mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-19 02:12:49 +08:00
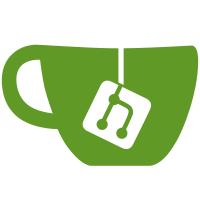
This makes it possible for encoders to output better representation of the actual objects instead of working with chopped up segments. * IAnsiConsole.Write now takes an IRenderable instead of segments * Calculating cell width does no longer require a render context * Removed RenderContext.LegacyConsole * Removed RenderContext.Encoding * Added Capabilities.Unicode
71 lines
2.0 KiB
C#
71 lines
2.0 KiB
C#
using Spectre.Console.Rendering;
|
|
|
|
namespace Spectre.Console
|
|
{
|
|
internal sealed class LegacyConsoleBackend : IAnsiConsoleBackend
|
|
{
|
|
private readonly IAnsiConsole _console;
|
|
private Style _lastStyle;
|
|
|
|
public IAnsiConsoleCursor Cursor { get; }
|
|
|
|
public LegacyConsoleBackend(IAnsiConsole console)
|
|
{
|
|
_console = console ?? throw new System.ArgumentNullException(nameof(console));
|
|
_lastStyle = Style.Plain;
|
|
|
|
Cursor = new LegacyConsoleCursor();
|
|
}
|
|
|
|
public void Clear(bool home)
|
|
{
|
|
var (x, y) = (System.Console.CursorLeft, System.Console.CursorTop);
|
|
|
|
System.Console.Clear();
|
|
|
|
if (!home)
|
|
{
|
|
// Set the cursor position
|
|
System.Console.SetCursorPosition(x, y);
|
|
}
|
|
}
|
|
|
|
public void Write(IRenderable renderable)
|
|
{
|
|
foreach (var segment in renderable.GetSegments(_console))
|
|
{
|
|
if (segment.IsControlCode)
|
|
{
|
|
continue;
|
|
}
|
|
|
|
if (_lastStyle?.Equals(segment.Style) != true)
|
|
{
|
|
SetStyle(segment.Style);
|
|
}
|
|
|
|
_console.Profile.Out.Write(segment.Text.NormalizeNewLines(native: true));
|
|
}
|
|
}
|
|
|
|
private void SetStyle(Style style)
|
|
{
|
|
_lastStyle = style;
|
|
|
|
System.Console.ResetColor();
|
|
|
|
var background = Color.ToConsoleColor(style.Background);
|
|
if (_console.Profile.ColorSystem != ColorSystem.NoColors && (int)background != -1)
|
|
{
|
|
System.Console.BackgroundColor = background;
|
|
}
|
|
|
|
var foreground = Color.ToConsoleColor(style.Foreground);
|
|
if (_console.Profile.ColorSystem != ColorSystem.NoColors && (int)foreground != -1)
|
|
{
|
|
System.Console.ForegroundColor = foreground;
|
|
}
|
|
}
|
|
}
|
|
}
|