U000026F7";
|
|
|
|
/// <summary>
|
|
/// Gets the "skis" emoji.
|
|
/// Description: Skis.
|
|
/// </summary>
|
|
public const string Skis = "\U0001F3BF";
|
|
|
|
/// <summary>
|
|
/// Gets the "skull" emoji.
|
|
/// Description: Skull.
|
|
/// </summary>
|
|
public const string Skull = "\U0001F480";
|
|
|
|
/// <summary>
|
|
/// Gets the "skull_and_crossbones" emoji.
|
|
/// Description: Skull and crossbones.
|
|
/// </summary>
|
|
public const string SkullAndCrossbones = "\U00002620";
|
|
|
|
/// <summary>
|
|
/// Gets the "skunk" emoji.
|
|
/// Description: Skunk.
|
|
/// </summary>
|
|
public const string Skunk = "\U0001F9A8";
|
|
|
|
/// <summary>
|
|
/// Gets the "sled" emoji.
|
|
/// Description: Sled.
|
|
/// </summary>
|
|
public const string Sled = "\U0001F6F7";
|
|
|
|
/// <summary>
|
|
/// Gets the "sleeping_face" emoji.
|
|
/// Description: Sleeping face.
|
|
/// </summary>
|
|
public const string SleepingFace = "\U0001F634";
|
|
|
|
/// <summary>
|
|
/// Gets the "sleepy_face" emoji.
|
|
/// Description: Sleepy face.
|
|
/// </summary>
|
|
public const string SleepyFace = "\U0001F62A";
|
|
|
|
/// <summary>
|
|
/// Gets the "slightly_frowning_face" emoji.
|
|
/// Description: Slightly frowning face.
|
|
/// </summary>
|
|
public const string SlightlyFrowningFace = "\U0001F641";
|
|
|
|
/// <summary>
|
|
/// Gets the "slightly_smiling_face" emoji.
|
|
/// Description: Slightly smiling face.
|
|
/// </summary>
|
|
public const string SlightlySmilingFace = "\U0001F642";
|
|
|
|
/// <summary>
|
|
/// Gets the "sloth" emoji.
|
|
/// Description: Sloth.
|
|
/// </summary>
|
|
public const string Sloth = "\U0001F9A5";
|
|
|
|
/// <summary>
|
|
/// Gets the "slot_machine" emoji.
|
|
/// Description: Slot machine.
|
|
/// </summary>
|
|
public const string SlotMachine = "\U0001F3B0";
|
|
|
|
/// <summary>
|
|
/// Gets the "small_airplane" emoji.
|
|
/// Description: Small airplane.
|
|
/// </summary>
|
|
public const string SmallAirplane = "\U0001F6E9";
|
|
|
|
/// <summary>
|
|
/// Gets the "small_blue_diamond" emoji.
|
|
/// Description: Small blue diamond.
|
|
/// </summary>
|
|
public const string SmallBlueDiamond = "\U0001F539";
|
|
|
|
/// <summary>
|
|
/// Gets the "small_orange_diamond" emoji.
|
|
/// Description: Small orange diamond.
|
|
/// </summary>
|
|
public const string SmallOrangeDiamond = "\U0001F538";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_cat_with_heart_eyes" emoji.
|
|
/// Description: smiling cat with heart eyes.
|
|
/// </summary>
|
|
public const string SmilingCatWithHeartEyes = "\U0001F63B";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face" emoji.
|
|
/// Description: Smiling face.
|
|
/// </summary>
|
|
public const string SmilingFace = "\U0000263A";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face_with_halo" emoji.
|
|
/// Description: Smiling face with halo.
|
|
/// </summary>
|
|
public const string SmilingFaceWithHalo = "\U0001F607";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face_with_heart_eyes" emoji.
|
|
/// Description: smiling face with heart eyes.
|
|
/// </summary>
|
|
public const string SmilingFaceWithHeartEyes = "\U0001F60D";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face_with_hearts" emoji.
|
|
/// Description: Smiling face with hearts.
|
|
/// </summary>
|
|
public const string SmilingFaceWithHearts = "\U0001F970";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face_with_horns" emoji.
|
|
/// Description: Smiling face with horns.
|
|
/// </summary>
|
|
public const string SmilingFaceWithHorns = "\U0001F608";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face_with_smiling_eyes" emoji.
|
|
/// Description: Smiling face with smiling eyes.
|
|
/// </summary>
|
|
public const string SmilingFaceWithSmilingEyes = "\U0001F60A";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face_with_sunglasses" emoji.
|
|
/// Description: Smiling face with sunglasses.
|
|
/// </summary>
|
|
public const string SmilingFaceWithSunglasses = "\U0001F60E";
|
|
|
|
/// <summary>
|
|
/// Gets the "smiling_face_with_tear" emoji.
|
|
/// Description: Smiling face with tear.
|
|
/// </summary>
|
|
public const string SmilingFaceWithTear = "\U0001F972";
|
|
|
|
/// <summary>
|
|
/// Gets the "smirking_face" emoji.
|
|
/// Description: Smirking face.
|
|
/// </summary>
|
|
public const string SmirkingFace = "\U0001F60F";
|
|
|
|
/// <summary>
|
|
/// Gets the "snail" emoji.
|
|
/// Description: Snail.
|
|
/// </summary>
|
|
public const string Snail = "\U0001F40C";
|
|
|
|
/// <summary>
|
|
/// Gets the "snake" emoji.
|
|
/// Description: Snake.
|
|
/// </summary>
|
|
public const string Snake = "\U0001F40D";
|
|
|
|
/// <summary>
|
|
/// Gets the "sneezing_face" emoji.
|
|
/// Description: Sneezing face.
|
|
/// </summary>
|
|
public const string SneezingFace = "\U0001F927";
|
|
|
|
/// <summary>
|
|
/// Gets the "snowboarder" emoji.
|
|
/// Description: Snowboarder.
|
|
/// </summary>
|
|
public const string Snowboarder = "\U0001F3C2";
|
|
|
|
/// <summary>
|
|
/// Gets the "snow_capped_mountain" emoji.
|
|
/// Description: snow capped mountain.
|
|
/// </summary>
|
|
public const string SnowCappedMountain = "\U0001F3D4";
|
|
|
|
/// <summary>
|
|
/// Gets the "snowflake" emoji.
|
|
/// Description: Snowflake.
|
|
/// </summary>
|
|
public const string Snowflake = "\U00002744";
|
|
|
|
/// <summary>
|
|
/// Gets the "snowman" emoji.
|
|
/// Description: Snowman.
|
|
/// </summary>
|
|
public const string Snowman = "\U00002603";
|
|
|
|
/// <summary>
|
|
/// Gets the "snowman_without_snow" emoji.
|
|
/// Description: Snowman without snow.
|
|
/// </summary>
|
|
public const string SnowmanWithoutSnow = "\U000026C4";
|
|
|
|
/// <summary>
|
|
/// Gets the "soap" emoji.
|
|
/// Description: Soap.
|
|
/// </summary>
|
|
public const string Soap = "\U0001F9FC";
|
|
|
|
/// <summary>
|
|
/// Gets the "soccer_ball" emoji.
|
|
/// Description: Soccer ball.
|
|
/// </summary>
|
|
public const string SoccerBall = "\U000026BD";
|
|
|
|
/// <summary>
|
|
/// Gets the "socks" emoji.
