mirror of
https://github.com/nsnail/spectre.console.git
synced 2025-07-01 02:28:14 +08:00
Add more borders for grids, tables, and panels
* Ascii2 * AsciiDoubleHead * Double * DoubleEdge * Heavy * HeavyEdge * HeavyHead * Horizontal * Minimal * MinimalDoubleHead * MinimalHeavyHead * Simple * SimpleHeavy
This commit is contained in:
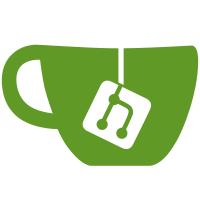
committed by
Patrik Svensson
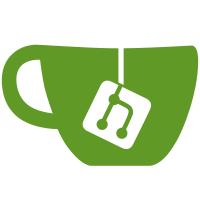
parent
3cc19520b0
commit
3e9796849b
14
examples/Borders/Borders.csproj
Normal file
14
examples/Borders/Borders.csproj
Normal file
@ -0,0 +1,14 @@
|
||||
<Project Sdk="Microsoft.NET.Sdk">
|
||||
|
||||
<PropertyGroup>
|
||||
<OutputType>Exe</OutputType>
|
||||
<TargetFramework>netcoreapp3.1</TargetFramework>
|
||||
<IsPackable>false</IsPackable>
|
||||
<Description>Demonstrates the different kind of borders.</Description>
|
||||
</PropertyGroup>
|
||||
|
||||
<ItemGroup>
|
||||
<ProjectReference Include="..\..\src\Spectre.Console\Spectre.Console.csproj" />
|
||||
</ItemGroup>
|
||||
|
||||
</Project>
|
48
examples/Borders/Program.cs
Normal file
48
examples/Borders/Program.cs
Normal file
@ -0,0 +1,48 @@
|
||||
using System;
|
||||
using Spectre.Console;
|
||||
using Spectre.Console.Rendering;
|
||||
|
||||
namespace Borders
|
||||
{
|
||||
public static class Program
|
||||
{
|
||||
public static void Main()
|
||||
{
|
||||
var items = new[]
|
||||
{
|
||||
Create("Ascii", Border.Ascii),
|
||||
Create("Ascii2", Border.Ascii2),
|
||||
Create("AsciiDoubleHead", Border.AsciiDoubleHead),
|
||||
Create("Horizontal", Border.Horizontal),
|
||||
Create("Simple", Border.Simple),
|
||||
Create("SimpleHeavy", Border.SimpleHeavy),
|
||||
Create("Minimal", Border.Minimal),
|
||||
Create("MinimalHeavyHead", Border.MinimalHeavyHead),
|
||||
Create("MinimalDoubleHead", Border.MinimalDoubleHead),
|
||||
Create("Square", Border.Square),
|
||||
Create("Rounded", Border.Rounded),
|
||||
Create("Heavy", Border.Heavy),
|
||||
Create("HeavyEdge", Border.HeavyEdge),
|
||||
Create("HeavyHead", Border.HeavyHead),
|
||||
Create("Double", Border.Double),
|
||||
Create("DoubleEdge", Border.DoubleEdge),
|
||||
};
|
||||
|
||||
AnsiConsole.WriteLine();
|
||||
AnsiConsole.Render(new Columns(items).Collapse());
|
||||
}
|
||||
|
||||
private static IRenderable Create(string name, Border border)
|
||||
{
|
||||
var table = new Table().SetBorder(border);
|
||||
table.AddColumns("[yellow]Header 1[/]", "[yellow]Header 2[/]");
|
||||
table.AddRow("Cell", "Cell");
|
||||
table.AddRow("Cell", "Cell");
|
||||
|
||||
return new Panel(table)
|
||||
.SetHeader($" {name} ", Style.Parse("blue"), Justify.Center)
|
||||
.SetBorderStyle(Style.Parse("grey"))
|
||||
.NoBorder();
|
||||
}
|
||||
}
|
||||
}
|
@ -1,6 +1,6 @@
|
||||
using Shouldly;
|
||||
using Xunit;
|
||||
using Spectre.Console.Rendering;
|
||||
using Xunit;
|
||||
|
||||
namespace Spectre.Console.Tests.Unit
|
||||
{
|
||||
@ -30,6 +30,23 @@ namespace Spectre.Console.Tests.Unit
|
||||
border.ShouldBeSameAs(Border.None);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().NoBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(3);
|
||||
console.Lines[0].ShouldBe("Header 1 Header 2");
|
||||
console.Lines[1].ShouldBe("Cell Cell ");
|
||||
console.Lines[2].ShouldBe("Cell Cell ");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class AsciiBorder
|
||||
@ -56,6 +73,118 @@ namespace Spectre.Console.Tests.Unit
|
||||
border.ShouldBeSameAs(Border.Ascii);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().AsciiBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("+---------------------+");
|
||||
console.Lines[1].ShouldBe("| Header 1 | Header 2 |");
|
||||
console.Lines[2].ShouldBe("|----------+----------|");
|
||||
console.Lines[3].ShouldBe("| Cell | Cell |");
|
||||
console.Lines[4].ShouldBe("| Cell | Cell |");
|
||||
console.Lines[5].ShouldBe("+---------------------+");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class Ascii2Border
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.Ascii2.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.Ascii2.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Ascii2);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().Ascii2Border();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("+----------+----------+");
|
||||
console.Lines[1].ShouldBe("| Header 1 | Header 2 |");
|
||||
console.Lines[2].ShouldBe("|----------+----------|");
|
||||
console.Lines[3].ShouldBe("| Cell | Cell |");
|
||||
console.Lines[4].ShouldBe("| Cell | Cell |");
|
||||
console.Lines[5].ShouldBe("+----------+----------+");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class AsciiDoubleHeadBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.AsciiDoubleHead.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.AsciiDoubleHead.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.AsciiDoubleHead);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().AsciiDoubleHeadBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("+----------+----------+");
