mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-25 14:12:50 +08:00
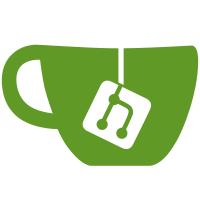
* hacked together load balancing reroutes in fileconfig * some renaming and refactoring * more renames * hacked away the old config json * test for issue 213 * renamed key * dont share ports * oops * updated docs * mvoed docs around * port being used
45 lines
1.2 KiB
C#
45 lines
1.2 KiB
C#
using Xunit;
|
|
using Shouldly;
|
|
using TestStack.BDDfy;
|
|
using Ocelot.Cache;
|
|
using System;
|
|
using Moq;
|
|
using System.Net.Http;
|
|
using System.Collections.Generic;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
|
|
namespace Ocelot.UnitTests.Controllers
|
|
{
|
|
public class OutputCacheControllerTests
|
|
{
|
|
private OutputCacheController _controller;
|
|
private Mock<IOcelotCache<CachedResponse>> _cache;
|
|
private IActionResult _result;
|
|
|
|
public OutputCacheControllerTests()
|
|
{
|
|
_cache = new Mock<IOcelotCache<CachedResponse>>();
|
|
_controller = new OutputCacheController(_cache.Object);
|
|
}
|
|
|
|
[Fact]
|
|
public void should_delete_key()
|
|
{
|
|
this.When(_ => WhenIDeleteTheKey("a"))
|
|
.Then(_ => ThenTheKeyIsDeleted("a"))
|
|
.BDDfy();
|
|
}
|
|
|
|
private void ThenTheKeyIsDeleted(string key)
|
|
{
|
|
_result.ShouldBeOfType<NoContentResult>();
|
|
_cache
|
|
.Verify(x => x.ClearRegion(key), Times.Once);
|
|
}
|
|
|
|
private void WhenIDeleteTheKey(string key)
|
|
{
|
|
_result = _controller.Delete(key);
|
|
}
|
|
}
|
|
} |