mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-01 02:28:14 +08:00
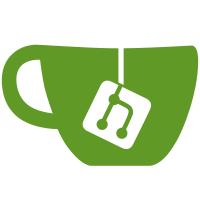
Removed the verbs from all extension methods that manipulate properties which makes the API more succinct and easier to read. Also added implicit conversion from string to Style. As a good OSS citizen, I've obsoleted the old methods with a warning for now, so this shouldn't break anyone using the old methods.
81 lines
2.8 KiB
C#
81 lines
2.8 KiB
C#
using System;
|
|
|
|
namespace Spectre.Console
|
|
{
|
|
/// <summary>
|
|
/// Contains extension methods for <see cref="IOverflowable"/>.
|
|
/// </summary>
|
|
public static class OverflowableExtensions
|
|
{
|
|
/// <summary>
|
|
/// Folds any overflowing text.
|
|
/// </summary>
|
|
/// <typeparam name="T">An object implementing <see cref="IOverflowable"/>.</typeparam>
|
|
/// <param name="obj">The overflowable object instance.</param>
|
|
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
|
public static T Fold<T>(this T obj)
|
|
where T : class, IOverflowable
|
|
{
|
|
if (obj is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(obj));
|
|
}
|
|
|
|
return Overflow(obj, Console.Overflow.Fold);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Crops any overflowing text.
|
|
/// </summary>
|
|
/// <typeparam name="T">An object implementing <see cref="IOverflowable"/>.</typeparam>
|
|
/// <param name="obj">The overflowable object instance.</param>
|
|
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
|
public static T Crop<T>(this T obj)
|
|
where T : class, IOverflowable
|
|
{
|
|
if (obj is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(obj));
|
|
}
|
|
|
|
return Overflow(obj, Console.Overflow.Crop);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Crops any overflowing text and adds an ellipsis to the end.
|
|
/// </summary>
|
|
/// <typeparam name="T">An object implementing <see cref="IOverflowable"/>.</typeparam>
|
|
/// <param name="obj">The overflowable object instance.</param>
|
|
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
|
public static T Ellipsis<T>(this T obj)
|
|
where T : class, IOverflowable
|
|
{
|
|
if (obj is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(obj));
|
|
}
|
|
|
|
return Overflow(obj, Console.Overflow.Ellipsis);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Sets the overflow strategy.
|
|
/// </summary>
|
|
/// <typeparam name="T">An object implementing <see cref="IOverflowable"/>.</typeparam>
|
|
/// <param name="obj">The overflowable object instance.</param>
|
|
/// <param name="overflow">The overflow strategy to use.</param>
|
|
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
|
public static T Overflow<T>(this T obj, Overflow overflow)
|
|
where T : class, IOverflowable
|
|
{
|
|
if (obj is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(obj));
|
|
}
|
|
|
|
obj.Overflow = overflow;
|
|
return obj;
|
|
}
|
|
}
|
|
}
|