|
||||
console.Lines[1].ShouldBe("| Header 1 | Header 2 |");
|
||||
console.Lines[2].ShouldBe("|==========+==========|");
|
||||
console.Lines[3].ShouldBe("| Cell | Cell |");
|
||||
console.Lines[4].ShouldBe("| Cell | Cell |");
|
||||
console.Lines[5].ShouldBe("+----------+----------+");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class SquareBorder
|
||||
@ -82,6 +211,26 @@ namespace Spectre.Console.Tests.Unit
|
||||
border.ShouldBeSameAs(Border.Square);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().SquareBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("┌──────────┬──────────┐");
|
||||
console.Lines[1].ShouldBe("│ Header 1 │ Header 2 │");
|
||||
console.Lines[2].ShouldBe("├──────────┼──────────┤");
|
||||
console.Lines[3].ShouldBe("│ Cell │ Cell │");
|
||||
console.Lines[4].ShouldBe("│ Cell │ Cell │");
|
||||
console.Lines[5].ShouldBe("└──────────┴──────────┘");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class RoundedBorder
|
||||
@ -108,6 +257,544 @@ namespace Spectre.Console.Tests.Unit
|
||||
border.ShouldBeSameAs(Border.Square);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().RoundedBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("╭──────────┬──────────╮");
|
||||
console.Lines[1].ShouldBe("│ Header 1 │ Header 2 │");
|
||||
console.Lines[2].ShouldBe("├──────────┼──────────┤");
|
||||
console.Lines[3].ShouldBe("│ Cell │ Cell │");
|
||||
console.Lines[4].ShouldBe("│ Cell │ Cell │");
|
||||
console.Lines[5].ShouldBe("╰──────────┴──────────╯");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class MinimalBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.Minimal.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.Minimal.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Minimal);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().MinimalBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe(" ");
|
||||
console.Lines[1].ShouldBe(" Header 1 │ Header 2 ");
|
||||
console.Lines[2].ShouldBe(" ──────────┼────────── ");
|
||||
console.Lines[3].ShouldBe(" Cell │ Cell ");
|
||||
console.Lines[4].ShouldBe(" Cell │ Cell ");
|
||||
console.Lines[5].ShouldBe(" ");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class MinimalHeavyHeadBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.MinimalHeavyHead.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.MinimalHeavyHead.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Minimal);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().MinimalHeavyHeadBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe(" ");
|
||||
console.Lines[1].ShouldBe(" Header 1 │ Header 2 ");
|
||||
console.Lines[2].ShouldBe(" ━━━━━━━━━━┿━━━━━━━━━━ ");
|
||||
console.Lines[3].ShouldBe(" Cell │ Cell ");
|
||||
console.Lines[4].ShouldBe(" Cell │ Cell ");
|
||||
console.Lines[5].ShouldBe(" ");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class MinimalDoubleHeadBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.MinimalDoubleHead.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.MinimalDoubleHead.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.MinimalDoubleHead);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().MinimalDoubleHeadBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe(" ");
|
||||
console.Lines[1].ShouldBe(" Header 1 │ Header 2 ");
|
||||
console.Lines[2].ShouldBe(" ══════════╪══════════ ");
|
||||
console.Lines[3].ShouldBe(" Cell │ Cell ");
|
||||
console.Lines[4].ShouldBe(" Cell │ Cell ");
|
||||
console.Lines[5].ShouldBe(" ");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class SimpleBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.Simple.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.Simple.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Simple);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().SimpleBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe(" ");
|
||||
console.Lines[1].ShouldBe(" Header 1 Header 2 ");
|
||||
console.Lines[2].ShouldBe("───────────────────────");
|
||||
console.Lines[3].ShouldBe(" Cell Cell ");
|
||||
console.Lines[4].ShouldBe(" Cell Cell ");
|
||||
console.Lines[5].ShouldBe(" ");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class HorizontalBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.Horizontal.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.Horizontal.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Horizontal);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().HorizontalBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("───────────────────────");
|
||||
console.Lines[1].ShouldBe(" Header 1 Header 2 ");
|
||||
console.Lines[2].ShouldBe("───────────────────────");
|
||||
console.Lines[3].ShouldBe(" Cell Cell ");
|
||||
console.Lines[4].ShouldBe(" Cell Cell ");
|
||||
