mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-16 00:42:51 +08:00
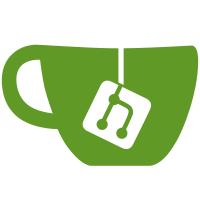
* Allow setting the style of the default value in TextPrompt Add property "DefaultValueStyle" to TextPrompt which allows setting the style in which the default value is rendered. When no style is set, the value is displayed as green text, keeping the existing behavior. * Allow setting the style in which a TextPrompt's choices are displayed Add property "ChoicesStyle" to TextPrompt which allows setting the style in the prompt's choices are rendered. When no style is set, choices are displayed as blue text, keeping the existing behavior.
337 lines
8.9 KiB
C#
337 lines
8.9 KiB
C#
namespace Spectre.Console.Tests.Unit;
|
|
|
|
[UsesVerify]
|
|
[ExpectationPath("Prompts/Text")]
|
|
public sealed class TextPromptTests
|
|
{
|
|
[Fact]
|
|
public void Should_Return_Entered_Text()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushTextWithEnter("Hello World");
|
|
|
|
// When
|
|
var result = console.Prompt(new TextPrompt<string>("Enter text:"));
|
|
|
|
// Then
|
|
result.ShouldBe("Hello World");
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("ConversionError")]
|
|
public Task Should_Return_Validation_Error_If_Value_Cannot_Be_Converted()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushTextWithEnter("ninety-nine");
|
|
console.Input.PushTextWithEnter("99");
|
|
|
|
// When
|
|
console.Prompt(new TextPrompt<int>("Age?"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Lines);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("DefaultValue")]
|
|
public Task Should_Chose_Default_Value_If_Nothing_Is_Entered()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushKey(ConsoleKey.Enter);
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Favorite fruit?")
|
|
.AddChoice("Banana")
|
|
.AddChoice("Orange")
|
|
.DefaultValue("Banana"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("InvalidChoice")]
|
|
public Task Should_Return_Error_If_An_Invalid_Choice_Is_Made()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushTextWithEnter("Apple");
|
|
console.Input.PushTextWithEnter("Banana");
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Favorite fruit?")
|
|
.AddChoice("Banana")
|
|
.AddChoice("Orange")
|
|
.DefaultValue("Banana"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("AcceptChoice")]
|
|
public Task Should_Accept_Choice_In_List()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushTextWithEnter("Orange");
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Favorite fruit?")
|
|
.AddChoice("Banana")
|
|
.AddChoice("Orange")
|
|
.DefaultValue("Banana"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("AutoComplete_Empty")]
|
|
public Task Should_Auto_Complete_To_First_Choice_If_Pressing_Tab_On_Empty_String()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushKey(ConsoleKey.Tab);
|
|
console.Input.PushKey(ConsoleKey.Enter);
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Favorite fruit?")
|
|
.AddChoice("Banana")
|
|
.AddChoice("Orange")
|
|
.DefaultValue("Banana"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("AutoComplete_BestMatch")]
|
|
public Task Should_Auto_Complete_To_Best_Match()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushText("Band");
|
|
console.Input.PushKey(ConsoleKey.Tab);
|
|
console.Input.PushKey(ConsoleKey.Enter);
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Favorite fruit?")
|
|
.AddChoice("Banana")
|
|
.AddChoice("Bandana")
|
|
.AddChoice("Orange"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("AutoComplete_NextChoice")]
|
|
public Task Should_Auto_Complete_To_Next_Choice_When_Pressing_Tab_On_A_Match()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushText("Apple");
|
|
console.Input.PushKey(ConsoleKey.Tab);
|
|
console.Input.PushKey(ConsoleKey.Enter);
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Favorite fruit?")
