mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-19 02:12:49 +08:00
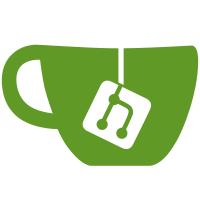
The style parameter is actually nullable. Also, the documentation of the style parameter has been made explicit that `Style.Plain` is used when a `null` style is passed.
79 lines
2.4 KiB
C#
79 lines
2.4 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Diagnostics.CodeAnalysis;
|
|
using Spectre.Console.Rendering;
|
|
|
|
namespace Spectre.Console
|
|
{
|
|
/// <summary>
|
|
/// A renderable piece of text.
|
|
/// </summary>
|
|
[DebuggerDisplay("{_text,nq}")]
|
|
[SuppressMessage("Naming", "CA1724:Type names should not match namespaces")]
|
|
public sealed class Text : Renderable, IAlignable, IOverflowable
|
|
{
|
|
private readonly Paragraph _paragraph;
|
|
|
|
/// <summary>
|
|
/// Gets an empty <see cref="Text"/> instance.
|
|
/// </summary>
|
|
public static Text Empty { get; } = new Text(string.Empty);
|
|
|
|
/// <summary>
|
|
/// Gets an instance of <see cref="Text"/> containing a new line.
|
|
/// </summary>
|
|
public static Text NewLine { get; } = new Text(Environment.NewLine, Style.Plain);
|
|
|
|
/// <summary>
|
|
/// Initializes a new instance of the <see cref="Text"/> class.
|
|
/// </summary>
|
|
/// <param name="text">The text.</param>
|
|
/// <param name="style">The style of the text or <see cref="Style.Plain"/> if <see langword="null"/>.</param>
|
|
public Text(string text, Style? style = null)
|
|
{
|
|
_paragraph = new Paragraph(text, style);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Gets or sets the text alignment.
|
|
/// </summary>
|
|
public Justify? Alignment
|
|
{
|
|
get => _paragraph.Alignment;
|
|
set => _paragraph.Alignment = value;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Gets or sets the text overflow strategy.
|
|
/// </summary>
|
|
public Overflow? Overflow
|
|
{
|
|
get => _paragraph.Overflow;
|
|
set => _paragraph.Overflow = value;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Gets the character count.
|
|
/// </summary>
|
|
public int Length => _paragraph.Length;
|
|
|
|
/// <summary>
|
|
/// Gets the number of lines in the text.
|
|
/// </summary>
|
|
public int Lines => _paragraph.Lines;
|
|
|
|
/// <inheritdoc/>
|
|
protected override Measurement Measure(RenderContext context, int maxWidth)
|
|
{
|
|
return ((IRenderable)_paragraph).Measure(context, maxWidth);
|
|
}
|
|
|
|
/// <inheritdoc/>
|
|
protected override IEnumerable<Segment> Render(RenderContext context, int maxWidth)
|
|
{
|
|
return ((IRenderable)_paragraph).Render(context, maxWidth);
|
|
}
|
|
}
|
|
}
|