console.Lines[5].ShouldBe("───────────────────────");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class SimpleHeavyBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.SimpleHeavy.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.SimpleHeavy.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Simple);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().SimpleHeavyBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe(" ");
|
||||
console.Lines[1].ShouldBe(" Header 1 Header 2 ");
|
||||
console.Lines[2].ShouldBe("━━━━━━━━━━━━━━━━━━━━━━━");
|
||||
console.Lines[3].ShouldBe(" Cell Cell ");
|
||||
console.Lines[4].ShouldBe(" Cell Cell ");
|
||||
console.Lines[5].ShouldBe(" ");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class HeavyBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.Heavy.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.Heavy.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Square);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().HeavyBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("┏━━━━━━━━━━┳━━━━━━━━━━┓");
|
||||
console.Lines[1].ShouldBe("┃ Header 1 ┃ Header 2 ┃");
|
||||
console.Lines[2].ShouldBe("┣━━━━━━━━━━╋━━━━━━━━━━┫");
|
||||
console.Lines[3].ShouldBe("┃ Cell ┃ Cell ┃");
|
||||
console.Lines[4].ShouldBe("┃ Cell ┃ Cell ┃");
|
||||
console.Lines[5].ShouldBe("┗━━━━━━━━━━┻━━━━━━━━━━┛");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class HeavyEdgeBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.HeavyEdge.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.HeavyEdge.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Square);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().HeavyEdgeBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("┏━━━━━━━━━━┯━━━━━━━━━━┓");
|
||||
console.Lines[1].ShouldBe("┃ Header 1 │ Header 2 ┃");
|
||||
console.Lines[2].ShouldBe("┠──────────┼──────────┨");
|
||||
console.Lines[3].ShouldBe("┃ Cell │ Cell ┃");
|
||||
console.Lines[4].ShouldBe("┃ Cell │ Cell ┃");
|
||||
console.Lines[5].ShouldBe("┗━━━━━━━━━━┷━━━━━━━━━━┛");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class HeavyHeadBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.HeavyHead.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.HeavyHead.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Square);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().HeavyHeadBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("┏━━━━━━━━━━┳━━━━━━━━━━┓");
|
||||
console.Lines[1].ShouldBe("┃ Header 1 ┃ Header 2 ┃");
|
||||
console.Lines[2].ShouldBe("┡━━━━━━━━━━╇━━━━━━━━━━┩");
|
||||
console.Lines[3].ShouldBe("│ Cell │ Cell │");
|
||||
console.Lines[4].ShouldBe("│ Cell │ Cell │");
|
||||
console.Lines[5].ShouldBe("└──────────┴──────────┘");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class DoubleBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.Double.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.Double.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.Double);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().DoubleBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("╔══════════╦══════════╗");
|
||||
console.Lines[1].ShouldBe("║ Header 1 ║ Header 2 ║");
|
||||
console.Lines[2].ShouldBe("╠══════════╬══════════╣");
|
||||
console.Lines[3].ShouldBe("║ Cell ║ Cell ║");
|
||||
console.Lines[4].ShouldBe("║ Cell ║ Cell ║");
|
||||
console.Lines[5].ShouldBe("╚══════════╩══════════╝");
|
||||
}
|
||||
}
|
||||
|
||||
public sealed class DoubleEdgeBorder
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Correct_Visibility()
|
||||
{
|
||||
// Given, When
|
||||
var visibility = Border.DoubleEdge.Visible;
|
||||
|
||||
// Then
|
||||
visibility.ShouldBeTrue();
|
||||
}
|
||||
|
||||
public sealed class TheSafeGetBorderMethod
|
||||
{
|
||||
[Fact]
|
||||
public void Should_Return_Safe_Border()
|
||||
{
|
||||
// Given, When
|
||||
var border = Border.DoubleEdge.GetSafeBorder(safe: true);
|
||||
|
||||
// Then
|
||||
border.ShouldBeSameAs(Border.DoubleEdge);
|
||||
}
|
||||
}
|
||||
|
||||
[Fact]
|
||||
public void Should_Render_As_Expected()
|
||||
{
|
||||
// Given
|
||||
var console = new PlainConsole();
|
||||
var table = Fixture.GetTable().DoubleEdgeBorder();
|
||||
|
||||
// When
|
||||
console.Render(table);
|
||||
|
||||
// Then
|
||||
console.Lines.Count.ShouldBe(6);
|
||||
console.Lines[0].ShouldBe("╔══════════╤══════════╗");
|
||||
console.Lines[1].ShouldBe("║ Header 1 │ Header 2 ║");
|
||||
console.Lines[2].ShouldBe("╟──────────┼──────────╢");
|
||||
console.Lines[3].ShouldBe("║ Cell │ Cell ║");
|
||||
console.Lines[4].ShouldBe("║ Cell │ Cell ║");
|
||||
console.Lines[5].ShouldBe("╚══════════╧══════════╝");
|
||||
}
|
||||
}
|
||||
|
||||
private static class Fixture
|
||||
{
|
||||
public static Table GetTable()
|
||||
{
|
||||
var table = new Table();
|
||||
table.AddColumns("Header 1", "Header 2");
|
||||
table.AddRow("Cell", "Cell");
|
||||
table.AddRow("Cell", "Cell");
|
||||
return table;
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
@ -29,6 +29,8 @@ Project("{9A19103F-16F7-4668-BE54-9A1E7A4F7556}") = "Columns", "..\examples\Colu
|
||||
EndProject
|
||||
