mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-02 02:48:17 +08:00
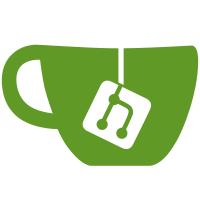
This makes it possible for encoders to output better representation of the actual objects instead of working with chopped up segments. * IAnsiConsole.Write now takes an IRenderable instead of segments * Calculating cell width does no longer require a render context * Removed RenderContext.LegacyConsole * Removed RenderContext.Encoding * Added Capabilities.Unicode
71 lines
2.0 KiB
C#
71 lines
2.0 KiB
C#
using System;
|
|
using Spectre.Console.Rendering;
|
|
|
|
namespace Spectre.Console
|
|
{
|
|
/// <summary>
|
|
/// A console capable of writing ANSI escape sequences.
|
|
/// </summary>
|
|
public static partial class AnsiConsole
|
|
{
|
|
/// <summary>
|
|
/// Starts recording the console output.
|
|
/// </summary>
|
|
public static void Record()
|
|
{
|
|
if (_recorder == null)
|
|
{
|
|
_recorder = new Recorder(Console);
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Exports all recorded console output as text.
|
|
/// </summary>
|
|
/// <returns>The recorded output as text.</returns>
|
|
public static string ExportText()
|
|
{
|
|
if (_recorder == null)
|
|
{
|
|
throw new InvalidOperationException("Cannot export text since a recording hasn't been started.");
|
|
}
|
|
|
|
return _recorder.ExportText();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Exports all recorded console output as HTML text.
|
|
/// </summary>
|
|
/// <returns>The recorded output as HTML text.</returns>
|
|
public static string ExportHtml()
|
|
{
|
|
if (_recorder == null)
|
|
{
|
|
throw new InvalidOperationException("Cannot export HTML since a recording hasn't been started.");
|
|
}
|
|
|
|
return _recorder.ExportHtml();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Exports all recorded console output using a custom encoder.
|
|
/// </summary>
|
|
/// <param name="encoder">The encoder to use.</param>
|
|
/// <returns>The recorded output.</returns>
|
|
public static string ExportCustom(IAnsiConsoleEncoder encoder)
|
|
{
|
|
if (_recorder == null)
|
|
{
|
|
throw new InvalidOperationException("Cannot export HTML since a recording hasn't been started.");
|
|
}
|
|
|
|
if (encoder is null)
|
|
{
|
|
throw new ArgumentNullException(nameof(encoder));
|
|
}
|
|
|
|
return _recorder.Export(encoder);
|
|
}
|
|
}
|
|
}
|