mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-19 10:12:50 +08:00
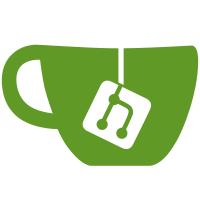
Removed the verbs from all extension methods that manipulate properties which makes the API more succinct and easier to read. Also added implicit conversion from string to Style. As a good OSS citizen, I've obsoleted the old methods with a warning for now, so this shouldn't break anyone using the old methods.
55 lines
1.5 KiB
C#
55 lines
1.5 KiB
C#
using System;
|
|
|
|
namespace Spectre.Console
|
|
{
|
|
/// <summary>
|
|
/// Contains extension methods for <see cref="RuleExtensions"/>.
|
|
/// </summary>
|
|
public static class RuleExtensions
|
|
{
|
|
/// <summary>
|
|
/// Sets the rule title.
|
|
/// </summary>
|
|
/// <param name="rule">The rule.</param>
|
|
/// <param name="title">The title.</param>
|
|
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
|
public static Rule RuleTitle(this Rule rule, string title)
|
|
{
|
|
if (rule is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(rule));
|
|
}
|
|
|
|
if (title is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(title));
|
|
}
|
|
|
|
rule.Title = title;
|
|
return rule;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Sets the rule style.
|
|
/// </summary>
|
|
/// <param name="rule">The rule.</param>
|
|
/// <param name="style">The rule style.</param>
|
|
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
|
public static Rule RuleStyle(this Rule rule, Style style)
|
|
{
|
|
if (rule is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(rule));
|
|
}
|
|
|
|
if (style is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(style));
|
|
}
|
|
|
|
rule.Style = style;
|
|
return rule;
|
|
}
|
|
}
|
|
}
|