Project("{9A19103F-16F7-4668-BE54-9A1E7A4F7556}") = "Info", "..\examples\Info\Info.csproj", "{225CE0D4-06AB-411A-8D29-707504FE53B3}"
|
||||
EndProject
|
||||
Project("{FAE04EC0-301F-11D3-BF4B-00C04F79EFBC}") = "Borders", "..\examples\Borders\Borders.csproj", "{094245E6-4C94-485D-B5AC-3153E878B112}"
|
||||
EndProject
|
||||
Global
|
||||
GlobalSection(SolutionConfigurationPlatforms) = preSolution
|
||||
Debug|Any CPU = Debug|Any CPU
|
||||
@ -135,6 +137,18 @@ Global
|
||||
{225CE0D4-06AB-411A-8D29-707504FE53B3}.Release|x64.Build.0 = Release|Any CPU
|
||||
{225CE0D4-06AB-411A-8D29-707504FE53B3}.Release|x86.ActiveCfg = Release|Any CPU
|
||||
{225CE0D4-06AB-411A-8D29-707504FE53B3}.Release|x86.Build.0 = Release|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Debug|Any CPU.ActiveCfg = Debug|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Debug|Any CPU.Build.0 = Debug|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Debug|x64.ActiveCfg = Debug|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Debug|x64.Build.0 = Debug|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Debug|x86.ActiveCfg = Debug|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Debug|x86.Build.0 = Debug|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Release|Any CPU.ActiveCfg = Release|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Release|Any CPU.Build.0 = Release|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Release|x64.ActiveCfg = Release|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Release|x64.Build.0 = Release|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Release|x86.ActiveCfg = Release|Any CPU
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112}.Release|x86.Build.0 = Release|Any CPU
|
||||
EndGlobalSection
|
||||
GlobalSection(SolutionProperties) = preSolution
|
||||
HideSolutionNode = FALSE
|
||||
@ -146,6 +160,7 @@ Global
|
||||
{1F51C55C-BA4C-4856-9001-0F7924FFB179} = {F0575243-121F-4DEE-9F6B-246E26DC0844}
|
||||
{33357599-C79D-4299-888F-634E2C3EACEF} = {F0575243-121F-4DEE-9F6B-246E26DC0844}
|
||||
{225CE0D4-06AB-411A-8D29-707504FE53B3} = {F0575243-121F-4DEE-9F6B-246E26DC0844}
|
||||
{094245E6-4C94-485D-B5AC-3153E878B112} = {F0575243-121F-4DEE-9F6B-246E26DC0844}
|
||||
EndGlobalSection
|
||||
GlobalSection(ExtensibilityGlobals) = postSolution
|
||||
SolutionGuid = {5729B071-67A0-48FB-8B1B-275E6822086C}
|
||||
|
@ -1,3 +1,4 @@
|
||||
using System.Diagnostics.CodeAnalysis;
|
||||
using Spectre.Console.Rendering;
|
||||
|
||||
namespace Spectre.Console
|
||||
@ -17,6 +18,16 @@ namespace Spectre.Console
|
||||
/// </summary>
|
||||
public static Border Ascii { get; } = new AsciiBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets another ASCII border.
|
||||
/// </summary>
|
||||
public static Border Ascii2 { get; } = new Ascii2Border();
|
||||
|
||||
/// <summary>
|
||||
/// Gets an ASCII border with a double header border.
|
||||
/// </summary>
|
||||
public static Border AsciiDoubleHead { get; } = new AsciiDoubleHeadBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a square border.
|
||||
/// </summary>
|
||||
@ -26,5 +37,61 @@ namespace Spectre.Console
|
||||
/// Gets a rounded border.
|
||||
/// </summary>
|
||||
public static Border Rounded { get; } = new RoundedBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a minimal border.
|
||||
/// </summary>
|
||||
public static Border Minimal { get; } = new MinimalBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a minimal border with a heavy head.
|
||||
/// </summary>
|
||||
public static Border MinimalHeavyHead { get; } = new MinimalHeavyHeadBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a minimal border with a double header border.
|
||||
/// </summary>
|
||||
public static Border MinimalDoubleHead { get; } = new MinimalDoubleHeadBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a simple border.
|
||||
/// </summary>
|
||||
public static Border Simple { get; } = new SimpleBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a simple border with heavy lines.
|
||||
/// </summary>
|
||||
public static Border SimpleHeavy { get; } = new SimpleHeavyBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a horizontal border.
|
||||
/// </summary>
|
||||
public static Border Horizontal { get; } = new HorizontalBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a heavy border.
|
||||
/// </summary>
|
||||
public static Border Heavy { get; } = new HeavyBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a border with a heavy edge.
|
||||
/// </summary>
|
||||
public static Border HeavyEdge { get; } = new HeavyEdgeBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a border with a heavy header.
|
||||
/// </summary>
|
||||
public static Border HeavyHead { get; } = new HeavyHeadBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a double border.
|
||||
/// </summary>
|
||||
[SuppressMessage("Naming", "CA1720:Identifier contains type name")]
|
||||
public static Border Double { get; } = new DoubleBorder();
|
||||
|
||||
/// <summary>
|
||||
/// Gets a border with a double edge.
