mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-07-01 04:48:15 +08:00
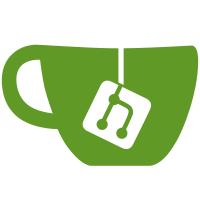
* hacked together load balancing reroutes in fileconfig * some renaming and refactoring * more renames * hacked away the old config json * test for issue 213 * renamed key * dont share ports * oops * updated docs * mvoed docs around * port being used
33 lines
887 B
C#
33 lines
887 B
C#
using System;
|
|
using System.Security.Cryptography;
|
|
using Microsoft.AspNetCore.Cryptography.KeyDerivation;
|
|
using Shouldly;
|
|
using Xunit;
|
|
|
|
namespace Ocelot.UnitTests.Configuration
|
|
{
|
|
public class HashCreationTests
|
|
{
|
|
[Fact]
|
|
public void should_create_hash_and_salt()
|
|
{
|
|
var password = "secret";
|
|
|
|
var salt = new byte[128 / 8];
|
|
|
|
using (var rng = RandomNumberGenerator.Create())
|
|
{
|
|
rng.GetBytes(salt);
|
|
}
|
|
|
|
var storedSalt = Convert.ToBase64String(salt);
|
|
|
|
var storedHash = Convert.ToBase64String(KeyDerivation.Pbkdf2(
|
|
password: password,
|
|
salt: salt,
|
|
prf: KeyDerivationPrf.HMACSHA256,
|
|
iterationCount: 10000,
|
|
numBytesRequested: 256 / 8));
|
|
}
|
|
}
|
|
} |