mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-02 02:48:17 +08:00
Remove BorderKind in favour of Border
This commit is contained in:
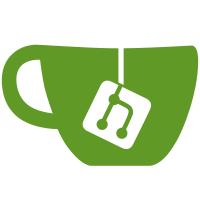
committed by
Patrik Svensson
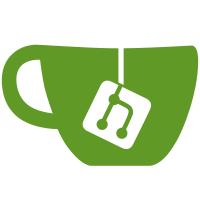
parent
e6e9a4d950
commit
87bde3e5a2
2
.github/workflows/ci.yaml
vendored
2
.github/workflows/ci.yaml
vendored
@ -64,7 +64,7 @@ jobs:
|
|||||||
shell: bash
|
shell: bash
|
||||||
run: |
|
run: |
|
||||||
dotnet tool restore
|
dotnet tool restore
|
||||||
dotnet example diagnostic
|
dotnet example info
|
||||||
dotnet example table
|
dotnet example table
|
||||||
dotnet example grid
|
dotnet example grid
|
||||||
dotnet example panel
|
dotnet example panel
|
||||||
|
@ -1,6 +1,4 @@
|
|||||||
using System.Collections.Generic;
|
using System.Collections.Generic;
|
||||||
using System.IO;
|
|
||||||
using System.Linq;
|
|
||||||
using System.Net.Http;
|
using System.Net.Http;
|
||||||
using System.Threading.Tasks;
|
using System.Threading.Tasks;
|
||||||
using Newtonsoft.Json.Linq;
|
using Newtonsoft.Json.Linq;
|
||||||
|
@ -1,5 +1,4 @@
|
|||||||
using Spectre.Console;
|
using Spectre.Console;
|
||||||
using Spectre.Console.Rendering;
|
|
||||||
|
|
||||||
namespace PanelExample
|
namespace PanelExample
|
||||||
{
|
{
|
||||||
@ -14,7 +13,7 @@ namespace PanelExample
|
|||||||
AnsiConsole.Render(
|
AnsiConsole.Render(
|
||||||
new Panel(
|
new Panel(
|
||||||
new Panel(content)
|
new Panel(content)
|
||||||
.SetBorderKind(BorderKind.Rounded)));
|
.SetBorder(Border.Rounded)));
|
||||||
|
|
||||||
// Left adjusted panel with text
|
// Left adjusted panel with text
|
||||||
AnsiConsole.Render(
|
AnsiConsole.Render(
|
||||||
|
@ -35,7 +35,7 @@ namespace TableExample
|
|||||||
private static void RenderBigTable()
|
private static void RenderBigTable()
|
||||||
{
|
{
|
||||||
// Create the table.
|
// Create the table.
|
||||||
var table = new Table().SetBorderKind(BorderKind.Rounded);
|
var table = new Table().SetBorder(Border.Rounded);
|
||||||
table.AddColumn("[red underline]Foo[/]");
|
table.AddColumn("[red underline]Foo[/]");
|
||||||
table.AddColumn(new TableColumn("[blue]Bar[/]") { Alignment = Justify.Right, NoWrap = true });
|
table.AddColumn(new TableColumn("[blue]Bar[/]") { Alignment = Justify.Right, NoWrap = true });
|
||||||
|
|
||||||
@ -57,7 +57,7 @@ namespace TableExample
|
|||||||
private static void RenderNestedTable()
|
private static void RenderNestedTable()
|
||||||
{
|
{
|
||||||
// Create simple table.
|
// Create simple table.
|
||||||
var simple = new Table().SetBorderKind(BorderKind.Rounded).SetBorderColor(Color.Red);
|
var simple = new Table().SetBorder(Border.Rounded).SetBorderColor(Color.Red);
|
||||||
simple.AddColumn(new TableColumn("[u]Foo[/]").Centered());
|
simple.AddColumn(new TableColumn("[u]Foo[/]").Centered());
|
||||||
simple.AddColumn(new TableColumn("[u]Bar[/]"));
|
simple.AddColumn(new TableColumn("[u]Bar[/]"));
|
||||||
simple.AddColumn(new TableColumn("[u]Baz[/]"));
|
simple.AddColumn(new TableColumn("[u]Baz[/]"));
|
||||||
@ -66,7 +66,7 @@ namespace TableExample
|
|||||||
simple.AddRow("[blue]Hej[/]", "[yellow]Världen![/]", "");
|
simple.AddRow("[blue]Hej[/]", "[yellow]Världen![/]", "");
|
||||||
|
|
||||||
// Create other table.
|
// Create other table.