|
||||
/// </summary>
|
||||
public static Border DoubleEdge { get; } = new DoubleEdgeBorder();
|
||||
}
|
||||
}
|
||||
|
37
src/Spectre.Console/Rendering/Borders/Ascii2Border.cs
Normal file
37
src/Spectre.Console/Rendering/Borders/Ascii2Border.cs
Normal file
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents another old school ASCII border.
|
||||
/// </summary>
|
||||
public sealed class Ascii2Border : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "+",
|
||||
BorderPart.HeaderTop => "-",
|
||||
BorderPart.HeaderTopSeparator => "+",
|
||||
BorderPart.HeaderTopRight => "+",
|
||||
BorderPart.HeaderLeft => "|",
|
||||
BorderPart.HeaderSeparator => "|",
|
||||
BorderPart.HeaderRight => "|",
|
||||
BorderPart.HeaderBottomLeft => "|",
|
||||
BorderPart.HeaderBottom => "-",
|
||||
BorderPart.HeaderBottomSeparator => "+",
|
||||
BorderPart.HeaderBottomRight => "|",
|
||||
BorderPart.CellLeft => "|",
|
||||
BorderPart.CellSeparator => "|",
|
||||
BorderPart.CellRight => "|",
|
||||
BorderPart.FooterBottomLeft => "+",
|
||||
BorderPart.FooterBottom => "-",
|
||||
BorderPart.FooterBottomSeparator => "+",
|
||||
BorderPart.FooterBottomRight => "+",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents an old school ASCII border with a double header border.
|
||||
/// </summary>
|
||||
public sealed class AsciiDoubleHeadBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "+",
|
||||
BorderPart.HeaderTop => "-",
|
||||
BorderPart.HeaderTopSeparator => "+",
|
||||
BorderPart.HeaderTopRight => "+",
|
||||
BorderPart.HeaderLeft => "|",
|
||||
BorderPart.HeaderSeparator => "|",
|
||||
BorderPart.HeaderRight => "|",
|
||||
BorderPart.HeaderBottomLeft => "|",
|
||||
BorderPart.HeaderBottom => "=",
|
||||
BorderPart.HeaderBottomSeparator => "+",
|
||||
BorderPart.HeaderBottomRight => "|",
|
||||
BorderPart.CellLeft => "|",
|
||||
BorderPart.CellSeparator => "|",
|
||||
BorderPart.CellRight => "|",
|
||||
BorderPart.FooterBottomLeft => "+",
|
||||
BorderPart.FooterBottom => "-",
|
||||
BorderPart.FooterBottomSeparator => "+",
|
||||
BorderPart.FooterBottomRight => "+",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
37
src/Spectre.Console/Rendering/Borders/DoubleBorder.cs
Normal file
37
src/Spectre.Console/Rendering/Borders/DoubleBorder.cs
Normal file
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a double border.
|
||||
/// </summary>
|
||||
public sealed class DoubleBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "╔",
|
||||
BorderPart.HeaderTop => "═",
|
||||
BorderPart.HeaderTopSeparator => "╦",
|
||||
BorderPart.HeaderTopRight => "╗",
|
||||
BorderPart.HeaderLeft => "║",
|
||||
BorderPart.HeaderSeparator => "║",
|
||||
BorderPart.HeaderRight => "║",
|
||||
BorderPart.HeaderBottomLeft => "╠",
|
||||
BorderPart.HeaderBottom => "═",
|
||||
BorderPart.HeaderBottomSeparator => "╬",
|
||||
BorderPart.HeaderBottomRight => "╣",
|
||||
BorderPart.CellLeft => "║",
|
||||
BorderPart.CellSeparator => "║",
|
||||
BorderPart.CellRight => "║",
|
||||
BorderPart.FooterBottomLeft => "╚",
|
||||
BorderPart.FooterBottom => "═",
|
||||
BorderPart.FooterBottomSeparator => "╩",
|
||||
BorderPart.FooterBottomRight => "╝",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
37
src/Spectre.Console/Rendering/Borders/DoubleEdgeBorder.cs
Normal file
37
src/Spectre.Console/Rendering/Borders/DoubleEdgeBorder.cs
Normal file
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a border with a double edge.
|
||||
/// </summary>
|
||||
public sealed class DoubleEdgeBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "╔",
|
||||
BorderPart.HeaderTop => "═",
|
||||
BorderPart.HeaderTopSeparator => "╤",
|
||||
BorderPart.HeaderTopRight => "╗",
|
||||
BorderPart.HeaderLeft => "║",
|
||||
BorderPart.HeaderSeparator => "│",
|
||||
BorderPart.HeaderRight => "║",
|
||||
BorderPart.HeaderBottomLeft => "╟",
|
||||
BorderPart.HeaderBottom => "─",
|
||||
BorderPart.HeaderBottomSeparator => "┼",
|
||||
BorderPart.HeaderBottomRight => "╢",
|
||||
BorderPart.CellLeft => "║",
|
||||
BorderPart.CellSeparator => "│",
|
||||
BorderPart.CellRight => "║",
|
||||
BorderPart.FooterBottomLeft => "╚",
|
||||
BorderPart.FooterBottom => "═",
|
||||
BorderPart.FooterBottomSeparator => "╧",
|
||||
BorderPart.FooterBottomRight => "╝",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
40
src/Spectre.Console/Rendering/Borders/HeavyBorder.cs
Normal file
40
src/Spectre.Console/Rendering/Borders/HeavyBorder.cs
Normal file
@ -0,0 +1,40 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a heavy border.
