mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-07-06 02:28:16 +08:00
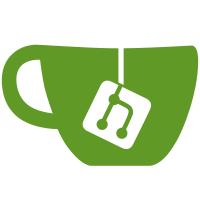
* hacked together load balancing reroutes in fileconfig * some renaming and refactoring * more renames * hacked away the old config json * test for issue 213 * renamed key * dont share ports * oops * updated docs * mvoed docs around * port being used
152 lines
4.9 KiB
C#
152 lines
4.9 KiB
C#
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Security.Claims;
|
|
using Moq;
|
|
using Ocelot.Configuration;
|
|
using Ocelot.Errors;
|
|
using Ocelot.Headers;
|
|
using Ocelot.Infrastructure.Claims.Parser;
|
|
using Ocelot.Responses;
|
|
using Shouldly;
|
|
using TestStack.BDDfy;
|
|
using Xunit;
|
|
using System.Net.Http;
|
|
|
|
namespace Ocelot.UnitTests.Headers
|
|
{
|
|
public class AddHeadersToRequestTests
|
|
{
|
|
private readonly AddHeadersToRequest _addHeadersToRequest;
|
|
private readonly Mock<IClaimsParser> _parser;
|
|
private readonly HttpRequestMessage _downstreamRequest;
|
|
private List<Claim> _claims;
|
|
private List<ClaimToThing> _configuration;
|
|
private Response _result;
|
|
private Response<string> _claimValue;
|
|
|
|
public AddHeadersToRequestTests()
|
|
{
|
|
_parser = new Mock<IClaimsParser>();
|
|
_addHeadersToRequest = new AddHeadersToRequest(_parser.Object);
|
|
_downstreamRequest = new HttpRequestMessage();
|
|
}
|
|
|
|
[Fact]
|
|
public void should_add_headers_to_downstreamRequest()
|
|
{
|
|
var claims = new List<Claim>
|
|
{
|
|
new Claim("test", "data")
|
|
};
|
|
|
|
this.Given(
|
|
x => x.GivenConfigurationHeaderExtractorProperties(new List<ClaimToThing>
|
|
{
|
|
new ClaimToThing("header-key", "", "", 0)
|
|
}))
|
|
.Given(x => x.GivenClaims(claims))
|
|
.And(x => x.GivenTheClaimParserReturns(new OkResponse<string>("value")))
|
|
.When(x => x.WhenIAddHeadersToTheRequest())
|
|
.Then(x => x.ThenTheResultIsSuccess())
|
|
.And(x => x.ThenTheHeaderIsAdded())
|
|
.BDDfy();
|
|
}
|
|
|
|
[Fact]
|
|
public void should_replace_existing_headers_on_request()
|
|
{
|
|
this.Given(
|
|
x => x.GivenConfigurationHeaderExtractorProperties(new List<ClaimToThing>
|
|
{
|
|
new ClaimToThing("header-key", "", "", 0)
|
|
}))
|
|
.Given(x => x.GivenClaims(new List<Claim>
|
|
{
|
|
new Claim("test", "data")
|
|
}))
|
|
.And(x => x.GivenTheClaimParserReturns(new OkResponse<string>("value")))
|
|
.And(x => x.GivenThatTheRequestContainsHeader("header-key", "initial"))
|
|
.When(x => x.WhenIAddHeadersToTheRequest())
|
|
.Then(x => x.ThenTheResultIsSuccess())
|
|
.And(x => x.ThenTheHeaderIsAdded())
|
|
.BDDfy();
|
|
}
|
|
|
|
[Fact]
|
|
public void should_return_error()
|
|
{
|
|
this.Given(
|
|
x => x.GivenConfigurationHeaderExtractorProperties(new List<ClaimToThing>
|
|
{
|
|
new ClaimToThing("", "", "", 0)
|
|
}))
|
|
.Given(x => x.GivenClaims(new List<Claim>()))
|
|
.And(x => x.GivenTheClaimParserReturns(new ErrorResponse<string>(new List<Error>
|
|
{
|
|
new AnyError()
|
|
})))
|
|
.When(x => x.WhenIAddHeadersToTheRequest())
|
|
.Then(x => x.ThenTheResultIsError())
|
|
.BDDfy();
|
|
}
|
|
|
|
private void GivenClaims(List<Claim> claims)
|
|
{
|
|
_claims = claims;
|
|
}
|
|
|
|
private void GivenConfigurationHeaderExtractorProperties(List<ClaimToThing> configuration)
|
|
{
|
|
_configuration = configuration;
|
|
}
|
|
|
|
private void GivenThatTheRequestContainsHeader(string key, string value)
|
|
{
|
|
_downstreamRequest.Headers.Add(key, value);
|
|
}
|
|
|
|
private void GivenTheClaimParserReturns(Response<string> claimValue)
|
|
{
|
|
_claimValue = claimValue;
|
|
_parser
|
|
.Setup(
|
|
x =>
|
|
x.GetValue(It.IsAny<IEnumerable<Claim>>(),
|
|
It.IsAny<string>(),
|
|
It.IsAny<string>(),
|
|
It.IsAny<int>()))
|
|
.Returns(_claimValue);
|
|
}
|
|
|
|
private void WhenIAddHeadersToTheRequest()
|
|
{
|
|
_result = _addHeadersToRequest.SetHeadersOnDownstreamRequest(_configuration, _claims, _downstreamRequest);
|
|
}
|
|
|
|
private void ThenTheResultIsSuccess()
|
|
{
|
|
_result.IsError.ShouldBe(false);
|
|
}
|
|
|
|
private void ThenTheResultIsError()
|
|
{
|
|
|
|
_result.IsError.ShouldBe(true);
|
|
}
|
|
|
|
private void ThenTheHeaderIsAdded()
|
|
{
|
|
var header = _downstreamRequest.Headers.First(x => x.Key == "header-key");
|
|
header.Value.First().ShouldBe(_claimValue.Data);
|
|
}
|
|
|
|
class AnyError : Error
|
|
{
|
|
public AnyError()
|
|
: base("blahh", OcelotErrorCode.UnknownError)
|
|
{
|
|
}
|
|
}
|
|
}
|
|
}
|