|
|
/// Description: Socks.
|
|
/// </summary>
|
|
public const string Socks = "\U0001F9E6";
|
|
|
|
/// <summary>
|
|
/// Gets the "softball" emoji.
|
|
/// Description: Softball.
|
|
/// </summary>
|
|
public const string Softball = "\U0001F94E";
|
|
|
|
/// <summary>
|
|
/// Gets the "soft_ice_cream" emoji.
|
|
/// Description: Soft ice cream.
|
|
/// </summary>
|
|
public const string SoftIceCream = "\U0001F366";
|
|
|
|
/// <summary>
|
|
/// Gets the "soon_arrow" emoji.
|
|
/// Description: SOON arrow.
|
|
/// </summary>
|
|
public const string SoonArrow = "\U0001F51C";
|
|
|
|
/// <summary>
|
|
/// Gets the "sos_button" emoji.
|
|
/// Description: SOS button.
|
|
/// </summary>
|
|
public const string SosButton = "\U0001F198";
|
|
|
|
/// <summary>
|
|
/// Gets the "spade_suit" emoji.
|
|
/// Description: Spade suit.
|
|
/// </summary>
|
|
public const string SpadeSuit = "\U00002660";
|
|
|
|
/// <summary>
|
|
/// Gets the "spaghetti" emoji.
|
|
/// Description: Spaghetti.
|
|
/// </summary>
|
|
public const string Spaghetti = "\U0001F35D";
|
|
|
|
/// <summary>
|
|
/// Gets the "sparkle" emoji.
|
|
/// Description: Sparkle.
|
|
/// </summary>
|
|
public const string Sparkle = "\U00002747";
|
|
|
|
/// <summary>
|
|
/// Gets the "sparkler" emoji.
|
|
/// Description: Sparkler.
|
|
/// </summary>
|
|
public const string Sparkler = "\U0001F387";
|
|
|
|
/// <summary>
|
|
/// Gets the "sparkles" emoji.
|
|
/// Description: Sparkles.
|
|
/// </summary>
|
|
public const string Sparkles = "\U00002728";
|
|
|
|
/// <summary>
|
|
/// Gets the "sparkling_heart" emoji.
|
|
/// Description: Sparkling heart.
|
|
/// </summary>
|
|
public const string SparklingHeart = "\U0001F496";
|
|
|
|
/// <summary>
|
|
/// Gets the "speaker_high_volume" emoji.
|
|
/// Description: Speaker high volume.
|
|
/// </summary>
|
|
public const string SpeakerHighVolume = "\U0001F50A";
|
|
|
|
/// <summary>
|
|
/// Gets the "speaker_low_volume" emoji.
|
|
/// Description: Speaker low volume.
|
|
/// </summary>
|
|
public const string SpeakerLowVolume = "\U0001F508";
|
|
|
|
/// <summary>
|
|
/// Gets the "speaker_medium_volume" emoji.
|
|
/// Description: Speaker medium volume.
|
|
/// </summary>
|
|
public const string SpeakerMediumVolume = "\U0001F509";
|
|
|
|
/// <summary>
|
|
/// Gets the "speaking_head" emoji.
|
|
/// Description: Speaking head.
|
|
/// </summary>
|
|
public const string SpeakingHead = "\U0001F5E3";
|
|
|
|
/// <summary>
|
|
/// Gets the "speak_no_evil_monkey" emoji.
|
|
/// Description: speak no evil monkey.
|
|
/// </summary>
|
|
public const string SpeakNoEvilMonkey = "\U0001F64A";
|
|
|
|
/// <summary>
|
|
/// Gets the "speech_balloon" emoji.
|
|
/// Description: Speech balloon.
|
|
/// </summary>
|
|
public const string SpeechBalloon = "\U0001F4AC";
|
|
|
|
/// <summary>
|
|
/// Gets the "speedboat" emoji.
|
|
/// Description: Speedboat.
|
|
/// </summary>
|
|
public const string Speedboat = "\U0001F6A4";
|
|
|
|
/// <summary>
|
|
/// Gets the "spider" emoji.
|
|
/// Description: Spider.
|
|
/// </summary>
|
|
public const string Spider = "\U0001F577";
|
|
|
|
/// <summary>
|
|
/// Gets the "spider_web" emoji.
|
|
/// Description: Spider web.
|
|
/// </summary>
|
|
public const string SpiderWeb = "\U0001F578";
|
|
|
|
/// <summary>
|
|
/// Gets the "spiral_calendar" emoji.
|
|
/// Description: Spiral calendar.
|
|
/// </summary>
|
|
public const string SpiralCalendar = "\U0001F5D3";
|
|
|
|
/// <summary>
|
|
/// Gets the "spiral_notepad" emoji.
|
|
/// Description: Spiral notepad.
|
|
/// </summary>
|
|
public const string SpiralNotepad = "\U0001F5D2";
|
|
|
|
/// <summary>
|
|
/// Gets the "spiral_shell" emoji.
|
|
/// Description: Spiral shell.
|
|
/// </summary>
|
|
public const string SpiralShell = "\U0001F41A";
|
|
|
|
/// <summary>
|
|
/// Gets the "sponge" emoji.
|
|
/// Description: Sponge.
|
|
/// </summary>
|
|
public const string Sponge = "\U0001F9FD";
|
|
|
|
/// <summary>
|
|
/// Gets the "spoon" emoji.
|
|
/// Description: Spoon.
|
|
/// </summary>
|
|
public const string Spoon = "\U0001F944";
|
|
|
|
/// <summary>
|
|
/// Gets the "sports_medal" emoji.
|
|
/// Description: Sports medal.
|
|
/// </summary>
|
|
public const string SportsMedal = "\U0001F3C5";
|
|
|
|
/// <summary>
|
|
/// Gets the "sport_utility_vehicle" emoji.
|
|
/// Description: Sport utility vehicle.
|
|
/// </summary>
|
|
public const string SportUtilityVehicle = "\U0001F699";
|
|
|
|
/// <summary>
|
|
/// Gets the "spouting_whale" emoji.
|
|
/// Description: Spouting whale.
|
|
/// </summary>
|
|
public const string SpoutingWhale = "\U0001F433";
|
|
|
|
/// <summary>
|
|
/// Gets the "squid" emoji.
|
|
/// Description: Squid.
|
|
/// </summary>
|
|
public const string Squid = "\U0001F991";
|
|
|
|
/// <summary>
|
|
/// Gets the "squinting_face_with_tongue" emoji.
|
|
/// Description: Squinting face with tongue.
|
|
/// </summary>
|
|
public const string SquintingFaceWithTongue = "\U0001F61D";
|
|
|
|
/// <summary>
|
|
/// Gets the "stadium" emoji.
|
|
/// Description: Stadium.
|
|
/// </summary>
|
|
public const string Stadium = "\U0001F3DF";
|
|
|
|
/// <summary>
|
|
/// Gets the "star" emoji.
|
|
/// Description: Star.
|
|
/// </summary>
|
|
public const string Star = "\U00002B50";
|
|
|
|
/// <summary>
|
|
/// Gets the "star_and_crescent" emoji.
|
|
/// Description: Star and crescent.