|
||||
/// </summary>
|
||||
public sealed class HeavyBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
public override Border? SafeBorder => Border.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "┏",
|
||||
BorderPart.HeaderTop => "━",
|
||||
BorderPart.HeaderTopSeparator => "┳",
|
||||
BorderPart.HeaderTopRight => "┓",
|
||||
BorderPart.HeaderLeft => "┃",
|
||||
BorderPart.HeaderSeparator => "┃",
|
||||
BorderPart.HeaderRight => "┃",
|
||||
BorderPart.HeaderBottomLeft => "┣",
|
||||
BorderPart.HeaderBottom => "━",
|
||||
BorderPart.HeaderBottomSeparator => "╋",
|
||||
BorderPart.HeaderBottomRight => "┫",
|
||||
BorderPart.CellLeft => "┃",
|
||||
BorderPart.CellSeparator => "┃",
|
||||
BorderPart.CellRight => "┃",
|
||||
BorderPart.FooterBottomLeft => "┗",
|
||||
BorderPart.FooterBottom => "━",
|
||||
BorderPart.FooterBottomSeparator => "┻",
|
||||
BorderPart.FooterBottomRight => "┛",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
40
src/Spectre.Console/Rendering/Borders/HeavyEdgeBorder.cs
Normal file
40
src/Spectre.Console/Rendering/Borders/HeavyEdgeBorder.cs
Normal file
@ -0,0 +1,40 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a border with a heavy edge.
|
||||
/// </summary>
|
||||
public sealed class HeavyEdgeBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
public override Border? SafeBorder => Border.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "┏",
|
||||
BorderPart.HeaderTop => "━",
|
||||
BorderPart.HeaderTopSeparator => "┯",
|
||||
BorderPart.HeaderTopRight => "┓",
|
||||
BorderPart.HeaderLeft => "┃",
|
||||
BorderPart.HeaderSeparator => "│",
|
||||
BorderPart.HeaderRight => "┃",
|
||||
BorderPart.HeaderBottomLeft => "┠",
|
||||
BorderPart.HeaderBottom => "─",
|
||||
BorderPart.HeaderBottomSeparator => "┼",
|
||||
BorderPart.HeaderBottomRight => "┨",
|
||||
BorderPart.CellLeft => "┃",
|
||||
BorderPart.CellSeparator => "│",
|
||||
BorderPart.CellRight => "┃",
|
||||
BorderPart.FooterBottomLeft => "┗",
|
||||
BorderPart.FooterBottom => "━",
|
||||
BorderPart.FooterBottomSeparator => "┷",
|
||||
BorderPart.FooterBottomRight => "┛",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
40
src/Spectre.Console/Rendering/Borders/HeavyHeadBorder.cs
Normal file
40
src/Spectre.Console/Rendering/Borders/HeavyHeadBorder.cs
Normal file
@ -0,0 +1,40 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a border with a heavy header.
|
||||
/// </summary>
|
||||
public sealed class HeavyHeadBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
public override Border? SafeBorder => Border.Square;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "┏",
|
||||
BorderPart.HeaderTop => "━",
|
||||
BorderPart.HeaderTopSeparator => "┳",
|
||||
BorderPart.HeaderTopRight => "┓",
|
||||
BorderPart.HeaderLeft => "┃",
|
||||
BorderPart.HeaderSeparator => "┃",
|
||||
BorderPart.HeaderRight => "┃",
|
||||
BorderPart.HeaderBottomLeft => "┡",
|
||||
BorderPart.HeaderBottom => "━",
|
||||
BorderPart.HeaderBottomSeparator => "╇",
|
||||
BorderPart.HeaderBottomRight => "┩",
|
||||
BorderPart.CellLeft => "│",
|
||||
BorderPart.CellSeparator => "│",
|
||||
BorderPart.CellRight => "│",
|
||||
BorderPart.FooterBottomLeft => "└",
|
||||
BorderPart.FooterBottom => "─",
|
||||
BorderPart.FooterBottomSeparator => "┴",
|
||||
BorderPart.FooterBottomRight => "┘",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
37
src/Spectre.Console/Rendering/Borders/HorizontalBorder.cs
Normal file
37
src/Spectre.Console/Rendering/Borders/HorizontalBorder.cs
Normal file
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a horizontal border.
|
||||
/// </summary>
|
||||
public sealed class HorizontalBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => "─",
|
||||
BorderPart.HeaderTop => "─",
|
||||
BorderPart.HeaderTopSeparator => "─",
|
||||
BorderPart.HeaderTopRight => "─",
|
||||
BorderPart.HeaderLeft => " ",
|
||||
BorderPart.HeaderSeparator => " ",
|
||||
BorderPart.HeaderRight => " ",
|
||||
BorderPart.HeaderBottomLeft => "─",
|
||||
BorderPart.HeaderBottom => "─",
|
||||
BorderPart.HeaderBottomSeparator => "─",
|
||||
BorderPart.HeaderBottomRight => "─",
|
||||
BorderPart.CellLeft => " ",
|
||||
BorderPart.CellSeparator => " ",
|
||||
BorderPart.CellRight => " ",
|
||||
BorderPart.FooterBottomLeft => "─",
|
||||
BorderPart.FooterBottom => "─",
|
||||
BorderPart.FooterBottomSeparator => "─",
|
||||
BorderPart.FooterBottomRight => "─",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
37
src/Spectre.Console/Rendering/Borders/MinimalBorder.cs
Normal file
37
src/Spectre.Console/Rendering/Borders/MinimalBorder.cs
Normal file
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a minimal border.