|
||||||
var second = new Table().SetBorderKind(BorderKind.Square).SetBorderColor(Color.Green);
|
var second = new Table().SetBorder(Border.Square).SetBorderColor(Color.Green);
|
||||||
second.AddColumn(new TableColumn("[u]Foo[/]"));
|
second.AddColumn(new TableColumn("[u]Foo[/]"));
|
||||||
second.AddColumn(new TableColumn("[u]Bar[/]"));
|
second.AddColumn(new TableColumn("[u]Bar[/]"));
|
||||||
second.AddColumn(new TableColumn("[u]Baz[/]"));
|
second.AddColumn(new TableColumn("[u]Baz[/]"));
|
||||||
@ -74,7 +74,7 @@ namespace TableExample
|
|||||||
second.AddRow(simple, new Text("Whaaat"), new Text("Lolz"));
|
second.AddRow(simple, new Text("Whaaat"), new Text("Lolz"));
|
||||||
second.AddRow("[blue]Hej[/]", "[yellow]Världen![/]", "");
|
second.AddRow("[blue]Hej[/]", "[yellow]Världen![/]", "");
|
||||||
|
|
||||||
var table = new Table().SetBorderKind(BorderKind.Rounded);
|
var table = new Table().SetBorder(Border.Rounded);
|
||||||
table.AddColumn(new TableColumn(new Panel("[u]Foo[/]").SetBorderColor(Color.Red)));
|
table.AddColumn(new TableColumn(new Panel("[u]Foo[/]").SetBorderColor(Color.Red)));
|
||||||
table.AddColumn(new TableColumn(new Panel("[u]Bar[/]").SetBorderColor(Color.Green)));
|
table.AddColumn(new TableColumn(new Panel("[u]Bar[/]").SetBorderColor(Color.Green)));
|
||||||
table.AddColumn(new TableColumn(new Panel("[u]Baz[/]").SetBorderColor(Color.Blue)));
|
table.AddColumn(new TableColumn(new Panel("[u]Baz[/]").SetBorderColor(Color.Blue)));
|
||||||
|
@ -1,41 +1,112 @@
|
|||||||
using System;
|
|
||||||
using Shouldly;
|
using Shouldly;
|
||||||
using Spectre.Console.Rendering;
|
|
||||||
using Xunit;
|
using Xunit;
|
||||||
|
using Spectre.Console.Rendering;
|
||||||
|
|
||||||
namespace Spectre.Console.Tests.Unit
|
namespace Spectre.Console.Tests.Unit
|
||||||
{
|
{
|
||||||
public sealed class BorderTests
|
public sealed class BorderTests
|
||||||
{
|
{
|
||||||
public sealed class TheGetBorderMethod
|
public sealed class NoBorder
|
||||||
{
|
{
|
||||||
[Theory]
|
[Fact]
|
||||||
[InlineData(BorderKind.None, false, typeof(NoBorder))]
|
public void Should_Return_Correct_Visibility()
|
||||||
[InlineData(BorderKind.Ascii, false, typeof(AsciiBorder))]
|
|
||||||
[InlineData(BorderKind.Square, false, typeof(SquareBorder))]
|
|
||||||
[InlineData(BorderKind.Rounded, false, typeof(RoundedBorder))]
|
|
||||||
[InlineData(BorderKind.None, true, typeof(NoBorder))]
|
|
||||||
[InlineData(BorderKind.Ascii, true, typeof(AsciiBorder))]
|
|
||||||
[InlineData(BorderKind.Square, true, typeof(SquareBorder))]
|
|
||||||
[InlineData(BorderKind.Rounded, true, typeof(SquareBorder))]
|
|
||||||
public void Should_Return_Correct_Border_For_Specified_Kind(BorderKind kind, bool safe, Type expected)
|
|
||||||
{
|
{
|
||||||
// Given, When
|
// Given, When
|
||||||
var result = Border.GetBorder(kind, safe);
|
var visibility = Border.None.Visible;
|
||||||
|
|
||||||
// Then
|
// Then
|
||||||
result.ShouldBeOfType(expected);
|
visibility.ShouldBeFalse();
|
||||||
}
|
}
|
||||||
|
|
||||||
|
public sealed class TheSafeGetBorderMethod
|
||||||
|
{
|
||||||
[Fact]
|
[Fact]
|
||||||
public void Should_Throw_If_Unknown_Border_Kind_Is_Specified()
|
public void Should_Return_Safe_Border()
|
||||||
