mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-05-01 10:12:51 +08:00
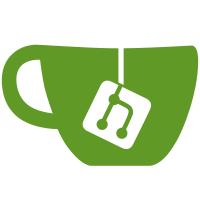
* moving things around to see if I can get consul to store fileconfiguration rather than ocelotconfiguration * more refactoring to see if we can get a test for the feature * acceptance test passing for updating in consul..need to sort object comparison out * fixed the failing tests
42 lines
1.3 KiB
C#
42 lines
1.3 KiB
C#
using System.Threading.Tasks;
|
|
using Ocelot.Configuration.Creator;
|
|
using Ocelot.Configuration.File;
|
|
using Ocelot.Configuration.Repository;
|
|
using Ocelot.Responses;
|
|
|
|
namespace Ocelot.Configuration.Setter
|
|
{
|
|
public class FileConfigurationSetter : IFileConfigurationSetter
|
|
{
|
|
private readonly IOcelotConfigurationRepository _configRepo;
|
|
private readonly IOcelotConfigurationCreator _configCreator;
|
|
private readonly IFileConfigurationRepository _repo;
|
|
|
|
public FileConfigurationSetter(IOcelotConfigurationRepository configRepo,
|
|
IOcelotConfigurationCreator configCreator, IFileConfigurationRepository repo)
|
|
{
|
|
_configRepo = configRepo;
|
|
_configCreator = configCreator;
|
|
_repo = repo;
|
|
}
|
|
|
|
public async Task<Response> Set(FileConfiguration fileConfig)
|
|
{
|
|
var response = await _repo.Set(fileConfig);
|
|
|
|
if(response.IsError)
|
|
{
|
|
return new ErrorResponse(response.Errors);
|
|
}
|
|
|
|
var config = await _configCreator.Create(fileConfig);
|
|
|
|
if(!config.IsError)
|
|
{
|
|
await _configRepo.AddOrReplace(config.Data);
|
|
}
|
|
|
|
return new ErrorResponse(config.Errors);
|
|
}
|
|
}
|
|
} |