mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-26 14:32:51 +08:00
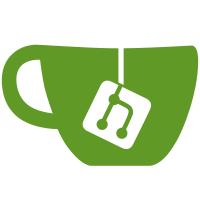
* tidy up some code so I can understand it * broke al the things that make config out into their own classes etc..sigh * fix the things i broked * #597 test for issue, works on this branch :E * #597 removed comments * added tests for new load balancer creator..basic * added tests for lb creator and aggregates creator * added tests for config creator * boiler plate for tests * added dynamics tests * wip * finished refactoring for now
55 lines
1.6 KiB
C#
55 lines
1.6 KiB
C#
namespace Ocelot.UnitTests.Configuration
|
|
{
|
|
using Ocelot.Configuration;
|
|
using Ocelot.Configuration.Creator;
|
|
using Ocelot.Configuration.File;
|
|
using Shouldly;
|
|
using TestStack.BDDfy;
|
|
using Xunit;
|
|
|
|
public class LoadBalancerOptionsCreatorTests
|
|
{
|
|
private readonly ILoadBalancerOptionsCreator _creator;
|
|
private FileLoadBalancerOptions _fileLoadBalancerOptions;
|
|
private LoadBalancerOptions _result;
|
|
|
|
public LoadBalancerOptionsCreatorTests()
|
|
{
|
|
_creator = new LoadBalancerOptionsCreator();
|
|
}
|
|
|
|
[Fact]
|
|
public void should_create()
|
|
{
|
|
var fileLoadBalancerOptions = new FileLoadBalancerOptions
|
|
{
|
|
Type = "test",
|
|
Key = "west",
|
|
Expiry = 1
|
|
};
|
|
|
|
this.Given(_ => GivenThe(fileLoadBalancerOptions))
|
|
.When(_ => WhenICreate())
|
|
.Then(_ => ThenTheOptionsAreCreated(fileLoadBalancerOptions))
|
|
.BDDfy();
|
|
}
|
|
|
|
private void ThenTheOptionsAreCreated(FileLoadBalancerOptions expected)
|
|
{
|
|
_result.Type.ShouldBe(expected.Type);
|
|
_result.Key.ShouldBe(expected.Key);
|
|
_result.ExpiryInMs.ShouldBe(expected.Expiry);
|
|
}
|
|
|
|
private void WhenICreate()
|
|
{
|
|
_result = _creator.Create(_fileLoadBalancerOptions);
|
|
}
|
|
|
|
private void GivenThe(FileLoadBalancerOptions fileLoadBalancerOptions)
|
|
{
|
|
_fileLoadBalancerOptions = fileLoadBalancerOptions;
|
|
}
|
|
}
|
|
}
|