{
|
{
|
||||||
// Given, When
|
// Given, When
|
||||||
var result = Record.Exception(() => Border.GetBorder((BorderKind)int.MaxValue, false));
|
var border = Border.None.GetSafeBorder(safe: true);
|
||||||
|
|
||||||
// Then
|
// Then
|
||||||
result.ShouldBeOfType<InvalidOperationException>();
|
border.ShouldBeSameAs(Border.None);
|
||||||
result.Message.ShouldBe("Unknown border kind");
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
public sealed class AsciiBorder
|
||||||
|
{
|
||||||
|
[Fact]
|
||||||
|
public void Should_Return_Correct_Visibility()
|
||||||
|
{
|
||||||
|
// Given, When
|
||||||
|
var visibility = Border.Ascii.Visible;
|
||||||
|
|
||||||
|
// Then
|
||||||
|
visibility.ShouldBeTrue();
|
||||||
|
}
|
||||||
|
|
||||||
|
public sealed class TheSafeGetBorderMethod
|
||||||
|
{
|
||||||
|
[Fact]
|
||||||
|
public void Should_Return_Safe_Border()
|
||||||
|
{
|
||||||
|
// Given, When
|
||||||
|
var border = Border.Ascii.GetSafeBorder(safe: true);
|
||||||
|
|
||||||
|
// Then
|
||||||
|
border.ShouldBeSameAs(Border.Ascii);
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
public sealed class SquareBorder
|
||||||
|
{
|
||||||
|
[Fact]
|
||||||
|
public void Should_Return_Correct_Visibility()
|
||||||
|
{
|
||||||
|
// Given, When
|
||||||
|
var visibility = Border.Square.Visible;
|
||||||
|
|
||||||
|
// Then
|
||||||
|
visibility.ShouldBeTrue();
|
||||||
|
}
|
||||||
|
|
||||||
|
public sealed class TheSafeGetBorderMethod
|
||||||
|
{
|
||||||
|
[Fact]
|
||||||
|
public void Should_Return_Safe_Border()
|
||||||
|
{
|
||||||
|
// Given, When
|
||||||
|
var border = Border.Square.GetSafeBorder(safe: true);
|
||||||
|
|
||||||
|
// Then
|
||||||
|
border.ShouldBeSameAs(Border.Square);
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
public sealed class RoundedBorder
|
||||||
|
{
|
||||||
|
[Fact]
|
||||||
|
public void Should_Return_Correct_Visibility()
|
||||||
|
{
|
||||||
|
// Given, When
|
||||||
|
var visibility = Border.Rounded.Visible;
|
||||||
|
|
||||||
|
// Then
|
||||||
|
visibility.ShouldBeTrue();
|
||||||
|
}
|
||||||
|
|
||||||
|
public sealed class TheSafeGetBorderMethod
|
||||||
|
{
|
||||||
|
[Fact]
|
||||||
|
public void Should_Return_Safe_Border()
|
||||||
|
{
|
||||||
|
// Given, When
|
||||||
|
var border = Border.Rounded.GetSafeBorder(safe: true);
|
||||||
|
|
||||||
|
// Then
|
||||||
|
border.ShouldBeSameAs(Border.Square);
|
||||||
|
}
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
@ -173,7 +173,7 @@ namespace Spectre.Console.Tests.Unit
|
|||||||
{
|
{
|
||||||
// A simple table
|
// A simple table
|
||||||
var console = new PlainConsole(width: 80);
|
var console = new PlainConsole(width: 80);
|
||||||
var table = new Table() { BorderKind = BorderKind.Rounded };
|
var table = new Table() { Border = Border.Rounded };
|
||||||
table.AddColumn("Foo");
|
table.AddColumn("Foo");
|
||||||
table.AddColumn("Bar");
|
table.AddColumn("Bar");
|
||||||
table.AddColumn(new TableColumn("Baz") { Alignment = Justify.Right });
|
table.AddColumn(new TableColumn("Baz") { Alignment = Justify.Right });
|
||||||
@ -183,7 +183,7 @@ namespace Spectre.Console.Tests.Unit
|
|||||||
// Render a table in some panels.
|
// Render a table in some panels.
