mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-07-06 07:08:14 +08:00
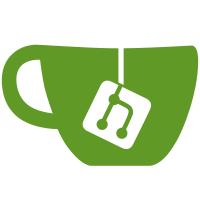
* feat: update to asp.net core 3.0 preview 9 * fix : AspDotNetLogger unittest * feat: update generic host and useMvc 1、Using 'UseMvc' to configure MVC is not supported while using Endpoint Routing https://github.com/aspnet/AspNetCore/issues/9542 2、 use IHost and IHostBuilder * feat : update .net core 3.0 rc1 * eureka extension * fixed logger formatter error * fixed synchronous operations are disallowed of ReadToEnd method * fix log tests * Flush method of FakeStream should do nothing * Update ContentTests.cs * Fixed ws tests * feat: delelte comment code * feat: update .net core 3.0 RTM * Update OcelotBuilderTests.cs * Update .travis.yml mono 6.0.0 and dotnet 3.0.100 * Update Ocelot.IntegrationTests.csproj update Microsoft.Data.SQLite 3.0.0 * Update .travis.yml * feat: remove FrameworkReference 1、 remove FrameworkReference 2、 update package * add appveyor configuration to use version of VS2019 with dotnet core 3 sdk support * update obsoleted SetCollectionValidator method * Swap out OpenCover for Coverlet * Bump Cake to 0.35.0 * Downgrade coveralls.net to 0.7.0 Fix disposing of PollConsul instance * Remove environment specific path separator * Do not return ReportGenerator on Mac/Linux * Remove direct dependency on IInternalConfiguration * Fix ordering of variable assignment * Fix broken tests * Fix acceptance tests for Consul
81 lines
2.7 KiB
C#
81 lines
2.7 KiB
C#
namespace Ocelot.UnitTests.Logging
|
|
{
|
|
using Microsoft.Extensions.Logging;
|
|
using Moq;
|
|
using Ocelot.Infrastructure.RequestData;
|
|
using Ocelot.Logging;
|
|
using System;
|
|
using Xunit;
|
|
|
|
public class AspDotNetLoggerTests
|
|
{
|
|
private readonly Mock<ILogger<object>> _coreLogger;
|
|
private readonly Mock<IRequestScopedDataRepository> _repo;
|
|
private readonly AspDotNetLogger _logger;
|
|
private readonly string _b;
|
|
private readonly string _a;
|
|
private readonly Exception _ex;
|
|
|
|
public AspDotNetLoggerTests()
|
|
{
|
|
_a = "tom";
|
|
_b = "laura";
|
|
_ex = new Exception("oh no");
|
|
_coreLogger = new Mock<ILogger<object>>();
|
|
_repo = new Mock<IRequestScopedDataRepository>();
|
|
_logger = new AspDotNetLogger(_coreLogger.Object, _repo.Object);
|
|
}
|
|
|
|
[Fact]
|
|
public void should_log_trace()
|
|
{
|
|
_logger.LogTrace($"a message from {_a} to {_b}");
|
|
|
|
ThenLevelIsLogged("requestId: no request id, previousRequestId: no previous request id, message: a message from tom to laura", LogLevel.Trace);
|
|
}
|
|
|
|
[Fact]
|
|
public void should_log_info()
|
|
{
|
|
_logger.LogInformation($"a message from {_a} to {_b}");
|
|
|
|
ThenLevelIsLogged("requestId: no request id, previousRequestId: no previous request id, message: a message from tom to laura", LogLevel.Information);
|
|
}
|
|
|
|
[Fact]
|
|
public void should_log_warning()
|
|
{
|
|
_logger.LogWarning($"a message from {_a} to {_b}");
|
|
|
|
ThenLevelIsLogged("requestId: no request id, previousRequestId: no previous request id, message: a message from tom to laura", LogLevel.Warning);
|
|
}
|
|
|
|
[Fact]
|
|
public void should_log_error()
|
|
{
|
|
_logger.LogError($"a message from {_a} to {_b}", _ex);
|
|
|
|
ThenLevelIsLogged("requestId: no request id, previousRequestId: no previous request id, message: a message from tom to laura", LogLevel.Error, _ex);
|
|
}
|
|
|
|
[Fact]
|
|
public void should_log_critical()
|
|
{
|
|
_logger.LogCritical($"a message from {_a} to {_b}", _ex);
|
|
|
|
ThenLevelIsLogged("requestId: no request id, previousRequestId: no previous request id, message: a message from tom to laura", LogLevel.Critical, _ex);
|
|
}
|
|
|
|
private void ThenLevelIsLogged(string expected, LogLevel expectedLogLevel, Exception ex = null)
|
|
{
|
|
_coreLogger.Verify(
|
|
x => x.Log(
|
|
expectedLogLevel,
|
|
default(EventId),
|
|
expected,
|
|
ex,
|
|
It.IsAny<Func<string, Exception, string>>()), Times.Once);
|
|
}
|
|
}
|
|
}
|