mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-25 07:02:51 +08:00
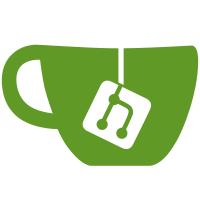
* #574 consolidate some code, man the config stuff is a mess! * #574 just use the downstream re route and the key for caching http clients * #574 added benchmark, i was suprised to learn using a complex type was faster than a string in benchmark .net dictionary tests, hey ho probably dont have enough data in the type...
78 lines
4.1 KiB
C#
78 lines
4.1 KiB
C#
using BenchmarkDotNet.Attributes;
|
|
using BenchmarkDotNet.Columns;
|
|
using BenchmarkDotNet.Configs;
|
|
using System.Net.Http;
|
|
using BenchmarkDotNet.Diagnosers;
|
|
using BenchmarkDotNet.Validators;
|
|
|
|
namespace Ocelot.Benchmarks
|
|
{
|
|
using System.Collections.Concurrent;
|
|
using Configuration;
|
|
using Configuration.Builder;
|
|
using Requester;
|
|
|
|
[Config(typeof(DictionaryBenchmarks))]
|
|
public class DictionaryBenchmarks : ManualConfig
|
|
{
|
|
private ConcurrentDictionary<DownstreamReRoute, IHttpClient> _downstreamReRouteDictionary;
|
|
private ConcurrentDictionary<string, IHttpClient> _stringReRouteDictionary;
|
|
private HttpClientWrapper _client;
|
|
private string _stringKey;
|
|
private DownstreamReRoute _downstreamReRouteKey;
|
|
|
|
public DictionaryBenchmarks()
|
|
{
|
|
Add(StatisticColumn.AllStatistics);
|
|
Add(MemoryDiagnoser.Default);
|
|
Add(BaselineValidator.FailOnError);
|
|
}
|
|
|
|
[GlobalSetup]
|
|
public void SetUp()
|
|
{
|
|
_downstreamReRouteKey = new DownstreamReRouteBuilder().Build();
|
|
_stringKey = "test";
|
|
_client = new HttpClientWrapper(new HttpClient());
|
|
_downstreamReRouteDictionary = new ConcurrentDictionary<DownstreamReRoute, IHttpClient>();
|
|
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
_downstreamReRouteDictionary.TryAdd(new DownstreamReRouteBuilder().Build(), new HttpClientWrapper(new HttpClient()));
|
|
|
|
_stringReRouteDictionary = new ConcurrentDictionary<string, IHttpClient>();
|
|
_stringReRouteDictionary.TryAdd("1", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("2", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("3", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("4", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("5", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("6", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("7", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("8", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("9", new HttpClientWrapper(new HttpClient()));
|
|
_stringReRouteDictionary.TryAdd("10", new HttpClientWrapper(new HttpClient()));
|
|
}
|
|
|
|
[Benchmark(Baseline = true)]
|
|
public IHttpClient StringKey()
|
|
{
|
|
_stringReRouteDictionary.AddOrUpdate(_stringKey, _client, (k, oldValue) => _client);
|
|
return _stringReRouteDictionary.TryGetValue(_stringKey, out var client) ? client : null;
|
|
}
|
|
|
|
[Benchmark]
|
|
public IHttpClient DownstreamReRouteKey()
|
|
{
|
|
_downstreamReRouteDictionary.AddOrUpdate(_downstreamReRouteKey, _client, (k, oldValue) => _client);
|
|
return _downstreamReRouteDictionary.TryGetValue(_downstreamReRouteKey, out var client) ? client : null;
|
|
}
|
|
}
|
|
}
|