mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-01 02:28:14 +08:00
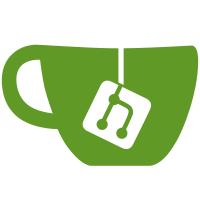
Uses the typenamehelper from Ben.Demystifer to help break down things like generic lists into their actual type display name.
39 lines
1.0 KiB
C#
39 lines
1.0 KiB
C#
namespace Spectre.Console.Tests.Data;
|
|
|
|
public static class TestExceptions
|
|
{
|
|
public static bool MethodThatThrows(int? number) => throw new InvalidOperationException("Throwing!");
|
|
|
|
public static bool GenericMethodThatThrows<T0, T1, TRet>(int? number) => throw new InvalidOperationException("Throwing!");
|
|
|
|
public static void ThrowWithInnerException()
|
|
{
|
|
try
|
|
{
|
|
MethodThatThrows(null);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new InvalidOperationException("Something threw!", ex);
|
|
}
|
|
}
|
|
|
|
public static void ThrowWithGenericInnerException()
|
|
{
|
|
try
|
|
{
|
|
GenericMethodThatThrows<int, float, double>(null);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new InvalidOperationException("Something threw!", ex);
|
|
}
|
|
}
|
|
|
|
public static List<T> GenericMethodWithOutThatThrows<T>(out List<T> firstFewItems)
|
|
{
|
|
firstFewItems = new List<T>();
|
|
throw new InvalidOperationException("Throwing!");
|
|
}
|
|
}
|