mirror of
https://github.com/nsnail/FreeSql.git
synced 2025-04-22 02:32:50 +08:00
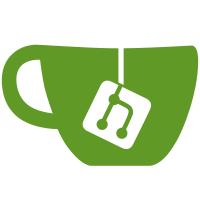
- 修复 ToList 选择指定对象时,应附加所有字段查询返回; - 修复 Lazy 延时类与实体关系冲突 bug; - 修复 附加对象读取时,记录为空应该返回null,而不是返回非null(字段默认值)对象;
65 lines
1.8 KiB
C#
65 lines
1.8 KiB
C#
using System;
|
|
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using System.Data;
|
|
using System.Data.Common;
|
|
using System.Linq;
|
|
using System.Linq.Expressions;
|
|
using System.Text;
|
|
using System.Threading.Tasks;
|
|
|
|
namespace FreeSql {
|
|
public abstract class DbSet<TEntity> where TEntity : class {
|
|
|
|
protected DbContext _ctx;
|
|
|
|
public ISelect<TEntity> Select => _ctx._fsql.Select<TEntity>().WithTransaction(_ctx.GetOrBeginTransaction(false));
|
|
|
|
public IInsert<TEntity> Insert(TEntity source) => _ctx._fsql.Insert<TEntity>(source).WithTransaction(_ctx.GetOrBeginTransaction());
|
|
public IInsert<TEntity> Insert(TEntity[] source) => _ctx._fsql.Insert<TEntity>(source).WithTransaction(_ctx.GetOrBeginTransaction());
|
|
public IInsert<TEntity> Insert(IEnumerable<TEntity> source) => _ctx._fsql.Insert<TEntity>(source).WithTransaction(_ctx.GetOrBeginTransaction());
|
|
|
|
public IUpdate<TEntity> Update => _ctx._fsql.Update<TEntity>().WithTransaction(_ctx.GetOrBeginTransaction());
|
|
public IDelete<TEntity> Delete => _ctx._fsql.Delete<TEntity>().WithTransaction(_ctx.GetOrBeginTransaction());
|
|
|
|
//protected Dictionary<string, TEntity> _vals = new Dictionary<string, TEntity>();
|
|
//protected tableinfo
|
|
|
|
//public void Add(TEntity source) {
|
|
|
|
//}
|
|
//public void AddRange(TEntity[] source) {
|
|
|
|
//}
|
|
//public void AddRange(IEnumerable<TEntity> source) {
|
|
|
|
//}
|
|
//public void Update(TEntity source) {
|
|
|
|
//}
|
|
//public void UpdateRange(TEntity[] source) {
|
|
|
|
//}
|
|
//public void UpdateRange(IEnumerable<TEntity> source) {
|
|
|
|
//}
|
|
//public void Remove(TEntity source) {
|
|
|
|
//}
|
|
//public void RemoveRange(TEntity[] source) {
|
|
|
|
//}
|
|
//public void RemoveRange(IEnumerable<TEntity> source) {
|
|
|
|
//}
|
|
|
|
}
|
|
|
|
internal class BaseDbSet<TEntity> : DbSet<TEntity> where TEntity : class {
|
|
|
|
public BaseDbSet(DbContext ctx) {
|
|
_ctx = ctx;
|
|
}
|
|
}
|
|
}
|