|
||||||
console.Render(new Panel(new Panel(table)
|
console.Render(new Panel(new Panel(table)
|
||||||
{
|
{
|
||||||
BorderKind = BorderKind.Ascii,
|
Border = Border.Ascii,
|
||||||
}));
|
}));
|
||||||
|
|
||||||
// Then
|
// Then
|
||||||
@ -255,7 +255,7 @@ namespace Spectre.Console.Tests.Unit
|
|||||||
{
|
{
|
||||||
// Given
|
// Given
|
||||||
var console = new PlainConsole(width: 80);
|
var console = new PlainConsole(width: 80);
|
||||||
var table = new Table { BorderKind = BorderKind.Ascii };
|
var table = new Table { Border = Border.Ascii };
|
||||||
table.AddColumns("Foo", "Bar", "Baz");
|
table.AddColumns("Foo", "Bar", "Baz");
|
||||||
table.AddRow("Qux", "Corgi", "Waldo");
|
table.AddRow("Qux", "Corgi", "Waldo");
|
||||||
table.AddRow("Grault", "Garply", "Fred");
|
table.AddRow("Grault", "Garply", "Fred");
|
||||||
@ -278,7 +278,7 @@ namespace Spectre.Console.Tests.Unit
|
|||||||
{
|
{
|
||||||
// Given
|
// Given
|
||||||
var console = new PlainConsole(width: 80);
|
var console = new PlainConsole(width: 80);
|
||||||
var table = new Table { BorderKind = BorderKind.Rounded };
|
var table = new Table { Border = Border.Rounded };
|
||||||
table.AddColumns("Foo", "Bar", "Baz");
|
table.AddColumns("Foo", "Bar", "Baz");
|
||||||
table.AddRow("Qux", "Corgi", "Waldo");
|
table.AddRow("Qux", "Corgi", "Waldo");
|
||||||
table.AddRow("Grault", "Garply", "Fred");
|
table.AddRow("Grault", "Garply", "Fred");
|
||||||
@ -301,7 +301,7 @@ namespace Spectre.Console.Tests.Unit
|
|||||||
{
|
{
|
||||||
// Given
|
// Given
|
||||||
var console = new PlainConsole(width: 80);
|
var console = new PlainConsole(width: 80);
|
||||||
var table = new Table { BorderKind = BorderKind.None };
|
var table = new Table { Border = Border.None };
|
||||||
table.AddColumns("Foo", "Bar", "Baz");
|
table.AddColumns("Foo", "Bar", "Baz");
|
||||||
table.AddRow("Qux", "Corgi", "Waldo");
|
table.AddRow("Qux", "Corgi", "Waldo");
|
||||||
table.AddRow("Grault", "Garply", "Fred");
|
table.AddRow("Grault", "Garply", "Fred");
|
||||||
|
30
src/Spectre.Console/Rendering/Border.Known.cs
Normal file
30
src/Spectre.Console/Rendering/Border.Known.cs
Normal file
@ -0,0 +1,30 @@
|
|||||||
|
using Spectre.Console.Rendering;
|
||||||
|
|
||||||
|
namespace Spectre.Console
|
||||||
|
{
|
||||||
|
/// <summary>
|
||||||
|
/// Represents a border.
|
||||||
|
/// </summary>
|
||||||
|
public abstract partial class Border
|
||||||
|
{
|
||||||
|
/// <summary>
|
||||||
|
/// Gets an invisible border.
|
||||||
|
/// </summary>
|
||||||
|
public static Border None { get; } = new NoBorder();
|
||||||
|
|
||||||
|
/// <summary>
|
||||||
|
/// Gets an ASCII border.
|
||||||
|
/// </summary>
|
||||||
|
public static Border Ascii { get; } = new AsciiBorder();
|
||||||
|
|
||||||
|
/// <summary>
|
||||||
|
/// Gets a square border.
|
||||||
|
/// </summary>
|
||||||
|
public static Border Square { get; } = new SquareBorder();
|
||||||
|
|
||||||
|
/// <summary>
|
||||||
|
/// Gets a rounded border.
|
||||||
|
/// </summary>
|
||||||
|
public static Border Rounded { get; } = new RoundedBorder();
|
||||||
|
}
|
||||||
|
}
|
@ -2,28 +2,26 @@ using System;
|
|||||||
using System.Collections.Generic;
|
using System.Collections.Generic;
|
||||||
using System.Globalization;
|
using System.Globalization;
|
||||||
using System.Linq;
|
using System.Linq;
|
||||||
|
using Spectre.Console.Rendering;
|
||||||
|
|
||||||
namespace Spectre.Console.Rendering
|
namespace Spectre.Console
|
||||||
{
|
{
|
||||||
/// <summary>
|
/// <summary>
|
||||||
/// Represents a border used by tables.
|
/// Represents a border.