|
||||
/// </summary>
|
||||
public sealed class MinimalBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => " ",
|
||||
BorderPart.HeaderTop => " ",
|
||||
BorderPart.HeaderTopSeparator => " ",
|
||||
BorderPart.HeaderTopRight => " ",
|
||||
BorderPart.HeaderLeft => " ",
|
||||
BorderPart.HeaderSeparator => "│",
|
||||
BorderPart.HeaderRight => " ",
|
||||
BorderPart.HeaderBottomLeft => " ",
|
||||
BorderPart.HeaderBottom => "─",
|
||||
BorderPart.HeaderBottomSeparator => "┼",
|
||||
BorderPart.HeaderBottomRight => " ",
|
||||
BorderPart.CellLeft => " ",
|
||||
BorderPart.CellSeparator => "│",
|
||||
BorderPart.CellRight => " ",
|
||||
BorderPart.FooterBottomLeft => " ",
|
||||
BorderPart.FooterBottom => " ",
|
||||
BorderPart.FooterBottomSeparator => " ",
|
||||
BorderPart.FooterBottomRight => " ",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a minimal border with a double header border.
|
||||
/// </summary>
|
||||
public sealed class MinimalDoubleHeadBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => " ",
|
||||
BorderPart.HeaderTop => " ",
|
||||
BorderPart.HeaderTopSeparator => " ",
|
||||
BorderPart.HeaderTopRight => " ",
|
||||
BorderPart.HeaderLeft => " ",
|
||||
BorderPart.HeaderSeparator => "│",
|
||||
BorderPart.HeaderRight => " ",
|
||||
BorderPart.HeaderBottomLeft => " ",
|
||||
BorderPart.HeaderBottom => "═",
|
||||
BorderPart.HeaderBottomSeparator => "╪",
|
||||
BorderPart.HeaderBottomRight => " ",
|
||||
BorderPart.CellLeft => " ",
|
||||
BorderPart.CellSeparator => "│",
|
||||
BorderPart.CellRight => " ",
|
||||
BorderPart.FooterBottomLeft => " ",
|
||||
BorderPart.FooterBottom => " ",
|
||||
BorderPart.FooterBottomSeparator => " ",
|
||||
BorderPart.FooterBottomRight => " ",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,40 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a minimal border with a heavy header.
|
||||
/// </summary>
|
||||
public sealed class MinimalHeavyHeadBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
public override Border? SafeBorder => Border.Minimal;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => " ",
|
||||
BorderPart.HeaderTop => " ",
|
||||
BorderPart.HeaderTopSeparator => " ",
|
||||
BorderPart.HeaderTopRight => " ",
|
||||
BorderPart.HeaderLeft => " ",
|
||||
BorderPart.HeaderSeparator => "│",
|
||||
BorderPart.HeaderRight => " ",
|
||||
BorderPart.HeaderBottomLeft => " ",
|
||||
BorderPart.HeaderBottom => "━",
|
||||
BorderPart.HeaderBottomSeparator => "┿",
|
||||
BorderPart.HeaderBottomRight => " ",
|
||||
BorderPart.CellLeft => " ",
|
||||
BorderPart.CellSeparator => "│",
|
||||
BorderPart.CellRight => " ",
|
||||
BorderPart.FooterBottomLeft => " ",
|
||||
BorderPart.FooterBottom => " ",
|
||||
BorderPart.FooterBottomSeparator => " ",
|
||||
BorderPart.FooterBottomRight => " ",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
37
src/Spectre.Console/Rendering/Borders/SimpleBorder.cs
Normal file
37
src/Spectre.Console/Rendering/Borders/SimpleBorder.cs
Normal file
@ -0,0 +1,37 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a simple border.
|
||||
/// </summary>
|
||||
public sealed class SimpleBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => " ",
|
||||
BorderPart.HeaderTop => " ",
|
||||
BorderPart.HeaderTopSeparator => " ",
|
||||
BorderPart.HeaderTopRight => " ",
|
||||
BorderPart.HeaderLeft => " ",
|
||||
BorderPart.HeaderSeparator => " ",
|
||||
BorderPart.HeaderRight => " ",
|
||||
BorderPart.HeaderBottomLeft => "─",
|
||||
BorderPart.HeaderBottom => "─",
|
||||
BorderPart.HeaderBottomSeparator => "─",
|
||||
BorderPart.HeaderBottomRight => "─",
|
||||
BorderPart.CellLeft => " ",
|
||||
BorderPart.CellSeparator => " ",
|
||||
BorderPart.CellRight => " ",
|
||||
BorderPart.FooterBottomLeft => " ",
|
||||
BorderPart.FooterBottom => " ",
|
||||
BorderPart.FooterBottomSeparator => " ",
|
||||
BorderPart.FooterBottomRight => " ",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
40
src/Spectre.Console/Rendering/Borders/SimpleHeavyBorder.cs
Normal file
40
src/Spectre.Console/Rendering/Borders/SimpleHeavyBorder.cs
Normal file
@ -0,0 +1,40 @@
|
||||
using System;
|
||||
|
||||
namespace Spectre.Console.Rendering
|
||||
{
|
||||
/// <summary>
|
||||
/// Represents a simple border with heavy lines.