|
|
/// </summary>
|
|
public const string StarAndCrescent = "\U0000262A";
|
|
|
|
/// <summary>
|
|
/// Gets the "star_of_david" emoji.
|
|
/// Description: Star of david.
|
|
/// </summary>
|
|
public const string StarOfDavid = "\U00002721";
|
|
|
|
/// <summary>
|
|
/// Gets the "star_struck" emoji.
|
|
/// Description: star struck.
|
|
/// </summary>
|
|
public const string StarStruck = "\U0001F929";
|
|
|
|
/// <summary>
|
|
/// Gets the "station" emoji.
|
|
/// Description: Station.
|
|
/// </summary>
|
|
public const string Station = "\U0001F689";
|
|
|
|
/// <summary>
|
|
/// Gets the "statue_of_liberty" emoji.
|
|
/// Description: Statue of liberty.
|
|
/// </summary>
|
|
public const string StatueOfLiberty = "\U0001F5FD";
|
|
|
|
/// <summary>
|
|
/// Gets the "steaming_bowl" emoji.
|
|
/// Description: Steaming bowl.
|
|
/// </summary>
|
|
public const string SteamingBowl = "\U0001F35C";
|
|
|
|
/// <summary>
|
|
/// Gets the "stethoscope" emoji.
|
|
/// Description: Stethoscope.
|
|
/// </summary>
|
|
public const string Stethoscope = "\U0001FA7A";
|
|
|
|
/// <summary>
|
|
/// Gets the "stop_button" emoji.
|
|
/// Description: Stop button.
|
|
/// </summary>
|
|
public const string StopButton = "\U000023F9";
|
|
|
|
/// <summary>
|
|
/// Gets the "stop_sign" emoji.
|
|
/// Description: Stop sign.
|
|
/// </summary>
|
|
public const string StopSign = "\U0001F6D1";
|
|
|
|
/// <summary>
|
|
/// Gets the "stopwatch" emoji.
|
|
/// Description: Stopwatch.
|
|
/// </summary>
|
|
public const string Stopwatch = "\U000023F1";
|
|
|
|
/// <summary>
|
|
/// Gets the "straight_ruler" emoji.
|
|
/// Description: Straight ruler.
|
|
/// </summary>
|
|
public const string StraightRuler = "\U0001F4CF";
|
|
|
|
/// <summary>
|
|
/// Gets the "strawberry" emoji.
|
|
/// Description: Strawberry.
|
|
/// </summary>
|
|
public const string Strawberry = "\U0001F353";
|
|
|
|
/// <summary>
|
|
/// Gets the "studio_microphone" emoji.
|
|
/// Description: Studio microphone.
|
|
/// </summary>
|
|
public const string StudioMicrophone = "\U0001F399";
|
|
|
|
/// <summary>
|
|
/// Gets the "stuffed_flatbread" emoji.
|
|
/// Description: Stuffed flatbread.
|
|
/// </summary>
|
|
public const string StuffedFlatbread = "\U0001F959";
|
|
|
|
/// <summary>
|
|
/// Gets the "sun" emoji.
|
|
/// Description: Sun.
|
|
/// </summary>
|
|
public const string Sun = "\U00002600";
|
|
|
|
/// <summary>
|
|
/// Gets the "sun_behind_cloud" emoji.
|
|
/// Description: Sun behind cloud.
|
|
/// </summary>
|
|
public const string SunBehindCloud = "\U000026C5";
|
|
|
|
/// <summary>
|
|
/// Gets the "sun_behind_large_cloud" emoji.
|
|
/// Description: Sun behind large cloud.
|
|
/// </summary>
|
|
public const string SunBehindLargeCloud = "\U0001F325";
|
|
|
|
/// <summary>
|
|
/// Gets the "sun_behind_rain_cloud" emoji.
|
|
/// Description: Sun behind rain cloud.
|
|
/// </summary>
|
|
public const string SunBehindRainCloud = "\U0001F326";
|
|
|
|
/// <summary>
|
|
/// Gets the "sun_behind_small_cloud" emoji.
|
|
/// Description: Sun behind small cloud.
|
|
/// </summary>
|
|
public const string SunBehindSmallCloud = "\U0001F324";
|
|
|
|
/// <summary>
|
|
/// Gets the "sunflower" emoji.
|
|
/// Description: Sunflower.
|
|
/// </summary>
|
|
public const string Sunflower = "\U0001F33B";
|
|
|
|
/// <summary>
|
|
/// Gets the "sunglasses" emoji.
|
|
/// Description: Sunglasses.
|
|
/// </summary>
|
|
public const string Sunglasses = "\U0001F576";
|
|
|
|
/// <summary>
|
|
/// Gets the "sunrise" emoji.
|
|
/// Description: Sunrise.
|
|
/// </summary>
|
|
public const string Sunrise = "\U0001F305";
|
|
|
|
/// <summary>
|
|
/// Gets the "sunrise_over_mountains" emoji.
|
|
/// Description: Sunrise over mountains.
|
|
/// </summary>
|
|
public const string SunriseOverMountains = "\U0001F304";
|
|
|
|
/// <summary>
|
|
/// Gets the "sunset" emoji.
|
|
/// Description: Sunset.
|
|
/// </summary>
|
|
public const string Sunset = "\U0001F307";
|
|
|
|
/// <summary>
|
|
/// Gets the "sun_with_face" emoji.
|
|
/// Description: Sun with face.
|
|
/// </summary>
|
|
public const string SunWithFace = "\U0001F31E";
|
|
|
|
/// <summary>
|
|
/// Gets the "superhero" emoji.
|
|
/// Description: Superhero.
|
|
/// </summary>
|
|
public const string Superhero = "\U0001F9B8";
|
|
|
|
/// <summary>
|
|
/// Gets the "supervillain" emoji.
|
|
/// Description: Supervillain.
|
|
/// </summary>
|
|
public const string Supervillain = "\U0001F9B9";
|
|
|
|
/// <summary>
|
|
/// Gets the "sushi" emoji.
|
|
/// Description: Sushi.
|
|
/// </summary>
|
|
public const string Sushi = "\U0001F363";
|
|
|
|
/// <summary>
|
|
/// Gets the "suspension_railway" emoji.
|
|
/// Description: Suspension railway.
|
|
/// </summary>
|
|
public const string SuspensionRailway = "\U0001F69F";
|
|
|
|
/// <summary>
|
|
/// Gets the "swan" emoji.
|
|
/// Description: Swan.
|
|
/// </summary>
|
|
public const string Swan = "\U0001F9A2";
|
|
|
|
/// <summary>
|
|
/// Gets the "sweat_droplets" emoji.
|
|
/// Description: Sweat droplets.
|
|
/// </summary>
|
|
public const string SweatDroplets = "\U0001F4A6";
|
|
|
|
/// <summary>
|
|
/// Gets the "synagogue" emoji.
|
|
/// Description: Synagogue.
|
|
/// </summary>
|
|
public const string Synagogue = "\U0001F54D";
|
|
|
|
/// <summary>
|
|
/// Gets the "syringe" emoji.
|
|
/// Description: Syringe.
|
|
/// </summary>
|
|
public const string Syringe = "\U0001F489";
|
|
|
|
/// <summary>
|
|
/// Gets the "taco" emoji.
|
|
/// Description: Taco.
