mirror of
https://github.com/nsnail/Ocelot.git
synced 2025-04-20 07:42:51 +08:00
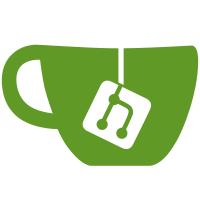
* feat: update to asp.net core 3.0 preview 9 * fix : AspDotNetLogger unittest * feat: update generic host and useMvc 1、Using 'UseMvc' to configure MVC is not supported while using Endpoint Routing https://github.com/aspnet/AspNetCore/issues/9542 2、 use IHost and IHostBuilder * feat : update .net core 3.0 rc1 * eureka extension * fixed logger formatter error * fixed synchronous operations are disallowed of ReadToEnd method * fix log tests * Flush method of FakeStream should do nothing * Update ContentTests.cs * Fixed ws tests * feat: delelte comment code * feat: update .net core 3.0 RTM * Update OcelotBuilderTests.cs * Update .travis.yml mono 6.0.0 and dotnet 3.0.100 * Update Ocelot.IntegrationTests.csproj update Microsoft.Data.SQLite 3.0.0 * Update .travis.yml * feat: remove FrameworkReference 1、 remove FrameworkReference 2、 update package * add appveyor configuration to use version of VS2019 with dotnet core 3 sdk support * update obsoleted SetCollectionValidator method * Swap out OpenCover for Coverlet * Bump Cake to 0.35.0 * Downgrade coveralls.net to 0.7.0 Fix disposing of PollConsul instance * Remove environment specific path separator * Do not return ReportGenerator on Mac/Linux * Remove direct dependency on IInternalConfiguration * Fix ordering of variable assignment * Fix broken tests * Fix acceptance tests for Consul
82 lines
3.0 KiB
C#
82 lines
3.0 KiB
C#
using Ocelot.Requester;
|
|
|
|
namespace Ocelot.ManualTest
|
|
{
|
|
using Microsoft.AspNetCore.Hosting;
|
|
using Microsoft.Extensions.Configuration;
|
|
using Microsoft.Extensions.DependencyInjection;
|
|
using Microsoft.Extensions.Logging;
|
|
using Ocelot.DependencyInjection;
|
|
using Ocelot.Middleware;
|
|
using System;
|
|
using System.IO;
|
|
using System.Net.Http;
|
|
using System.Threading;
|
|
using System.Threading.Tasks;
|
|
|
|
public class Program
|
|
{
|
|
public static void Main(string[] args)
|
|
{
|
|
new WebHostBuilder()
|
|
.UseKestrel()
|
|
.UseContentRoot(Directory.GetCurrentDirectory())
|
|
.ConfigureAppConfiguration((hostingContext, config) =>
|
|
{
|
|
config
|
|
.SetBasePath(hostingContext.HostingEnvironment.ContentRootPath)
|
|
.AddJsonFile("appsettings.json", true, true)
|
|
.AddJsonFile($"appsettings.{hostingContext.HostingEnvironment.EnvironmentName}.json", true, true)
|
|
.AddJsonFile("ocelot.json", false, false)
|
|
.AddEnvironmentVariables();
|
|
})
|
|
.ConfigureServices(s =>
|
|
{
|
|
s.AddAuthentication();
|
|
//.AddJwtBearer("TestKey", x =>
|
|
//{
|
|
// x.Authority = "test";
|
|
// x.Audience = "test";
|
|
//});
|
|
|
|
s.AddSingleton<QosDelegatingHandlerDelegate>((x, t) => new FakeHandler());
|
|
s.AddOcelot()
|
|
.AddDelegatingHandler<FakeHandler>(true);
|
|
// .AddCacheManager(x =>
|
|
// {
|
|
// x.WithDictionaryHandle();
|
|
// })
|
|
// .AddOpenTracing(option =>
|
|
// {
|
|
// option.CollectorUrl = "http://localhost:9618";
|
|
// option.Service = "Ocelot.ManualTest";
|
|
// })
|
|
// .AddAdministration("/administration", "secret");
|
|
})
|
|
.ConfigureLogging((hostingContext, logging) =>
|
|
{
|
|
logging.AddConfiguration(hostingContext.Configuration.GetSection("Logging"));
|
|
logging.AddConsole();
|
|
})
|
|
.UseIISIntegration()
|
|
.Configure(app =>
|
|
{
|
|
app.UseOcelot().Wait();
|
|
})
|
|
.Build()
|
|
.Run();
|
|
}
|
|
}
|
|
|
|
public class FakeHandler : DelegatingHandler
|
|
{
|
|
protected override async Task<HttpResponseMessage> SendAsync(HttpRequestMessage request, CancellationToken cancellationToken)
|
|
{
|
|
Console.WriteLine(request.RequestUri);
|
|
|
|
//do stuff and optionally call the base handler..
|
|
return await base.SendAsync(request, cancellationToken);
|
|
}
|
|
}
|
|
}
|