mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-22 14:02:49 +08:00
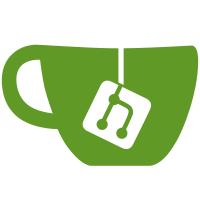
* moving things around to see if I can get consul to store fileconfiguration rather than ocelotconfiguration * more refactoring to see if we can get a test for the feature * acceptance test passing for updating in consul..need to sort object comparison out * fixed the failing tests
55 lines
1.2 KiB
C#
55 lines
1.2 KiB
C#
using System;
|
|
using System.Diagnostics;
|
|
|
|
namespace Ocelot.UnitTests
|
|
{
|
|
public class Wait
|
|
{
|
|
public static Waiter WaitFor(int milliSeconds)
|
|
{
|
|
return new Waiter(milliSeconds);
|
|
}
|
|
}
|
|
|
|
public class Waiter
|
|
{
|
|
private readonly int _milliSeconds;
|
|
|
|
public Waiter(int milliSeconds)
|
|
{
|
|
_milliSeconds = milliSeconds;
|
|
}
|
|
|
|
public bool Until(Func<bool> condition)
|
|
{
|
|
var stopwatch = Stopwatch.StartNew();
|
|
var passed = false;
|
|
while (stopwatch.ElapsedMilliseconds < _milliSeconds)
|
|
{
|
|
if (condition.Invoke())
|
|
{
|
|
passed = true;
|
|
break;
|
|
}
|
|
}
|
|
|
|
return passed;
|
|
}
|
|
|
|
public bool Until<T>(Func<bool> condition)
|
|
{
|
|
var stopwatch = Stopwatch.StartNew();
|
|
var passed = false;
|
|
while (stopwatch.ElapsedMilliseconds < _milliSeconds)
|
|
{
|
|
if (condition.Invoke())
|
|
{
|
|
passed = true;
|
|
break;
|
|
}
|
|
}
|
|
|
|
return passed;
|
|
}
|
|
}
|
|
} |