|
|
/// </summary>
|
|
public const string Taco = "\U0001F32E";
|
|
|
|
/// <summary>
|
|
/// Gets the "takeout_box" emoji.
|
|
/// Description: Takeout box.
|
|
/// </summary>
|
|
public const string TakeoutBox = "\U0001F961";
|
|
|
|
/// <summary>
|
|
/// Gets the "tamale" emoji.
|
|
/// Description: Tamale.
|
|
/// </summary>
|
|
public const string Tamale = "\U0001FAD4";
|
|
|
|
/// <summary>
|
|
/// Gets the "tanabata_tree" emoji.
|
|
/// Description: Tanabata tree.
|
|
/// </summary>
|
|
public const string TanabataTree = "\U0001F38B";
|
|
|
|
/// <summary>
|
|
/// Gets the "tangerine" emoji.
|
|
/// Description: Tangerine.
|
|
/// </summary>
|
|
public const string Tangerine = "\U0001F34A";
|
|
|
|
/// <summary>
|
|
/// Gets the "taurus" emoji.
|
|
/// Description: Taurus.
|
|
/// </summary>
|
|
public const string Taurus = "\U00002649";
|
|
|
|
/// <summary>
|
|
/// Gets the "taxi" emoji.
|
|
/// Description: Taxi.
|
|
/// </summary>
|
|
public const string Taxi = "\U0001F695";
|
|
|
|
/// <summary>
|
|
/// Gets the "teacup_without_handle" emoji.
|
|
/// Description: Teacup without handle.
|
|
/// </summary>
|
|
public const string TeacupWithoutHandle = "\U0001F375";
|
|
|
|
/// <summary>
|
|
/// Gets the "teapot" emoji.
|
|
/// Description: Teapot.
|
|
/// </summary>
|
|
public const string Teapot = "\U0001FAD6";
|
|
|
|
/// <summary>
|
|
/// Gets the "tear_off_calendar" emoji.
|
|
/// Description: tear off calendar.
|
|
/// </summary>
|
|
public const string TearOffCalendar = "\U0001F4C6";
|
|
|
|
/// <summary>
|
|
/// Gets the "teddy_bear" emoji.
|
|
/// Description: Teddy bear.
|
|
/// </summary>
|
|
public const string TeddyBear = "\U0001F9F8";
|
|
|
|
/// <summary>
|
|
/// Gets the "telephone" emoji.
|
|
/// Description: Telephone.
|
|
/// </summary>
|
|
public const string Telephone = "\U0000260E";
|
|
|
|
/// <summary>
|
|
/// Gets the "telephone_receiver" emoji.
|
|
/// Description: Telephone receiver.
|
|
/// </summary>
|
|
public const string TelephoneReceiver = "\U0001F4DE";
|
|
|
|
/// <summary>
|
|
/// Gets the "telescope" emoji.
|
|
/// Description: Telescope.
|
|
/// </summary>
|
|
public const string Telescope = "\U0001F52D";
|
|
|
|
/// <summary>
|
|
/// Gets the "television" emoji.
|
|
/// Description: Television.
|
|
/// </summary>
|
|
public const string Television = "\U0001F4FA";
|
|
|
|
/// <summary>
|
|
/// Gets the "tennis" emoji.
|
|
/// Description: Tennis.
|
|
/// </summary>
|
|
public const string Tennis = "\U0001F3BE";
|
|
|
|
/// <summary>
|
|
/// Gets the "ten_o_clock" emoji.
|
|
/// Description: Ten o clock.
|
|
/// </summary>
|
|
public const string TenOClock = "\U0001F559";
|
|
|
|
/// <summary>
|
|
/// Gets the "tent" emoji.
|
|
/// Description: Tent.
|
|
/// </summary>
|
|
public const string Tent = "\U000026FA";
|
|
|
|
/// <summary>
|
|
/// Gets the "ten_thirty" emoji.
|
|
/// Description: ten thirty.
|
|
/// </summary>
|
|
public const string TenThirty = "\U0001F565";
|
|
|
|
/// <summary>
|
|
/// Gets the "test_tube" emoji.
|
|
/// Description: Test tube.
|
|
/// </summary>
|
|
public const string TestTube = "\U0001F9EA";
|
|
|
|
/// <summary>
|
|
/// Gets the "thermometer" emoji.
|
|
/// Description: Thermometer.
|
|
/// </summary>
|
|
public const string Thermometer = "\U0001F321";
|
|
|
|
/// <summary>
|
|
/// Gets the "thinking_face" emoji.
|
|
/// Description: Thinking face.
|
|
/// </summary>
|
|
public const string ThinkingFace = "\U0001F914";
|
|
|
|
/// <summary>
|
|
/// Gets the "3rd_place_medal" emoji.
|
|
/// Description: 3rd place medal.
|
|
/// </summary>
|
|
public const string ThirdPlaceMedal = "\U0001F949";
|
|
|
|
/// <summary>
|
|
/// Gets the "thong_sandal" emoji.
|
|
/// Description: Thong sandal.
|
|
/// </summary>
|
|
public const string ThongSandal = "\U0001FA74";
|
|
|
|
/// <summary>
|
|
/// Gets the "thought_balloon" emoji.
|
|
/// Description: Thought balloon.
|
|
/// </summary>
|
|
public const string ThoughtBalloon = "\U0001F4AD";
|
|
|
|
/// <summary>
|
|
/// Gets the "thread" emoji.
|
|
/// Description: Thread.
|
|
/// </summary>
|
|
public const string Thread = "\U0001F9F5";
|
|
|
|
/// <summary>
|
|
/// Gets the "three_o_clock" emoji.
|
|
/// Description: Three o clock.
|
|
/// </summary>
|
|
public const string ThreeOClock = "\U0001F552";
|
|
|
|
/// <summary>
|
|
/// Gets the "three_thirty" emoji.
|
|
/// Description: three thirty.
|
|
/// </summary>
|
|
public const string ThreeThirty = "\U0001F55E";
|
|
|
|
/// <summary>
|
|
/// Gets the "thumbs_down" emoji.
|
|
/// Description: Thumbs down.
|
|
/// </summary>
|
|
public const string ThumbsDown = "\U0001F44E";
|
|
|
|
/// <summary>
|
|
/// Gets the "thumbs_up" emoji.
|
|
/// Description: Thumbs up.
|
|
/// </summary>
|
|
public const string ThumbsUp = "\U0001F44D";
|
|
|
|
/// <summary>
|
|
/// Gets the "ticket" emoji.
|
|
/// Description: Ticket.
|
|
/// </summary>
|
|
public const string Ticket = "\U0001F3AB";
|
|
|
|
/// <summary>
|
|
/// Gets the "tiger" emoji.
|
|
/// Description: Tiger.
|
|
/// </summary>
|
|
public const string Tiger = "\U0001F405";
|
|
|
|
/// <summary>
|
|
/// Gets the "tiger_face" emoji.
|
|
/// Description: Tiger face.
|
|
/// </summary>
|
|
public const string TigerFace = "\U0001F42F";
|
|
|
|
/// <summary>
|
|
/// Gets the "timer_clock" emoji.
|
|
/// Description: Timer clock.
|
|
/// </summary>
|
|
public const string TimerClock = "\U000023F2";
|
|
|
|
/// <summary>
|
|
/// Gets the "tired_face" emoji.
