mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-05 20:18:15 +08:00
(#502) Added GetParent and GetParents to MultiSelectionPrompt
So it it possible to find the parent(s) of a given item.
This commit is contained in:
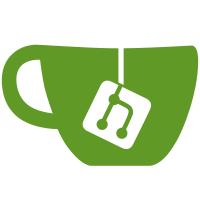
committed by
Patrik Svensson
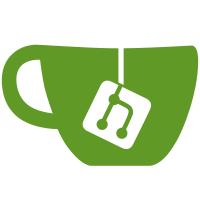
parent
f5a2735501
commit
949f35defd
@ -112,6 +112,41 @@ namespace Spectre.Console
|
||||
.ToList();
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Returns all parent items of the given <paramref name="item"/>.
|
||||
/// </summary>
|
||||
/// <param name="item">The item for which to find the parents.</param>
|
||||
/// <returns>The parent items, or an empty list, if the given item has no parents.</returns>
|
||||
public IEnumerable<T> GetParents(T item)
|
||||
{
|
||||
var promptItem = Tree.Find(item);
|
||||
if (promptItem == null)
|
||||
{
|
||||
throw new ArgumentOutOfRangeException(nameof(item), "item not found in tree.");
|
||||
}
|
||||
|
||||
var parents = new List<ListPromptItem<T>>();
|
||||
while (promptItem.Parent != null)
|
||||
{
|
||||
promptItem = promptItem.Parent;
|
||||
parents.Add(promptItem);
|
||||
}
|
||||
|
||||
return parents
|
||||
.ReverseEnumerable()
|
||||
.Select(x => x.Data);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Returns the parent item of the given <paramref name="item"/>.
|
||||
/// </summary>
|
||||
/// <param name="item">The item for which to find the parent.</param>
|
||||
/// <returns>The parent item, or <c>null</c> if the given item has no parent.</returns>
|
||||
public T? GetParent(T item)
|
||||
{
|
||||
return GetParents(item).LastOrDefault();
|
||||
}
|
||||
|
||||
/// <inheritdoc/>
|
||||
ListPromptInputResult IListPromptStrategy<T>.HandleInput(ConsoleKeyInfo key, ListPromptState<T> state)
|
||||
{
|
||||
|
@ -82,5 +82,75 @@ namespace Spectre.Console.Tests.Unit
|
||||
// Then
|
||||
choice.IsSelected.ShouldBeTrue();
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Get_The_Direct_Parent()
|
||||
{
|
||||
// Given
|
||||
var prompt = new MultiSelectionPrompt<string>();
|
||||
prompt.AddChoice("root").AddChild("level-1").AddChild("level-2").AddChild("item");
|
||||
|
||||
// When
|
||||
var actual = prompt.GetParent("item");
|
||||
|
||||
// Then
|
||||
actual.ShouldBe("level-2");
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Get_The_List_Of_All_Parents()
|
||||
{
|
||||
// Given
|
||||
var prompt = new MultiSelectionPrompt<string>();
|
||||
prompt.AddChoice("root").AddChild("level-1").AddChild("level-2").AddChild("item");
|
||||
|
||||
// When
|
||||
var actual = prompt.GetParents("item");
|
||||
|
||||
// Then
|
||||
actual.ShouldBe(new []{"root", "level-1", "level-2"});
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Get_An_Empty_List_Of_Parents_For_Root_Node()
|
||||
{
|
||||
// Given
|
||||
var prompt = new MultiSelectionPrompt<string>();
|
||||
prompt.AddChoice("root");
|
||||
|
||||
// When
|
||||
var actual = prompt.GetParents("root");
|
||||
|
||||
// Then
|
||||
actual.ShouldBeEmpty();
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Get_Null_As_Direct_Parent_Of_Root_Node()
|
||||
{
|
||||
// Given
|
||||
var prompt = new MultiSelectionPrompt<string>();
|
||||
prompt.AddChoice("root");
|
||||
|
||||
// When
|
||||
var actual = prompt.GetParent("root");
|
||||
|
||||
// Then
|
||||
actual.ShouldBeNull();
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Throw_When_Getting_Parents_Of_Non_Existing_Node()
|
||||
{
|
||||
// Given
|
||||
var prompt = new MultiSelectionPrompt<string>();
|
||||
prompt.AddChoice("root").AddChild("level-1").AddChild("level-2").AddChild("item");
|
||||
|
||||
// When
|
||||
Action action = () => prompt.GetParents("non-existing");
|
||||
|
||||
// Then
|
||||
action.ShouldThrow<ArgumentOutOfRangeException>();
|
||||
}
|
||||
}
|
||||
}
|
||||
|
Reference in New Issue
Block a user