mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-16 00:42:51 +08:00
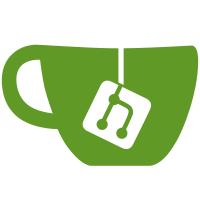
* Add selection prompt search as you type * Fix small bug * Simplify * Simplify * Remove spacebar as a selection prompt submit key * Trigger CI * Update src/Spectre.Console/Prompts/SelectionPrompt.cs Co-authored-by: Martin Costello <martin@martincostello.com> * Simplifty Mask method * Handle multi-selection prompt better * Update API naming * Address feedback * Add some tests * Remove whitespace * Improve search and highlighting * Add test case for previous issue * Add extra test case * Make prompt searchable --------- Co-authored-by: Martin Costello <martin@martincostello.com> Co-authored-by: Patrik Svensson <patrik@patriksvensson.se>
83 lines
2.0 KiB
C#
83 lines
2.0 KiB
C#
using Spectre.Console;
|
|
|
|
namespace Namespace;
|
|
|
|
public class HighlightTests
|
|
{
|
|
private readonly Style _highlightStyle = new Style(foreground: Color.Default, background: Color.Yellow, Decoration.Bold);
|
|
|
|
[Fact]
|
|
public void Should_Return_Same_Value_When_SearchText_Is_Empty()
|
|
{
|
|
// Given
|
|
var value = "Sample text";
|
|
var searchText = string.Empty;
|
|
var highlightStyle = new Style();
|
|
|
|
// When
|
|
var result = value.Highlight(searchText, highlightStyle);
|
|
|
|
// Then
|
|
result.ShouldBe(value);
|
|
}
|
|
|
|
[Fact]
|
|
public void Should_Highlight_Matched_Text()
|
|
{
|
|
// Given
|
|
var value = "Sample text with test word";
|
|
var searchText = "test";
|
|
var highlightStyle = _highlightStyle;
|
|
|
|
// When
|
|
var result = value.Highlight(searchText, highlightStyle);
|
|
|
|
// Then
|
|
result.ShouldBe("Sample text with [bold on yellow]test[/] word");
|
|
}
|
|
|
|
[Fact]
|
|
public void Should_Not_Match_Text_Across_Tokens()
|
|
{
|
|
// Given
|
|
var value = "[red]Sample text[/] with test word";
|
|
var searchText = "text with";
|
|
var highlightStyle = _highlightStyle;
|
|
|
|
// When
|
|
var result = value.Highlight(searchText, highlightStyle);
|
|
|
|
// Then
|
|
result.ShouldBe(value);
|
|
}
|
|
|
|
[Fact]
|
|
public void Should_Highlight_Only_First_Matched_Text()
|
|
{
|
|
// Given
|
|
var value = "Sample text with test word";
|
|
var searchText = "te";
|
|
var highlightStyle = _highlightStyle;
|
|
|
|
// When
|
|
var result = value.Highlight(searchText, highlightStyle);
|
|
|
|
// Then
|
|
result.ShouldBe("Sample [bold on yellow]te[/]xt with test word");
|
|
}
|
|
|
|
[Fact]
|
|
public void Should_Not_Match_Text_Outside_Of_Text_Tokens()
|
|
{
|
|
// Given
|
|
var value = "[red]Sample text with test word[/]";
|
|
var searchText = "red";
|
|
var highlightStyle = _highlightStyle;
|
|
|
|
// When
|
|
var result = value.Highlight(searchText, highlightStyle);
|
|
|
|
// Then
|
|
result.ShouldBe(value);
|
|
}
|
|
} |