|
|
/// Description: Tired face.
|
|
/// </summary>
|
|
public const string TiredFace = "\U0001F62B";
|
|
|
|
/// <summary>
|
|
/// Gets the "toilet" emoji.
|
|
/// Description: Toilet.
|
|
/// </summary>
|
|
public const string Toilet = "\U0001F6BD";
|
|
|
|
/// <summary>
|
|
/// Gets the "tokyo_tower" emoji.
|
|
/// Description: Tokyo tower.
|
|
/// </summary>
|
|
public const string TokyoTower = "\U0001F5FC";
|
|
|
|
/// <summary>
|
|
/// Gets the "tomato" emoji.
|
|
/// Description: Tomato.
|
|
/// </summary>
|
|
public const string Tomato = "\U0001F345";
|
|
|
|
/// <summary>
|
|
/// Gets the "tongue" emoji.
|
|
/// Description: Tongue.
|
|
/// </summary>
|
|
public const string Tongue = "\U0001F445";
|
|
|
|
/// <summary>
|
|
/// Gets the "toolbox" emoji.
|
|
/// Description: Toolbox.
|
|
/// </summary>
|
|
public const string Toolbox = "\U0001F9F0";
|
|
|
|
/// <summary>
|
|
/// Gets the "tooth" emoji.
|
|
/// Description: Tooth.
|
|
/// </summary>
|
|
public const string Tooth = "\U0001F9B7";
|
|
|
|
/// <summary>
|
|
/// Gets the "toothbrush" emoji.
|
|
/// Description: Toothbrush.
|
|
/// </summary>
|
|
public const string Toothbrush = "\U0001FAA5";
|
|
|
|
/// <summary>
|
|
/// Gets the "top_arrow" emoji.
|
|
/// Description: TOP arrow.
|
|
/// </summary>
|
|
public const string TopArrow = "\U0001F51D";
|
|
|
|
/// <summary>
|
|
/// Gets the "top_hat" emoji.
|
|
/// Description: Top hat.
|
|
/// </summary>
|
|
public const string TopHat = "\U0001F3A9";
|
|
|
|
/// <summary>
|
|
/// Gets the "tornado" emoji.
|
|
/// Description: Tornado.
|
|
/// </summary>
|
|
public const string Tornado = "\U0001F32A";
|
|
|
|
/// <summary>
|
|
/// Gets the "trackball" emoji.
|
|
/// Description: Trackball.
|
|
/// </summary>
|
|
public const string Trackball = "\U0001F5B2";
|
|
|
|
/// <summary>
|
|
/// Gets the "tractor" emoji.
|
|
/// Description: Tractor.
|
|
/// </summary>
|
|
public const string Tractor = "\U0001F69C";
|
|
|
|
/// <summary>
|
|
/// Gets the "trade_mark" emoji.
|
|
/// Description: Trade mark.
|
|
/// </summary>
|
|
public const string TradeMark = "\U00002122";
|
|
|
|
/// <summary>
|
|
/// Gets the "train" emoji.
|
|
/// Description: Train.
|
|
/// </summary>
|
|
public const string Train = "\U0001F686";
|
|
|
|
/// <summary>
|
|
/// Gets the "tram" emoji.
|
|
/// Description: Tram.
|
|
/// </summary>
|
|
public const string Tram = "\U0001F68A";
|
|
|
|
/// <summary>
|
|
/// Gets the "tram_car" emoji.
|
|
/// Description: Tram car.
|
|
/// </summary>
|
|
public const string TramCar = "\U0001F68B";
|
|
|
|
/// <summary>
|
|
/// Gets the "transgender_symbol" emoji.
|
|
/// Description: Transgender symbol.
|
|
/// </summary>
|
|
public const string TransgenderSymbol = "\U000026A7";
|
|
|
|
/// <summary>
|
|
/// Gets the "t_rex" emoji.
|
|
/// Description: T Rex.
|
|
/// </summary>
|
|
public const string TRex = "\U0001F996";
|
|
|
|
/// <summary>
|
|
/// Gets the "triangular_flag" emoji.
|
|
/// Description: Triangular flag.
|
|
/// </summary>
|
|
public const string TriangularFlag = "\U0001F6A9";
|
|
|
|
/// <summary>
|
|
/// Gets the "triangular_ruler" emoji.
|
|
/// Description: Triangular ruler.
|
|
/// </summary>
|
|
public const string TriangularRuler = "\U0001F4D0";
|
|
|
|
/// <summary>
|
|
/// Gets the "trident_emblem" emoji.
|
|
/// Description: Trident emblem.
|
|
/// </summary>
|
|
public const string TridentEmblem = "\U0001F531";
|
|
|
|
/// <summary>
|
|
/// Gets the "trolleybus" emoji.
|
|
/// Description: Trolleybus.
|
|
/// </summary>
|
|
public const string Trolleybus = "\U0001F68E";
|
|
|
|
/// <summary>
|
|
/// Gets the "trophy" emoji.
|
|
/// Description: Trophy.
|
|
/// </summary>
|
|
public const string Trophy = "\U0001F3C6";
|
|
|
|
/// <summary>
|
|
/// Gets the "tropical_drink" emoji.
|
|
/// Description: Tropical drink.
|
|
/// </summary>
|
|
public const string TropicalDrink = "\U0001F379";
|
|
|
|
/// <summary>
|
|
/// Gets the "tropical_fish" emoji.
|
|
/// Description: Tropical fish.
|
|
/// </summary>
|
|
public const string TropicalFish = "\U0001F420";
|
|
|
|
/// <summary>
|
|
/// Gets the "trumpet" emoji.
|
|
/// Description: Trumpet.
|
|
/// </summary>
|
|
public const string Trumpet = "\U0001F3BA";
|
|
|
|
/// <summary>
|
|
/// Gets the "t_shirt" emoji.
|
|
/// Description: t shirt.
|
|
/// </summary>
|
|
public const string TShirt = "\U0001F455";
|
|
|
|
/// <summary>
|
|
/// Gets the "tulip" emoji.
|
|
/// Description: Tulip.
|
|
/// </summary>
|
|
public const string Tulip = "\U0001F337";
|
|
|
|
/// <summary>
|
|
/// Gets the "tumbler_glass" emoji.
|
|
/// Description: Tumbler glass.
|
|
/// </summary>
|
|
public const string TumblerGlass = "\U0001F943";
|
|
|
|
/// <summary>
|
|
/// Gets the "turkey" emoji.
|
|
/// Description: Turkey.
|
|
/// </summary>
|
|
public const string Turkey = "\U0001F983";
|
|
|
|
/// <summary>
|
|
/// Gets the "turtle" emoji.
|
|
/// Description: Turtle.
|
|
/// </summary>
|
|
public const string Turtle = "\U0001F422";
|
|
|
|
/// <summary>
|
|
/// Gets the "twelve_o_clock" emoji.
|
|
/// Description: Twelve o clock.
|
|
/// </summary>
|
|
public const string TwelveOClock = "\U0001F55B";
|
|
|
|
/// <summary>
|
|
/// Gets the "twelve_thirty" emoji.
|
|
/// Description: twelve thirty.
|
|
/// </summary>
|
|
public const string TwelveThirty = "\U0001F567";
|
|
|
|
/// <summary>
|
|
/// Gets the "two_hearts" emoji.
