mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-13 15:42:50 +08:00
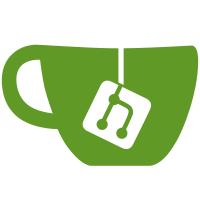
* Adding a dark mode * Adding reference for types to summary pages * Adding API Reference * Adding modifiers to methods/fields/etc * Minimizing files input * Caching a lot of the output pages * Cache only for each execution * Adding API references to existing docs
45 lines
1.2 KiB
C#
45 lines
1.2 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
|
|
namespace Progress;
|
|
|
|
public static class DescriptionGenerator
|
|
{
|
|
private static readonly string[] _verbs = new[] { "Downloading", "Rerouting", "Retriculating", "Collapsing", "Folding", "Solving", "Colliding", "Measuring" };
|
|
private static readonly string[] _nouns = new[] { "internet", "splines", "space", "capacitators", "quarks", "algorithms", "data structures", "spacetime" };
|
|
|
|
private static readonly Random _random;
|
|
private static readonly HashSet<string> _used;
|
|
|
|
static DescriptionGenerator()
|
|
{
|
|
_random = new Random(DateTime.Now.Millisecond);
|
|
_used = new HashSet<string>();
|
|
}
|
|
|
|
public static bool TryGenerate(out string name)
|
|
{
|
|
var iterations = 0;
|
|
while (iterations < 25)
|
|
{
|
|
name = Generate();
|
|
if (!_used.Contains(name))
|
|
{
|
|
_used.Add(name);
|
|
return true;
|
|
}
|
|
|
|
iterations++;
|
|
}
|
|
|
|
name = Generate();
|
|
return false;
|
|
}
|
|
|
|
public static string Generate()
|
|
{
|
|
return _verbs[_random.Next(0, _verbs.Length)]
|
|
+ " " + _nouns[_random.Next(0, _nouns.Length)];
|
|
}
|
|
}
|