|
||||||
/// </summary>
|
/// </summary>
|
||||||
public abstract class Border
|
public abstract partial class Border
|
||||||
{
|
{
|
||||||
private readonly Dictionary<BorderPart, string> _lookup;
|
private readonly Dictionary<BorderPart, string> _lookup;
|
||||||
|
|
||||||
private static readonly Dictionary<BorderKind, Border> _borders = new Dictionary<BorderKind, Border>
|
/// <summary>
|
||||||
{
|
/// Gets a value indicating whether or not the border is visible.
|
||||||
{ BorderKind.None, new NoBorder() },
|
/// </summary>
|
||||||
{ BorderKind.Ascii, new AsciiBorder() },
|
public virtual bool Visible { get; } = true;
|
||||||
{ BorderKind.Square, new SquareBorder() },
|
|
||||||
{ BorderKind.Rounded, new RoundedBorder() },
|
|
||||||
};
|
|
||||||
|
|
||||||
private static readonly Dictionary<BorderKind, BorderKind> _safeLookup = new Dictionary<BorderKind, BorderKind>
|
/// <summary>
|
||||||
{
|
/// Gets the safe border for this border or <c>null</c> if none exist.
|
||||||
{ BorderKind.Rounded, BorderKind.Square },
|
/// </summary>
|
||||||
};
|
public virtual Border? SafeBorder { get; }
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
/// Initializes a new instance of the <see cref="Border"/> class.
|
/// Initializes a new instance of the <see cref="Border"/> class.
|
||||||
@ -33,27 +31,6 @@ namespace Spectre.Console.Rendering
|
|||||||
_lookup = Initialize();
|
_lookup = Initialize();
|
||||||
}
|
}
|
||||||
|
|
||||||
/// <summary>
|
|
||||||
/// Gets a <see cref="Border"/> represented by the specified <see cref="BorderKind"/>.
|
|
||||||
/// </summary>
|
|
||||||
/// <param name="kind">The kind of border to get.</param>
|
|
||||||
/// <param name="safe">Whether or not to get a "safe" border that can be rendered in a legacy console.</param>
|
|
||||||
/// <returns>A <see cref="Border"/> instance representing the specified <see cref="BorderKind"/>.</returns>
|
|
||||||
public static Border GetBorder(BorderKind kind, bool safe)
|
|
||||||
{
|
|
||||||
if (safe && _safeLookup.TryGetValue(kind, out var safeKind))
|
|
||||||
{
|
|
||||||
kind = safeKind;
|
|
||||||
}
|
|
||||||
|
|
||||||
if (!_borders.TryGetValue(kind, out var border))
|
|
||||||
{
|
|
||||||
throw new InvalidOperationException("Unknown border kind");
|
|
||||||
}
|
|
||||||
|
|
||||||
return border;
|
|
||||||
}
|
|
||||||
|
|
||||||
private Dictionary<BorderPart, string> Initialize()
|
private Dictionary<BorderPart, string> Initialize()
|
||||||
{
|
{
|
||||||
var lookup = new Dictionary<BorderPart, string>();
|
var lookup = new Dictionary<BorderPart, string>();
|
||||||
|
31
src/Spectre.Console/Rendering/BorderExtensions.cs
Normal file
31
src/Spectre.Console/Rendering/BorderExtensions.cs
Normal file
@ -0,0 +1,31 @@
|
|||||||
|
using System;
|
||||||
|
|
||||||
|
namespace Spectre.Console.Rendering
|
||||||
|
{
|
||||||
|
/// <summary>
|
||||||
|
/// Contains extension methods for <see cref="Border"/>.
|
||||||
|
/// </summary>
|
||||||
|
public static class BorderExtensions
|
||||||
|
{
|
||||||
|
/// <summary>
|
||||||
|
/// Gets the safe border for a border.
|
||||||
|
/// </summary>
|
||||||
|
/// <param name="border">The border to get the safe border for.</param>
|
||||||
|
/// <param name="safe">Whether or not to return the safe border.</param>
|
||||||
|
/// <returns>The safe border if one exist, otherwise the original border.</returns>
|
||||||
|
public static Border GetSafeBorder(this Border border, bool safe)
|
||||||
|
{
|
||||||
|
if (border is null)
|
||||||
|
{
|
||||||
|
throw new ArgumentNullException(nameof(border));
|
||||||
|
}
|
||||||
|
|
||||||
|
if (safe && border.SafeBorder != null)
|
||||||
|
{
|
||||||
|
border = border.SafeBorder;
|
||||||
|
}
|
||||||
|
|
||||||
|
return border;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
@ -1,28 +0,0 @@
|
|||||||
namespace Spectre.Console
|
|
||||||
{
|
|
||||||
/// <summary>
|
|
||||||
/// Represents different kinds of borders.
