mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-07-03 12:08:16 +08:00
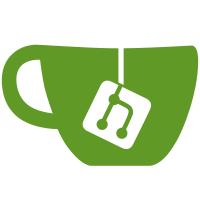
* feat: update to asp.net core 3.0 preview 9 * fix : AspDotNetLogger unittest * feat: update generic host and useMvc 1、Using 'UseMvc' to configure MVC is not supported while using Endpoint Routing https://github.com/aspnet/AspNetCore/issues/9542 2、 use IHost and IHostBuilder * feat : update .net core 3.0 rc1 * eureka extension * fixed logger formatter error * fixed synchronous operations are disallowed of ReadToEnd method * fix log tests * Flush method of FakeStream should do nothing * Update ContentTests.cs * Fixed ws tests * feat: delelte comment code * feat: update .net core 3.0 RTM * Update OcelotBuilderTests.cs * Update .travis.yml mono 6.0.0 and dotnet 3.0.100 * Update Ocelot.IntegrationTests.csproj update Microsoft.Data.SQLite 3.0.0 * Update .travis.yml * feat: remove FrameworkReference 1、 remove FrameworkReference 2、 update package * add appveyor configuration to use version of VS2019 with dotnet core 3 sdk support * update obsoleted SetCollectionValidator method * Swap out OpenCover for Coverlet * Bump Cake to 0.35.0 * Downgrade coveralls.net to 0.7.0 Fix disposing of PollConsul instance * Remove environment specific path separator * Do not return ReportGenerator on Mac/Linux * Remove direct dependency on IInternalConfiguration * Fix ordering of variable assignment * Fix broken tests * Fix acceptance tests for Consul
112 lines
3.9 KiB
C#
112 lines
3.9 KiB
C#
namespace Ocelot.UnitTests.CacheManager
|
|
{
|
|
using global::CacheManager.Core;
|
|
using Microsoft.AspNetCore.Hosting;
|
|
using Microsoft.Extensions.Configuration;
|
|
using Microsoft.Extensions.DependencyInjection;
|
|
using Microsoft.Extensions.Hosting.Internal;
|
|
using Moq;
|
|
using Ocelot.Cache;
|
|
using Ocelot.Cache.CacheManager;
|
|
using Ocelot.Configuration;
|
|
using Ocelot.Configuration.File;
|
|
using Ocelot.DependencyInjection;
|
|
using Shouldly;
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Reflection;
|
|
using TestStack.BDDfy;
|
|
using Xunit;
|
|
|
|
public class OcelotBuilderExtensionsTests
|
|
{
|
|
private readonly IServiceCollection _services;
|
|
private IServiceProvider _serviceProvider;
|
|
private readonly IConfiguration _configRoot;
|
|
private IOcelotBuilder _ocelotBuilder;
|
|
private readonly int _maxRetries;
|
|
private Exception _ex;
|
|
|
|
public OcelotBuilderExtensionsTests()
|
|
{
|
|
_configRoot = new ConfigurationRoot(new List<IConfigurationProvider>());
|
|
_services = new ServiceCollection();
|
|
_services.AddSingleton<IWebHostEnvironment>(GetHostingEnvironment());
|
|
_services.AddSingleton(_configRoot);
|
|
_maxRetries = 100;
|
|
}
|
|
|
|
private IWebHostEnvironment GetHostingEnvironment()
|
|
{
|
|
var environment = new Mock<IWebHostEnvironment>();
|
|
environment
|
|
.Setup(e => e.ApplicationName)
|
|
.Returns(typeof(OcelotBuilderExtensionsTests).GetTypeInfo().Assembly.GetName().Name);
|
|
|
|
return environment.Object;
|
|
}
|
|
|
|
[Fact]
|
|
public void should_set_up_cache_manager()
|
|
{
|
|
this.Given(x => WhenISetUpOcelotServices())
|
|
.When(x => WhenISetUpCacheManager())
|
|
.Then(x => ThenAnExceptionIsntThrown())
|
|
.And(x => OnlyOneVersionOfEachCacheIsRegistered())
|
|
.BDDfy();
|
|
}
|
|
|
|
private void OnlyOneVersionOfEachCacheIsRegistered()
|
|
{
|
|
var outputCache = _services.Single(x => x.ServiceType == typeof(IOcelotCache<CachedResponse>));
|
|
var outputCacheManager = _services.Single(x => x.ServiceType == typeof(ICacheManager<CachedResponse>));
|
|
var instance = (ICacheManager<CachedResponse>)outputCacheManager.ImplementationInstance;
|
|
var ocelotConfigCache = _services.Single(x => x.ServiceType == typeof(IOcelotCache<IInternalConfiguration>));
|
|
var ocelotConfigCacheManager = _services.Single(x => x.ServiceType == typeof(ICacheManager<IInternalConfiguration>));
|
|
var fileConfigCache = _services.Single(x => x.ServiceType == typeof(IOcelotCache<FileConfiguration>));
|
|
var fileConfigCacheManager = _services.Single(x => x.ServiceType == typeof(ICacheManager<FileConfiguration>));
|
|
|
|
instance.Configuration.MaxRetries.ShouldBe(_maxRetries);
|
|
outputCache.ShouldNotBeNull();
|
|
ocelotConfigCache.ShouldNotBeNull();
|
|
ocelotConfigCacheManager.ShouldNotBeNull();
|
|
fileConfigCache.ShouldNotBeNull();
|
|
fileConfigCacheManager.ShouldNotBeNull();
|
|
}
|
|
|
|
private void WhenISetUpOcelotServices()
|
|
{
|
|
try
|
|
{
|
|
_ocelotBuilder = _services.AddOcelot(_configRoot);
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
_ex = e;
|
|
}
|
|
}
|
|
|
|
private void WhenISetUpCacheManager()
|
|
{
|
|
try
|
|
{
|
|
_ocelotBuilder.AddCacheManager(x =>
|
|
{
|
|
x.WithMaxRetries(_maxRetries);
|
|
x.WithDictionaryHandle();
|
|
});
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
_ex = e;
|
|
}
|
|
}
|
|
|
|
private void ThenAnExceptionIsntThrown()
|
|
{
|
|
_ex.ShouldBeNull();
|
|
}
|
|
}
|
|
}
|