mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-16 00:42:51 +08:00
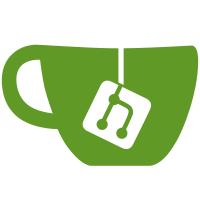
* Add support for C# 12 * Run all tests on all major .NET SDKs * Only build on Ubuntu * Do not build docs for pull requests * Add Cédric Luthi, and Frank Ray to authors * Drop netstandard2.0 for ImageSharp plugin
68 lines
1.8 KiB
C#
68 lines
1.8 KiB
C#
namespace Spectre.Console.Tests.Unit;
|
|
|
|
[ExpectationPath("Widgets/Padder")]
|
|
public sealed class PadderTests
|
|
{
|
|
[Fact]
|
|
[Expectation("Render")]
|
|
public Task Should_Render_Padded_Object_Correctly()
|
|
{
|
|
// Given
|
|
var console = new TestConsole().Width(60);
|
|
var table = new Table();
|
|
table.AddColumn("Foo");
|
|
table.AddColumn("Bar");
|
|
table.AddRow("Baz", "Qux");
|
|
table.AddRow("Corgi", "Waldo");
|
|
|
|
// When
|
|
console.Write(new Padder(table).Padding(1, 2, 3, 4));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("Render_Expanded")]
|
|
public Task Should_Render_Expanded_Padded_Object_Correctly()
|
|
{
|
|
// Given
|
|
var console = new TestConsole().Width(60);
|
|
var table = new Table();
|
|
table.AddColumn("Foo");
|
|
table.AddColumn("Bar");
|
|
table.AddRow("Baz", "Qux");
|
|
table.AddRow("Corgi", "Waldo");
|
|
|
|
// When
|
|
console.Write(new Padder(table)
|
|
.Padding(1, 2, 3, 4)
|
|
.Expand());
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("Render_Nested")]
|
|
public Task Should_Render_Padded_Object_Correctly_When_Nested_Within_Other_Object()
|
|
{
|
|
// Given
|
|
var console = new TestConsole().Width(60);
|
|
var table = new Table();
|
|
table.AddColumn("Foo");
|
|
table.AddColumn("Bar", c => c.PadLeft(0).PadRight(0));
|
|
table.AddRow("Baz", "Qux");
|
|
table.AddRow(new Text("Corgi"), new Padder(new Panel("Waldo"))
|
|
.Padding(2, 1));
|
|
|
|
// When
|
|
console.Write(new Padder(table)
|
|
.Padding(1, 2, 3, 4)
|
|
.Expand());
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
}
|