mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-23 04:42:51 +08:00
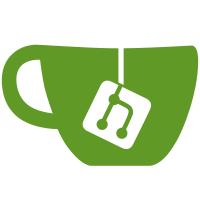
* copied everything from repos back to ocelot repo * added src projects to sln * removed all test projects that have no tests * added all test projects to sln * removed test not on master * merged unit tests * merged acceptance tests * merged integration tests * fixed namepaces * build script creates packages for all projects * updated docs to make sure no references to external repos that we will remove * +semver: breaking
36 lines
1005 B
C#
36 lines
1005 B
C#
namespace Ocelot.Provider.Eureka
|
|
{
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Threading.Tasks;
|
|
using ServiceDiscovery.Providers;
|
|
using Steeltoe.Common.Discovery;
|
|
using Values;
|
|
|
|
public class Eureka : IServiceDiscoveryProvider
|
|
{
|
|
private readonly IDiscoveryClient _client;
|
|
private readonly string _serviceName;
|
|
|
|
public Eureka(string serviceName, IDiscoveryClient client)
|
|
{
|
|
_client = client;
|
|
_serviceName = serviceName;
|
|
}
|
|
|
|
public Task<List<Service>> Get()
|
|
{
|
|
var services = new List<Service>();
|
|
|
|
var instances = _client.GetInstances(_serviceName);
|
|
|
|
if (instances != null && instances.Any())
|
|
{
|
|
services.AddRange(instances.Select(i => new Service(i.ServiceId, new ServiceHostAndPort(i.Host, i.Port), "", "", new List<string>())));
|
|
}
|
|
|
|
return Task.FromResult(services);
|
|
}
|
|
}
|
|
}
|