|
||||
/// </summary>
|
||||
public sealed class SimpleHeavyBorder : Border
|
||||
{
|
||||
/// <inheritdoc/>
|
||||
public override Border? SafeBorder => Border.Simple;
|
||||
|
||||
/// <inheritdoc/>
|
||||
protected override string GetBoxPart(BorderPart part)
|
||||
{
|
||||
return part switch
|
||||
{
|
||||
BorderPart.HeaderTopLeft => " ",
|
||||
BorderPart.HeaderTop => " ",
|
||||
BorderPart.HeaderTopSeparator => " ",
|
||||
BorderPart.HeaderTopRight => " ",
|
||||
BorderPart.HeaderLeft => " ",
|
||||
BorderPart.HeaderSeparator => " ",
|
||||
BorderPart.HeaderRight => " ",
|
||||
BorderPart.HeaderBottomLeft => "━",
|
||||
BorderPart.HeaderBottom => "━",
|
||||
BorderPart.HeaderBottomSeparator => "━",
|
||||
BorderPart.HeaderBottomRight => "━",
|
||||
BorderPart.CellLeft => " ",
|
||||
BorderPart.CellSeparator => " ",
|
||||
BorderPart.CellRight => " ",
|
||||
BorderPart.FooterBottomLeft => " ",
|
||||
BorderPart.FooterBottom => " ",
|
||||
BorderPart.FooterBottomSeparator => " ",
|
||||
BorderPart.FooterBottomRight => " ",
|
||||
_ => throw new InvalidOperationException("Unknown box part."),
|
||||
};
|
||||
}
|
||||
}
|
||||
}
|
@ -230,7 +230,8 @@ namespace Spectre.Console
|
||||
if (firstCell && showBorder)
|
||||
{
|
||||
// Show left column edge
|
||||
result.Add(new Segment(border.GetPart(BorderPart.CellLeft), borderStyle));
|
||||
var part = firstRow && ShowHeaders ? BorderPart.HeaderLeft : BorderPart.CellLeft;
|
||||
result.Add(new Segment(border.GetPart(part), borderStyle));
|
||||
}
|
||||
|
||||
// Pad column on left side.
|
||||
@ -266,12 +267,14 @@ namespace Spectre.Console
|
||||
if (lastCell && showBorder)
|
||||
{
|
||||
// Add right column edge
|
||||
result.Add(new Segment(border.GetPart(BorderPart.CellRight), borderStyle));
|
||||
var part = firstRow && ShowHeaders ? BorderPart.HeaderRight : BorderPart.CellRight;
|
||||
result.Add(new Segment(border.GetPart(part), borderStyle));
|
||||
}
|
||||
else if (showBorder)
|
||||
{
|
||||
// Add column separator
|
||||
result.Add(new Segment(border.GetPart(BorderPart.CellSeparator), borderStyle));
|
||||
var part = firstRow && ShowHeaders ? BorderPart.HeaderSeparator : BorderPart.CellSeparator;
|
||||
result.Add(new Segment(border.GetPart(part), borderStyle));
|
||||
}
|
||||
}
|
||||
|
||||
|
@ -43,6 +43,30 @@ namespace Spectre.Console
|
||||
return SetBorder(obj, Border.Ascii);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display another ASCII border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T Ascii2Border<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.Ascii2);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display an ASCII border with a double header border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T AsciiDoubleHeadBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.AsciiDoubleHead);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a rounded border.
|
||||
/// </summary>
|
||||
@ -55,6 +79,138 @@ namespace Spectre.Console
|
||||
return SetBorder(obj, Border.Rounded);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a minimal border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T MinimalBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.Minimal);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a minimal border with a heavy head.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T MinimalHeavyHeadBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.MinimalHeavyHead);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a minimal border with a double header border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T MinimalDoubleHeadBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.MinimalDoubleHead);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a simple border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T SimpleBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.Simple);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a simple border with heavy lines.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T SimpleHeavyBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.SimpleHeavy);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a simple border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T HorizontalBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.Horizontal);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a heavy border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T HeavyBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.Heavy);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a border with a heavy edge.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T HeavyEdgeBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.HeavyEdge);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a border with a heavy header.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T HeavyHeadBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.HeavyHead);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a double border.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T DoubleBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.Double);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Display a border with a double edge.
|
||||
/// </summary>
|
||||
/// <typeparam name="T">An object type with a border.</typeparam>
|
||||
/// <param name="obj">The object to set the border for.</param>
|
||||
/// <returns>The same instance so that multiple calls can be chained.</returns>
|
||||
public static T DoubleEdgeBorder<T>(this T obj)
|
||||
where T : class, IHasBorder
|
||||
{
|
||||
return SetBorder(obj, Border.DoubleEdge);
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
/// Sets the border.
|
||||
/// </summary>
|
||||
|
Reference in New Issue
Block a user