mirror of
https://github.com/nsnail/FreeSql.git
synced 2025-04-28 05:32:51 +08:00
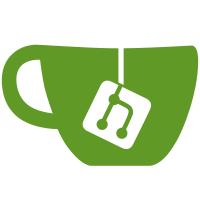
- 兼容 GetTableByEntity 有可能因为传入数组类型的错误; - 修复 UnitOfWork 事务创建逻辑 bug; - 增加 FreeSql.DbContext 扩展包; - 调整 UnitOfWork、DbContext 不提交时默认会回滚;
71 lines
1.3 KiB
C#
71 lines
1.3 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Threading.Tasks;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
|
|
namespace dbcontext_01.Controllers
|
|
{
|
|
[Route("api/[controller]")]
|
|
[ApiController]
|
|
public class ValuesController : ControllerBase
|
|
{
|
|
|
|
IFreeSql _orm;
|
|
public ValuesController(SongContext songContext, IFreeSql orm) {
|
|
|
|
_orm = orm;
|
|
|
|
}
|
|
|
|
// GET api/values
|
|
[HttpGet]
|
|
async public Task<string> Get()
|
|
{
|
|
|
|
long id = 0;
|
|
|
|
try {
|
|
using (var ctx = new SongContext()) {
|
|
|
|
id = await ctx.Songs.Insert(new Song { }).ExecuteIdentityAsync();
|
|
|
|
var item = await ctx.Songs.Select.Where(a => a.Id == id).FirstAsync();
|
|
|
|
throw new Exception("回滚");
|
|
|
|
}
|
|
} catch {
|
|
var item = await _orm.Select<Song>().Where(a => a.Id == id).FirstAsync();
|
|
|
|
throw;
|
|
}
|
|
}
|
|
|
|
// GET api/values/5
|
|
[HttpGet("{id}")]
|
|
public ActionResult<string> Get(int id)
|
|
{
|
|
return "value";
|
|
}
|
|
|
|
// POST api/values
|
|
[HttpPost]
|
|
public void Post([FromBody] string value)
|
|
{
|
|
}
|
|
|
|
// PUT api/values/5
|
|
[HttpPut("{id}")]
|
|
public void Put(int id, [FromBody] string value)
|
|
{
|
|
}
|
|
|
|
// DELETE api/values/5
|
|
[HttpDelete("{id}")]
|
|
public void Delete(int id)
|
|
{
|
|
}
|
|
}
|
|
}
|