|
|
/// Description: Two hearts.
|
|
/// </summary>
|
|
public const string TwoHearts = "\U0001F495";
|
|
|
|
/// <summary>
|
|
/// Gets the "two_hump_camel" emoji.
|
|
/// Description: two hump camel.
|
|
/// </summary>
|
|
public const string TwoHumpCamel = "\U0001F42B";
|
|
|
|
/// <summary>
|
|
/// Gets the "two_o_clock" emoji.
|
|
/// Description: Two o clock.
|
|
/// </summary>
|
|
public const string TwoOClock = "\U0001F551";
|
|
|
|
/// <summary>
|
|
/// Gets the "two_thirty" emoji.
|
|
/// Description: two thirty.
|
|
/// </summary>
|
|
public const string TwoThirty = "\U0001F55D";
|
|
|
|
/// <summary>
|
|
/// Gets the "umbrella" emoji.
|
|
/// Description: Umbrella.
|
|
/// </summary>
|
|
public const string Umbrella = "\U00002602";
|
|
|
|
/// <summary>
|
|
/// Gets the "umbrella_on_ground" emoji.
|
|
/// Description: Umbrella on ground.
|
|
/// </summary>
|
|
public const string UmbrellaOnGround = "\U000026F1";
|
|
|
|
/// <summary>
|
|
/// Gets the "umbrella_with_rain_drops" emoji.
|
|
/// Description: Umbrella with rain drops.
|
|
/// </summary>
|
|
public const string UmbrellaWithRainDrops = "\U00002614";
|
|
|
|
/// <summary>
|
|
/// Gets the "unamused_face" emoji.
|
|
/// Description: Unamused face.
|
|
/// </summary>
|
|
public const string UnamusedFace = "\U0001F612";
|
|
|
|
/// <summary>
|
|
/// Gets the "unicorn" emoji.
|
|
/// Description: Unicorn.
|
|
/// </summary>
|
|
public const string Unicorn = "\U0001F984";
|
|
|
|
/// <summary>
|
|
/// Gets the "unlocked" emoji.
|
|
/// Description: Unlocked.
|
|
/// </summary>
|
|
public const string Unlocked = "\U0001F513";
|
|
|
|
/// <summary>
|
|
/// Gets the "up_arrow" emoji.
|
|
/// Description: Up arrow.
|
|
/// </summary>
|
|
public const string UpArrow = "\U00002B06";
|
|
|
|
/// <summary>
|
|
/// Gets the "up_button" emoji.
|
|
/// Description: UP button.
|
|
/// </summary>
|
|
public const string UpButton = "\U0001F199";
|
|
|
|
/// <summary>
|
|
/// Gets the "up_down_arrow" emoji.
|
|
/// Description: up down arrow.
|
|
/// </summary>
|
|
public const string UpDownArrow = "\U00002195";
|
|
|
|
/// <summary>
|
|
/// Gets the "up_left_arrow" emoji.
|
|
/// Description: up left arrow.
|
|
/// </summary>
|
|
public const string UpLeftArrow = "\U00002196";
|
|
|
|
/// <summary>
|
|
/// Gets the "up_right_arrow" emoji.
|
|
/// Description: up right arrow.
|
|
/// </summary>
|
|
public const string UpRightArrow = "\U00002197";
|
|
|
|
/// <summary>
|
|
/// Gets the "upside_down_face" emoji.
|
|
/// Description: upside down face.
|
|
/// </summary>
|
|
public const string UpsideDownFace = "\U0001F643";
|
|
|
|
/// <summary>
|
|
/// Gets the "upwards_button" emoji.
|
|
/// Description: Upwards button.
|
|
/// </summary>
|
|
public const string UpwardsButton = "\U0001F53C";
|
|
|
|
/// <summary>
|
|
/// Gets the "vampire" emoji.
|
|
/// Description: Vampire.
|
|
/// </summary>
|
|
public const string Vampire = "\U0001F9DB";
|
|
|
|
/// <summary>
|
|
/// Gets the "vertical_traffic_light" emoji.
|
|
/// Description: Vertical traffic light.
|
|
/// </summary>
|
|
public const string VerticalTrafficLight = "\U0001F6A6";
|
|
|
|
/// <summary>
|
|
/// Gets the "vibration_mode" emoji.
|
|
/// Description: Vibration mode.
|
|
/// </summary>
|
|
public const string VibrationMode = "\U0001F4F3";
|
|
|
|
/// <summary>
|
|
/// Gets the "victory_hand" emoji.
|
|
/// Description: Victory hand.
|
|
/// </summary>
|
|
public const string VictoryHand = "\U0000270C";
|
|
|
|
/// <summary>
|
|
/// Gets the "video_camera" emoji.
|
|
/// Description: Video camera.
|
|
/// </summary>
|
|
public const string VideoCamera = "\U0001F4F9";
|
|
|
|
/// <summary>
|
|
/// Gets the "videocassette" emoji.
|
|
/// Description: Videocassette.
|
|
/// </summary>
|
|
public const string Videocassette = "\U0001F4FC";
|
|
|
|
/// <summary>
|
|
/// Gets the "video_game" emoji.
|
|
/// Description: Video game.
|
|
/// </summary>
|
|
public const string VideoGame = "\U0001F3AE";
|
|
|
|
/// <summary>
|
|
/// Gets the "violin" emoji.
|
|
/// Description: Violin.
|
|
/// </summary>
|
|
public const string Violin = "\U0001F3BB";
|
|
|
|
/// <summary>
|
|
/// Gets the "virgo" emoji.
|
|
/// Description: Virgo.
|
|
/// </summary>
|
|
public const string Virgo = "\U0000264D";
|
|
|
|
/// <summary>
|
|
/// Gets the "volcano" emoji.
|
|
/// Description: Volcano.
|
|
/// </summary>
|
|
public const string Volcano = "\U0001F30B";
|
|
|
|
/// <summary>
|
|
/// Gets the "volleyball" emoji.
|
|
/// Description: Volleyball.
|
|
/// </summary>
|
|
public const string Volleyball = "\U0001F3D0";
|
|
|
|
/// <summary>
|
|
/// Gets the "vs_button" emoji.
|
|
/// Description: VS button.
|
|
/// </summary>
|
|
public const string VsButton = "\U0001F19A";
|
|
|
|
/// <summary>
|
|
/// Gets the "vulcan_salute" emoji.
|
|
/// Description: Vulcan salute.
|
|
/// </summary>
|
|
public const string VulcanSalute = "\U0001F596";
|
|
|
|
/// <summary>
|
|
/// Gets the "waffle" emoji.
|
|
/// Description: Waffle.
|
|
/// </summary>
|
|
public const string Waffle = "\U0001F9C7";
|
|
|
|
/// <summary>
|
|
/// Gets the "waning_crescent_moon" emoji.
|
|
/// Description: Waning crescent moon.
|
|
/// </summary>
|
|
public const string WaningCrescentMoon = "\U0001F318";
|
|
|
|
/// <summary>
|
|
/// Gets the "waning_gibbous_moon" emoji.
|
|
/// Description: Waning gibbous moon.
|
|
/// </summary>
|
|
public const string WaningGibbousMoon = "\U0001F316";
|
|
|
|
/// <summary>
|
|
/// Gets the "warning" emoji.