|
|
.AddChoice("Apple")
|
|
.AddChoice("Banana")
|
|
.AddChoice("Orange"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("CustomValidation")]
|
|
public Task Should_Return_Error_If_Custom_Validation_Fails()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushTextWithEnter("22");
|
|
console.Input.PushTextWithEnter("102");
|
|
console.Input.PushTextWithEnter("ABC");
|
|
console.Input.PushTextWithEnter("99");
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<int>("Guess number:")
|
|
.ValidationErrorMessage("Invalid input")
|
|
.Validate(age =>
|
|
{
|
|
if (age < 99)
|
|
{
|
|
return ValidationResult.Error("Too low");
|
|
}
|
|
else if (age > 99)
|
|
{
|
|
return ValidationResult.Error("Too high");
|
|
}
|
|
|
|
return ValidationResult.Success();
|
|
}));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("CustomConverter")]
|
|
public Task Should_Use_Custom_Converter()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushTextWithEnter("Banana");
|
|
|
|
// When
|
|
var result = console.Prompt(
|
|
new TextPrompt<(int, string)>("Favorite fruit?")
|
|
.AddChoice((1, "Apple"))
|
|
.AddChoice((2, "Banana"))
|
|
.WithConverter(testData => testData.Item2));
|
|
|
|
// Then
|
|
result.Item1.ShouldBe(2);
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("SecretDefaultValue")]
|
|
public Task Should_Chose_Masked_Default_Value_If_Nothing_Is_Entered_And_Prompt_Is_Secret()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushKey(ConsoleKey.Enter);
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Favorite fruit?")
|
|
.Secret()
|
|
.DefaultValue("Banana"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("NoSuffix")]
|
|
[GitHubIssue(413)]
|
|
public Task Should_Not_Append_Questionmark_Or_Colon_If_No_Choices_Are_Set()
|
|
{
|
|
// Given
|
|
var console = new TestConsole();
|
|
console.Input.PushTextWithEnter("Orange");
|
|
|
|
// When
|
|
console.Prompt(
|
|
new TextPrompt<string>("Enter command$"));
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("DefaultValueStyleNotSet")]
|
|
public Task Uses_default_style_for_default_value_if_no_style_is_set()
|
|
{
|
|
// Given
|
|
var console = new TestConsole
|
|
{
|
|
EmitAnsiSequences = true,
|
|
};
|
|
console.Input.PushTextWithEnter("Input");
|
|
|
|
var prompt = new TextPrompt<string>("Enter Value:")
|
|
.ShowDefaultValue()
|
|
.DefaultValue("default");
|
|
|
|
// When
|
|
console.Prompt(prompt);
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("DefaultValueStyleSet")]
|
|
public Task Uses_specified_default_value_style()
|
|
{
|
|
// Given
|
|
var console = new TestConsole
|
|
{
|
|
EmitAnsiSequences = true,
|
|
};
|
|
console.Input.PushTextWithEnter("Input");
|
|
|
|
var prompt = new TextPrompt<string>("Enter Value:")
|
|
.ShowDefaultValue()
|
|
.DefaultValue("default")
|
|
.DefaultValueStyle(new Style(foreground: Color.Red));
|
|
|
|
// When
|
|
console.Prompt(prompt);
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("ChoicesStyleNotSet")]
|
|
public Task Uses_default_style_for_choices_if_no_style_is_set()
|
|
{
|
|
// Given
|
|
var console = new TestConsole
|
|
{
|
|
EmitAnsiSequences = true,
|
|
};
|
|
console.Input.PushTextWithEnter("Choice 2");
|
|
|
|
var prompt = new TextPrompt<string>("Enter Value:")
|
|
.ShowChoices()
|
|
.AddChoice("Choice 1")
|
|
.AddChoice("Choice 2");
|
|
|
|
// When
|
|
console.Prompt(prompt);
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
|
|
[Fact]
|
|
[Expectation("ChoicesStyleSet")]
|
|
public Task Uses_the_specified_choices_style()
|
|
{
|
|
// Given
|
|
var console = new TestConsole
|
|
{
|
|
EmitAnsiSequences = true,
|
|
};
|
|
console.Input.PushTextWithEnter("Choice 2");
|
|
|
|
var prompt = new TextPrompt<string>("Enter Value:")
|
|
.ShowChoices()
|
|
.AddChoice("Choice 1")
|
|
.AddChoice("Choice 2")
|
|
.ChoicesStyle(new Style(foreground: Color.Red));
|
|
|
|
// When
|
|
console.Prompt(prompt);
|
|
|
|
// Then
|
|
return Verifier.Verify(console.Output);
|
|
}
|
|
}
|