mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-08-02 10:15:58 +08:00
Add Length and Lines properties to Paragraph
* Adds Length and Lines to `Paragraph`, `Markup` and `Text`. * Do not use Environment.NewLine
This commit is contained in:
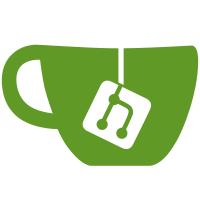
committed by
Patrik Svensson
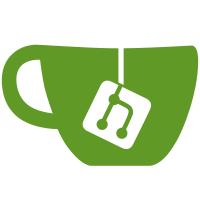
parent
e48ae9600a
commit
d1d94cdebe
@ -7,6 +7,44 @@ namespace Spectre.Console.Tests.Unit
|
||||
{
|
||||
public sealed class MarkupTests
|
||||
{
|
||||
public sealed class TheLengthProperty
|
||||
{
|
||||
[Theory]
|
||||
[InlineData("Hello", 5)]
|
||||
[InlineData("Hello\nWorld", 11)]
|
||||
[InlineData("[yellow]Hello[/]", 5)]
|
||||
public void Should_Return_The_Number_Of_Characters(string input, int expected)
|
||||
{
|
||||
// Given
|
||||
var markup = new Markup(input);
|
||||
|
||||
// When
|
||||
var result = markup.Length;
|
||||
|
||||
// Then
|
||||
result.ShouldBe(expected);
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class TheLinesProperty
|
||||
{
|
||||
[Theory]
|
||||
[InlineData("Hello", 1)]
|
||||
[InlineData("Hello\nWorld", 2)]
|
||||
[InlineData("[yellow]Hello[/]\nWorld", 2)]
|
||||
public void Should_Return_The_Number_Of_Lines(string input, int expected)
|
||||
{
|
||||
// Given
|
||||
var markup = new Markup(input);
|
||||
|
||||
// When
|
||||
var result = markup.Lines;
|
||||
|
||||
// Then
|
||||
result.ShouldBe(expected);
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class TheEscapeMethod
|
||||
{
|
||||
[Theory]
|
||||
|
@ -7,6 +7,42 @@ namespace Spectre.Console.Tests.Unit
|
||||
{
|
||||
public sealed class TextTests
|
||||
{
|
||||
public sealed class TheLengthProperty
|
||||
{
|
||||
[Theory]
|
||||
[InlineData("Hello", 5)]
|
||||
[InlineData("Hello\nWorld", 11)]
|
||||
public void Should_Return_The_Number_Of_Characters(string input, int expected)
|
||||
{
|
||||
// Given
|
||||
var markup = new Text(input);
|
||||
|
||||
// When
|
||||
var result = markup.Length;
|
||||
|
||||
// Then
|
||||
result.ShouldBe(expected);
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class TheLinesProperty
|
||||
{
|
||||
[Theory]
|
||||
[InlineData("Hello", 1)]
|
||||
[InlineData("Hello\nWorld", 2)]
|
||||
public void Should_Return_The_Number_Of_Lines(string input, int expected)
|
||||
{
|
||||
// Given
|
||||
var markup = new Text(input);
|
||||
|
||||
// When
|
||||
var result = markup.Lines;
|
||||
|
||||
// Then
|
||||
result.ShouldBe(expected);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Consider_The_Longest_Word_As_Minimum_Width()
|
||||
{
|
||||
|
@ -71,7 +71,7 @@ namespace Spectre.Console.Cli
|
||||
{
|
||||
for (var i = 0; i < count; i++)
|
||||
{
|
||||
_content.Append(Environment.NewLine);
|
||||
_content.Append('\n');
|
||||
}
|
||||
|
||||
return this;
|
||||
|
@ -59,7 +59,7 @@ namespace Spectre.Console
|
||||
/// <param name="value">The value to write.</param>
|
||||
public static void MarkupLine(this IAnsiConsole console, string value)
|
||||
{
|
||||
Markup(console, value + Environment.NewLine);
|
||||
Markup(console, value + "\n");
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
@ -71,7 +71,7 @@ namespace Spectre.Console
|
||||
/// <param name="args">An array of objects to write.</param>
|
||||
public static void MarkupLine(this IAnsiConsole console, IFormatProvider provider, string format, params object[] args)
|
||||
{
|
||||
Markup(console, provider, format + Environment.NewLine, args);
|
||||
Markup(console, provider, format + "\n", args);
|
||||
}
|
||||
}
|
||||
}
|
@ -73,7 +73,7 @@ namespace Spectre.Console
|
||||
throw new ArgumentNullException(nameof(console));
|
||||
}
|
||||
|
||||
console.Write(new Text(Environment.NewLine, Style.Plain));
|
||||
console.Write(new Text("\n", Style.Plain));
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
@ -104,7 +104,7 @@ namespace Spectre.Console
|
||||
throw new ArgumentNullException(nameof(text));
|
||||
}
|
||||
|
||||
console.Write(text + Environment.NewLine, style);
|
||||
console.Write(text + "\n", style);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
@ -11,11 +11,6 @@ namespace Spectre.Console
|
||||
/// </summary>
|
||||
public static class StringExtensions
|
||||
{
|
||||
// Cache whether or not internally normalized line endings
|
||||
// already are normalized. No reason to do yet another replace if it is.