|
|
/// Description: Warning.
|
|
/// </summary>
|
|
public const string Warning = "\U000026A0";
|
|
|
|
/// <summary>
|
|
/// Gets the "wastebasket" emoji.
|
|
/// Description: Wastebasket.
|
|
/// </summary>
|
|
public const string Wastebasket = "\U0001F5D1";
|
|
|
|
/// <summary>
|
|
/// Gets the "watch" emoji.
|
|
/// Description: Watch.
|
|
/// </summary>
|
|
public const string Watch = "\U0000231A";
|
|
|
|
/// <summary>
|
|
/// Gets the "water_buffalo" emoji.
|
|
/// Description: Water buffalo.
|
|
/// </summary>
|
|
public const string WaterBuffalo = "\U0001F403";
|
|
|
|
/// <summary>
|
|
/// Gets the "water_closet" emoji.
|
|
/// Description: Water closet.
|
|
/// </summary>
|
|
public const string WaterCloset = "\U0001F6BE";
|
|
|
|
/// <summary>
|
|
/// Gets the "watermelon" emoji.
|
|
/// Description: Watermelon.
|
|
/// </summary>
|
|
public const string Watermelon = "\U0001F349";
|
|
|
|
/// <summary>
|
|
/// Gets the "water_pistol" emoji.
|
|
/// Description: Water pistol.
|
|
/// </summary>
|
|
public const string WaterPistol = "\U0001F52B";
|
|
|
|
/// <summary>
|
|
/// Gets the "water_wave" emoji.
|
|
/// Description: Water wave.
|
|
/// </summary>
|
|
public const string WaterWave = "\U0001F30A";
|
|
|
|
/// <summary>
|
|
/// Gets the "waving_hand" emoji.
|
|
/// Description: Waving hand.
|
|
/// </summary>
|
|
public const string WavingHand = "\U0001F44B";
|
|
|
|
/// <summary>
|
|
/// Gets the "wavy_dash" emoji.
|
|
/// Description: Wavy dash.
|
|
/// </summary>
|
|
public const string WavyDash = "\U00003030";
|
|
|
|
/// <summary>
|
|
/// Gets the "waxing_crescent_moon" emoji.
|
|
/// Description: Waxing crescent moon.
|
|
/// </summary>
|
|
public const string WaxingCrescentMoon = "\U0001F312";
|
|
|
|
/// <summary>
|
|
/// Gets the "waxing_gibbous_moon" emoji.
|
|
/// Description: Waxing gibbous moon.
|
|
/// </summary>
|
|
public const string WaxingGibbousMoon = "\U0001F314";
|
|
|
|
/// <summary>
|
|
/// Gets the "weary_cat" emoji.
|
|
/// Description: Weary cat.
|
|
/// </summary>
|
|
public const string WearyCat = "\U0001F640";
|
|
|
|
/// <summary>
|
|
/// Gets the "weary_face" emoji.
|
|
/// Description: Weary face.
|
|
/// </summary>
|
|
public const string WearyFace = "\U0001F629";
|
|
|
|
/// <summary>
|
|
/// Gets the "wedding" emoji.
|
|
/// Description: Wedding.
|
|
/// </summary>
|
|
public const string Wedding = "\U0001F492";
|
|
|
|
/// <summary>
|
|
/// Gets the "whale" emoji.
|
|
/// Description: Whale.
|
|
/// </summary>
|
|
public const string Whale = "\U0001F40B";
|
|
|
|
/// <summary>
|
|
/// Gets the "wheelchair_symbol" emoji.
|
|
/// Description: Wheelchair symbol.
|
|
/// </summary>
|
|
public const string WheelchairSymbol = "\U0000267F";
|
|
|
|
/// <summary>
|
|
/// Gets the "wheel_of_dharma" emoji.
|
|
/// Description: Wheel of dharma.
|
|
/// </summary>
|
|
public const string WheelOfDharma = "\U00002638";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_cane" emoji.
|
|
/// Description: White cane.
|
|
/// </summary>
|
|
public const string WhiteCane = "\U0001F9AF";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_circle" emoji.
|
|
/// Description: White circle.
|
|
/// </summary>
|
|
public const string WhiteCircle = "\U000026AA";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_exclamation_mark" emoji.
|
|
/// Description: White exclamation mark.
|
|
/// </summary>
|
|
public const string WhiteExclamationMark = "\U00002755";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_flag" emoji.
|
|
/// Description: White flag.
|
|
/// </summary>
|
|
public const string WhiteFlag = "\U0001F3F3";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_flower" emoji.
|
|
/// Description: White flower.
|
|
/// </summary>
|
|
public const string WhiteFlower = "\U0001F4AE";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_hair" emoji.
|
|
/// Description: White hair.
|
|
/// </summary>
|
|
public const string WhiteHair = "\U0001F9B3";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_heart" emoji.
|
|
/// Description: White heart.
|
|
/// </summary>
|
|
public const string WhiteHeart = "\U0001F90D";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_large_square" emoji.
|
|
/// Description: White large square.
|
|
/// </summary>
|
|
public const string WhiteLargeSquare = "\U00002B1C";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_medium_small_square" emoji.
|
|
/// Description: white medium small square.
|
|
/// </summary>
|
|
public const string WhiteMediumSmallSquare = "\U000025FD";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_medium_square" emoji.
|
|
/// Description: White medium square.
|
|
/// </summary>
|
|
public const string WhiteMediumSquare = "\U000025FB";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_question_mark" emoji.
|
|
/// Description: White question mark.
|
|
/// </summary>
|
|
public const string WhiteQuestionMark = "\U00002754";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_small_square" emoji.
|
|
/// Description: White small square.
|
|
/// </summary>
|
|
public const string WhiteSmallSquare = "\U000025AB";
|
|
|
|
/// <summary>
|
|
/// Gets the "white_square_button" emoji.
|
|
/// Description: White square button.
|
|
/// </summary>
|
|
public const string WhiteSquareButton = "\U0001F533";
|
|
|
|
/// <summary>
|
|
/// Gets the "wilted_flower" emoji.
|
|
/// Description: Wilted flower.
|
|
/// </summary>
|
|
public const string WiltedFlower = "\U0001F940";
|
|
|
|
/// <summary>
|
|
/// Gets the "wind_chime" emoji.
|
|
/// Description: Wind chime.
|
|
/// </summary>
|
|
public const string WindChime = "\U0001F390";
|
|
|
|
/// <summary>
|
|
/// Gets the "wind_face" emoji.
|
|
/// Description: Wind face.
|
|
/// </summary>
|
|
public const string WindFace = "\U0001F32C";
|
|
|
|
/// <summary>
|
|
/// Gets the "window" emoji.
|
|
/// Description: Window.
|
|
/// </summary>
|
|
public const string Window = "\U0001FA9F";
|
|
|
|
/// <summary>
|
|
/// Gets the "wine_glass" emoji.
|
|
/// Description: Wine glass.
|
|
/// </summary>
|
|
public const string WineGlass = "\U0001F377";
|
|
|
|
/// <summary>
|
|
/// Gets the "winking_face" emoji.
|
|
/// Description: Winking face.