|
|
||||||
/// </summary>
|
|
||||||
public enum BorderKind
|
|
||||||
{
|
|
||||||
/// <summary>
|
|
||||||
/// No border.
|
|
||||||
/// </summary>
|
|
||||||
None = 0,
|
|
||||||
|
|
||||||
/// <summary>
|
|
||||||
/// A square border.
|
|
||||||
/// </summary>
|
|
||||||
Square = 1,
|
|
||||||
|
|
||||||
/// <summary>
|
|
||||||
/// An old school ASCII border.
|
|
||||||
/// </summary>
|
|
||||||
Ascii = 2,
|
|
||||||
|
|
||||||
/// <summary>
|
|
||||||
/// A rounded border.
|
|
||||||
/// </summary>
|
|
||||||
Rounded = 3,
|
|
||||||
}
|
|
||||||
}
|
|
@ -5,6 +5,9 @@ namespace Spectre.Console.Rendering
|
|||||||
/// </summary>
|
/// </summary>
|
||||||
public sealed class NoBorder : Border
|
public sealed class NoBorder : Border
|
||||||
{
|
{
|
||||||
|
/// <inheritdoc/>
|
||||||
|
public override bool Visible => false;
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
protected override string GetBoxPart(BorderPart part)
|
protected override string GetBoxPart(BorderPart part)
|
||||||
{
|
{
|
||||||
|
@ -7,6 +7,9 @@ namespace Spectre.Console.Rendering
|
|||||||
/// </summary>
|
/// </summary>
|
||||||
public sealed class RoundedBorder : Border
|
public sealed class RoundedBorder : Border
|
||||||
{
|
{
|
||||||
|
/// <inheritdoc/>
|
||||||
|
public override Border? SafeBorder { get; } = Border.Square;
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
protected override string GetBoxPart(BorderPart part)
|
protected override string GetBoxPart(BorderPart part)
|
||||||
{
|
{
|
||||||
|
@ -35,7 +35,7 @@ namespace Spectre.Console
|
|||||||
{
|
{
|
||||||
_table = new Table
|
_table = new Table
|
||||||
{
|
{
|
||||||
BorderKind = BorderKind.None,
|
Border = Border.None,
|
||||||
ShowHeaders = false,
|
ShowHeaders = false,
|
||||||
IsGrid = true,
|
IsGrid = true,
|
||||||
PadRightCell = false,
|
PadRightCell = false,
|
||||||
|
@ -2,7 +2,6 @@ using System;
|
|||||||
using System.Collections.Generic;
|
using System.Collections.Generic;
|
||||||
using System.Linq;
|
using System.Linq;
|
||||||
using Spectre.Console.Rendering;
|
using Spectre.Console.Rendering;
|
||||||
using SpectreBorder = Spectre.Console.Rendering.Border;
|
|
||||||
|
|
||||||
namespace Spectre.Console
|
namespace Spectre.Console
|
||||||
{
|
{
|
||||||
@ -16,10 +15,10 @@ namespace Spectre.Console
|
|||||||
private readonly IRenderable _child;
|
private readonly IRenderable _child;
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
public BorderKind BorderKind { get; set; } = BorderKind.Square;
|
public Border Border { get; set; } = Border.Square;
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
public bool SafeBorder { get; set; } = true;
|
public bool UseSafeBorder { get; set; } = true;
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
public Style? BorderStyle { get; set; }
|
public Style? BorderStyle { get; set; }
|
||||||
@ -71,7 +70,7 @@ namespace Spectre.Console
|
|||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
protected override IEnumerable<Segment> Render(RenderContext context, int maxWidth)
|
protected override IEnumerable<Segment> Render(RenderContext context, int maxWidth)
|
||||||
{
|
{
|
||||||
var border = SpectreBorder.GetBorder(BorderKind, (context.LegacyConsole || !context.Unicode) && SafeBorder);
|
var border = Border.GetSafeBorder((context.LegacyConsole || !context.Unicode) && UseSafeBorder);
|
||||||
var borderStyle = BorderStyle ?? Style.Plain;
|
var borderStyle = BorderStyle ?? Style.Plain;
