mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-25 16:32:51 +08:00
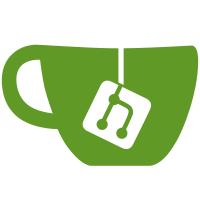
Added LogError(string) to interface as sometimes there isn't an exception to be logged. Additionally, split Logger and LoggerFactory implementations into seperate files just for tidiness. Additonally added some very basic unit tests to the HttpDataRepository as a bit of regression safety and to prove that Get never returns a null. Slightly refactored the logic within AspDotNetLogger under GetMessageWithOcelotRequestId so that the if statement is a little easier to read. Attempted to remove the requestId == null, however this broke numerous tests as the mocks don't set the behviour for dataReposioty getting the requestId
74 lines
2.6 KiB
C#
74 lines
2.6 KiB
C#
using Microsoft.AspNetCore.Http;
|
|
using Ocelot.Infrastructure.RequestData;
|
|
using Ocelot.Responses;
|
|
using Shouldly;
|
|
using TestStack.BDDfy;
|
|
using Xunit;
|
|
|
|
namespace Ocelot.UnitTests.Infrastructure
|
|
{
|
|
public class HttpDataRepositoryTests
|
|
{
|
|
private HttpContext _httpContext;
|
|
private IHttpContextAccessor _httpContextAccessor;
|
|
private HttpDataRepository _httpDataRepository;
|
|
private object _result;
|
|
|
|
public HttpDataRepositoryTests()
|
|
{
|
|
_httpContext = new DefaultHttpContext();
|
|
_httpContextAccessor = new HttpContextAccessor{HttpContext = _httpContext};
|
|
_httpDataRepository = new HttpDataRepository(_httpContextAccessor);
|
|
}
|
|
|
|
//TODO - Additional tests -> Type mistmatch aka Add string, request int
|
|
//TODO - Additional tests -> HttpContent null. This should never happen
|
|
|
|
[Fact]
|
|
public void Get_returns_correct_key_from_http_context()
|
|
{
|
|
|
|
this.Given(x => x.GivenAHttpContextContaining("key", "string"))
|
|
.When(x => x.GetIsCalledWithKey<string>("key"))
|
|
.Then(x => x.ThenTheResultIsAnOkResponse<string>("string"))
|
|
.BDDfy();
|
|
}
|
|
|
|
[Fact]
|
|
public void Get_returns_error_response_if_the_key_is_not_found() //Therefore does not return null
|
|
{
|
|
this.Given(x => x.GivenAHttpContextContaining("key", "string"))
|
|
.When(x => x.GetIsCalledWithKey<string>("keyDoesNotExist"))
|
|
.Then(x => x.ThenTheResultIsAnErrorReposnse<string>("string1"))
|
|
.BDDfy();
|
|
}
|
|
|
|
private void GivenAHttpContextContaining(string key, object o)
|
|
{
|
|
_httpContext.Items.Add(key, o);
|
|
}
|
|
|
|
private void GetIsCalledWithKey<T>(string key)
|
|
{
|
|
_result = _httpDataRepository.Get<T>(key);
|
|
}
|
|
|
|
private void ThenTheResultIsAnErrorReposnse<T>(object resultValue)
|
|
{
|
|
_result.ShouldBeOfType<ErrorResponse<T>>();
|
|
((ErrorResponse<T>) _result).Data.ShouldBeNull();
|
|
((ErrorResponse<T>)_result).IsError.ShouldBe(true);
|
|
((ErrorResponse<T>) _result).Errors.ShouldHaveSingleItem()
|
|
.ShouldBeOfType<CannotFindDataError>()
|
|
.Message.ShouldStartWith("Unable to find data for key: ");
|
|
}
|
|
|
|
private void ThenTheResultIsAnOkResponse<T>(object resultValue)
|
|
{
|
|
_result.ShouldBeOfType<OkResponse<T>>();
|
|
((OkResponse<T>)_result).Data.ShouldBe(resultValue);
|
|
}
|
|
|
|
}
|
|
}
|