mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-07-06 02:28:16 +08:00
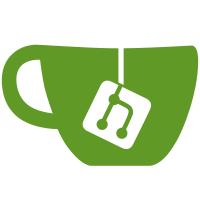
* brought in rafty * moved raft classes into Ocelot and deleted from int project * started to set up rafty in Ocelot * RAFTY INSIDE OCELOT...WOOT * more work adding rafty...just need to get auth working now * rudimentary authenticated raft requests working * asyn await stuff * hacked rafty into the fileconfigurationcontroller...everything seems to be working roughly but I have a lot of refactoring to do * updated to latest rafty that doesnt need an id * hacky but all tests passing * changed admin area set up to use builder not configuration.json, changed admin area auth to use client credentials * missing code coverage * ignore raft sectionf for code coverage * ignore raft sectionf for code coverage * back to normal filters * try exclude attr * missed these * moved client secret to builder for authentication and updated docs * lock to try and fix error accessing identity server created temprsa file on build server * updated postman scripts and changed Ocelot to not always use type handling as this looked crap when manually accessing the configuration endpoint * added rafty docs * changes I missed * added serialisation code we need for rafty to process commands when they proxy to leader * moved controllers into their feature slices
56 lines
1.8 KiB
C#
56 lines
1.8 KiB
C#
using System;
|
|
using System.IO;
|
|
using System.Linq;
|
|
using Microsoft.AspNetCore.Builder;
|
|
using Microsoft.AspNetCore.Hosting;
|
|
using Microsoft.AspNetCore.Http;
|
|
using Microsoft.Extensions.Configuration;
|
|
using Microsoft.Extensions.DependencyInjection;
|
|
using Microsoft.Extensions.Logging;
|
|
using Newtonsoft.Json;
|
|
using Ocelot.DependencyInjection;
|
|
using Ocelot.Middleware;
|
|
using Ocelot.Raft;
|
|
using Rafty.Concensus;
|
|
using Rafty.FiniteStateMachine;
|
|
using Rafty.Infrastructure;
|
|
using Rafty.Log;
|
|
using ConfigurationBuilder = Microsoft.Extensions.Configuration.ConfigurationBuilder;
|
|
|
|
namespace Ocelot.IntegrationTests
|
|
{
|
|
public class RaftStartup
|
|
{
|
|
public RaftStartup(IHostingEnvironment env)
|
|
{
|
|
var builder = new ConfigurationBuilder()
|
|
.SetBasePath(env.ContentRootPath)
|
|
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
|
|
.AddJsonFile($"appsettings.{env.EnvironmentName}.json", optional: true)
|
|
.AddJsonFile("peers.json", optional: true, reloadOnChange: true)
|
|
.AddJsonFile("configuration.json")
|
|
.AddEnvironmentVariables();
|
|
|
|
Configuration = builder.Build();
|
|
}
|
|
|
|
public IConfigurationRoot Configuration { get; }
|
|
|
|
public virtual void ConfigureServices(IServiceCollection services)
|
|
{
|
|
services
|
|
.AddOcelot(Configuration)
|
|
.AddAdministration("/administration", "secret")
|
|
.AddRafty();
|
|
}
|
|
|
|
public virtual void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
|
|
{
|
|
|
|
//this is from Ocelot...so we need to move stuff below into it...
|
|
loggerFactory.AddConsole(Configuration.GetSection("Logging"));
|
|
app.UseOcelot().Wait();
|
|
}
|
|
}
|
|
}
|