mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-06 04:28:15 +08:00
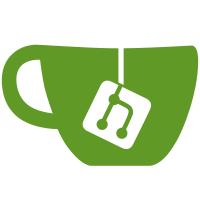
This is far from complete, but it's a start and it will enable us to create things like tables and other complex objects in the long run.
53 lines
1.4 KiB
C#
53 lines
1.4 KiB
C#
using System;
|
|
|
|
namespace Spectre.Console.Internal
|
|
{
|
|
internal sealed class MarkupStyleNode : IMarkupNode
|
|
{
|
|
private readonly Styles? _style;
|
|
private readonly Color? _foreground;
|
|
private readonly Color? _background;
|
|
private readonly IMarkupNode _element;
|
|
|
|
public MarkupStyleNode(
|
|
Styles? style,
|
|
Color? foreground,
|
|
Color? background,
|
|
IMarkupNode element)
|
|
{
|
|
_style = style;
|
|
_foreground = foreground;
|
|
_background = background;
|
|
_element = element ?? throw new ArgumentNullException(nameof(element));
|
|
}
|
|
|
|
public void Render(IAnsiConsole renderer)
|
|
{
|
|
var style = (IDisposable)null;
|
|
var foreground = (IDisposable)null;
|
|
var background = (IDisposable)null;
|
|
|
|
if (_style != null)
|
|
{
|
|
style = renderer.PushStyle(_style.Value);
|
|
}
|
|
|
|
if (_foreground != null)
|
|
{
|
|
foreground = renderer.PushColor(_foreground.Value, foreground: true);
|
|
}
|
|
|
|
if (_background != null)
|
|
{
|
|
background = renderer.PushColor(_background.Value, foreground: false);
|
|
}
|
|
|
|
_element.Render(renderer);
|
|
|
|
background?.Dispose();
|
|
foreground?.Dispose();
|
|
style?.Dispose();
|
|
}
|
|
}
|
|
}
|