|
||||||
|
|
||||||
var paddingWidth = Padding.GetHorizontalPadding();
|
var paddingWidth = Padding.GetHorizontalPadding();
|
||||||
@ -132,7 +131,7 @@ namespace Spectre.Console
|
|||||||
return result;
|
return result;
|
||||||
}
|
}
|
||||||
|
|
||||||
private static void AddBottomBorder(List<Segment> result, SpectreBorder border, Style borderStyle, int panelWidth)
|
private static void AddBottomBorder(List<Segment> result, Border border, Style borderStyle, int panelWidth)
|
||||||
{
|
{
|
||||||
result.Add(new Segment(border.GetPart(BorderPart.FooterBottomLeft), borderStyle));
|
result.Add(new Segment(border.GetPart(BorderPart.FooterBottomLeft), borderStyle));
|
||||||
result.Add(new Segment(border.GetPart(BorderPart.FooterBottom, panelWidth - EdgeWidth), borderStyle));
|
result.Add(new Segment(border.GetPart(BorderPart.FooterBottom, panelWidth - EdgeWidth), borderStyle));
|
||||||
@ -140,7 +139,7 @@ namespace Spectre.Console
|
|||||||
result.Add(Segment.LineBreak);
|
result.Add(Segment.LineBreak);
|
||||||
}
|
}
|
||||||
|
|
||||||
private void AddTopBorder(List<Segment> segments, RenderContext context, SpectreBorder border, Style borderStyle, int panelWidth)
|
private void AddTopBorder(List<Segment> segments, RenderContext context, Border border, Style borderStyle, int panelWidth)
|
||||||
{
|
{
|
||||||
segments.Add(new Segment(border.GetPart(BorderPart.HeaderTopLeft), borderStyle));
|
segments.Add(new Segment(border.GetPart(BorderPart.HeaderTopLeft), borderStyle));
|
||||||
|
|
||||||
|
@ -117,7 +117,7 @@ namespace Spectre.Console
|
|||||||
|
|
||||||
private int GetExtraWidth(bool includePadding)
|
private int GetExtraWidth(bool includePadding)
|
||||||
{
|
{
|
||||||
var hideBorder = BorderKind == BorderKind.None;
|
var hideBorder = !Border.Visible;
|
||||||
var separators = hideBorder ? 0 : _columns.Count - 1;
|
var separators = hideBorder ? 0 : _columns.Count - 1;
|
||||||
var edges = hideBorder ? 0 : EdgeCount;
|
var edges = hideBorder ? 0 : EdgeCount;
|
||||||
var padding = includePadding ? _columns.Select(x => x.Padding.GetHorizontalPadding()).Sum() : 0;
|
var padding = includePadding ? _columns.Select(x => x.Padding.GetHorizontalPadding()).Sum() : 0;
|
||||||
|
@ -3,7 +3,6 @@ using System.Collections.Generic;
|
|||||||
using System.Linq;
|
using System.Linq;
|
||||||
using Spectre.Console.Internal;
|
using Spectre.Console.Internal;
|
||||||
using Spectre.Console.Rendering;
|
using Spectre.Console.Rendering;
|
||||||
using SpectreBorder = Spectre.Console.Rendering.Border;
|
|
||||||
|
|
||||||
namespace Spectre.Console
|
namespace Spectre.Console
|
||||||
{
|
{
|
||||||
@ -26,13 +25,13 @@ namespace Spectre.Console
|
|||||||
public int RowCount => _rows.Count;
|
public int RowCount => _rows.Count;
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
public BorderKind BorderKind { get; set; } = BorderKind.Square;
|
public Border Border { get; set; } = Border.Square;
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
public Style? BorderStyle { get; set; }
|
public Style? BorderStyle { get; set; }
|
||||||
|
|
||||||
/// <inheritdoc/>
|
/// <inheritdoc/>
|
||||||
public bool SafeBorder { get; set; } = true;
|
public bool UseSafeBorder { get; set; } = true;
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
/// Gets or sets a value indicating whether or not table headers should be shown.
|
/// Gets or sets a value indicating whether or not table headers should be shown.
