mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-23 20:12:51 +08:00
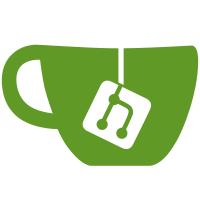
* #330 Fix and issue with the httpclient being cached on the url but not taking the http verb into accont. This caused an issue because if the same url but different verbs had handlers, the incorrect handler would be called * Amend so that UnableToCompleteRequestError returns 500 rather than 404. This error occur when a delegate handler throws an exception * Fix test that broke because of the change from 404 to 500 when delegate changes
147 lines
5.5 KiB
C#
147 lines
5.5 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.IO;
|
|
using System.Net;
|
|
using Microsoft.AspNetCore.Builder;
|
|
using Microsoft.AspNetCore.Hosting;
|
|
using Microsoft.AspNetCore.Http;
|
|
using Ocelot.Configuration.File;
|
|
using Shouldly;
|
|
using TestStack.BDDfy;
|
|
using Xunit;
|
|
|
|
namespace Ocelot.AcceptanceTests
|
|
{
|
|
public class SslTests : IDisposable
|
|
{
|
|
private IWebHost _builder;
|
|
private readonly Steps _steps;
|
|
private string _downstreamPath;
|
|
|
|
public SslTests()
|
|
{
|
|
_steps = new Steps();
|
|
}
|
|
|
|
[Fact]
|
|
public void should_dangerous_accept_any_server_certificate_validator()
|
|
{
|
|
int port = 51129;
|
|
|
|
var configuration = new FileConfiguration
|
|
{
|
|
ReRoutes = new List<FileReRoute>
|
|
{
|
|
new FileReRoute
|
|
{
|
|
DownstreamPathTemplate = "/",
|
|
DownstreamScheme = "https",
|
|
DownstreamHostAndPorts = new List<FileHostAndPort>
|
|
{
|
|
new FileHostAndPort
|
|
{
|
|
Host = "localhost",
|
|
Port = port,
|
|
}
|
|
},
|
|
UpstreamPathTemplate = "/",
|
|
UpstreamHttpMethod = new List<string> { "Get" },
|
|
DangerousAcceptAnyServerCertificateValidator = true
|
|
}
|
|
}
|
|
};
|
|
|
|
this.Given(x => x.GivenThereIsAServiceRunningOn($"https://localhost:{port}", "/", 200, "Hello from Laura", port))
|
|
.And(x => _steps.GivenThereIsAConfiguration(configuration))
|
|
.And(x => _steps.GivenOcelotIsRunning())
|
|
.When(x => _steps.WhenIGetUrlOnTheApiGateway("/"))
|
|
.Then(x => _steps.ThenTheStatusCodeShouldBe(HttpStatusCode.OK))
|
|
.And(x => _steps.ThenTheResponseBodyShouldBe("Hello from Laura"))
|
|
.BDDfy();
|
|
}
|
|
|
|
[Fact]
|
|
public void should_not_dangerous_accept_any_server_certificate_validator()
|
|
{
|
|
int port = 52129;
|
|
|
|
var configuration = new FileConfiguration
|
|
{
|
|
ReRoutes = new List<FileReRoute>
|
|
{
|
|
new FileReRoute
|
|
{
|
|
DownstreamPathTemplate = "/",
|
|
DownstreamScheme = "https",
|
|
DownstreamHostAndPorts = new List<FileHostAndPort>
|
|
{
|
|
new FileHostAndPort
|
|
{
|
|
Host = "localhost",
|
|
Port = port,
|
|
}
|
|
},
|
|
UpstreamPathTemplate = "/",
|
|
UpstreamHttpMethod = new List<string> { "Get" },
|
|
DangerousAcceptAnyServerCertificateValidator = false
|
|
}
|
|
}
|
|
};
|
|
|
|
this.Given(x => x.GivenThereIsAServiceRunningOn($"https://localhost:{port}", "/", 200, "Hello from Laura", port))
|
|
.And(x => _steps.GivenThereIsAConfiguration(configuration))
|
|
.And(x => _steps.GivenOcelotIsRunning())
|
|
.When(x => _steps.WhenIGetUrlOnTheApiGateway("/"))
|
|
.Then(x => _steps.ThenTheStatusCodeShouldBe(HttpStatusCode.InternalServerError))
|
|
.BDDfy();
|
|
}
|
|
|
|
private void GivenThereIsAServiceRunningOn(string baseUrl, string basePath, int statusCode, string responseBody, int port)
|
|
{
|
|
_builder = new WebHostBuilder()
|
|
.UseUrls(baseUrl)
|
|
.UseKestrel(options =>
|
|
{
|
|
options.Listen(IPAddress.Loopback, port, listenOptions =>
|
|
{
|
|
listenOptions.UseHttps("idsrv3test.pfx", "idsrv3test");
|
|
});
|
|
})
|
|
.UseContentRoot(Directory.GetCurrentDirectory())
|
|
.Configure(app =>
|
|
{
|
|
app.UsePathBase(basePath);
|
|
app.Run(async context =>
|
|
{
|
|
_downstreamPath = !string.IsNullOrEmpty(context.Request.PathBase.Value) ? context.Request.PathBase.Value : context.Request.Path.Value;
|
|
|
|
if(_downstreamPath != basePath)
|
|
{
|
|
context.Response.StatusCode = statusCode;
|
|
await context.Response.WriteAsync("downstream path didnt match base path");
|
|
}
|
|
else
|
|
{
|
|
context.Response.StatusCode = statusCode;
|
|
await context.Response.WriteAsync(responseBody);
|
|
}
|
|
});
|
|
})
|
|
.Build();
|
|
|
|
_builder.Start();
|
|
}
|
|
|
|
internal void ThenTheDownstreamUrlPathShouldBe(string expectedDownstreamPath)
|
|
{
|
|
_downstreamPath.ShouldBe(expectedDownstreamPath);
|
|
}
|
|
|
|
public void Dispose()
|
|
{
|
|
_builder?.Dispose();
|
|
_steps.Dispose();
|
|
}
|
|
}
|
|
}
|