|
|
/// </summary>
|
|
public const string WinkingFace = "\U0001F609";
|
|
|
|
/// <summary>
|
|
/// Gets the "winking_face_with_tongue" emoji.
|
|
/// Description: Winking face with tongue.
|
|
/// </summary>
|
|
public const string WinkingFaceWithTongue = "\U0001F61C";
|
|
|
|
/// <summary>
|
|
/// Gets the "wolf" emoji.
|
|
/// Description: Wolf.
|
|
/// </summary>
|
|
public const string Wolf = "\U0001F43A";
|
|
|
|
/// <summary>
|
|
/// Gets the "woman" emoji.
|
|
/// Description: Woman.
|
|
/// </summary>
|
|
public const string Woman = "\U0001F469";
|
|
|
|
/// <summary>
|
|
/// Gets the "woman_and_man_holding_hands" emoji.
|
|
/// Description: Woman and man holding hands.
|
|
/// </summary>
|
|
public const string WomanAndManHoldingHands = "\U0001F46B";
|
|
|
|
/// <summary>
|
|
/// Gets the "woman_dancing" emoji.
|
|
/// Description: Woman dancing.
|
|
/// </summary>
|
|
public const string WomanDancing = "\U0001F483";
|
|
|
|
/// <summary>
|
|
/// Gets the "womans_boot" emoji.
|
|
/// Description: Woman s boot.
|
|
/// </summary>
|
|
public const string WomansBoot = "\U0001F462";
|
|
|
|
/// <summary>
|
|
/// Gets the "womans_clothes" emoji.
|
|
/// Description: Woman s clothes.
|
|
/// </summary>
|
|
public const string WomansClothes = "\U0001F45A";
|
|
|
|
/// <summary>
|
|
/// Gets the "womans_hat" emoji.
|
|
/// Description: Woman s hat.
|
|
/// </summary>
|
|
public const string WomansHat = "\U0001F452";
|
|
|
|
/// <summary>
|
|
/// Gets the "womans_sandal" emoji.
|
|
/// Description: Woman s sandal.
|
|
/// </summary>
|
|
public const string WomansSandal = "\U0001F461";
|
|
|
|
/// <summary>
|
|
/// Gets the "woman_with_headscarf" emoji.
|
|
/// Description: Woman with headscarf.
|
|
/// </summary>
|
|
public const string WomanWithHeadscarf = "\U0001F9D5";
|
|
|
|
/// <summary>
|
|
/// Gets the "women_holding_hands" emoji.
|
|
/// Description: Women holding hands.
|
|
/// </summary>
|
|
public const string WomenHoldingHands = "\U0001F46D";
|
|
|
|
/// <summary>
|
|
/// Gets the "womens_room" emoji.
|
|
/// Description: Women s room.
|
|
/// </summary>
|
|
public const string WomensRoom = "\U0001F6BA";
|
|
|
|
/// <summary>
|
|
/// Gets the "wood" emoji.
|
|
/// Description: Wood.
|
|
/// </summary>
|
|
public const string Wood = "\U0001FAB5";
|
|
|
|
/// <summary>
|
|
/// Gets the "woozy_face" emoji.
|
|
/// Description: Woozy face.
|
|
/// </summary>
|
|
public const string WoozyFace = "\U0001F974";
|
|
|
|
/// <summary>
|
|
/// Gets the "world_map" emoji.
|
|
/// Description: World map.
|
|
/// </summary>
|
|
public const string WorldMap = "\U0001F5FA";
|
|
|
|
/// <summary>
|
|
/// Gets the "worm" emoji.
|
|
/// Description: Worm.
|
|
/// </summary>
|
|
public const string Worm = "\U0001FAB1";
|
|
|
|
/// <summary>
|
|
/// Gets the "worried_face" emoji.
|
|
/// Description: Worried face.
|
|
/// </summary>
|
|
public const string WorriedFace = "\U0001F61F";
|
|
|
|
/// <summary>
|
|
/// Gets the "wrapped_gift" emoji.
|
|
/// Description: Wrapped gift.
|
|
/// </summary>
|
|
public const string WrappedGift = "\U0001F381";
|
|
|
|
/// <summary>
|
|
/// Gets the "wrench" emoji.
|
|
/// Description: Wrench.
|
|
/// </summary>
|
|
public const string Wrench = "\U0001F527";
|
|
|
|
/// <summary>
|
|
/// Gets the "writing_hand" emoji.
|
|
/// Description: Writing hand.
|
|
/// </summary>
|
|
public const string WritingHand = "\U0000270D";
|
|
|
|
/// <summary>
|
|
/// Gets the "yarn" emoji.
|
|
/// Description: Yarn.
|
|
/// </summary>
|
|
public const string Yarn = "\U0001F9F6";
|
|
|
|
/// <summary>
|
|
/// Gets the "yawning_face" emoji.
|
|
/// Description: Yawning face.
|
|
/// </summary>
|
|
public const string YawningFace = "\U0001F971";
|
|
|
|
/// <summary>
|
|
/// Gets the "yellow_circle" emoji.
|
|
/// Description: Yellow circle.
|
|
/// </summary>
|
|
public const string YellowCircle = "\U0001F7E1";
|
|
|
|
/// <summary>
|
|
/// Gets the "yellow_heart" emoji.
|
|
/// Description: Yellow heart.
|
|
/// </summary>
|
|
public const string YellowHeart = "\U0001F49B";
|
|
|
|
/// <summary>
|
|
/// Gets the "yellow_square" emoji.
|
|
/// Description: Yellow square.
|
|
/// </summary>
|
|
public const string YellowSquare = "\U0001F7E8";
|
|
|
|
/// <summary>
|
|
/// Gets the "yen_banknote" emoji.
|
|
/// Description: Yen banknote.
|
|
/// </summary>
|
|
public const string YenBanknote = "\U0001F4B4";
|
|
|
|
/// <summary>
|
|
/// Gets the "yin_yang" emoji.
|
|
/// Description: Yin yang.
|
|
/// </summary>
|
|
public const string YinYang = "\U0000262F";
|
|
|
|
/// <summary>
|
|
/// Gets the "yo_yo" emoji.
|
|
/// Description: yo yo.
|
|
/// </summary>
|
|
public const string YoYo = "\U0001FA80";
|
|
|
|
/// <summary>
|
|
/// Gets the "zany_face" emoji.
|
|
/// Description: Zany face.
|
|
/// </summary>
|
|
public const string ZanyFace = "\U0001F92A";
|
|
|
|
/// <summary>
|
|
/// Gets the "zebra" emoji.
|
|
/// Description: Zebra.
|
|
/// </summary>
|
|
public const string Zebra = "\U0001F993";
|
|
|
|
/// <summary>
|
|
/// Gets the "zipper_mouth_face" emoji.
|
|
/// Description: zipper mouth face.
|
|
/// </summary>
|
|
public const string ZipperMouthFace = "\U0001F910";
|
|
|
|
/// <summary>
|
|
/// Gets the "zombie" emoji.
|
|
/// Description: Zombie.
|
|
/// </summary>
|
|
public const string Zombie = "\U0001F9DF";
|
|
|
|
/// <summary>
|
|
/// Gets the "zzz" emoji.
|
|
/// Description: Zzz.
|
|
/// </summary>
|
|
public const string Zzz = "\U0001F4A4";
|
|
}
|
|
}
|
|
}
|