|
||||||
@ -148,13 +147,13 @@ namespace Spectre.Console
|
|||||||
throw new ArgumentNullException(nameof(context));
|
throw new ArgumentNullException(nameof(context));
|
||||||
}
|
}
|
||||||
|
|
||||||
var border = SpectreBorder.GetBorder(BorderKind, (context.LegacyConsole || !context.Unicode) && SafeBorder);
|
var border = Border.GetSafeBorder((context.LegacyConsole || !context.Unicode) && UseSafeBorder);
|
||||||
var borderStyle = BorderStyle ?? Style.Plain;
|
var borderStyle = BorderStyle ?? Style.Plain;
|
||||||
|
|
||||||
var tableWidth = maxWidth;
|
var tableWidth = maxWidth;
|
||||||
|
|
||||||
var showBorder = BorderKind != BorderKind.None;
|
var showBorder = Border.Visible;
|
||||||
var hideBorder = BorderKind == BorderKind.None;
|
var hideBorder = !Border.Visible;
|
||||||
var hasRows = _rows.Count > 0;
|
var hasRows = _rows.Count > 0;
|
||||||
|
|
||||||
if (Width != null)
|
if (Width != null)
|
||||||
|
@ -16,7 +16,7 @@ namespace Spectre.Console
|
|||||||
public static T NoBorder<T>(this T obj)
|
public static T NoBorder<T>(this T obj)
|
||||||
where T : class, IHasBorder
|
where T : class, IHasBorder
|
||||||
{
|
{
|
||||||
return SetBorderKind(obj, BorderKind.None);
|
return SetBorder(obj, Border.None);
|
||||||
}
|
}
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
@ -28,7 +28,7 @@ namespace Spectre.Console
|
|||||||
public static T SquareBorder<T>(this T obj)
|
public static T SquareBorder<T>(this T obj)
|
||||||
where T : class, IHasBorder
|
where T : class, IHasBorder
|
||||||
{
|
{
|
||||||
return SetBorderKind(obj, BorderKind.Square);
|
return SetBorder(obj, Border.Square);
|
||||||
}
|
}
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
@ -40,7 +40,7 @@ namespace Spectre.Console
|
|||||||
public static T AsciiBorder<T>(this T obj)
|
public static T AsciiBorder<T>(this T obj)
|
||||||
where T : class, IHasBorder
|
where T : class, IHasBorder
|
||||||
{
|
{
|
||||||
return SetBorderKind(obj, BorderKind.Ascii);
|
return SetBorder(obj, Border.Ascii);
|
||||||
}
|
}
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
@ -52,17 +52,17 @@ namespace Spectre.Console
|
|||||||
public static T RoundedBorder<T>(this T obj)
|
public static T RoundedBorder<T>(this T obj)
|
||||||
where T : class, IHasBorder
|
where T : class, IHasBorder
|
||||||
{
|
{
|
||||||
return SetBorderKind(obj, BorderKind.Rounded);
|
return SetBorder(obj, Border.Rounded);
|
||||||
}
|
}
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
/// Sets the border kind.
|
/// Sets the border.
|
||||||
/// </summary>
|
/// </summary>
|
||||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||||
/// <param name="obj">The object to set the border for.</param>
|
/// <param name="obj">The object to set the border for.</param>
|
||||||
/// <param name="border">The border kind to use.</param>
|
/// <param name="border">The border to use.</param>
|
||||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||||
public static T SetBorderKind<T>(this T obj, BorderKind border)
|
public static T SetBorder<T>(this T obj, Border border)
|
||||||
where T : class, IHasBorder
|
where T : class, IHasBorder
|
||||||
{
|
{
|
||||||
if (obj is null)
|
if (obj is null)
|
||||||
@ -70,7 +70,7 @@ namespace Spectre.Console
|
|||||||
throw new ArgumentNullException(nameof(obj));
|
throw new ArgumentNullException(nameof(obj));
|
||||||
}
|
}
|
||||||
|
|
||||||
obj.BorderKind = border;
|
obj.Border = border;
|
||||||
return obj;
|
return obj;
|
||||||
}
|
}
|
||||||
|
|
||||||
@ -88,7 +88,7 @@ namespace Spectre.Console
|
|||||||
throw new ArgumentNullException(nameof(obj));
|
throw new ArgumentNullException(nameof(obj));
|
||||||
}
|
}
|
||||||
|
|
||||||
obj.SafeBorder = false;
|
obj.UseSafeBorder = false;
|
||||||
return obj;
|
return obj;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
@ -10,12 +10,12 @@ namespace Spectre.Console
|
|||||||
/// a "safe" border on legacy consoles that might not be able
|
/// a "safe" border on legacy consoles that might not be able
|
||||||
/// to render non-ASCII characters.
|
/// to render non-ASCII characters.
|
||||||
/// </summary>
|
/// </summary>
|
||||||
bool SafeBorder { get; set; }
|
bool UseSafeBorder { get; set; }
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
/// Gets or sets the kind of border to use.
|
/// Gets or sets the border.
|
||||||
/// </summary>
|
/// </summary>
|
||||||
public BorderKind BorderKind { get; set; }
|
public Border Border { get; set; }
|
||||||
|
|
||||||
/// <summary>
|
/// <summary>
|
||||||
/// Gets or sets the border style.
|
/// Gets or sets the border style.
|
||||||
|
Reference in New Issue
Block a user