|
||||
private static readonly bool _alreadyNormalized
|
||||
= Environment.NewLine.Equals("\n", StringComparison.OrdinalIgnoreCase);
|
||||
|
||||
/// <summary>
|
||||
/// Escapes text so that it won’t be interpreted as markup.
|
||||
/// </summary>
|
||||
@ -91,12 +86,6 @@ namespace Spectre.Console
|
||||
{
|
||||
text = text?.ReplaceExact("\r\n", "\n");
|
||||
text ??= string.Empty;
|
||||
|
||||
if (native && !_alreadyNormalized)
|
||||
{
|
||||
text = text.ReplaceExact("\n", Environment.NewLine);
|
||||
}
|
||||
|
||||
return text;
|
||||
}
|
||||
|
||||
|
@ -36,7 +36,7 @@ namespace Spectre.Console
|
||||
|
||||
if (!last)
|
||||
{
|
||||
builder.Append(Environment.NewLine);
|
||||
builder.Append('\n');
|
||||
}
|
||||
}
|
||||
}
|
||||
|
@ -44,7 +44,7 @@ namespace Spectre.Console.Rendering
|
||||
/// <summary>
|
||||
/// Gets a segment representing a line break.
|
||||
/// </summary>
|
||||
public static Segment LineBreak { get; } = new Segment(Environment.NewLine, Style.Plain, true, false);
|
||||
public static Segment LineBreak { get; } = new Segment("\n", Style.Plain, true, false);
|
||||
|
||||
/// <summary>
|
||||
/// Gets an empty segment.
|
||||
|
@ -27,6 +27,16 @@ namespace Spectre.Console
|
||||
set => _paragraph.Overflow = value;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the character count.
|
||||
/// </summary>
|
||||
public int Length => _paragraph.Length;
|
||||
|
||||
/// <summary>
|
||||
/// Gets the number of lines.
|
||||
/// </summary>
|
||||
public int Lines => _paragraph.Lines;
|
||||
|
||||
/// <summary>
|
||||
/// Initializes a new instance of the <see cref="Markup"/> class.
|
||||
/// </summary>
|
||||
|
@ -2,6 +2,7 @@ using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Diagnostics;
|
||||
using System.Linq;
|
||||
using System.Text;
|
||||
using Spectre.Console.Rendering;
|
||||
|
||||
namespace Spectre.Console
|
||||
@ -25,6 +26,16 @@ namespace Spectre.Console
|
||||
/// </summary>
|
||||
public Overflow? Overflow { get; set; }
|
||||
|
||||
/// <summary>
|
||||
/// Gets the character count of the paragraph.
|
||||
/// </summary>
|
||||
public int Length => _lines.Sum(line => line.Length) + Math.Max(0, Lines - 1);
|
||||
|
||||
/// <summary>
|
||||
/// Gets the number of lines in the paragraph.
|
||||
/// </summary>
|
||||
public int Lines => _lines.Count;
|
||||
|
||||
/// <summary>
|
||||
/// Initializes a new instance of the <see cref="Paragraph"/> class.
|
||||
/// </summary>
|
||||
|
@ -29,7 +29,7 @@ namespace Spectre.Console
|
||||
if (_lastStatus != task.Description)
|
||||
{
|
||||
_lastStatus = task.Description;
|
||||
_renderable = new Markup(task.Description + Environment.NewLine);
|
||||
_renderable = new Markup(task.Description + "\n");
|
||||
return;
|
||||
}
|
||||
}
|
||||
|
@ -47,6 +47,16 @@ namespace Spectre.Console
|
||||
set => _paragraph.Overflow = value;
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Gets the character count.
|
||||
/// </summary>
|
||||
public int Length => _paragraph.Length;
|
||||
|
||||
/// <summary>
|
||||
/// Gets the number of lines in the text.
|
||||
/// </summary>
|
||||
public int Lines => _paragraph.Lines;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override Measurement Measure(RenderContext context, int maxWidth)
|
||||
{
|
||||
|
Reference in New Issue
Block a user