mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-06 04:28:15 +08:00
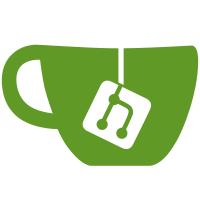
Removed the verbs from all extension methods that manipulate properties which makes the API more succinct and easier to read. Also added implicit conversion from string to Style. As a good OSS citizen, I've obsoleted the old methods with a warning for now, so this shouldn't break anyone using the old methods.
64 lines
1.7 KiB
C#
64 lines
1.7 KiB
C#
namespace Spectre.Console
|
|
{
|
|
/// <summary>
|
|
/// A console capable of writing ANSI escape sequences.
|
|
/// </summary>
|
|
public static partial class AnsiConsole
|
|
{
|
|
internal static Style CurrentStyle { get; private set; } = Style.Plain;
|
|
internal static bool Created { get; private set; }
|
|
|
|
/// <summary>
|
|
/// Gets or sets the foreground color.
|
|
/// </summary>
|
|
public static Color Foreground
|
|
{
|
|
get => CurrentStyle.Foreground;
|
|
set => CurrentStyle = CurrentStyle.Foreground(value);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Gets or sets the background color.
|
|
/// </summary>
|
|
public static Color Background
|
|
{
|
|
get => CurrentStyle.Background;
|
|
set => CurrentStyle = CurrentStyle.Background(value);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Gets or sets the text decoration.
|
|
/// </summary>
|
|
public static Decoration Decoration
|
|
{
|
|
get => CurrentStyle.Decoration;
|
|
set => CurrentStyle = CurrentStyle.Decoration(value);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Resets colors and text decorations.
|
|
/// </summary>
|
|
public static void Reset()
|
|
{
|
|
ResetColors();
|
|
ResetDecoration();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Resets the current applied text decorations.
|
|
/// </summary>
|
|
public static void ResetDecoration()
|
|
{
|
|
Decoration = Decoration.None;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Resets the current applied foreground and background colors.
|
|
/// </summary>
|
|
public static void ResetColors()
|
|
{
|
|
CurrentStyle = Style.Plain;
|
|
}
|
|
}
|
|
}
|