mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-27 13:42:51 +08:00
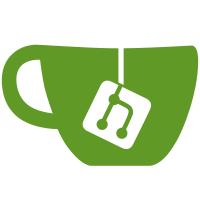
Also moves tests to `./test` which makes it possible for all test projects to share the same .editorconfig files and similar.
36 lines
975 B
C#
36 lines
975 B
C#
using System;
|
|
|
|
namespace Spectre.Console.Tests.Data
|
|
{
|
|
public static class TestExceptions
|
|
{
|
|
public static bool MethodThatThrows(int? number) => throw new InvalidOperationException("Throwing!");
|
|
|
|
public static bool GenericMethodThatThrows<T0, T1, TRet>(int? number) => throw new InvalidOperationException("Throwing!");
|
|
|
|
public static void ThrowWithInnerException()
|
|
{
|
|
try
|
|
{
|
|
MethodThatThrows(null);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new InvalidOperationException("Something threw!", ex);
|
|
}
|
|
}
|
|
|
|
public static void ThrowWithGenericInnerException()
|
|
{
|
|
try
|
|
{
|
|
GenericMethodThatThrows<int, float, double>(null);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new InvalidOperationException("Something threw!", ex);
|
|
}
|
|
}
|
|
}
|
|
}
|