mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-04-16 00:42:51 +08:00
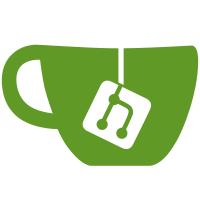
Allow custom help providers * Version option will show in help even with a default command * Reserve `-v` and `--version` as special Spectre.Console command line arguments (nb. breaking change for Spectre.Console users who have a default command with a settings class that uses either of these switches). * Help writer correctly determines if trailing commands exist and whether to display them as optional or mandatory in the usage statement. * Ability to control the number of indirect commands to display in the help text when the command itself doesn't have any examples of its own. Defaults to 5 (for backward compatibility) but can be set to any integer or zero to disable completely. * Significant increase in unit test coverage for the help writer. * Minor grammatical improvements to website documentation.
51 lines
1.5 KiB
C#
51 lines
1.5 KiB
C#
namespace Spectre.Console.Tests.Data;
|
|
|
|
public sealed class OptionalArgumentWithDefaultValueSettings : CommandSettings
|
|
{
|
|
[CommandArgument(0, "[GREETING]")]
|
|
[DefaultValue("Hello World")]
|
|
public string Greeting { get; set; }
|
|
}
|
|
|
|
public sealed class OptionalArgumentWithPropertyInitializerSettings : CommandSettings
|
|
{
|
|
[CommandArgument(0, "[NAMES]")]
|
|
public string[] Names { get; set; } = Array.Empty<string>();
|
|
|
|
[CommandOption("-c")]
|
|
public int Count { get; set; } = 1;
|
|
|
|
[CommandOption("--value")]
|
|
public int Value { get; set; } = 0;
|
|
}
|
|
|
|
public sealed class OptionalArgumentWithDefaultValueAndTypeConverterSettings : CommandSettings
|
|
{
|
|
[CommandArgument(0, "[GREETING]")]
|
|
[DefaultValue("5")]
|
|
[TypeConverter(typeof(StringToIntegerConverter))]
|
|
public int Greeting { get; set; }
|
|
}
|
|
|
|
public sealed class RequiredArgumentWithDefaultValueSettings : CommandSettings
|
|
{
|
|
[CommandArgument(0, "<GREETING>")]
|
|
[DefaultValue("Hello World")]
|
|
public string Greeting { get; set; }
|
|
}
|
|
|
|
public sealed class OptionWithArrayOfEnumDefaultValueSettings : CommandSettings
|
|
{
|
|
[CommandOption("--days")]
|
|
[DefaultValue(new[] { DayOfWeek.Sunday, DayOfWeek.Saturday })]
|
|
public DayOfWeek[] Days { get; set; }
|
|
}
|
|
|
|
public sealed class OptionWithArrayOfStringDefaultValueAndTypeConverterSettings : CommandSettings
|
|
{
|
|
[CommandOption("--numbers")]
|
|
[DefaultValue(new[] { "2", "3" })]
|
|
[TypeConverter(typeof(StringToIntegerConverter))]
|
|
public int[] Numbers { get; set; }
|
|
}
|